A C++ list iterator allows you to traverse and manipulate elements in a `std::list` container efficiently, using functions like `begin()`, `end()`, and `++` to navigate through the list.
Here’s a code snippet demonstrating the use of a list iterator in C++:
#include <iostream>
#include <list>
int main() {
std::list<int> myList = {1, 2, 3, 4, 5};
for (std::list<int>::iterator it = myList.begin(); it != myList.end(); ++it) {
std::cout << *it << " ";
}
return 0;
}
Understanding the C++ List
The C++ list is a part of the Standard Template Library (STL) that provides a flexible structure for storing collections of data. Lists are characterized by their ability to grow and shrink dynamically, which makes them particularly suitable for scenarios where frequent insertions and deletions are required. Unlike arrays, lists do not require contiguous memory, allowing for more efficient memory use and management.
One of the primary advantages of using a list over other STL containers, like vectors, is bidirectional access. This means that elements can be accessed and modified in both forward and backward directions, making it an excellent choice for algorithms that require traversal in either direction.
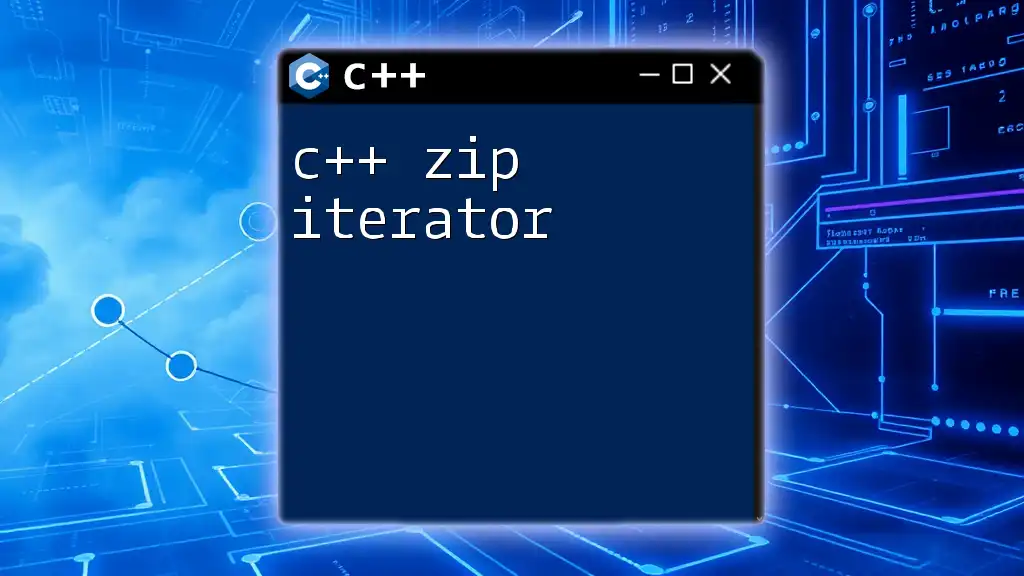
What is a List Iterator in C++?
A list iterator is specifically designed to navigate through a list in C++. Iterators serve as generalized pointers tailored for container structures, including lists. They allow access to the elements of the list without exposing the underlying structure, promoting better encapsulation and abstraction.
The main differences between iterators, pointers, and references in C++ include:
- Iterators: Generic and can work with various container types.
- Pointers: Can perform arithmetic operations and point to raw memory.
- References: Aliases to existing variables and do not have their own memory address.
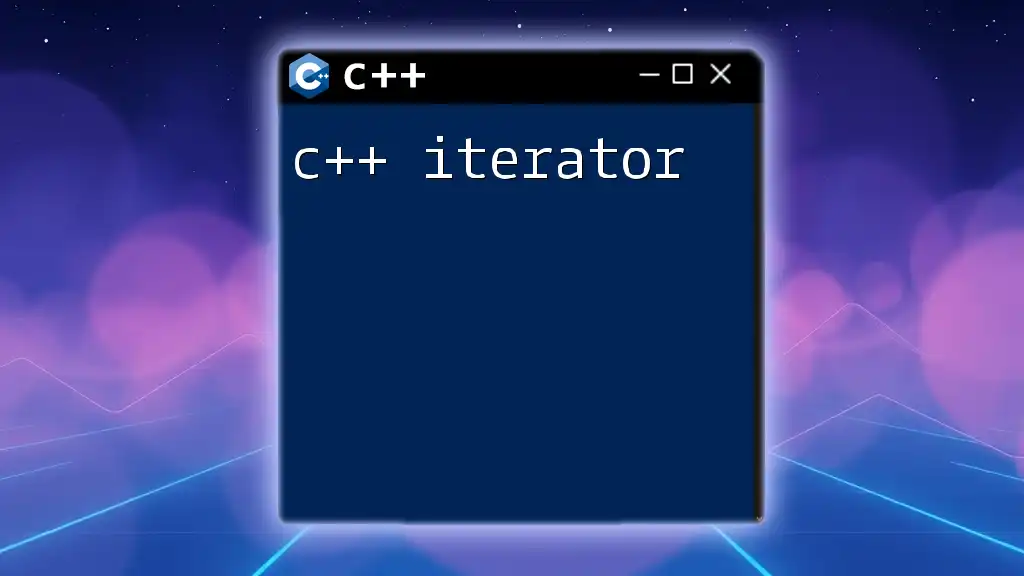
Basic Operations with C++ List Iterators
Creating and manipulating a C++ list is straightforward. To demonstrate using a cpp list iterator, we first need to initialize a list:
std::list<int> myList = {1, 2, 3, 4, 5};
With the list in place, we can perform basic operations such as adding and removing elements. For instance, you could add an element to the list using:
myList.push_back(6); // Adds 6 to the end of the list
And to remove an element, you might use:
myList.remove(3); // Removes the first occurrence of 3 from the list
Creating a List Iterator
Now that we have our list established, creating a cpp list iterator is simple. Here's how you can declare and initialize a list iterator:
std::list<int>::iterator it = myList.begin();
This line of code sets the iterator `it` to point to the beginning of `myList`.
Navigating through a List using Iterators
Navigating through the list with an iterator involves incrementing or decrementing it. For instance, if you want to move the iterator to the next element, you can do so by incrementing it:
++it; // Move to the next element
Conversely, if you need to step back to the previous element, decrementing the iterator is just as simple:
--it; // Move back to the previous element
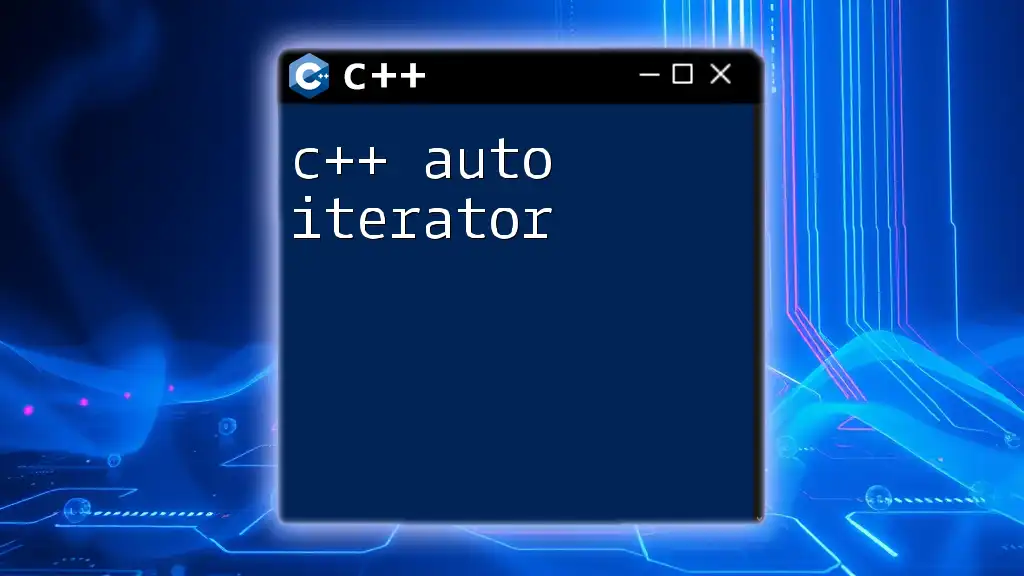
Iterating Over a List in C++
To effectively utilize a cpp list iterator, you can implement it within loops for traversal. One common approach is using a for loop:
for (std::list<int>::iterator it = myList.begin(); it != myList.end(); ++it) {
std::cout << *it << " "; // Dereferencing to get the value
}
Another method is through a while loop, as shown below:
std::list<int>::iterator it = myList.begin();
while (it != myList.end()) {
std::cout << *it << " ";
++it;
}
Both examples demonstrate how to effectively traverse through a list and access its values.
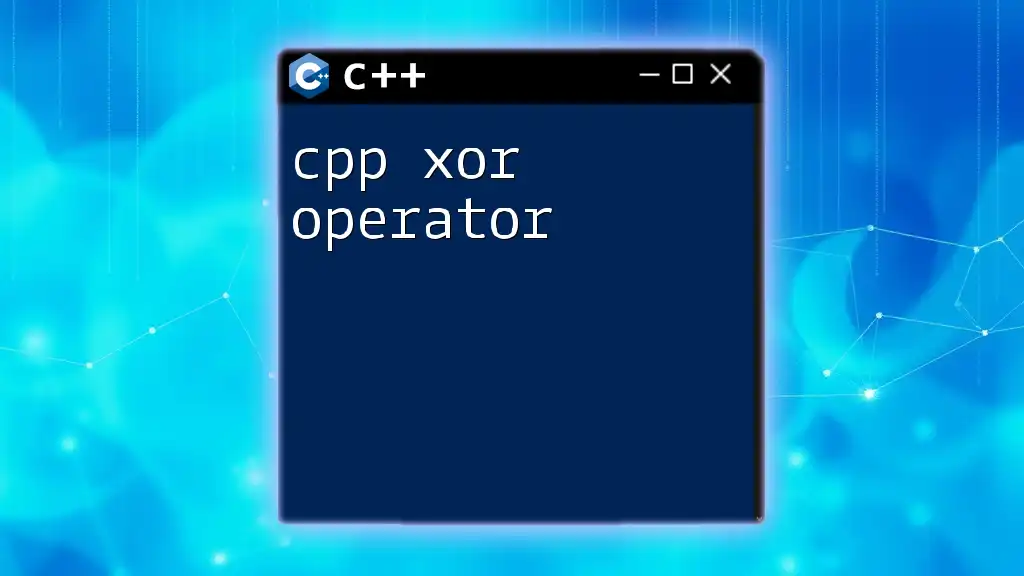
Advanced List Iterator Features
Reverse Iterators
A unique feature of C++ iterators is the ability to use reverse iterators. These special iterators allow for backward traversal of the list. Here's how to create and use a reverse iterator:
std::list<int>::reverse_iterator rit = myList.rbegin();
for (; rit != myList.rend(); ++rit) {
std::cout << *rit << " ";
}
By using reverse iterators, you gain the flexibility to access elements from the end to the beginning, which can be beneficial in various programming contexts.
Const Iterators
Another important type of iterator is the const iterator. This type allows you to traverse the list without risking modification of its elements. Using const iterators is a best practice in scenarios where you want to ensure that the list remains unchanged during the iteration:
std::list<int>::const_iterator cit = myList.cbegin();
Employing const iterators enhances code safety by preventing accidental alterations to data.
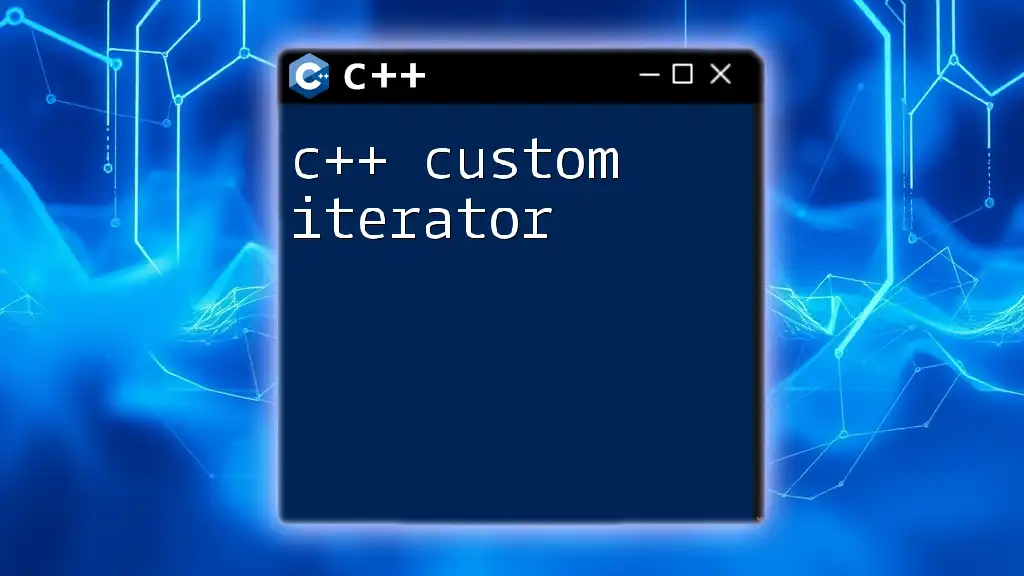
Common Mistakes When Using C++ List Iterators
While working with cpp list iterators, it’s essential to be aware of common pitfalls:
- Forgetting bounds checking can lead to dereferencing invalid memory, which causes runtime errors.
- Modifying the list during iteration may invalidate the iterator, resulting in unexpected behavior or crashes.
- Dereferencing invalid iterators, such as those that point to the `end()` of the list, can lead to program failures.
Being mindful of these issues can improve the stability and reliability of your code.
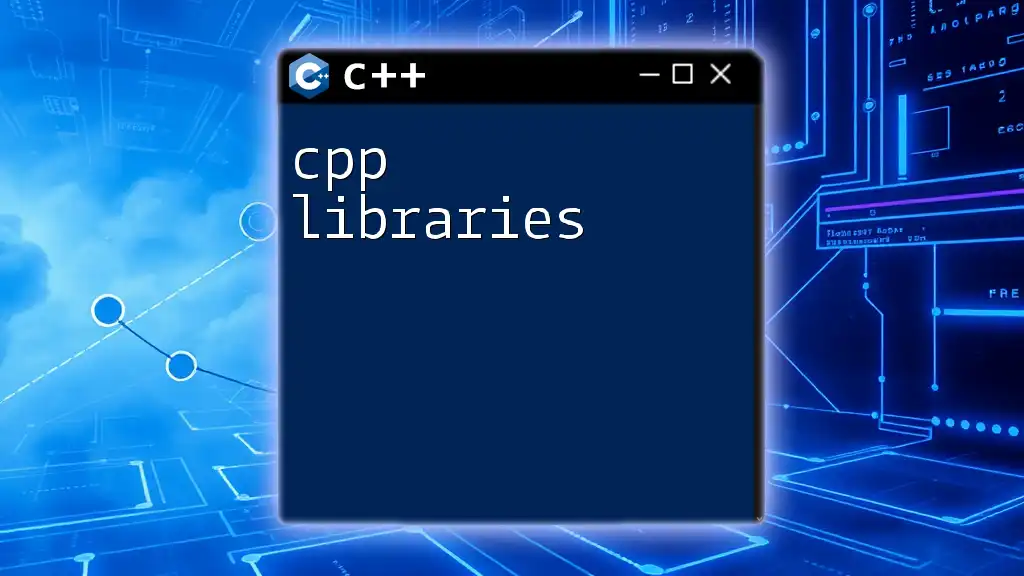
Performance Considerations
Understanding the time complexity of list iterator operations is crucial for writing efficient code. Iterators support both forward and backward movement in constant time, which is advantageous for many algorithms. However, if your application requires frequent access or modification of elements by index, consider using other containers, such as vectors, instead.
Selecting the right iterator type for your application can significantly affect performance and should be guided by the specific needs of your data structure and operations.
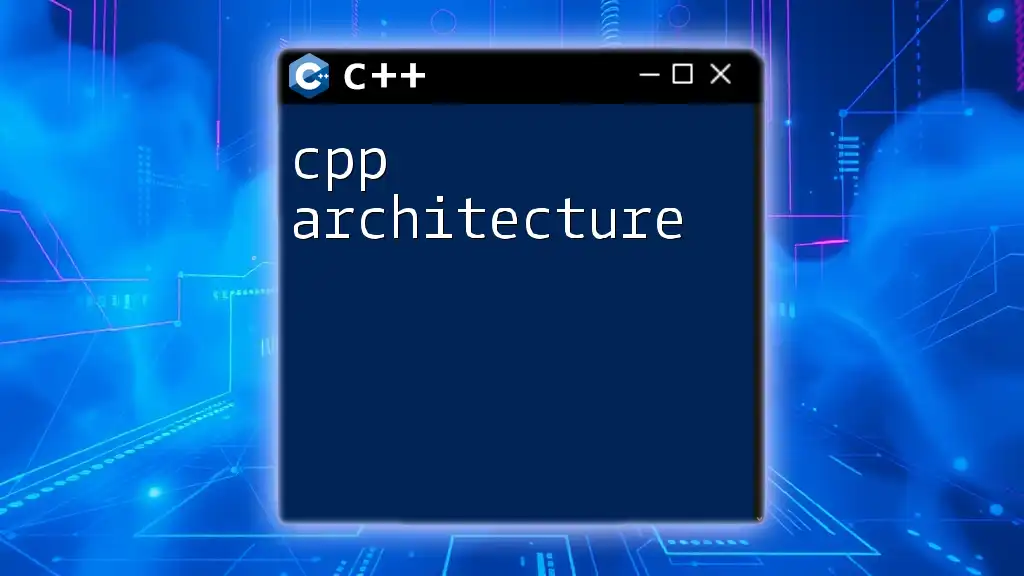
Conclusion
In summary, mastering the cpp list iterator opens the door to efficient and effective manipulation of list elements. By understanding how to create, navigate, and utilize different types of iterators, you'll be equipped with the skills needed to leverage C++ lists and STL effectively in your projects.
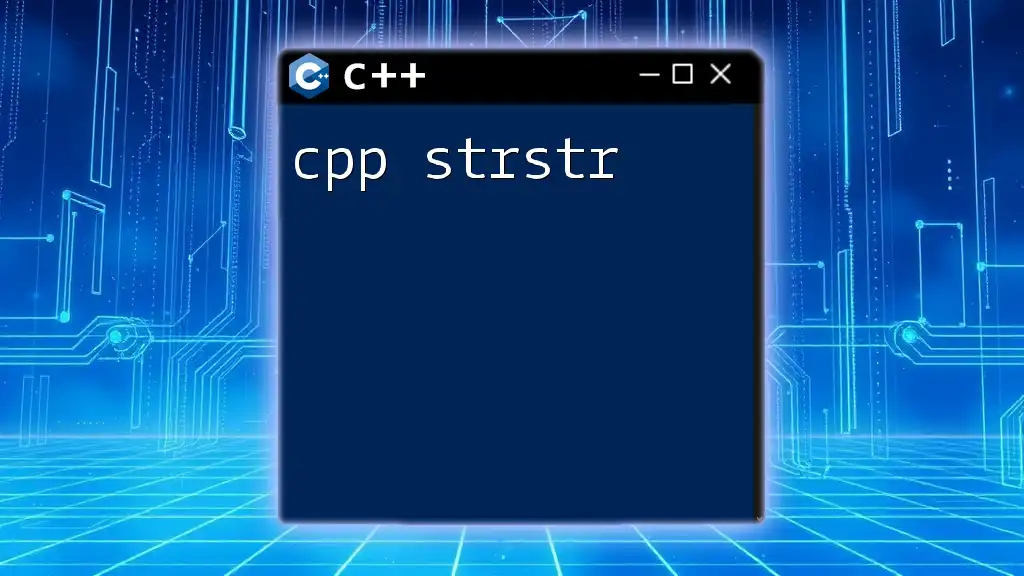
Additional Resources
To further enhance your understanding and capabilities with C++ and its STL, consider exploring online documentation, recommended literature, and community forums. Engaging with these resources can provide deeper insights into best practices and advanced features, helping you become proficient in using iterators and the C++ Standard Template Library.