In C++, the `delete` operator is used to deallocate memory that was previously allocated with `new`, preventing memory leaks.
Here's a code snippet demonstrating its usage:
int* ptr = new int(10); // allocate memory for an integer
delete ptr; // deallocate the memory
What is `delete` in C++?
The `delete` operator in C++ is essential for managing dynamic memory allocation. It is used to deallocate memory that was previously allocated using `new`, ensuring that the program can free up space and prevent memory leaks. In C++, it’s important to distinguish between using `delete` for single objects and `delete[]` for arrays.
Differences Between `delete` and `delete[]`
- `delete` is used when you want to remove a single object from memory.
- `delete[]` is specifically for deallocating memory allocated for an array of objects. Failing to use the correct delete operator can lead to undefined behavior.
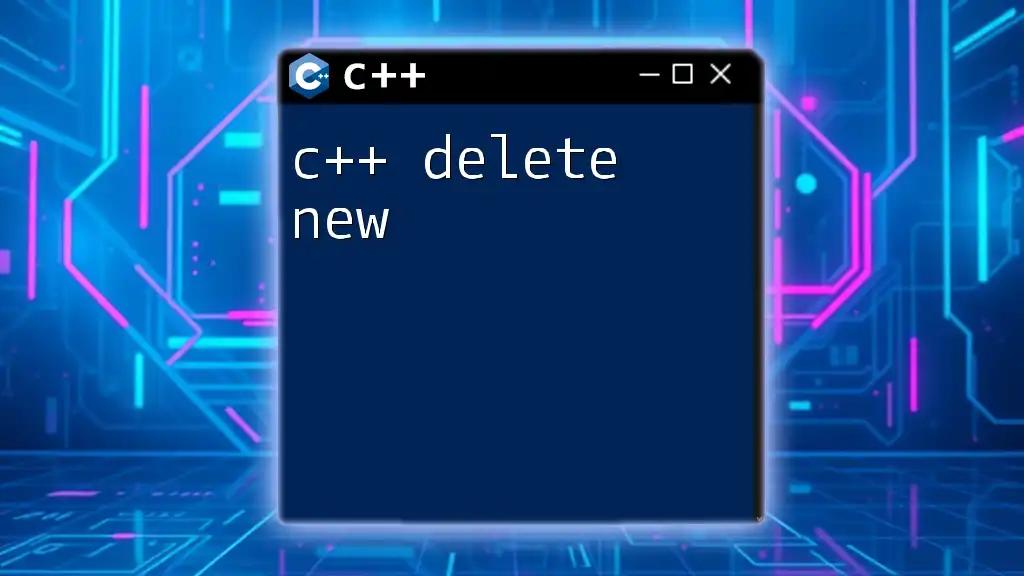
When to Use `delete` in C++
Understanding when to use `delete` is critical for effective memory management.
You should use `delete` in situations where:
- You have dynamically allocated memory, i.e., memory allocated with `new`.
- The object is no longer needed in your program, freeing up resources and preventing memory leaks.
Consequences of Neglecting to Use `delete`
If you forget to use `delete`, the allocated memory remains reserved even after the program has moved on from that allocation, leading to memory leaks. Over time, these leaks can consume all available memory, leading to program slowdowns or crashes.
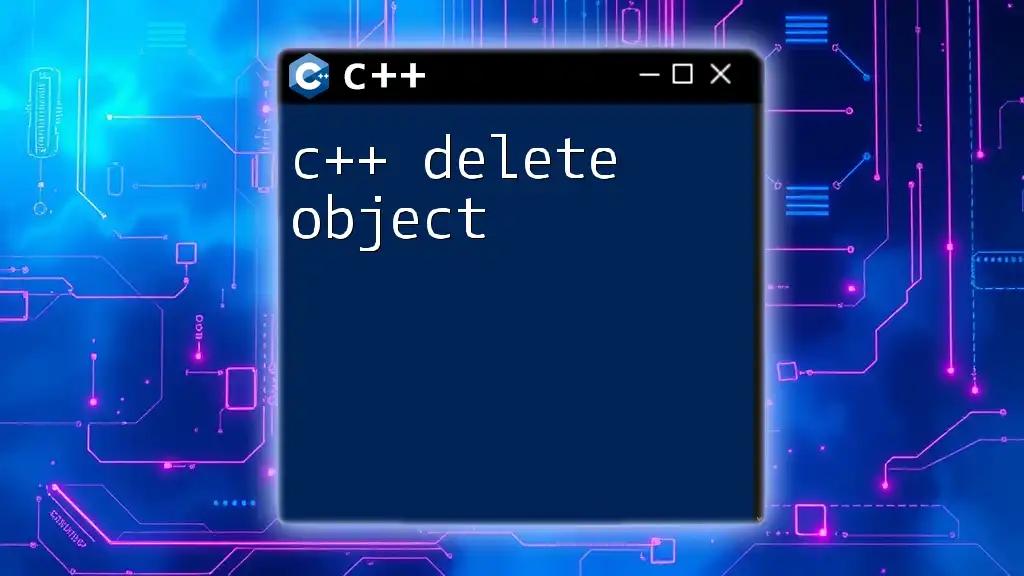
Syntax of the `delete` Operator
The syntax for using `delete` is straightforward, but understanding how it works is crucial.
Basic Syntax
To deallocate a single object, you use:
int* ptr = new int; // allocate memory
delete ptr; // deallocate memory
This command releases the memory allocated to `ptr`, allowing it to be reused later.
Using `delete[]`
For arrays, the syntax changes slightly:
int* arr = new int[10]; // allocate memory for an array
delete[] arr; // deallocate memory for the array
This ensures that all elements of the array are properly deallocated, preventing memory leaks.
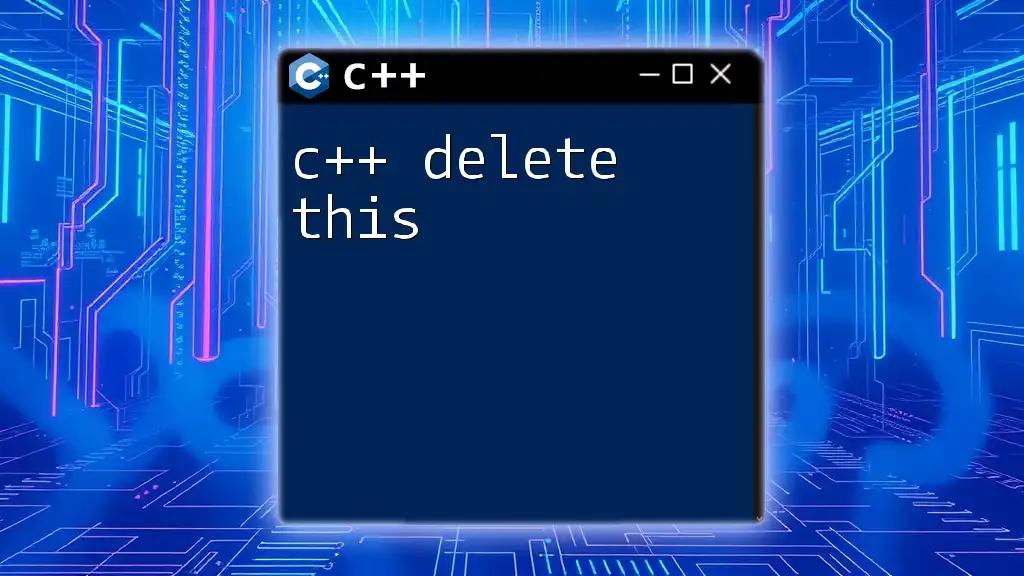
How to Use `delete` Effectively
Tips for Using `delete`
-
Ensure Pointers are Initialized: Always initialize your pointers. Using uninitialized pointers for deletion leads to undefined behavior.
-
Avoid Double Deletion: Never call `delete` on the same pointer more than once. This can corrupt your program’s memory management system, leading to crashes.
-
Set Pointers to `nullptr`: After deleting a pointer, set it to `nullptr` to avoid dangling pointers, which occur when a pointer still points to a deallocated memory block.
Code Snippet Example
Here’s an example that illustrates safe usage of `delete`:
int* num = new int(5);
// use the num pointer
delete num; // Clean up
num = nullptr; // Set to nullptr to avoid dangling pointer
In this example, after deleting the allocated memory, setting `num` to `nullptr` prevents accidental dereferencing.
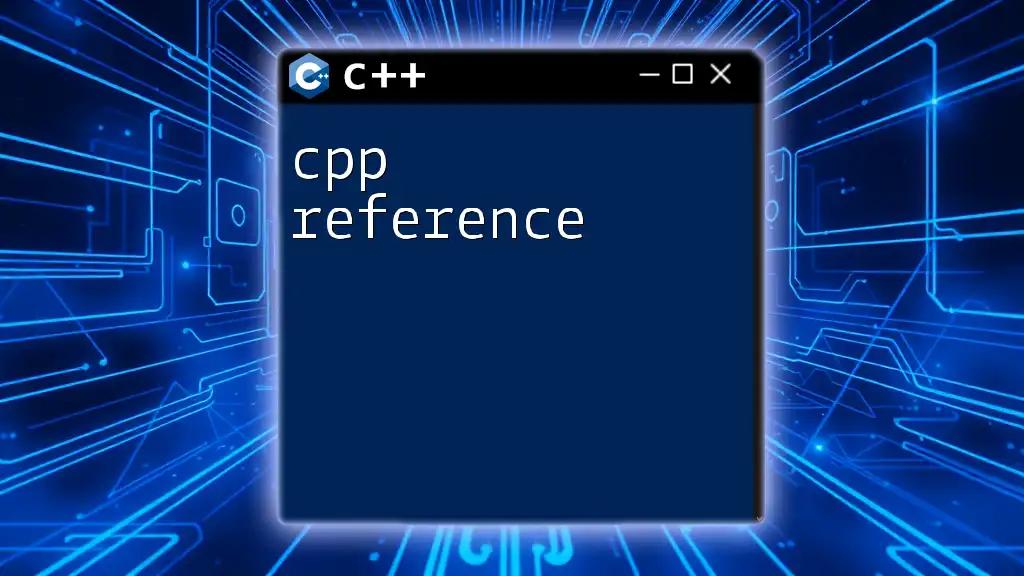
Common Issues with `delete`
Memory Leaks
Memory leaks occur when allocated memory is not released after its use. These leaks often happen when you forget to deallocate memory or lose track of pointers that point to allocated memory blocks.
To prevent memory leaks, always ensure that every `new` has a corresponding `delete`.
Dangling Pointers
A dangling pointer is a pointer that points to a memory location that has already been freed. Accessing a dangling pointer can cause bizarre program behavior or crashes.
To avoid creating dangling pointers, always set a pointer to `nullptr` after deleting the memory it points to. Consider the following example, which demonstrates a dangling pointer issue:
int* p = new int(10);
delete p;
// p is now a dangling pointer
In this case, `p` still holds the address of deallocated memory. If you try to dereference `p` now, the behavior is undefined.
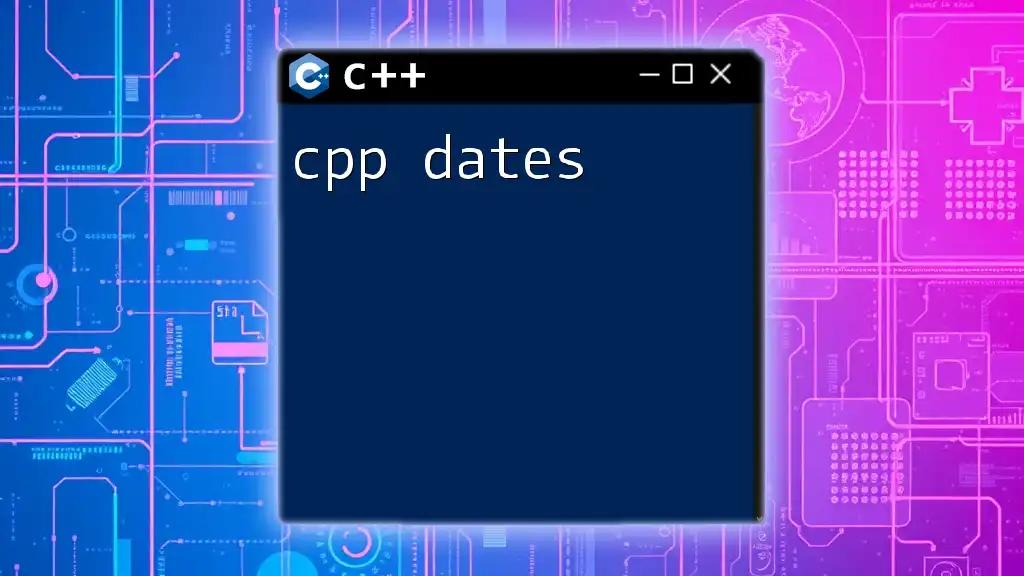
Best Practices for Memory Management in C++
Effective memory management is crucial for stable and efficient programs. Here are some best practices:
Overview of Best Practices
- Always pair `new` with `delete` and `new[]` with `delete[]`.
- Consider using smart pointers, such as `std::unique_ptr` and `std::shared_ptr`, which automate memory management and reduce the risk of leaks.
Advantages of RAII (Resource Acquisition Is Initialization)
RAII ensures that resources are properly disposed of when an object goes out of scope, thereby avoiding memory leaks. Using smart pointers as part of RAII principles helps minimize manual memory management, making your code cleaner and more secure.
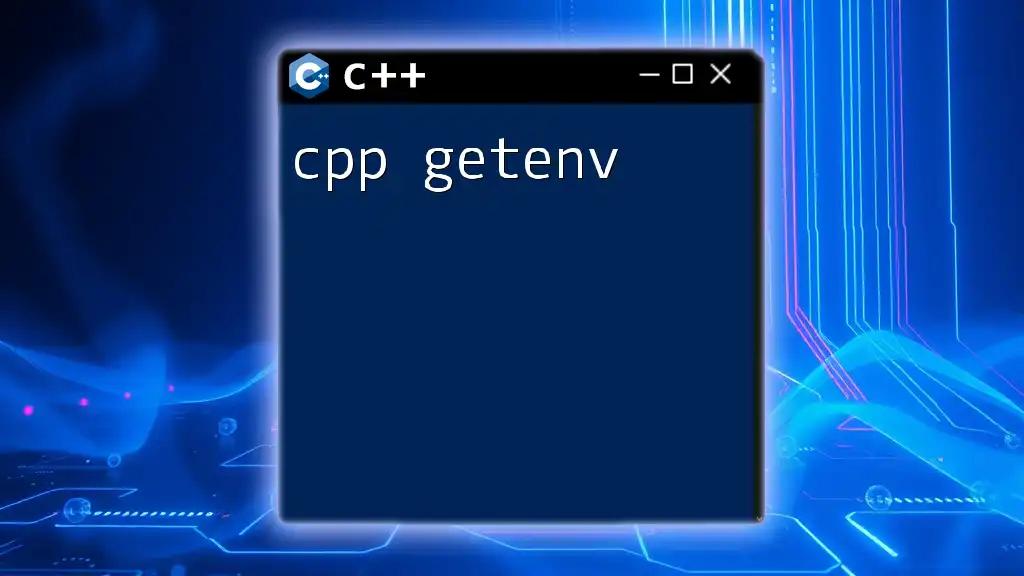
Conclusion
Understanding the `delete` command is fundamental to effective memory management in C++. Utilizing `delete` and `delete[]` correctly minimizes memory leaks and ensures that your programs run smoothly. Emphasizing safe deallocation practices and considering smart pointers will enhance your C++ programming experience.
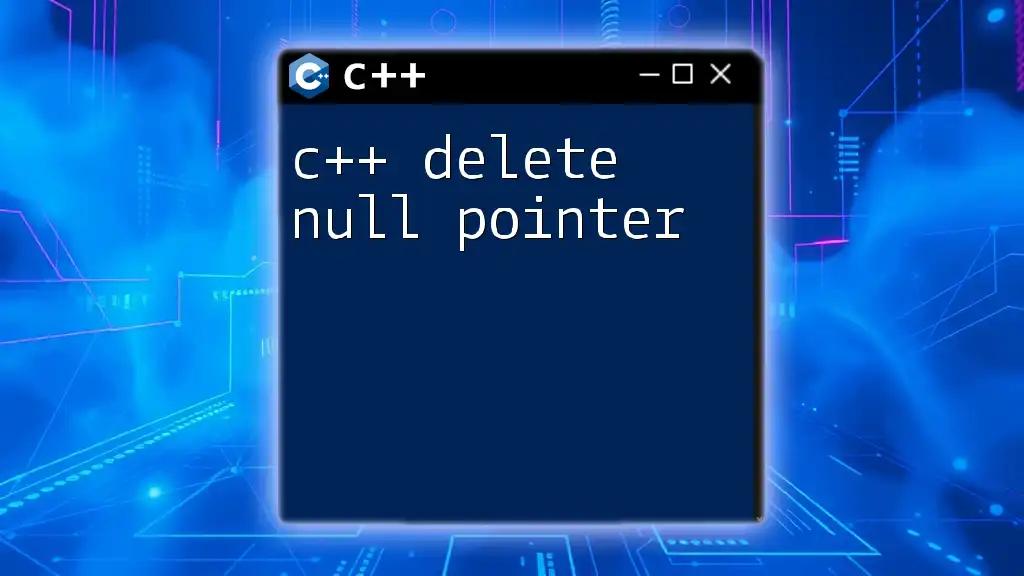
Additional Resources
To deepen your understanding, consider exploring recommended books and websites focusing on C++ memory management and best practices. Additionally, look for articles that discuss advanced topics in C++ that may incorporate the use of `delete` and other memory management techniques.

FAQs
What happens if I forget to use `delete`?
Forgetting to deallocate memory can lead to memory leaks, which gradually consume your system's memory resources, causing the program or system to slow down or crash.
Can I `delete` a pointer that was not allocated with `new`?
No, using `delete` on a pointer that was not allocated with `new` leads to undefined behavior. Always use `delete` only with memory allocated through `new`.
When should I use `delete[]` instead of `delete`?
Use `delete[]` when you want to deallocate memory that was allocated for an array. If you mistakenly use `delete` instead, it can lead to undefined behavior.
What are smart pointers and how do they relate to `delete`?
Smart pointers like `std::unique_ptr` and `std::shared_ptr` manage memory automatically. They ensure that memory is deallocated when the pointer goes out of scope, thus removing the burden of manual deletion and helping to prevent memory leaks.