A `bitset` in C++ is a class template that provides a way to represent a fixed-size sequence of bits and offers various operations to manipulate them efficiently.
Here's a simple example of using `std::bitset`:
#include <bitset>
#include <iostream>
int main() {
std::bitset<8> bits("10110011");
std::cout << "Original bits: " << bits << std::endl;
bits.flip(); // Flips all bits
std::cout << "Flipped bits: " << bits << std::endl;
return 0;
}
Understanding C++ Bitset
What is a Bitset?
A bitset in C++ allows for the efficient representation of a collection of bits using a single object. It's particularly useful for applications where you need to track multiple Boolean values, such as flags or states. The major advantage of using a bitset is its compact representation and the ease with which bit-level operations can be performed.
Importance in Programming
Bitsets can manage data in a way that reduces memory footprint compared to using a separate Boolean variable for each flag. This is especially useful in scenarios like configuration options, state management, or representing sets in competitive programming.
What is a Bitset in C++?
In C++, a `bitset` is defined in the `<bitset>` header file. It allows the storage of a sequence of bits in a compact manner. For example, a `bitset<8>` can hold 8 bits, which can represent numbers from 0 to 255.
Characteristics of C++ Bitsets
C++ bitsets are:
- Fixed in size: Once defined (e.g., `bitset<8>`), the size cannot change.
- Memory efficient: They enable effective use of memory, particularly in handling large collections of bits.
- Performance-oriented: Operations on bitsets are generally faster compared to equivalent operations on other data structures, like `std::vector<bool>`.
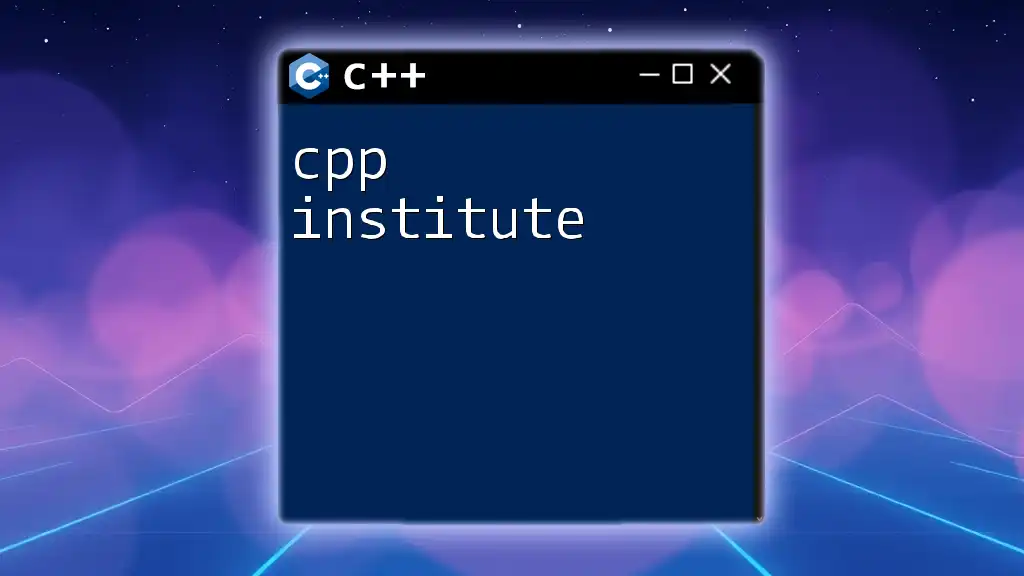
Creating and Initializing Bitsets
How to Create a Bitset
To create a bitset, you start by including the `<bitset>` header. The syntax is straightforward:
#include <bitset>
std::bitset<8> b1; // Creates an 8-bit bitset initialized to 0
In this example, `b1` is an 8-bit bitset, meaning it can hold the values 00000000 to 11111111.
Initializing Bitsets
Bitsets can be initialized in various ways:
- Using a binary string:
std::bitset<8> b2("10101010"); // Initializes with the binary representation 10101010
- Using an integer:
std::bitset<8> b3(170); // 170 in binary is 10101010
Both methods will result in bitsets holding the same bits but initialized through different input types.
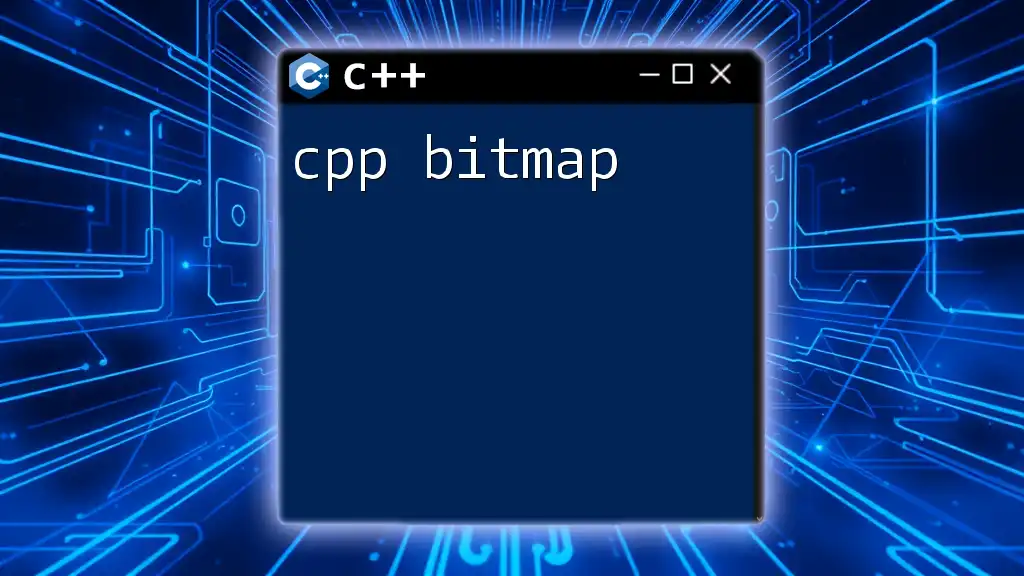
Basic Operations on C++ Bitsets
Accessing Bits
You can access individual bits within a bitset using the `[]` operator, where indexing starts from 0.
b1[2] = 1; // Set the 3rd bit (zero-based) to 1
For a quick check on whether a bit is set, you can use the `.test()` method:
bool isSet = b2.test(2); // Returns true if the 3rd bit is set
Modifying Bits
Bitsets allow you to manipulate bits directly using:
- .set():
b1.set(1); // Set the 2nd bit to 1
- .reset():
b1.reset(3); // Set the 4th bit to 0
- .flip(): This method toggles the state of all bits.
b1.flip(); // Flip all bits
Logical Operations on Bitsets
You can perform bitwise operations on bitsets just like on integers. For instance:
std::bitset<8> b4("11110000");
std::bitset<8> b5 = b2 & b4; // Bitwise AND
std::bitset<8> b6 = b2 | b4; // Bitwise OR
std::bitset<8> b7 = b2 ^ b4; // Bitwise XOR
These operations provide a powerful way to combine or compare bitsets in a highly efficient way.
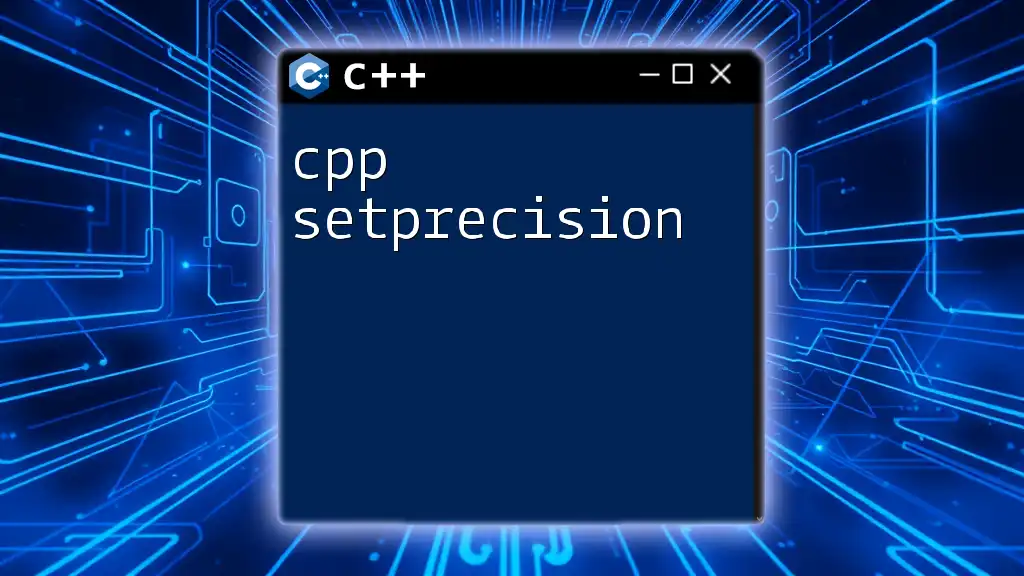
Advanced Bitset Techniques
Converting Between Data Types
C++ bitsets can easily convert to and from different types. To convert a bitset to its string representation, use:
std::string bitstring = b2.to_string(); // Convert to string
For numerical conversions, you can convert a bitset to an unsigned long:
unsigned long number = b2.to_ulong(); // Convert to unsigned long
Iterating Over Bitsets
If you need to traverse bits within a bitset, you can use a range-based for loop:
for (std::size_t i = 0; i < b2.size(); ++i) {
std::cout << b2[i]; // Outputs 10101010
}
This style of iteration allows for succinct and readable code when analyzing or modifying bits.
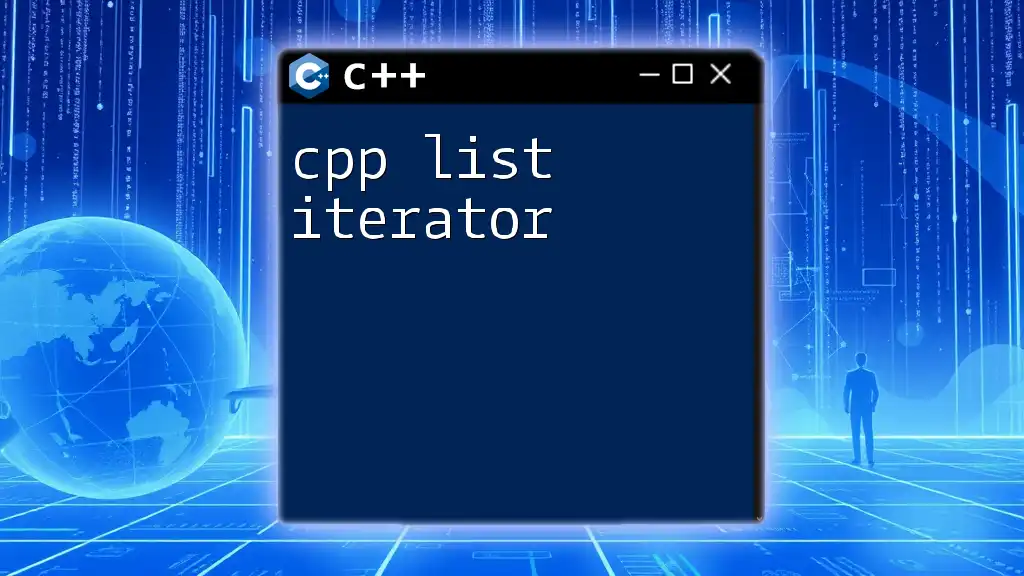
Use Cases of C++ Bitset
Game Development
In game development, you might need to manage multiple game states and options efficiently. A `bitset` makes it easy to toggle states like "isJumping", "isRunning", or "isShooting" without having a long list of Boolean variables.
Networking Protocols
When dealing with networking protocols, you can use bitsets to manage flags in data packets. Each bit can represent a specific feature or option within the protocol, allowing for efficient communication without overhead.
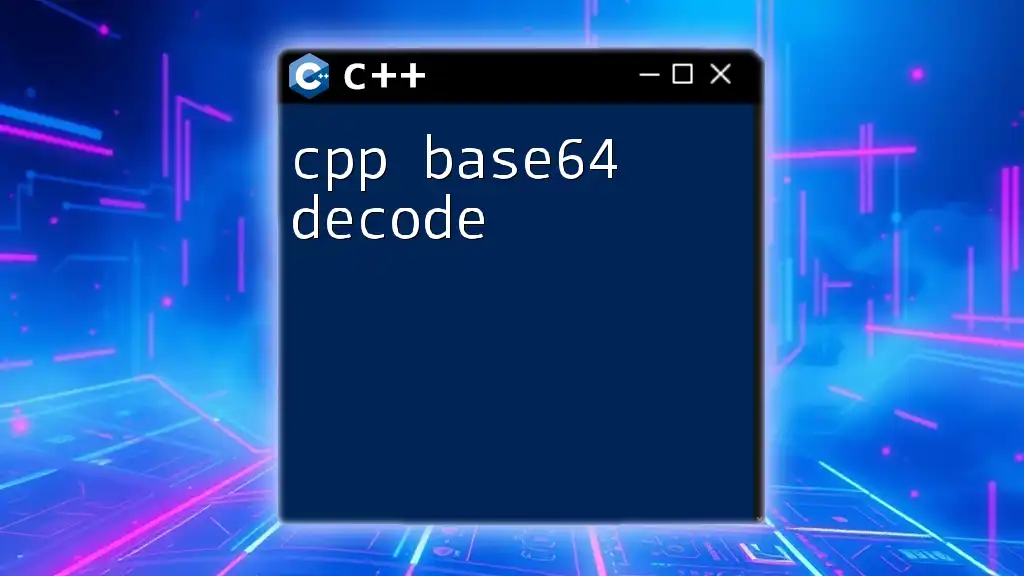
Performance Considerations
When considering data structures for managing flags and Boolean states in C++, bitsets are generally superior due to their performance and memory efficiency compared to other alternatives like `std::vector<bool>`.
However, bitsets are fixed-size, so they may not suit all applications. They are ideal when the number of flagged conditions is known and small but may not be the best choice for dynamically sized data structures.
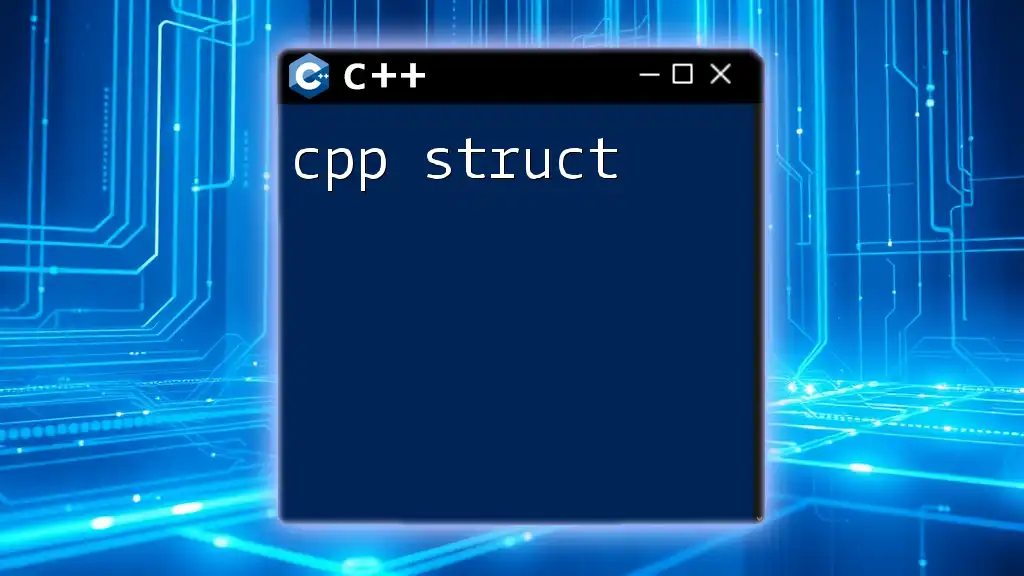
Conclusion
C++ bitsets represent a powerful and flexible tool for managing bits efficiently in your code. With operations that allow quick manipulation and examination of sets of bits, they can be integral in numerous applications ranging from game programming to data processing. Understanding how to leverage a `cpp bitset` will enhance your ability to write optimized C++ code quickly and effectively.
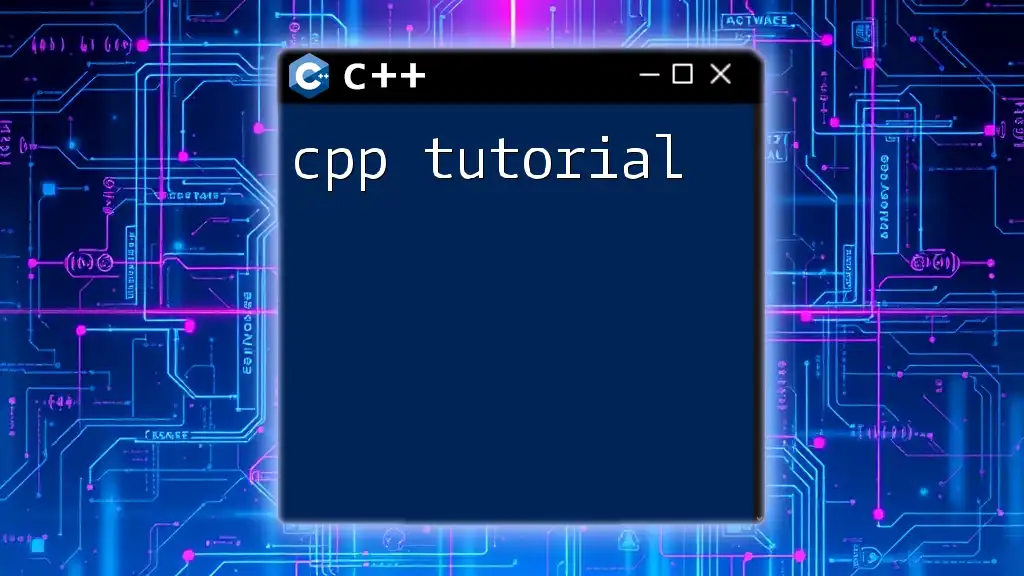
References and Further Reading
For additional insights and advanced techniques, consider exploring resources like:
- C++ Standard Library documentation
- Books on C++ programming
- Online forums and communities dedicated to C++ development