A C++ pointer to a member function allows you to define a pointer that can invoke a specific member function of a class on a particular instance of that class.
Here’s a simple code snippet demonstrating a pointer to a member function in C++:
#include <iostream>
class MyClass {
public:
void display() {
std::cout << "Hello from MyClass!" << std::endl;
}
};
int main() {
MyClass obj;
void (MyClass::*ptrToMemberFunc)() = &MyClass::display; // Pointer to member function
(obj.*ptrToMemberFunc)(); // Invoking the member function using the pointer
return 0;
}
Understanding Member Functions
What Are Member Functions?
Member functions are functions that operate as part of a class. In C++, they are essentially the methods defined within a class that have access to the data members of that class. Member functions enable encapsulation—allowing data manipulation while maintaining the integrity of the class structure.
Key Differences:
- Member Functions: Must be called on an object instance and can directly access the object's data members.
- Regular Functions: Cannot access the class data unless they have the object passed to them.
Types of Member Functions
C++ defines two main types of member functions:
- Instance Member Functions: Can be invoked on an instance of the class and have access to the instance's member variables.
- Static Member Functions: Belong to the class rather than any object instance, meaning they cannot access instance variables directly.
Here’s a brief illustration:
class MyClass {
public:
void instanceFunction() { /* code */ } // Instance member function
static void staticFunction() { /* code */ } // Static member function
};
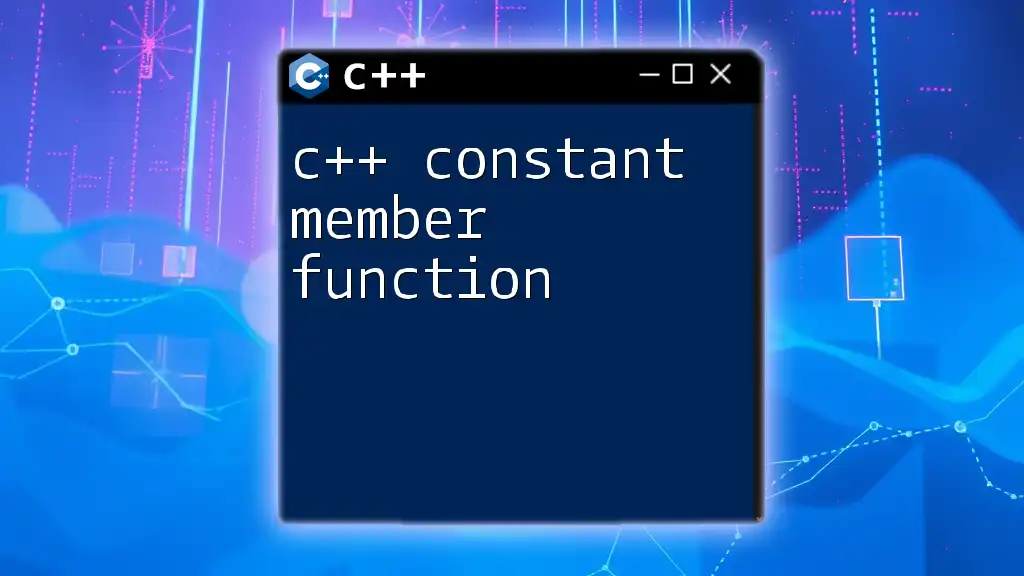
Introduction to Pointers
Understanding Pointers in C++
In C++, a pointer is a variable that holds the memory address of another variable—essentially serving as a reference to another object in memory. Pointers are fundamental in memory management and are especially crucial in dynamic memory allocation and data structure manipulation.
Employing pointers allows developers to directly manipulate memory locations and makes it possible to create complex data structures like linked lists and trees.
Syntax and Declaration of Pointers
To declare a pointer, you need to specify the type it points to. The following syntax demonstrates the declaration of a pointer to an integer:
int* p; // Declaring a pointer to an integer
Once declared, pointers can be manipulated to point to various memory locations, provided they adhere to the data type rules.
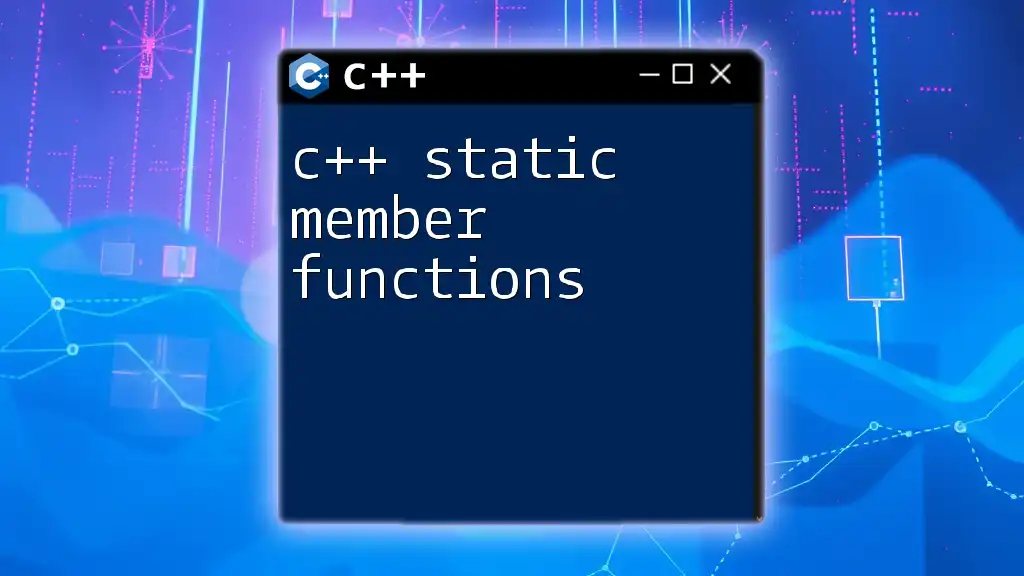
Pointers to Member Functions
What Is a Pointer to a Member Function?
A pointer to a member function allows you to indirectly invoke a member function of a class using a pointer, instead of accessing it directly through an instance of that class. This powerful feature enables developers to create flexible and dynamic function call mechanisms.
Syntax for Pointers to Member Functions
The syntax for declaring a pointer to a member function includes specifying the class name and the member function's signature. The general form is as follows:
void (ClassName::*pointerToMemberFunction)();
This syntax can seem daunting, but don’t worry—it becomes clearer with examples.
Initializing Pointers to Member Functions
To assign a pointer to a member function, you use the address-of operator (`&`) followed by the member function's name:
ClassName obj;
void (ClassName::*ptr)() = &ClassName::memberFunction;
In this example, `ptr` effectively points to `memberFunction` of the `ClassName` class.
Calling Member Functions Using Pointers
Basic Example
Here’s a straightforward example demonstrating how to call a member function using a pointer:
class MyClass {
public:
void myFunction() {
std::cout << "Hello, World!";
}
};
void example() {
MyClass obj;
void (MyClass::*ptr)() = &MyClass::myFunction;
(obj.*ptr)(); // Calling member function using pointer
}
In this code snippet:
- A class `MyClass` contains a member function `myFunction`.
- A pointer `ptr` to `myFunction` is declared and initialized.
- The syntax `(obj.ptr)();` calls `myFunction` using the pointer. The `.` operator is crucial here as it specifies that `ptr` is a pointer to a member function.
Scenarios and Use Cases
Pointers to member functions are particularly useful in situations where callbacks or event handlers are needed—such as GUI programming. For example, when you design a menu item that triggers a specific action in response to user input, you might use a pointer to member functions to dynamically call the corresponding function linked to that action.
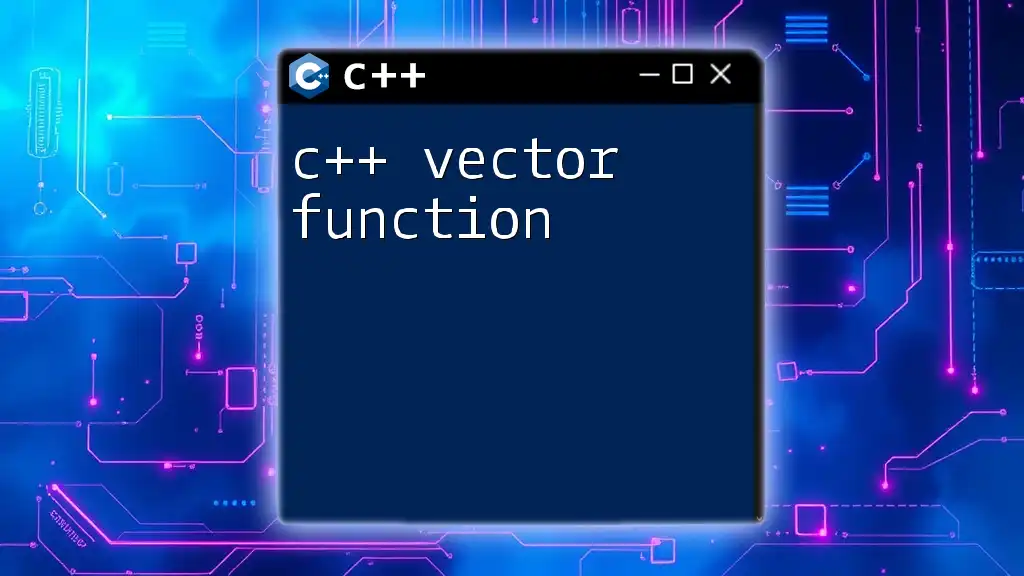
Advanced Topics
Pointers to Const Member Functions
In C++, you may need to work with const member functions, which don't modify the object they belong to. The syntax for a pointer to a const member function differs slightly:
void (ClassName::*ptr)() const = &ClassName::constMemberFunction;
This pointer can only call member functions marked `const`, making it safely usable in contexts where you want to ensure the object remains unchanged.
Pointers to Member Functions with Parameters
Just as with regular member functions, pointers can also be declared for member functions that accept parameters. This enables a more versatile approach to calling functions with varying inputs:
void (ClassName::*ptr)(int) = &ClassName::memberFunctionWithParam;
The process remains the same as before; you merely specify the parameters when declaring the function pointer.
Combining Pointers with Other Data Structures
You can use pointers to member functions in combination with Standard Template Library (STL) containers. For instance, you might create a vector of function pointers for handling a set of different event callbacks:
#include <vector>
class MyClass {
public:
void firstFunction() { /* implementation */ }
void secondFunction() { /* implementation */ }
};
std::vector<void (MyClass::*)()> functionPointers = {
&MyClass::firstFunction,
&MyClass::secondFunction
};
void callFunctions(MyClass& obj) {
for (auto funcPtr : functionPointers) {
(obj.*funcPtr)(); // Calling each member function
}
}
This example demonstrates how you can store multiple pointers to member functions and call them in a loop.
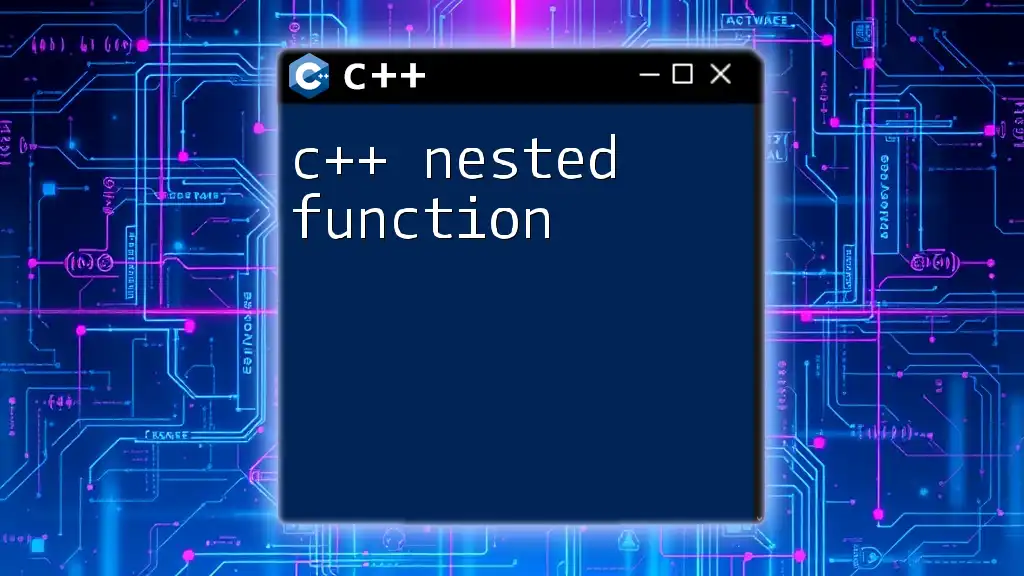
Common Mistakes and Troubleshooting
Pitfalls to Avoid
One common mistake with pointers to member functions is forgetting to dereference them correctly using `.` or `->`. Always make sure you use the appropriate syntax to avoid compilation errors or unexpected behavior.
Debugging Pointers to Member Functions
When debugging, pay attention to:
- Mismatched function signatures.
- Incorrect use of pointer dereferencing operators.
- Making sure the object instance is valid before calling the member function.
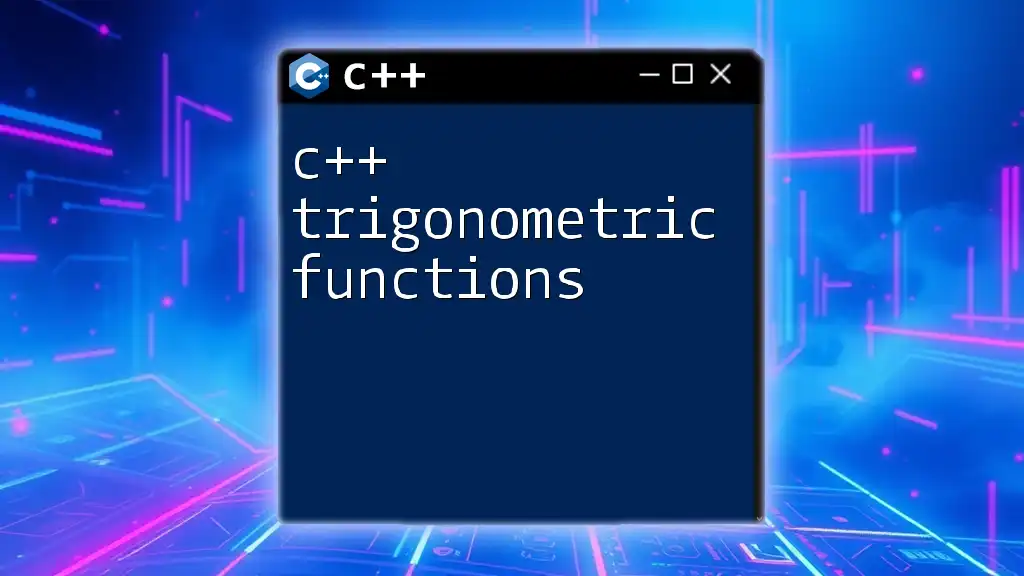
Conclusion
Understanding C++ pointers to member functions opens a variety of coding possibilities, including enhanced flexibility and dynamic behavior in your applications. Mastering this concept is critical for those looking to delve deeper into advanced C++ features.
Practice this concept through various exercises and soon, you will be comfortable using pointers to member functions as a powerful tool in your programming arsenal. With this foundational knowledge, you’ll be well-equipped to tackle more complex programming challenges.
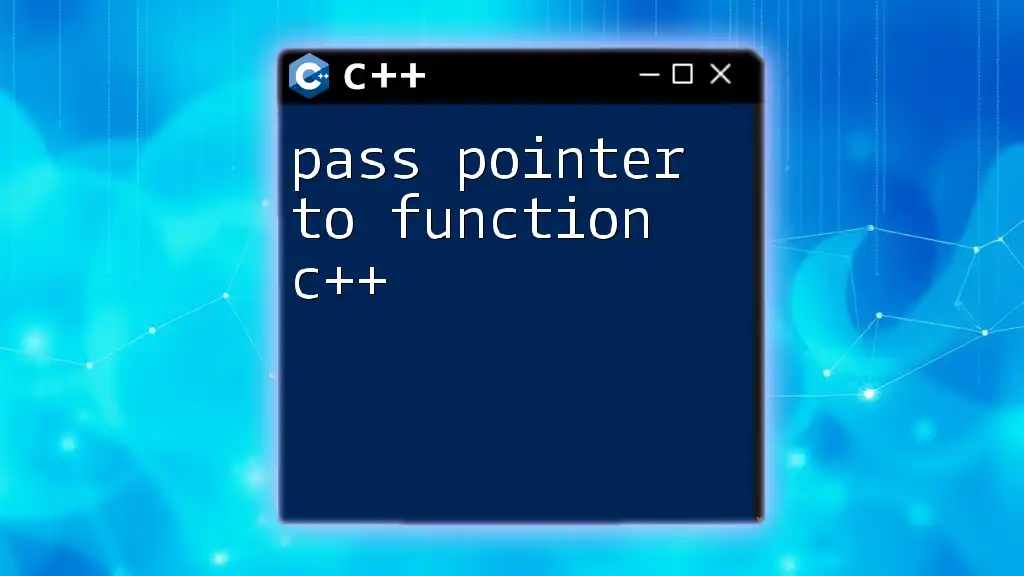
Further Reading and Resources
For readers eager to expand their knowledge further, consider exploring online courses that focus on C++ programming, advanced object-oriented principles, and memory management. Books such as "Effective C++" by Scott Meyers or "C++ Primer" by Stanley B. Lippman are excellent starting points for a deeper understanding of these topics.