The `cpp` (C Preprocessor) is a tool that processes directives before compilation, allowing for the inclusion of files, macro definitions, and conditional compilation.
Here’s a simple example of using the `#include` directive to include a standard library:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What Are C++ Directives?
C++ directives are special instructions that are processed by the preprocessor before actual compilation of the code begins. These directives serve various purposes, such as including files, defining macros, or controlling conditional compilation. Understanding how to effectively use C++ directives is crucial for writing maintainable and efficient code.
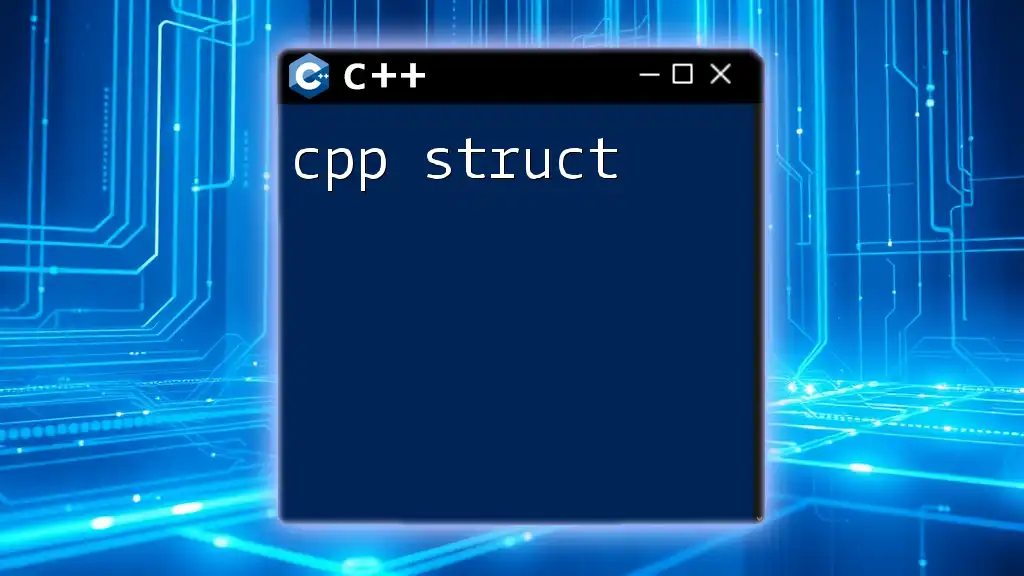
Brief History of C++ Directives
The concept of directives has been an integral part of C and has persisted into C++. With the rise of object-oriented programming and more complex software architectures, directives have evolved to accommodate the increasing need for organized, modular code. In C++, directives continue to play a pivotal role in code management and optimization.
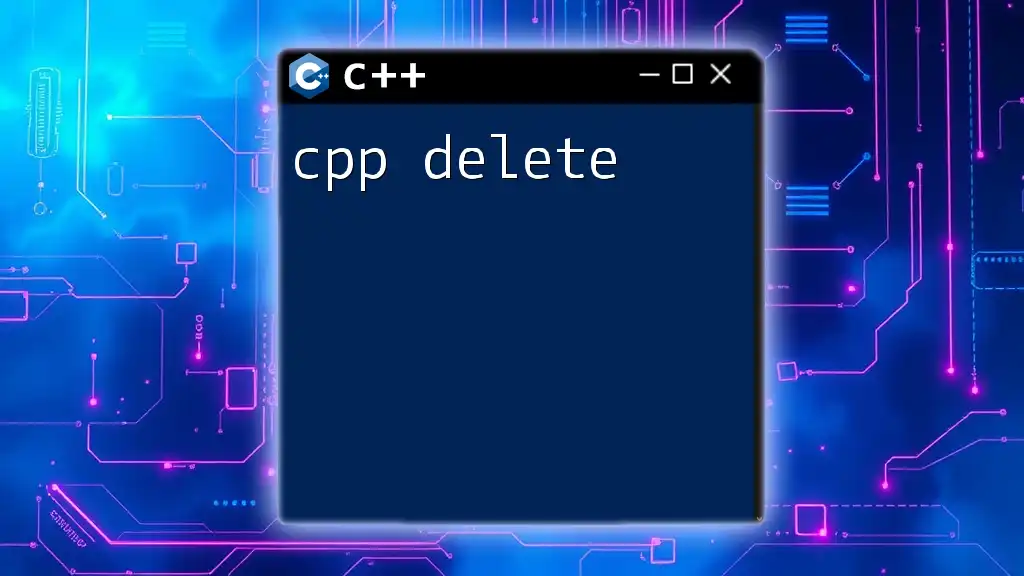
Understanding the Types of C++ Directives
Preprocessor Directives
Preprocessor directives are commands that are executed by the preprocessor before the actual compilation occurs. They begin with a `#` sign and don't require a semicolon. The most common preprocessor directives include:
Key Preprocessor Directives
-
`#include`
The `#include` directive is used to include header files in your program. By including standard or user-defined libraries, you can utilize various functionalities without writing the code from scratch.
Example:
#include <iostream>
-
`#define`
This directive is used to create macros, which are placeholders for values or expressions. Macros can help in defining constants or simplifying complex code.
Example:
#define PI 3.14159
-
`#ifdef` and `#ifndef`
Conditional compilation can be done using `#ifdef` (if defined) and `#ifndef` (if not defined) directives. These directives allow certain sections of code to be compiled based on the presence or absence of specific macros.
Example:
#ifdef DEBUG std::cout << "Debug mode is on." << std::endl; #endif
-
`#undef`
This directive is used to undefine macros that were previously defined using `#define`. It's useful for ensuring that a macro does not interfere with other sections of the code.
Example:
#undef PI
-
`#if`, `#else`, `#elif`
These directives enable more complex conditional compilation based on macro values. They add flexibility to your code by allowing different versions of code to be compiled.
Example:
#if defined(WIN32) std::cout << "Windows OS" << std::endl; #else std::cout << "Non-Windows OS" << std::endl; #endif
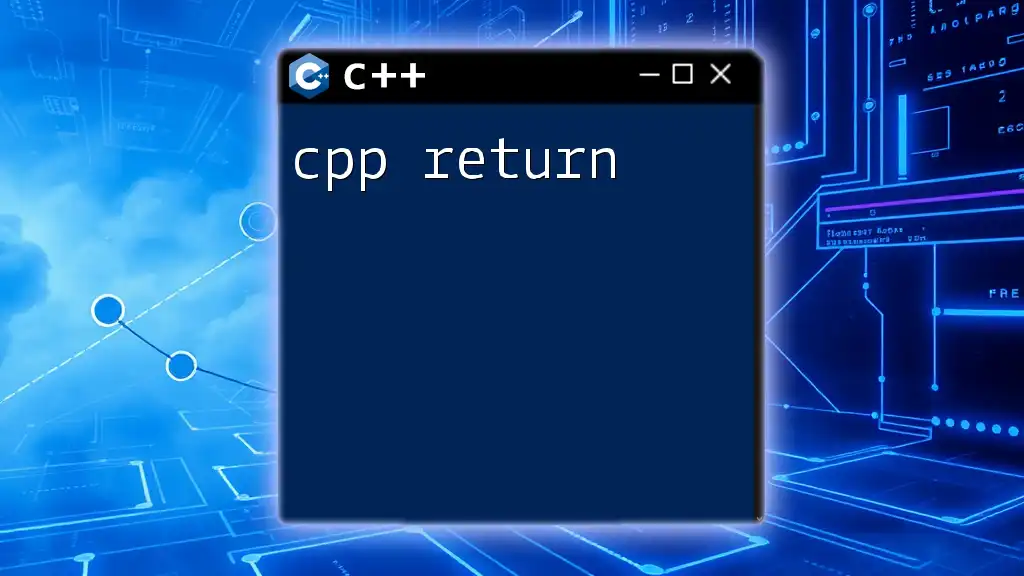
How to Use Directives Effectively
Organizing Code with Directives
Using directives can significantly enhance the structure and readability of your code. By separating different sections logically—such as declarations, definitions, and conditional compilations—you enable future developers (or yourself) to navigate the codebase more conveniently. Effective organization also supports easier debugging and testing processes.
Debugging with Directives
Directives like `#ifdef` enable you to isolate debugging information without altering your main code structure. For example, you can include debug messages only when you compile in a debug mode, thus keeping your releases clean.
Example:
#ifdef DEBUG
std::cout << "Debugging mode: Variable x = " << x << std::endl;
#endif
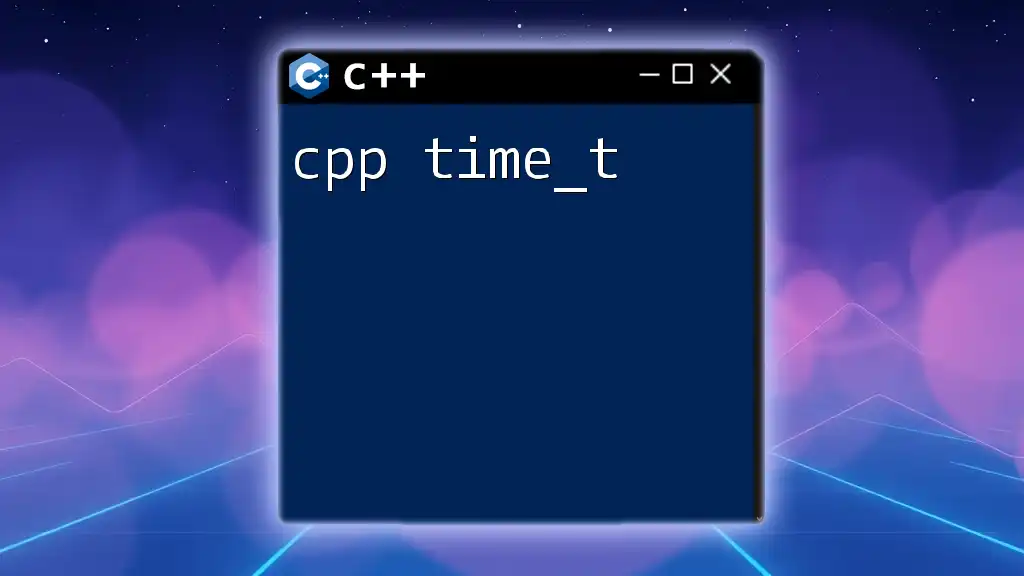
Best Practices for Using C++ Directives
Avoiding Common Pitfalls
One of the main pitfalls is the overuse of directives. Excessive macro definitions can clutter your code and make it hard to manage. For instance, directly changing macro values can lead to unexpected behavior elsewhere in the codebase.
Example of messy code:
#define A 10
A++;
When to Use and When to Avoid
Use directives judiciously. They are helpful in managing compilation features, but over-reliance may lead to complexities. Focus on clarity—make it easy for future developers to understand the reasoning behind certain directives. If a directive complicates your code’s structure or clarity, consider refactoring instead.
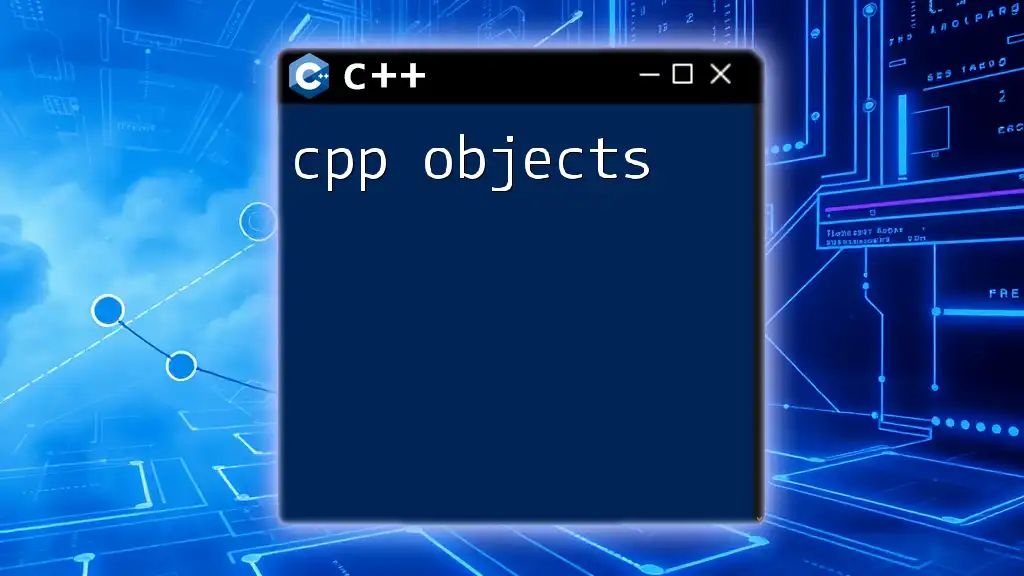
C++ Directives in Modern C++
New Additions and Features
With the advancements in C++11 onwards, new features have been incorporated into C++, impacting how we use directives. Concepts like name spaces, auto keyword, and lambda expressions have reduced the need for excess macro definitions, allowing for smoother and more straightforward code.
Standard Library and Directives
The inclusion of standard libraries via `#include` has become even more prevalent with the expansion of C++ Standard Template Library (STL). Including libraries allows developers to leverage extensive functionalities without the cumbersome task of implementing them from scratch.
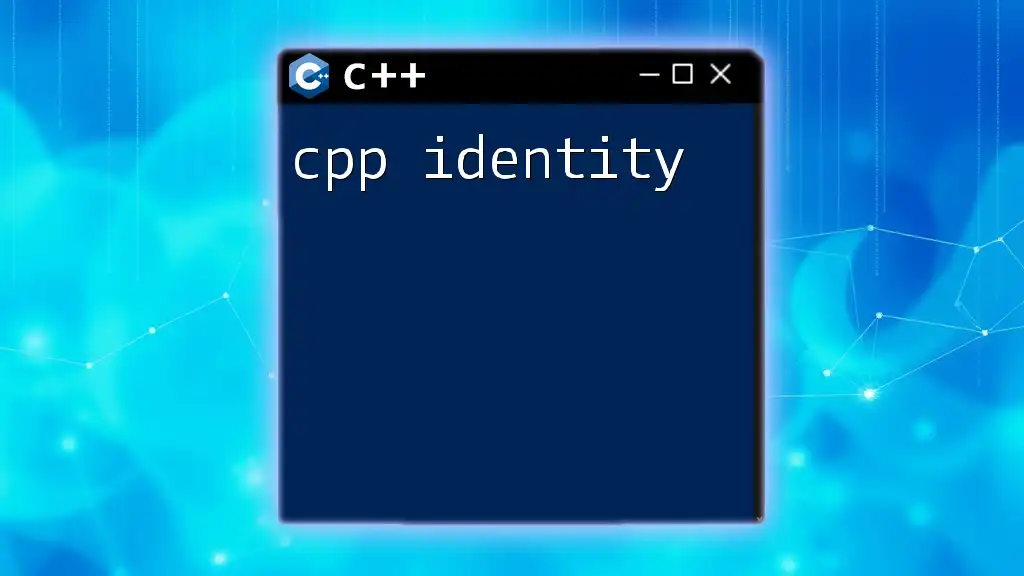
Conclusion
C++ directives, often overlooked, are the backbone of organized and efficient coding in C++. They allow for better modularity, clearer debugging paths, and robust condition management—all crucial elements in software development. Mastering the use of directives not only streams your workflow but helps in writing clean and maintainable code.
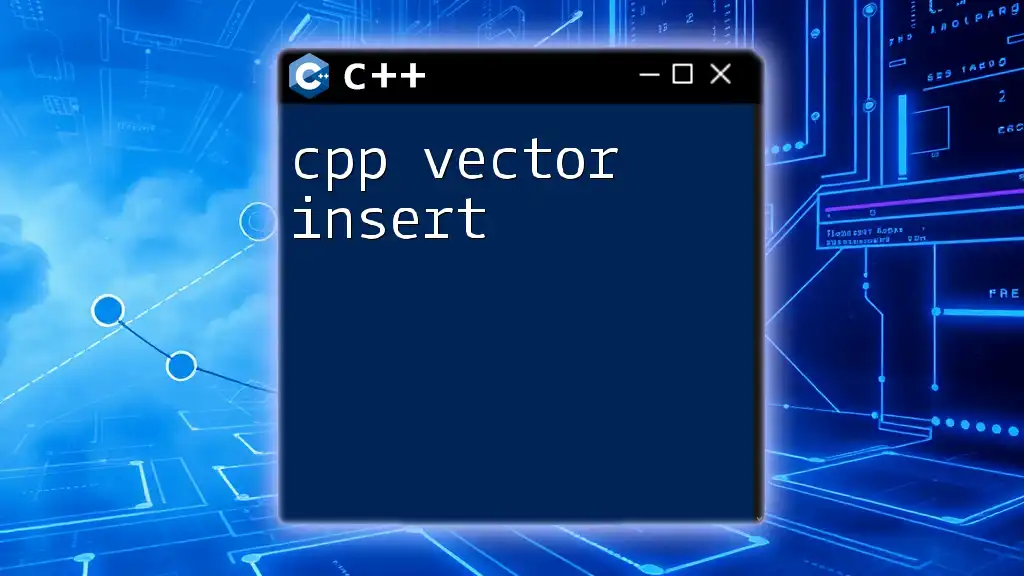
Additional Resources
To expand your knowledge on C++ directives and best practices, consider exploring related books and tutorials. Online courses are also invaluable resources for real-world applications. Engaging with community forums helps sharpen skills through shared experiences and solutions.
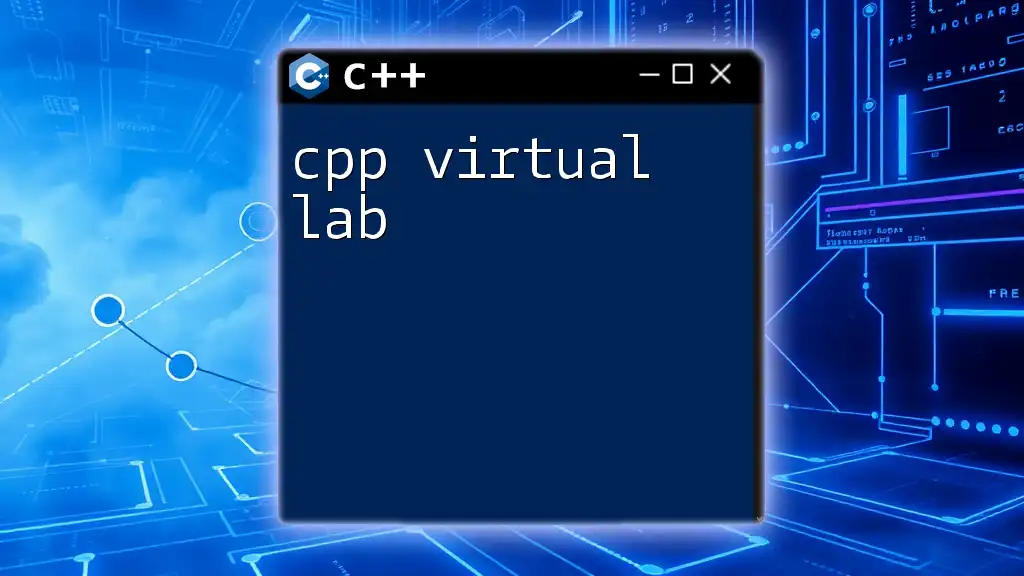
Q&A Section
Answering Common Questions about C++ Directives
-
What happens if you forget to include a directive?
If you forget to include necessary directives like `#include`, the compiler will generate errors indicating that particular types or functions are undefined. -
How do directives affect compilation?
Directives determine which parts of the code are compiled and help manage various configurations, leading to smoother compilations based on the defined macros and conditions.