The "cpp identity" refers to a preprocessor directive in C++ that helps in creating macros and is often used to create self-referential macros for generic programming.
Here’s a simple example of a macro that uses identity:
#define IDENTITY(x) (x)
int main() {
int a = 5;
int b = IDENTITY(a); // b will be 5
}
What is Identity in C++?
In C++, identity refers to the unique identity of an object that distinguishes it from all other objects. This is crucial in understanding how objects are managed in memory and how operations like assignment and comparison are performed. It's vital to note that identity is different from equality; two different objects can be equal (they hold the same value), but they do not share the same identity.
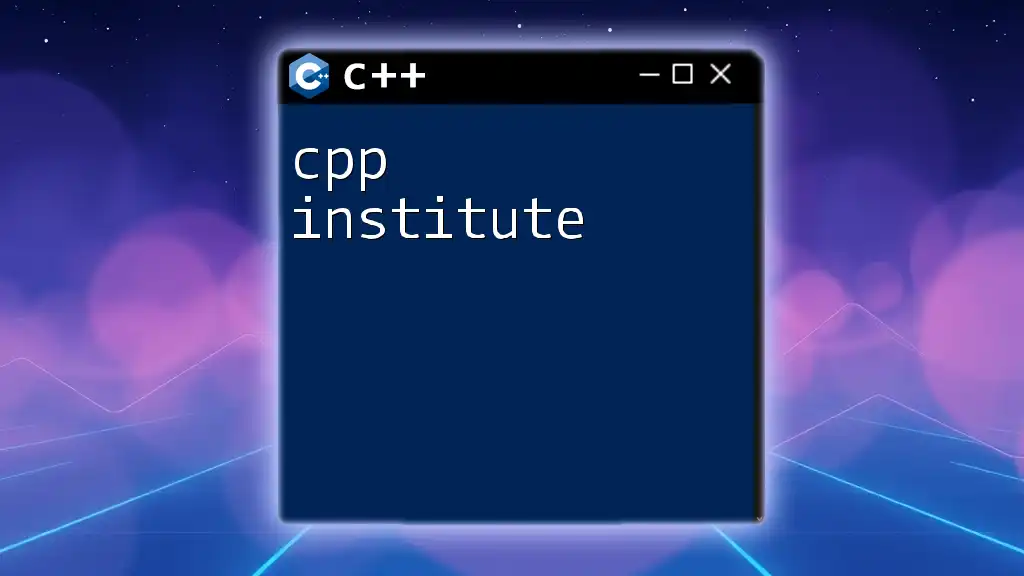
The Role of Pointers and References
Pointers and references play a significant role in defining the identity of objects in C++. They are both used to access data, but their implications on identity differ substantially.
Pointers
A pointer holds the memory address of a variable, which means it can point to different variables and even move across the memory space. When you utilize pointers, the identity of the object is embedded within the address itself.
References
A reference is essentially an alias for another variable. When you declare a reference to an object, you are not creating a separate entity but rather creating another name for the existing object, sharing its identity.
To illustrate the distinction, consider the following code snippet:
int main() {
int x = 10; // variable x
int* ptr = &x; // pointer to x
int& ref = x; // reference to x
// Displaying identity
std::cout << "Value: " << x << ", Pointer Address: " << ptr
<< ", Reference Value: " << ref << std::endl;
}
The output of this code shows that the pointer gives the address of `x`, while the reference `ref` yields the same value as `x`, thus highlighting the difference between references and pointers with respect to identity.
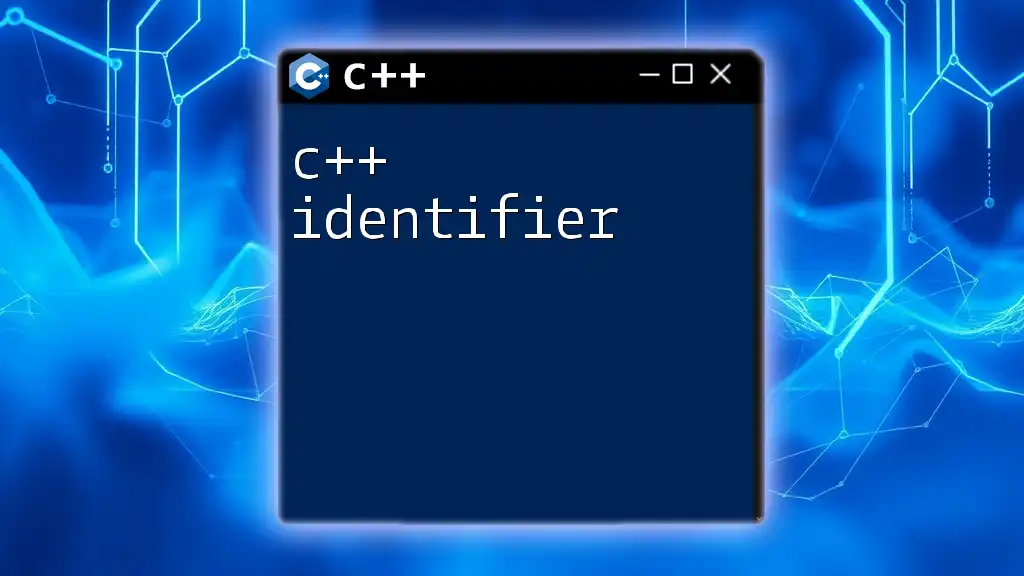
Identity of Objects
When we deal with user-defined types, such as classes, the concept of identity becomes more complex. Each instance of a class has a unique identity unless explicitly designed otherwise.
Deep vs Shallow Copy
Deep copy duplicates all objects and their referenced data, while shallow copy merely copies the reference, leading to shared identity between two objects.
Consider the following example that helps clarify this:
class MyClass {
public:
int data;
MyClass(int val) : data(val) {}
};
int main() {
MyClass obj1(5); // Original object
MyClass obj2 = obj1; // Shallow copy
MyClass obj3(obj1); // Another way of initialization
std::cout << (obj1.data == obj2.data) << std::endl; // true
std::cout << (&obj1 == &obj2) << std::endl; // false, different identities
}
In this code, while `obj1` and `obj2` may have the same data, they are distinct objects residing at different memory addresses, confirming their unique identities.
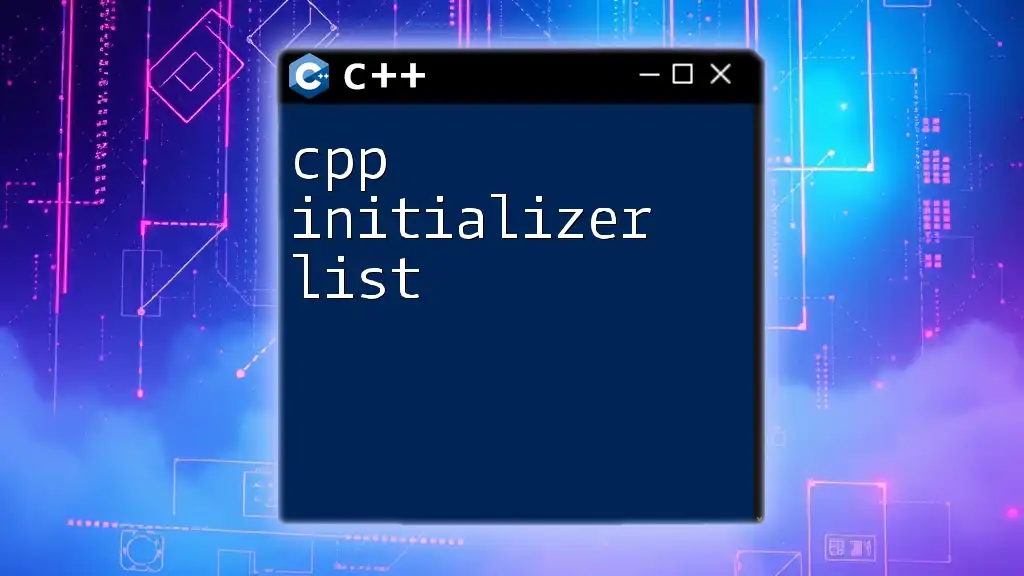
Comparing Identity in C++
Using `==` vs `is`
In C++, using `==` compares the values of objects, demonstrating equality, not identity. It's essential to apply identity checks correctly to find if two objects are the same in memory.
Unique Identifiers
Utilizing smart pointers, like `std::unique_ptr`, allows multiple ownership semantics, which can further complicate identity. A unique pointer ensures that a resource is owned by only a single pointer instance, thus maintaining a clear identity.
Here's an example of how `std::unique_ptr` works:
#include <memory>
int main() {
std::unique_ptr<int> ptr1 = std::make_unique<int>(10);
std::unique_ptr<int> ptr2 = std::move(ptr1);
// ptr1 is now null; ptr2 holds the unique identity
if(ptr1 == nullptr && ptr2 != nullptr)
std::cout << "ptr1 is null, ptr2 takes ownership." << std::endl;
}
In this example, after moving `ptr1` to `ptr2`, `ptr1` now holds a null value while `ptr2` retains the unique identity of the initially allocated integer.
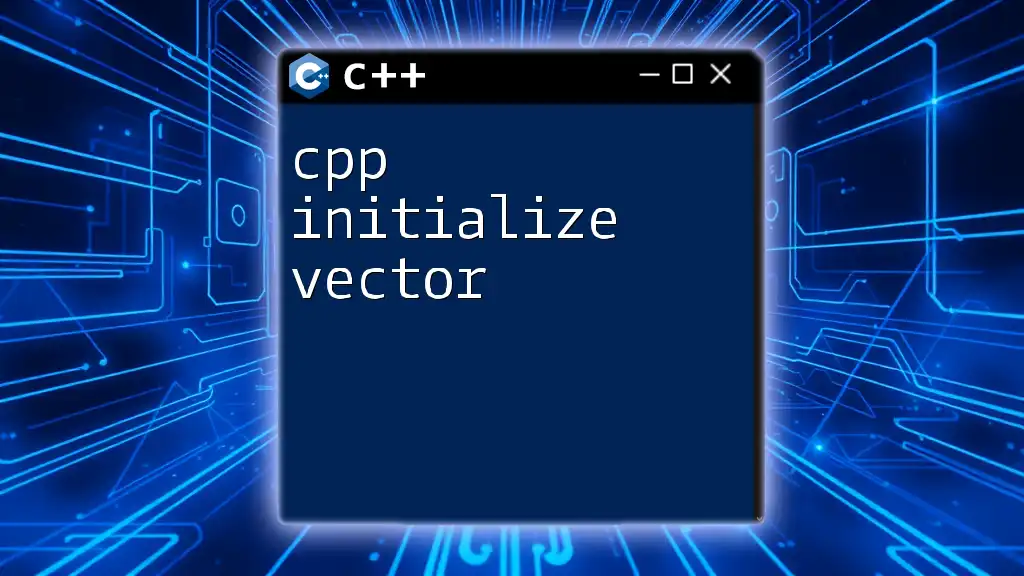
Identity and Object Lifespan
The lifespan of an object significantly influences its identity. Objects created on the stack have a limited lifespan confined to the block they are defined in. On the other hand, objects allocated on the heap remain in existence until they are explicitly deleted.
The following snippet demonstrates how object lifespan can affect identity:
#include <iostream>
void demoIdentity() {
int x = 20; // Stack allocation
int* y = new int(30); // Heap allocation
std::cout << "x: " << x << ", &x: " << &x << std::endl;
std::cout << "y: " << *y << ", &y: " << y << std::endl;
delete y; // Remember to release heap memory
}
int main() {
demoIdentity();
}
In this case, `x` will cease to exist once it leaves the scope of `demoIdentity`, while the memory allocated for `y` will remain available until it’s deleted, signifying how their identities are tied to their respective lifespans.
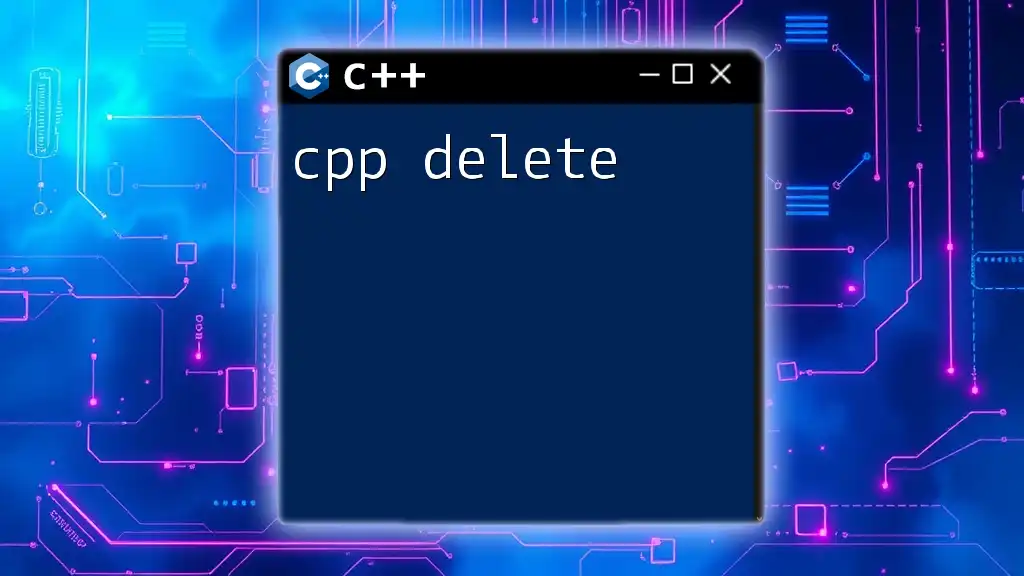
Practical Considerations for C++ Identity
Understanding how to manage memory and identities correctly is paramount in C++ programming. Failure to do so can lead to memory leaks and undefined behavior.
Copy Constructors and Assignment Operators
Copy constructors and assignment operators are fundamental; they dictate how identity is maintained when objects are assigned or passed. It's crucial to implement these functions correctly to ensure proper identity management.
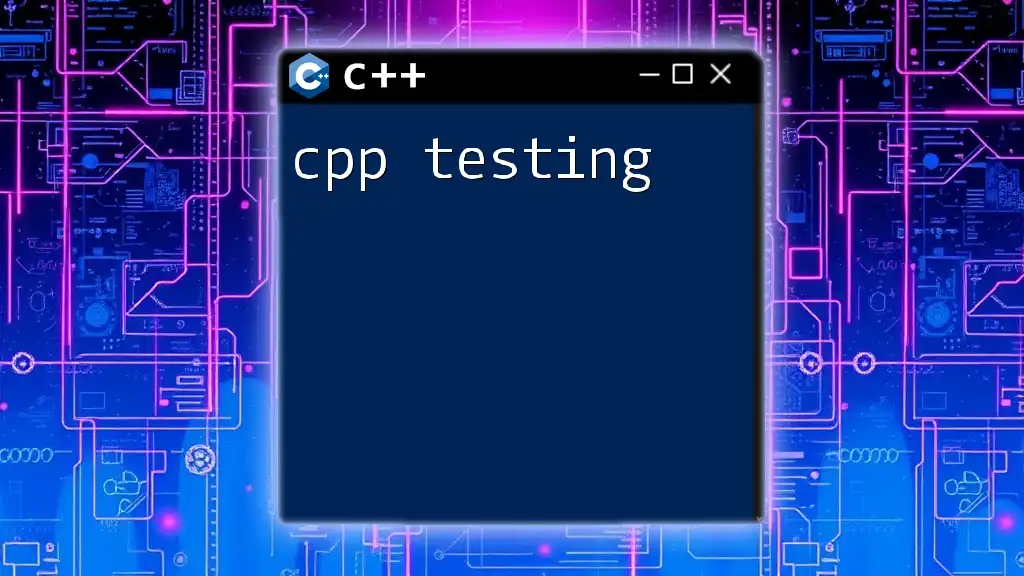
Example Projects Highlighting Identity
For practical application, consider developing mini-projects that can help reinforce your understanding of C++ identity. For instance:
- Object Collection Manager: Create a program that manages a dynamic collection of objects, demonstrating how identities are maintained through various operations.
- Custom Copy Constructor: Implement a class with a custom copy constructor to better understand how identities are preserved during object copying processes.
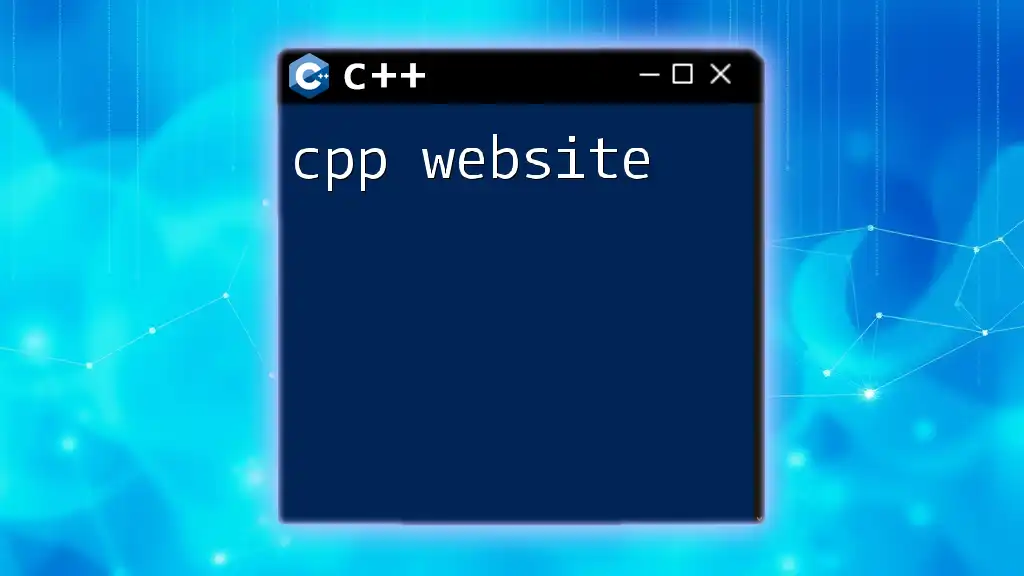
Conclusion
Understanding cpp identity is vital for any programmer working with C++. It is fundamental to managing objects correctly, from pointers and references to object lifespan and proper memory management. By grasping the nuances of identity, you can elevate your programming skills, ensuring that your applications are robust and efficient.
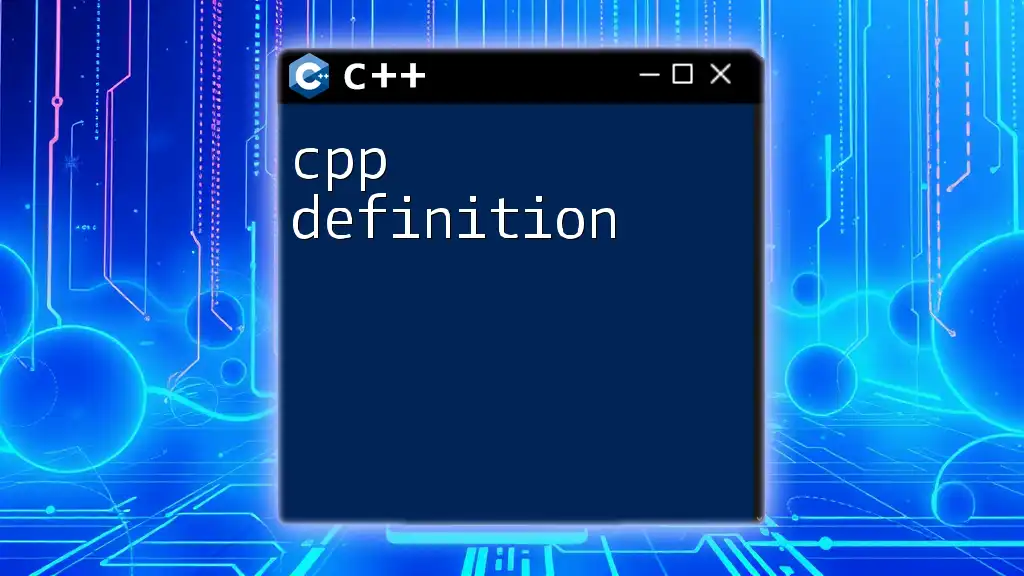
Further Reading and Resources
To deepen your understanding of C++ identity, consider exploring the following resources:
- Books: "Effective C++" by Scott Meyers
- Online Documentation: C++ Reference for smart pointers and memory management
- Community Forums: Engage with other programmers to share knowledge and experiences related to identities in C++.
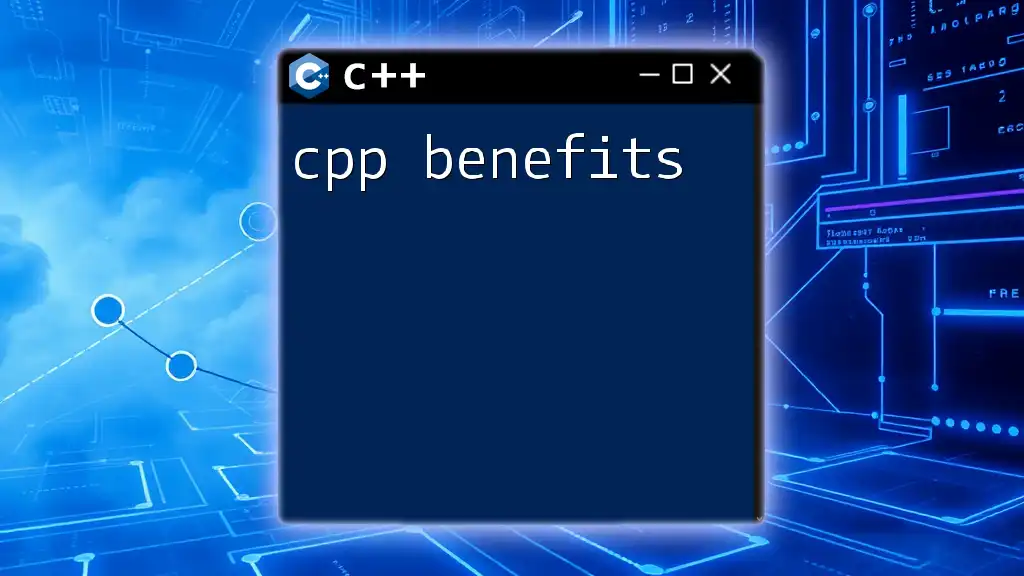
Call to Action
Stay updated with our latest articles on C++ commands by joining our newsletter! Share your own experiences or projects involving C++ identity in the comments below—we’d love to hear about your journey in mastering this important aspect of C++.