The C++ Institute is dedicated to providing efficient and concise tutorials on C++ commands, enabling learners to grasp essential programming concepts quickly.
Here’s a simple example of a C++ command that outputs "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is CPP Institute?
The CPP Institute is a dedicated platform designed to empower individuals with the essential skills needed to master C++. As the demand for proficient C++ developers continues to rise in various sectors, becoming adept at this robust programming language is not only advantageous but often necessary.
Why C++?
C++ is a versatile language used in a myriad of applications, such as system software, game development, real-time systems, and even high-performance computing. Companies like Microsoft, Google, and Adobe rely on C++ for its powerful capabilities and efficiency. By learning C++, you open yourself up to a plethora of career opportunities in software development, game engineering, and more.
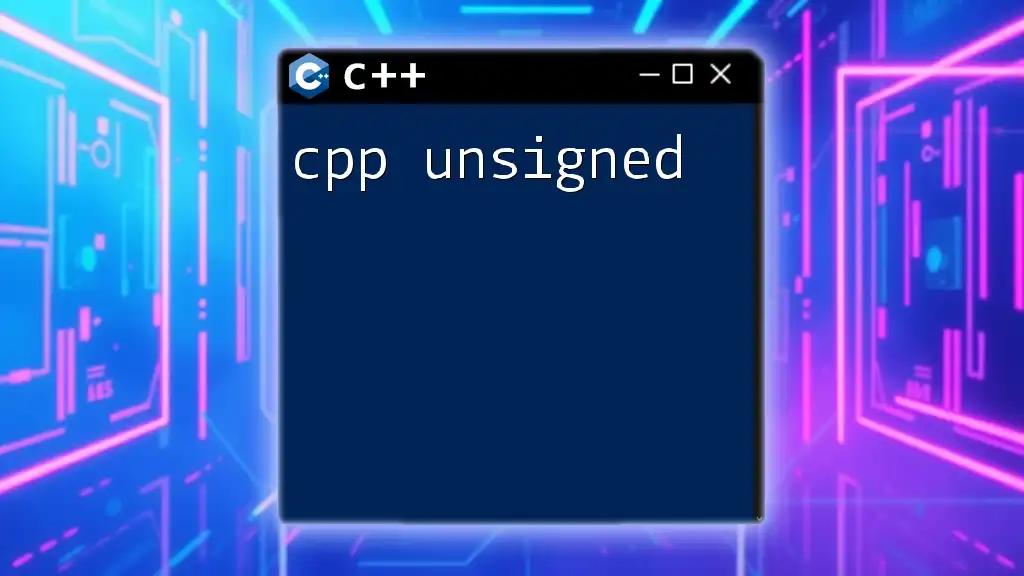
Structure of the CPP Institute
Course Design
The curriculum at the CPP Institute is meticulously structured to cater to learners of all levels. The courses are designed to ensure a smooth learning journey from basic concepts to complex applications. Each course progresses logically, with hands-on projects included at every level for practical experience.
Learning Formats
Online Learning
One of the standout features of the CPP Institute is the flexibility of the online learning format. This allows learners to access video tutorials, quizzes, and interactive exercises at their convenience. You can learn at your own pace, ensuring that you grasp each concept thoroughly before moving on.
In-Person Classes
For those who thrive in a more traditional learning environment, the CPP Institute also offers in-person classes. This format benefits students with direct interaction with instructors, fostering a collaborative environment where questions are encouraged, and hands-on coding experiences take precedence.
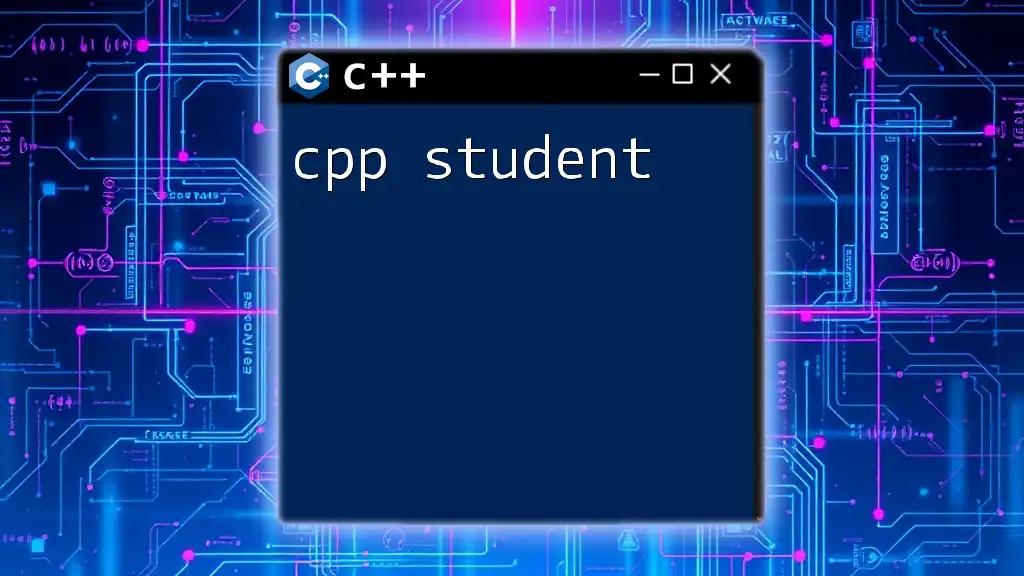
Fundamentals of C++ Programming
Overview of C++
C++ is an extension of the C programming language, adding object-oriented features. It has been widely adopted due to its ability to provide control over system resources and memory management, a crucial necessity for building complex software applications.
Setting Up Your Development Environment
To get started with C++, you must first set up your development environment. Here’s a step-by-step guide to installing popular IDEs and compilers:
-
Visual Studio: A widely used IDE that supports C++. Download and install it from the official Microsoft website. Select the C++ workload during installation for complete support.
-
Code::Blocks: An open-source IDE that provides all the basic functionalities needed for C++ development. You can download it from the Code::Blocks official site.
-
g++ (GNU Compiler): Ensure you have this compiler installed for compiling C++ programs on Windows, Mac, or Linux environments.
Understanding Basic Syntax
Data Types and Variables
C++ supports several built-in data types. Understanding how to declare and use them is fundamental.
int age = 30;
float height = 5.9;
char initial = 'J';
In the example above, you see how different data types can be declared, indicating the versatility of C++ in handling various kinds of data.
Operators
C++ has a robust set of operators to perform operations on variables. The arithmetic operators like `+`, `-`, `*`, and `/` allow you to do mathematical operations, while logical operators like `&&` (AND) and `||` (OR) help in decision making.
Control Structures
Conditional Statements
Control the flow of your programs effectively by using conditional statements such as `if`, `else if`, and `switch`. These statements allow your programs to make decisions based on conditions.
if (age > 18) {
cout << "Adult";
} else {
cout << "Minor";
}
In this example, the output is dependent on the variable's value, demonstrating the concept of control flow.
Loops
C++ is equipped with several types of loops to execute parts of your code multiple times. The `for`, `while`, and `do-while` loops are essential for performing repetitive tasks.
for (int i = 0; i < 5; i++) {
cout << i;
}
This simple `for` loop prints numbers from 0 to 4, showcasing how loops serve to minimize redundancy in your code.
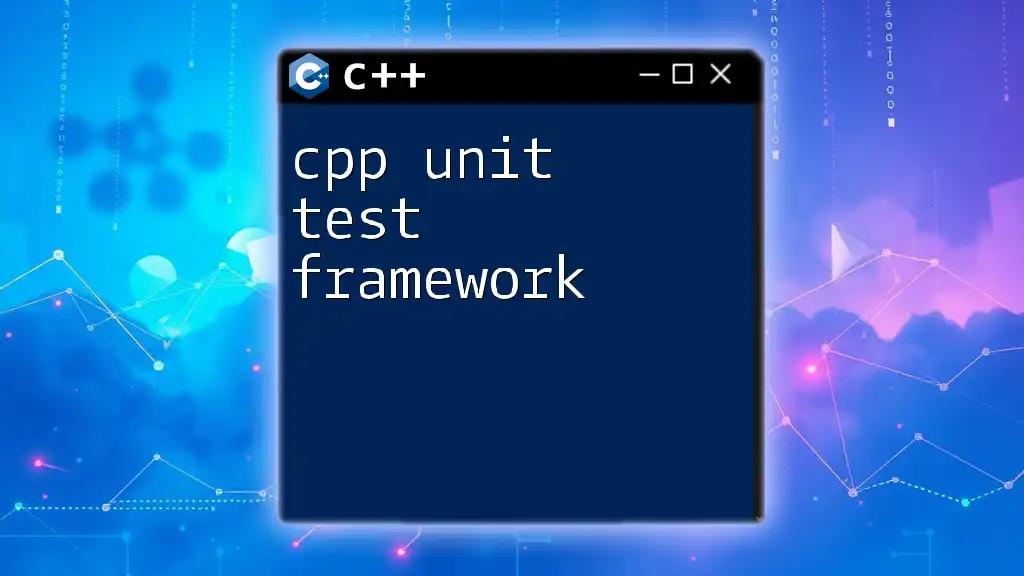
Advanced C++ Concepts
Object-Oriented Programming (OOP)
The principles of OOP—Encapsulation, Inheritance, and Polymorphism—are core to understanding C++.
- Encapsulation: This is the bundling of data and methods into a single unit, known as a class, which restricts direct access to certain components.
class Animal {
public:
void speak() {
cout << "Animal speaks";
}
};
- Inheritance: This allows a new class (derived class) to inherit properties and behaviors from an existing class (base class).
Templates and Generic Programming
Templates provide a mechanism for creating generic functions and classes in C++. This flexibility allows for type-safe functions and classes that can operate with any data type.
template <typename T>
T add(T a, T b) {
return a + b;
}
In this example, the `add` function can accept any data type, demonstrating the power of C++ templates.
STL (Standard Template Library)
The STL is a powerful library that provides many ready-to-use classes and functions. It enhances productivity and code efficiency. Key components include:
-
Vectors: Dynamic arrays that can resize automatically.
-
Lists: Doubly linked lists that allow for efficient insertion and deletion.
vector<int> numbers = {1, 2, 3, 4};
This example initiates a vector of integers that can be manipulated easily, showcasing the dynamic capabilities of STL.
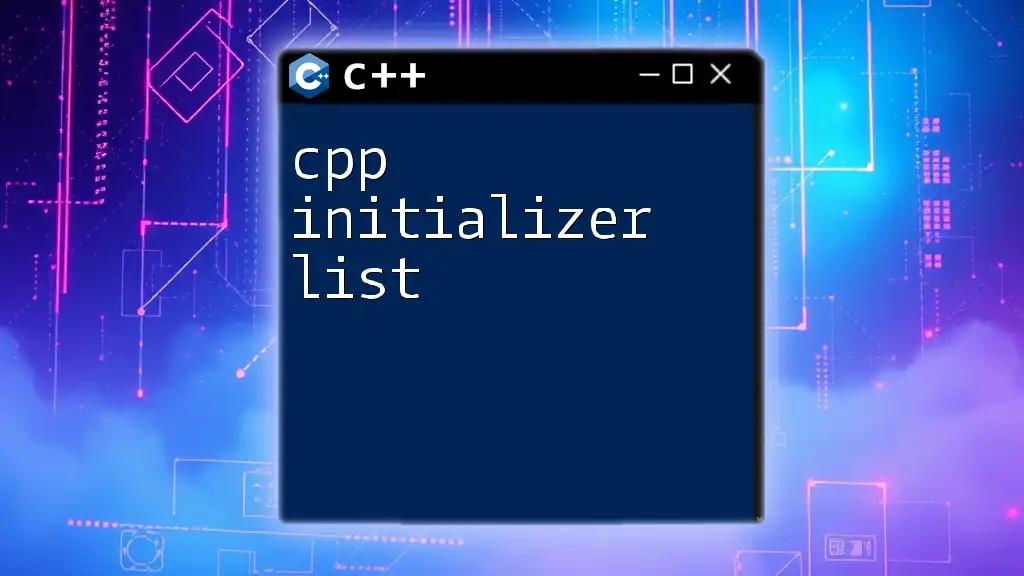
Practical Applications and Projects
Real-world Projects to Apply C++ Skills
Hands-on projects are critical for reinforcing your learning. You could undertake projects such as:
-
Game Development: Create simple games like Tic-Tac-Toe or more complex ones using graphics libraries.
-
System Software: Develop system utilities that enhance operating system functionalities.
-
Mobile Applications: Leverage C++ to build performance-centric mobile applications.
Contributing to Open Source
Engaging with open-source projects can deepen your understanding and give you real-world experience. You can find many C++ projects on platforms like GitHub. Contributing not only builds your skills but also provides networking opportunities within the developer community.
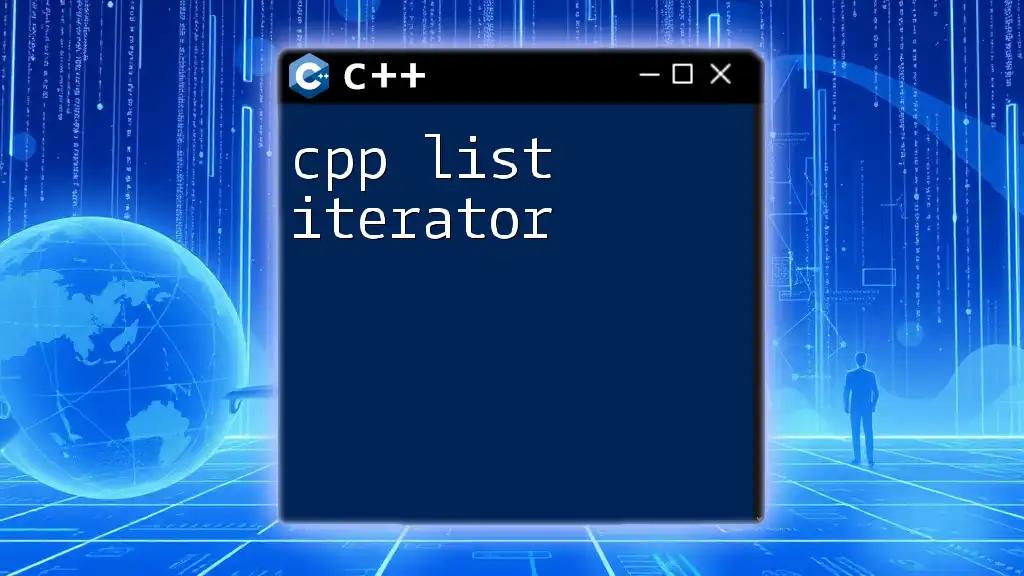
Resources and Tools
Recommended Reading and Online Resources
To further your understanding of C++, consider these resources:
- Books such as "C++ Primer" by Stanley B. Lippman.
- Online platforms like Coursera, Udacity, and Codecademy for structured learning.
Useful Tools and IDEs for C++ Development
- g++/Clang: Command-line compilers for testing and running your C++ code.
- JetBrains CLion: An intelligent cross-platform IDE that makes C++ development efficient and enjoyable.
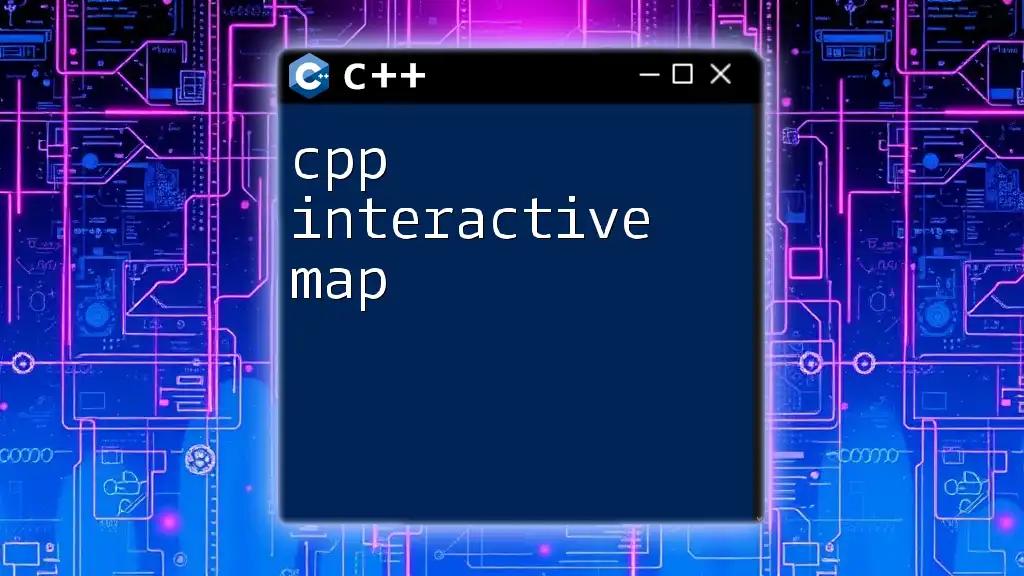
Join the CPP Institute
How to Enroll
Enrolling in the CPP Institute is straightforward. Visit the website, select your desired course, and follow the prompts to create an account and register for classes.
Community and Networking
Being part of the C++ community can be incredibly beneficial. Joining forums like Stack Overflow or Reddit’s r/cpp can connect you with other learners and professionals, providing invaluable support and guidance as you navigate your learning journey.
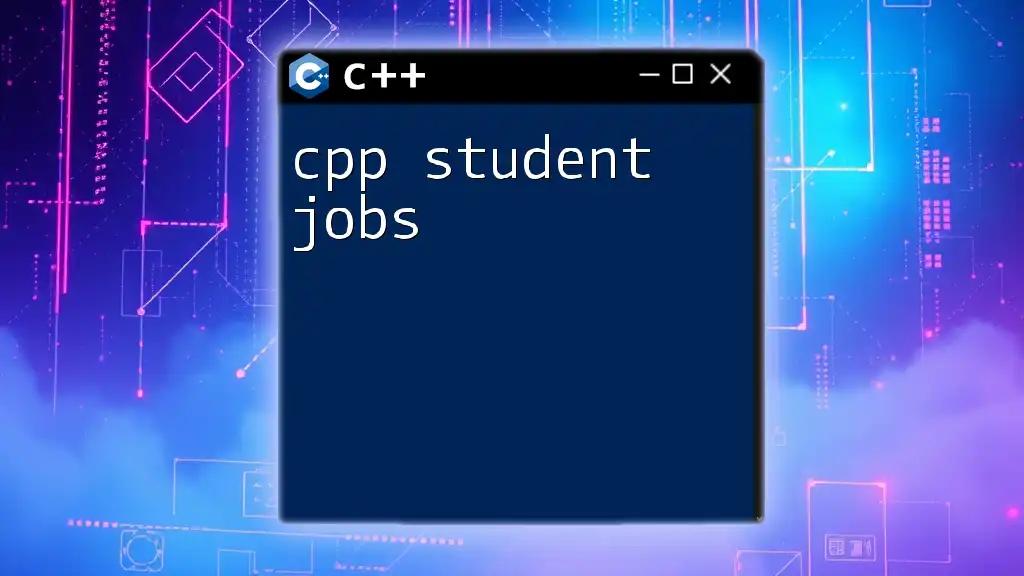
Conclusion
Learning C++ through the CPP Institute is a pathway to unlocking numerous professional opportunities in software development and beyond. By engaging with both foundational and advanced concepts, alongside practical applications, you will not only enhance your programming skills but also position yourself as a competitive candidate in the tech industry. Take that crucial first step today towards becoming a proficient C++ developer!