In C++, an identifier is a name used to identify a variable, function, class, or other user-defined item, and must follow specific rules such as starting with a letter or underscore and not containing spaces.
int myVariable = 10; // 'myVariable' is an identifier
Understanding Identifiers in C++
What is an Identifier?
A C++ identifier is a name used to identify a variable, function, class, or any other user-defined item in your code. Think of identifiers as labels that you assign to elements of your program to help make your code understandable and manageable. They serve a fundamental role in C++ programming, transforming complex operations into recognizable and meaningful symbols.
Rules for Naming Identifiers
To create valid identifiers, C++ programmers must adhere to specific rules regarding their structure:
- Valid Characters: Identifiers can consist of letters (both uppercase and lowercase), digits, and underscores. For example, `myVariable`, `age`, and `_count` are all valid identifiers.
- Invalid Characters: Reserved special symbols like `@`, `#`, and spaces are disallowed within identifiers. For instance, `my-variable` is invalid due to the hyphen and should be replaced with `my_variable`.
- Case Sensitivity: C++ treats identifiers as case-sensitive. This means that `Variable`, `variable`, and `VARIABLE` would be considered three distinct identifiers.
- Restrictions: You cannot use reserved words (keywords) as identifiers, such as `int`, `float`, and `return`. These keywords have predefined meanings in the C++ language.
Examples of Valid and Invalid Identifiers
Understanding practical examples can significantly aid in grasping the concept of identifiers.
Valid Identifiers:
int age; // Valid: uses letters and an underscore
float pi_value; // Valid: uses letters and an underscore
char firstName; // Valid: mixed case with no invalid characters
Invalid Identifiers:
int 2ndPlace; // Invalid: starts with a digit
float pi-value; // Invalid: contains a hyphen
char first Name; // Invalid: contains a space
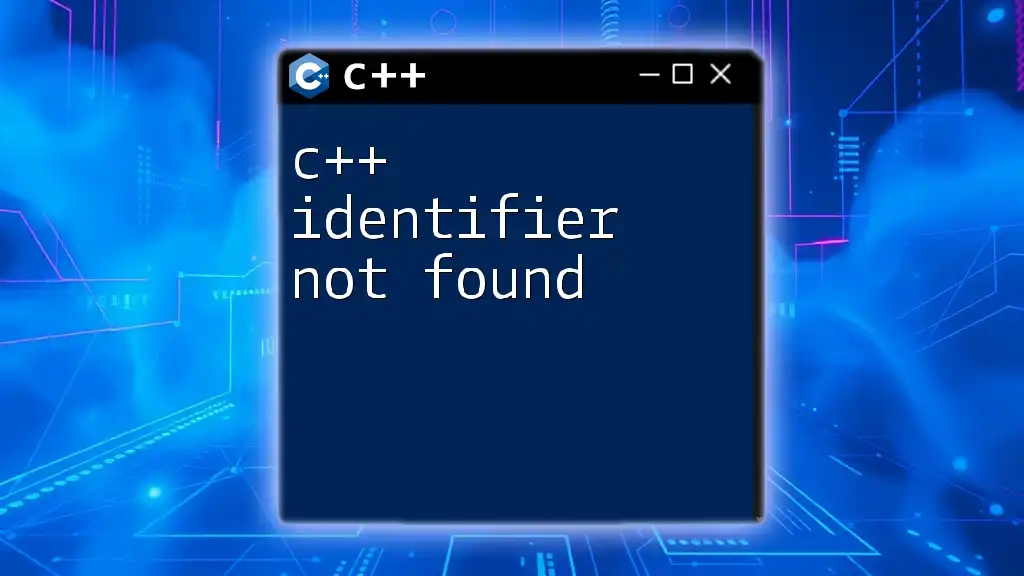
Types of Identifiers in C++
Built-in Identifiers
C++ provides a range of built-in identifiers, which are the default types recognized by the language, such as `int`, `char`, `float`, and `double`. These identifiers are essential for defining the types of variables you will use in your programs.
User-Defined Identifiers
User-defined identifiers are custom labels you create to define your own variables, functions, and classes. For instance, when you declare a variable like `int score;`, `score` is a user-defined identifier representing an integer value that you will work with in your program.
Special Identifiers
Global Identifiers
Global identifiers are defined at a file level and can be accessed from any function within that file. They remain in scope throughout the program’s life cycle.
Local Identifiers
Local identifiers are confined to the block of code (like a function) where they are declared. For example, a local variable defined inside a function is inaccessible from outside that function.
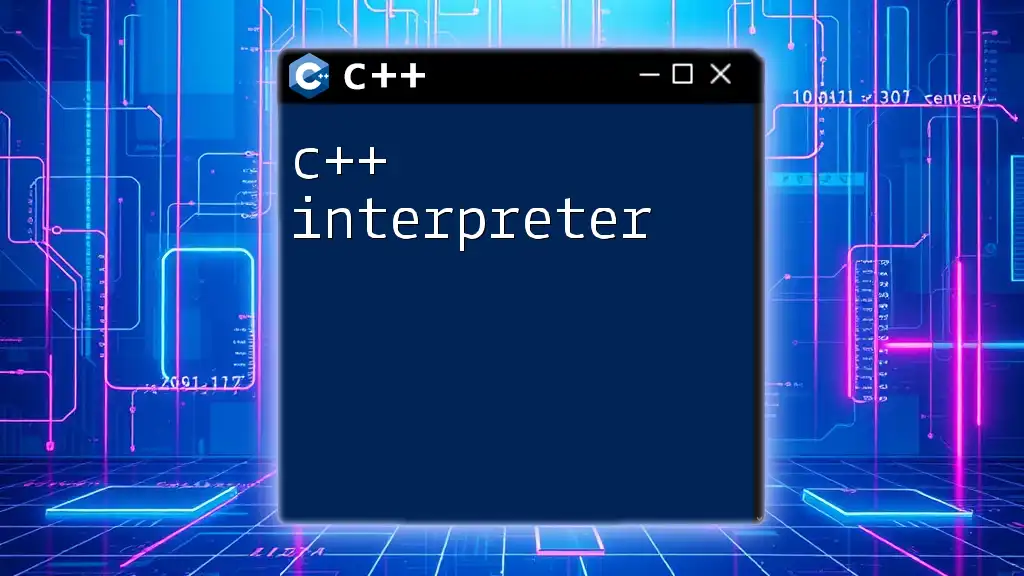
Best Practices for Naming Identifiers
Clarity and Readability
When naming identifiers, clarity and readability are paramount. Meaningful names reduce confusion and make your code more maintainable. For example, using `int x;` is vague and hard to interpret, while `int numberOfStudents;` gives immediate insight into the variable's purpose.
Consistency
Establish a consistent naming convention that reflects the purpose of your identifiers. Common practices include camelCase for variables (`numberOfStudents`) or snake_case for constants (`MAX_VALUE`). Adopting a uniform style enhances code readability and collaboration with other programmers.
Avoiding Confusion with Reserved Keywords
Reserved keywords in C++ have specific functions and cannot be used as identifiers. Familiarizing yourself with these terms—such as `break`, `class`, and `return`—is crucial to avoid potential confusion or compilation errors. Always double-check that your chosen identifier does not coincide with these reserved words.
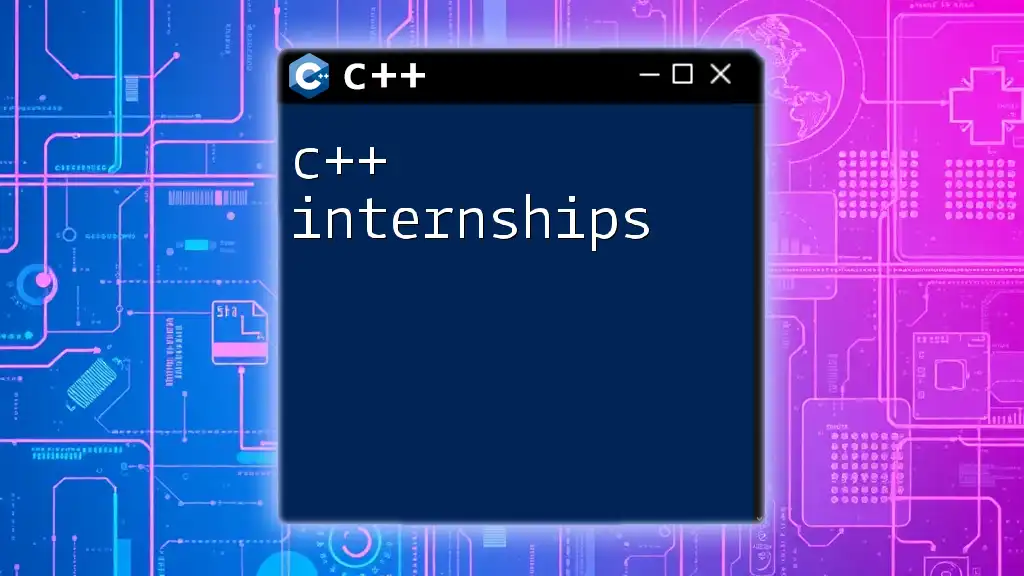
Practical Examples of C++ Identifiers in Action
Declaring Variables with Identifiers
Declaring variables is one of the most common uses of identifiers. Consider an example where you declare an integer identifier for the count of students enrolled:
int numberOfStudents; // A clear and descriptive identifier
Function Identifiers
Functions in C++ require identifiers to define what actions they perform. For instance, a simple function that prints a message can be structured as follows:
void displayMessage() {
cout << "Hello, C++ World!" << endl;
}
Here, `displayMessage` serves as the identifier for the function.
Class Identifiers
When creating classes in C++, identifiers play a crucial role in defining the class itself. Below is an example of a simple class with an identifier:
class Student {
public:
string name;
int age;
};
In this example, `Student` is the identifier for the class, while `name` and `age` are user-defined identifiers for its attributes.
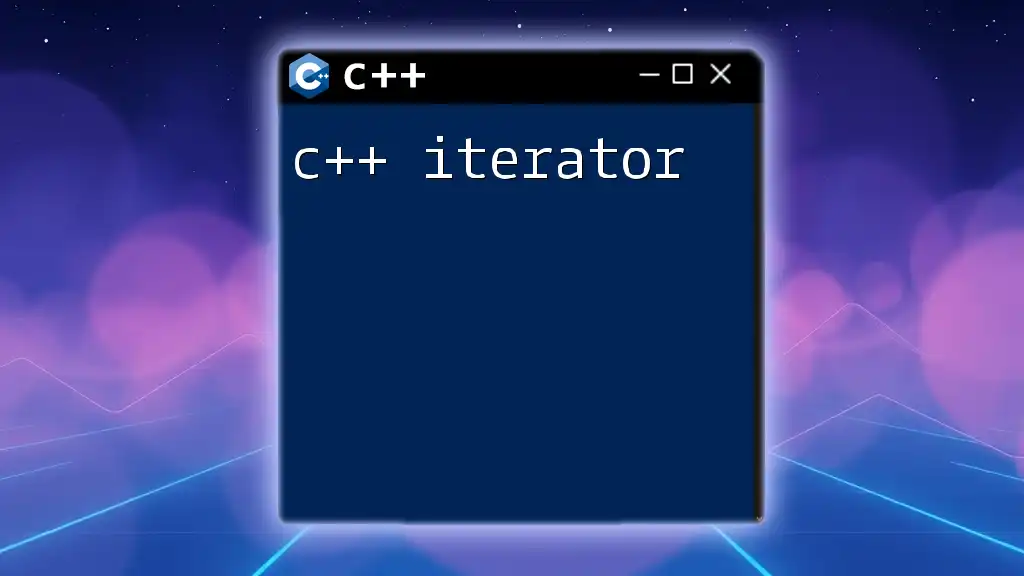
Common Mistakes with Identifiers
Even seasoned programmers can stumble when naming identifiers. Here are some common pitfalls to avoid:
- Using Too Generic Names: Identifiers like `data` or `temp` do not convey enough information. Strive to communicate the function or purpose through detailed names.
- Inconsistent Naming Conventions: Varying naming styles within the same code can lead to confusion. Stick to an established convention throughout your project.
- Confusion with Reserved Keywords: Forgetting that certain words are reserved can result in frustrating compilation errors. Always verify that the names you choose are unique within your coding context.
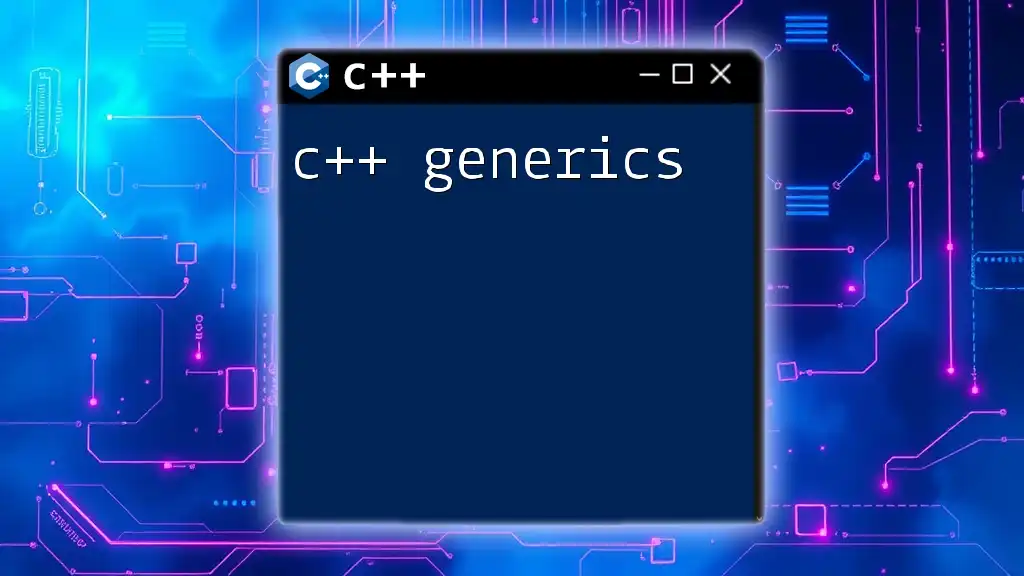
Tools and Resources for C++ Identifiers
IDE Features for Identifier Management
Modern Integrated Development Environments (IDEs) come equipped with features that help you manage identifiers effectively. Tools such as autocomplete, syntax highlighting, and linting can assist in checking the validity of your identifiers and ensuring consistency throughout your code.
Online Resources for C++ Programming
Numerous online resources can help deepen your understanding of C++ programming and the correct use of identifiers. Websites like Cplusplus.com, GeeksforGeeks, and the official C++ documentation provide a wealth of information, tutorials, and examples.
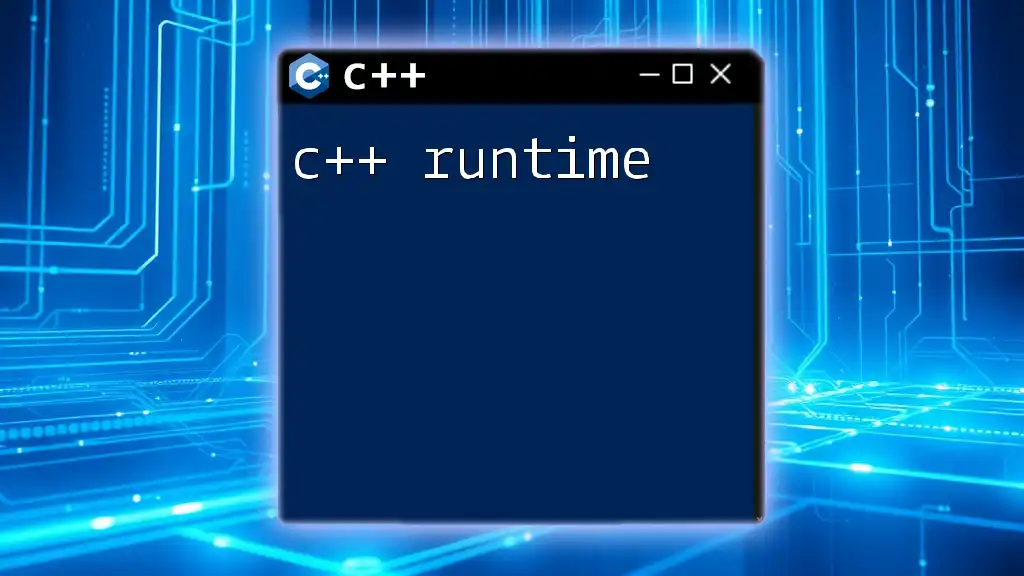
Conclusion
The use of identifiers in C++ is integral to software development, as they create the foundation for variable declaration, function definition, and class creation. By adhering to best practices around naming and understanding the types of identifiers, programmers can ensure their code is clear, maintainable, and compatible with the C++ language syntax.
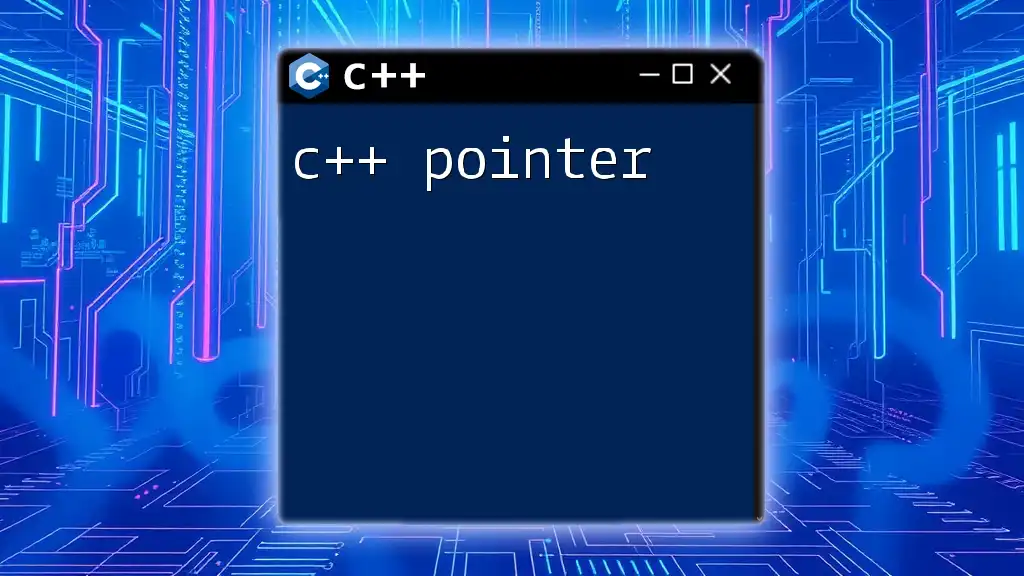
Call to Action
Share your experiences with C++ identifiers or any naming dilemmas you've encountered in your coding journey. Follow our blog for more tips and tricks to enhance your C++ programming skills, ensuring you stay ahead in the world of software development!