A C++ pointer is a variable that stores the memory address of another variable, allowing for efficient memory management and manipulation.
int main() {
int var = 42; // Declare an integer variable
int* ptr = &var; // Declare a pointer and store the address of var
std::cout << *ptr; // Dereference the pointer to access the value, outputs: 42
return 0;
}
Understanding Pointer Basics
What is a Pointer?
A C++ pointer is a variable that stores the memory address of another variable. Unlike ordinary variables, which store actual data values, pointers hold the address where data is stored in memory. This powerful feature enables dynamic memory management, efficient array handling, and more.
Understanding pointers is crucial, as they allow programmers to bypass certain limitations posed by C++, such as working with data structures like linked lists and trees.
Syntax of Pointers
To declare a pointer, you use the type of the variable you want to point to, followed by an asterisk (*) and the pointer's name. For example:
int* ptr;
In this syntax:
- `int` indicates that this pointer will point to an integer type.
- `*ptr` signifies that `ptr` is a pointer variable.
Proper understanding of this syntax forms the foundation for using pointers effectively in your applications.

How to Use Pointers in C++
Declaring and Initializing Pointers
To use a pointer, the first step is to declare it and optionally initialize it. Initialization often involves assigning it the memory address of an existing variable. For example:
int a = 5;
int* ptr = &a;
In this example:
- `a` is an integer variable initialized to 5.
- `&a` retrieves the memory address of `a`, which is then assigned to the pointer `ptr`.
Dereferencing a Pointer
Dereferencing a pointer means accessing the value stored at the memory address that the pointer references. This is done using the `*` operator. For example:
int value = *ptr;
In this scenario:
- `*ptr` accesses the value stored at the address contained in `ptr`, which is `5`.
- Thus, the variable `value` would also become `5`.
Understanding dereferencing is crucial for manipulating the values at specific memory addresses directly.
Pointer Arithmetic
Pointers can be altered using arithmetic operations, which is an advanced feature of C++. Pointer arithmetic allows one to navigate through an array or manipulate multiple memory locations conveniently. For instance:
ptr++;
In this code:
- `ptr++` increments the pointer to point to the next integer (4 bytes ahead on most systems).
- This is particularly useful in array processing, where you might want to traverse elements.

Types of Pointers in C++
Void Pointers
A void pointer is a special type of pointer that does not have a specific data type. It can hold the address of any data type but cannot be dereferenced directly without explicit type casting. For example:
void* ptrVoid = &a;
Void pointers are useful for implementing generic data structures and functions that can handle different data types.
Null Pointers
A null pointer points to nothing. In C++, you can initialize a pointer to a null value using `nullptr` or `NULL`. For instance:
int* nullPtr = nullptr;
Using null pointers is good practice for avoiding dangling pointers and unintentional dereferences.
Smart Pointers
Smart pointers, such as `std::unique_ptr` and `std::shared_ptr`, are objects that manage pointers for you, automatically handling memory allocation and deallocation. This leads to better memory management and reduces the risk of memory leaks.
For example:
std::unique_ptr<int> p1 = std::make_unique<int>(10);
Here, `p1` will automatically manage the memory for the integer. When `p1` goes out of scope, the memory will be freed automatically—eliminating the need for explicit deletion.

Advanced Pointer Concepts
Pointer to Pointer
A pointer to pointer is a pointer that stores the address of another pointer. This can be useful for dynamic 2D arrays or nested data structures. For instance:
int** ptrPtr = &ptr;
In this snippet:
- `ptrPtr` is a pointer that holds the address of `ptr`, allowing you to manipulate the pointer itself indirectly.
Function Pointers
Function pointers allow you to store the address of functions and call them dynamically. They enable you to implement callback mechanisms and pass functions as arguments to other functions. For example:
void (*funcPtr)(int) = &someFunction;
In this example, `funcPtr` is a pointer to a function that takes an `int` as a parameter, allowing you to call the function indirectly using `funcPtr(5)`.
Pointers and Arrays
C++ treats arrays as contiguous memory blocks, and pointers can be a powerful tool for manipulating them. The address of the first element of an array can be stored in a pointer, and by incrementing the pointer, you can traverse through the array elements effectively. For example:
int arr[] = {1, 2, 3, 4};
int* ptr = arr;
Here, `ptr` points to the first element of `arr`. You can access other elements using pointer arithmetic like so:
int secondValue = *(ptr + 1); // Retrieves the second element, which is 2

Best Practices for Using Pointers
Avoiding Memory Leaks
When using pointers, it's crucial to manage memory manually. A memory leak occurs when memory allocated dynamically is not released properly. To avoid this, always pair `new` with `delete`, and `new[]` with `delete[]` to clean up after allocation:
int* num = new int(42);
// ... use num ...
delete num; // proper cleanup
Using Smart Pointers Effectively
Smart pointers provide automatic memory management and should generally be preferred over raw pointers. When dealing with ownership (who is responsible for deleting the object), `std::unique_ptr` is typically used for single ownership, while `std::shared_ptr` can manage shared ownership of an object.

Conclusion
Mastering C++ pointers can elevate your programming capabilities, allowing for efficient memory management and complex data structure manipulation. Experimenting with pointers will lead to a deeper understanding of memory and performance optimizations in C++. Embracing smart pointers in your coding practices is encouraged to foster reliable and easy-to-maintain code.
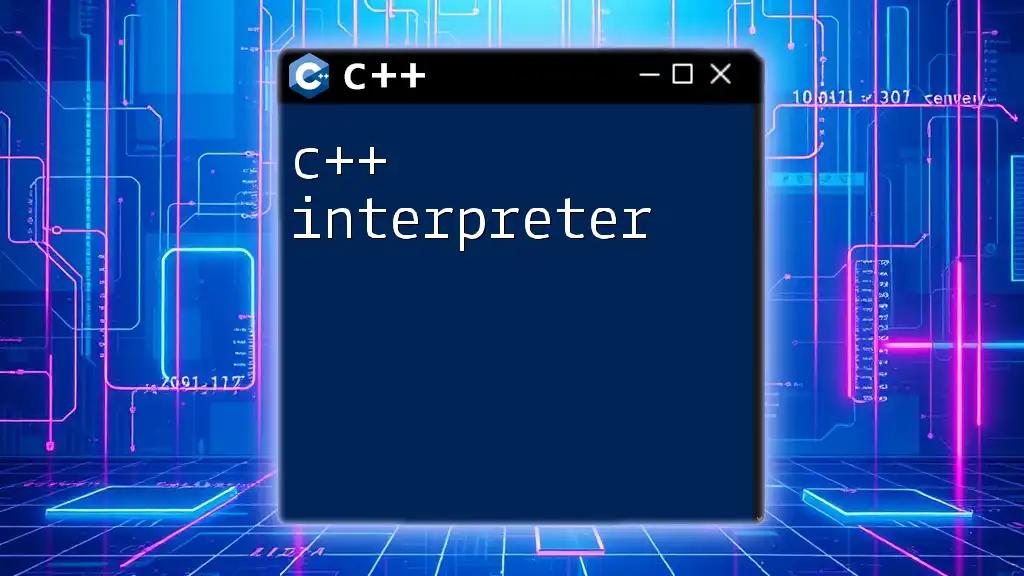
Further Reading and Resources
For those interested in expanding their knowledge of C++ pointers, various resources, including books, online courses, and the official C++ documentation, can provide deeper insights into memory management and efficient coding practices. Be sure to explore these materials to enhance your understanding and application of C++.