Base64 decoding in C++ can be achieved using libraries such as OpenSSL or custom implementations, allowing you to convert encoded data back into its original binary format.
Here's a simple example using your own implementation for Base64 decoding in C++:
#include <iostream>
#include <string>
#include <vector>
std::string base64_decode(const std::string &in) {
std::string out;
std::vector<int> T(256, -1);
for (int i = 0; i < 64; i++) T["ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/"[i]] = i;
int val = 0, valb = -8;
for (unsigned char c : in) {
if (T[c] == -1) break; // Ignore invalid characters
val = (val << 6) + T[c]; valb += 6;
if (valb >= 0) {
out.push_back(char((val >> valb) & 0xFF));
valb -= 8;
}
}
return out;
}
int main() {
std::string encoded = "SGVsbG8gV29ybGQ="; // Base64 encoded string
std::string decoded = base64_decode(encoded);
std::cout << "Decoded: " << decoded << std::endl;
return 0;
}
This code defines a function to decode a Base64-encoded string, showcasing how to handle encoding in C++.
Understanding Base64 Encoding
What is Base64?
Base64 is an encoding scheme that represents binary data in an ASCII string format. It converts data into a set of 64 characters that are compatible with most systems, making it especially useful for transmitting data over media that may not support binary formats. The Base64 character set consists of uppercase letters (A-Z), lowercase letters (a-z), digits (0-9), and two additional characters typically used as `+` and `/`, while the `=` character acts as padding for alignment.
How Base64 Works
The encoding process converts data into six-bit groups, allowing for compression of binary data into a string format. In a typical scenario, each set of three bytes is transformed into four Base64 characters. For instance, if you encode the string "ABC", it converts to `QUJD` in Base64 representation. The padding character `=` is utilized to properly encode data whose total byte length is not a multiple of three, ensuring proper alignment in the decoding phase.
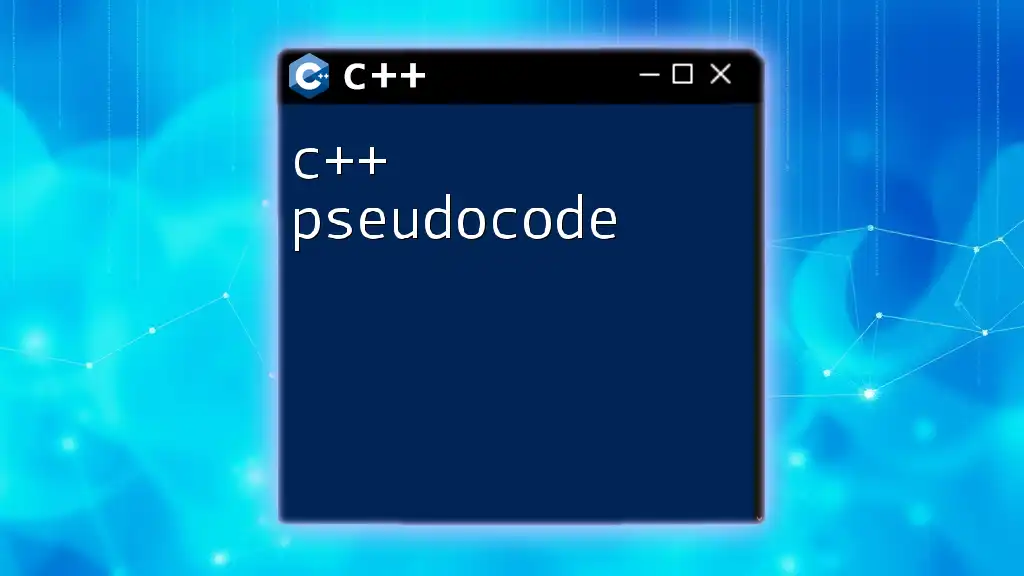
Why You Need to Decode Base64
Common Applications of Base64 Decoding
Base64 decoding is widely used in various applications, including:
- Email transmission: Base64 is commonly employed for encoding attachments in MIME formats, enabling seamless data transfer.
- Web data transfer: Encoded data is often sent within JSON and XML payloads when interoperability is required.
- Source code embedding: Base64 allows for storing binary files, such as images or executables, in a text-based format suitable for programming languages like C/C++.
Security Considerations
With the growing reliance on Base64 encoding, security becomes paramount. Improper handling of Base64 data can pose risks such as data integrity vulnerabilities or injection attacks. Thus, utilizing a reliable decoding method ensures that the integrity of the data is maintained.
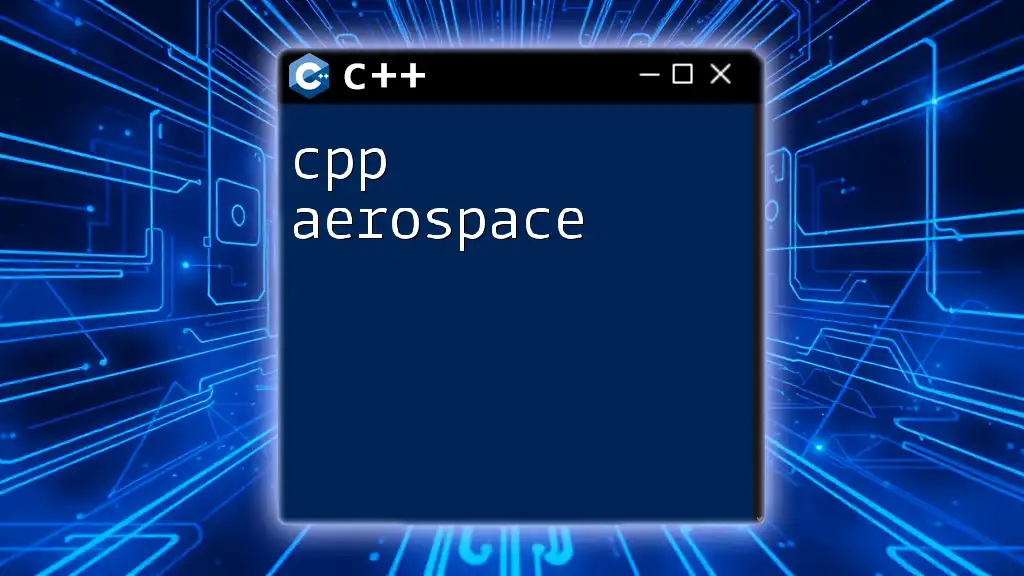
Base64 Decode in C++
Basic Concepts
When it comes to quickly implementing a C++ Base64 decode functionality, it's essential to harness the power of common libraries or functions. While C++ does not contain built-in support for Base64 operations, we can efficiently create our own using standard libraries like `<string>` and `<vector>`.
Creating a Base64 Decode Function
Step-by-Step Implementation
-
Include Required Headers
You will need to include the necessary headers to handle strings, vectors, and exceptions:
#include <string> #include <vector> #include <stdexcept>
-
Define Your Decoding Function
The core of your implementation can be summarized in a function that takes an encoded Base64 string and returns the decoded string. The function prototype might look like this:
std::string base64_decode(const std::string &in);
-
Utilize a Lookup Table for Base64 Characters
To facilitate the decoding, we need to create a lookup table that maps Base64 characters to their corresponding values. The lookup table is crucial for converting each Base64 character to its numerical value.
static const std::string base64_chars = "ABCDEFGHIJKLMNOPQRSTUVWXYZ" "abcdefghijklmnopqrstuvwxyz" "0123456789+/";
-
Handling Padding (‘=’) in Base64
Since Base64 encoding may involve padding to ensure proper formatting, the decode function must account for these padding characters. You must remove padding characters and adjust the processing accordingly.
Complete Code Example
Using the Base64 Decode Function
Here's a complete example of how the `base64_decode` function looks, alongside a main function demonstrating its usage:
#include <iostream>
#include <string>
#include <vector>
#include <stdexcept>
static const std::string base64_chars =
"ABCDEFGHIJKLMNOPQRSTUVWXYZ"
"abcdefghijklmnopqrstuvwxyz"
"0123456789+/";
std::string base64_decode(const std::string &in) {
size_t in_len = in.size();
if (in_len % 4 != 0) return ""; // Invalid input length
int padding = 0;
if (in_len > 0 && in[in_len - 1] == '=') padding++;
if (in_len > 1 && in[in_len - 2] == '=') padding++;
std::vector<unsigned char> buffer((in_len * 3) / 4 - padding);
for (size_t i = 0, j = 0; i < in_len;) {
uint32_t a = in[i] == '=' ? 0 : base64_chars.find(in[i++]);
uint32_t b = in[i] == '=' ? 0 : base64_chars.find(in[i++]);
uint32_t c = in[i] == '=' ? 0 : base64_chars.find(in[i++]);
uint32_t d = in[i] == '=' ? 0 : base64_chars.find(in[i++]);
buffer[j++] = (a << 2) | (b >> 4);
if (j < buffer.size()) buffer[j++] = (b << 4) | (c >> 2);
if (j < buffer.size()) buffer[j++] = (c << 6) | d;
}
return std::string(buffer.begin(), buffer.end());
}
int main() {
std::string encoded = "SGVsbG8gd29ybGQ="; // Example Base64 string
std::string decoded = base64_decode(encoded);
std::cout << "Decoded: " << decoded << std::endl; // Output: Hello world
return 0;
}
This code snippet implements a Base64 decode functionality that accurately converts an encoded string back to its original form. The example utilizes a common string encoding to showcase the operations in a straightforward manner.
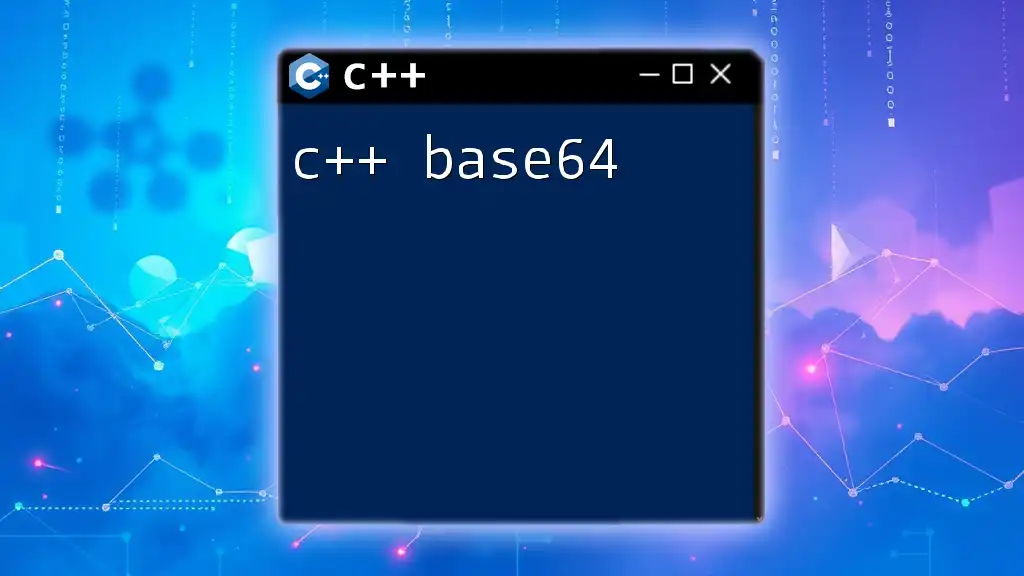
Testing Your Decode Function
Various Encoded Inputs
To ensure robustness, it's vital to test your Base64 decoding function with various encoded strings, including:
- Standard examples like "SGVsbG8gd29ybGQ=".
- Edge cases such as empty strings or strings containing invalid characters.
Testing with diverse inputs is crucial for identifying potential weaknesses in the decoding logic.
Validating Output
After decoding, you should validate your output against expected results. This verification can be accomplished with tools such as checksum comparisons or manual testing by encoding known strings to confirm the consistency of your decoding results.
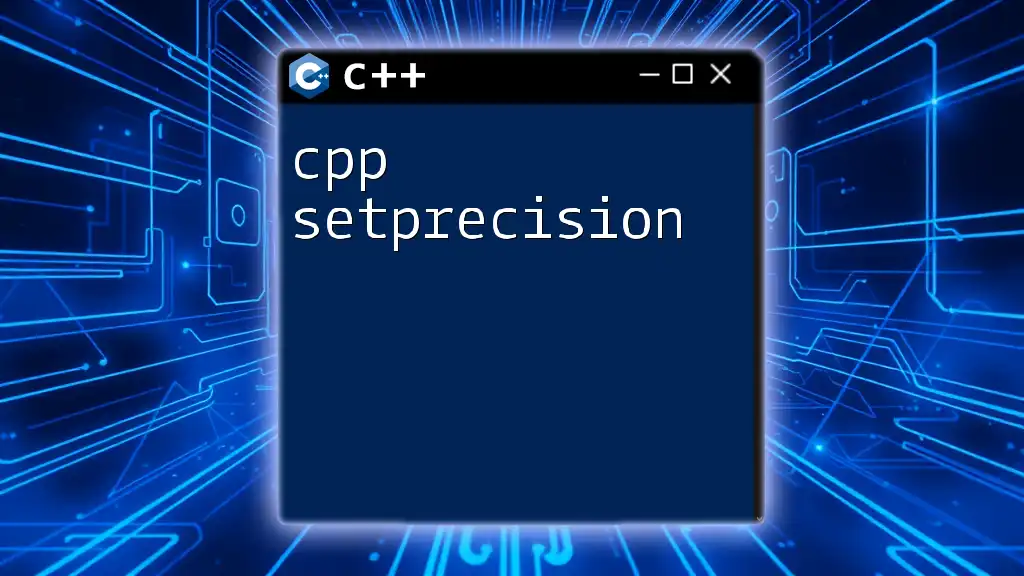
Error Handling and Best Practices
Common Errors in Base64 Decoding
While implementing your decoding function, be cautious of several common errors:
- Invalid input length: Always check that the input length is compatible with Base64 requirements.
- Improper character handling: Ensure that any unexpected characters are appropriately dealt with, which can contribute to runtime issues.
Best Practices for Base64 Decoding
Following best practices not only enhances your implementation's robustness but also improves maintainability. This includes:
- Writing unit tests to cover various scenarios, ensuring the function operates correctly under all conditions.
- Keeping your code clean and commenting on complex sections to help future development and maintenance.
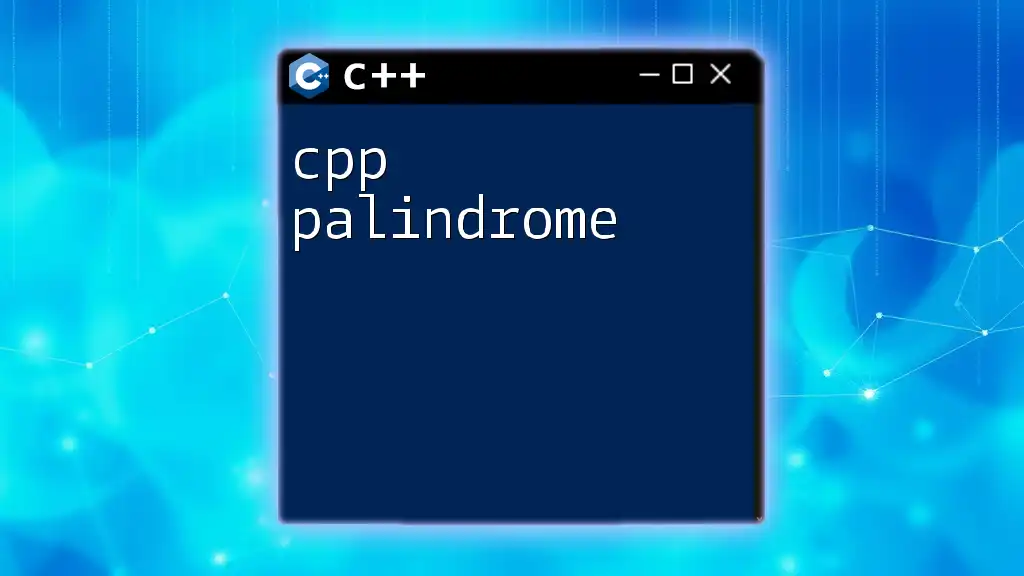
Conclusion
In this guide, we explored the concept of cpp base64 decode, highlighting its significance, implementation strategies, and best practices. By understanding how Base64 encoding and decoding function, you can easily implement these techniques in your applications and enhance your data management processes. I encourage you to experiment with your Base64 decoding implementation and see how it can be applied in various contexts. Happy coding!
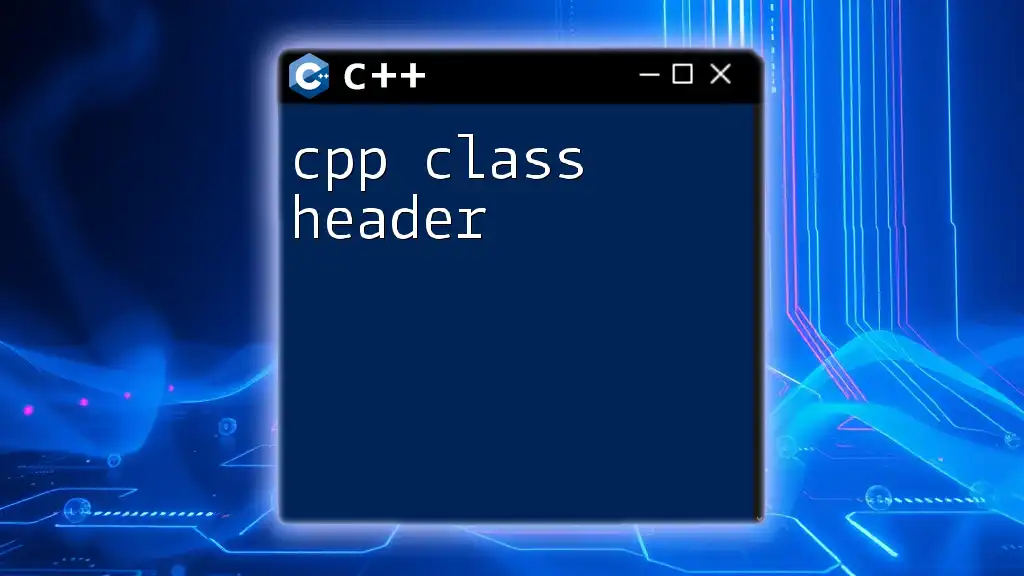
Additional Resources
For further reading and support, consider exploring documentation on Base64 or engaging with community forums where developers share insights and solutions regarding C++ coding challenges.