In C++, static member functions belong to the class rather than any particular object, allowing them to be called without creating an instance of the class, and they can only access static data members.
#include <iostream>
class Example {
public:
static int staticValue;
static void display() {
std::cout << "Static Value: " << staticValue << std::endl;
}
};
int Example::staticValue = 10;
int main() {
Example::display(); // Calls the static member function
return 0;
}
Introduction to C++ Static Member Functions
C++ static member functions are special functions that belong to the class rather than any object instance. This means that they can be invoked without creating an object of the class. They play a crucial role in various situations, especially when you want to maintain shared data or offer utility functions associated with the class.
Use Cases
Static member functions are particularly useful in scenarios such as:
- Utility functions that perform operations not tied to object state.
- Factory methods that create instances of the class.
- Accessors for shared data among multiple instances, such as counters.
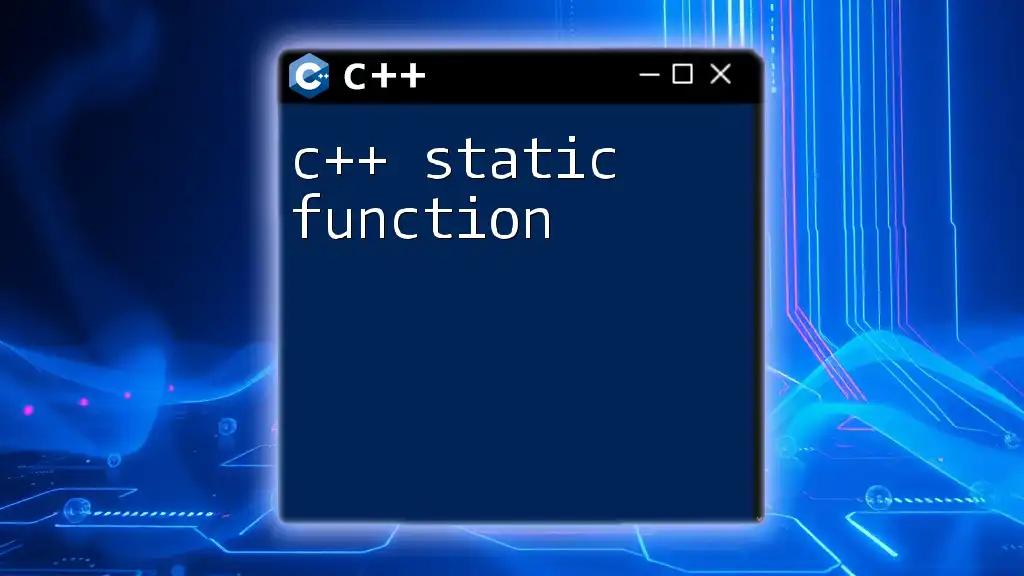
Understanding Static Members in C++
What are Static Members?
Static members in C++ are shared across all instances of a class. They exist separately from any specific object, meaning they are defined once and can be accessed through the class itself. This fundamental aspect allows for data to be isolated yet shared.
Memory Allocation for Static Members
Static members are allocated in a fixed memory location, distinguishing them from non-static members, which occupy space on an object’s heap. This allocation process contributes to enhanced performance, especially important when working with many instances of a class.

Defining a Static Member Function
Syntax for Static Member Functions
The syntax for defining a C++ static member function is straightforward. It involves the `static` keyword, declaring the function just like any member function but with an additional keyword. Here’s a glimpse at the syntax:
class MyClass {
public:
static void myStaticFunction();
};
In this example, `myStaticFunction` is declared as a static member function.
Accessing Static Members
You can access static member functions using the class name, directly without needing an instance of the class:
MyClass::myStaticFunction();
This capability emphasizes the simplicity of using static functions, allowing for cleaner code without the overhead of instantiating objects.

Characteristics of Static Member Functions
No Instance Dependency
A key characteristic of static member functions is their lack of dependency on class instances. This means they can operate without the need for any object state, making them suitable for utility operations or global functionality within the class context.
Can Only Access Static Data Members
Static member functions can only access static data members of the class. They cannot access non-static members directly since non-static members require an instance to be meaningful.
Here’s a demonstration with a code example:
class MyClass {
public:
static int staticData;
int instanceData;
static void staticFunction() {
// Allowed: Accessing static data member
staticData = 5;
// Not allowed: Accessing instance data member
// instanceData = 2; // This will cause a compilation error
}
};
In this example, you can see that `staticFunction` can modify the static member `staticData`, but attempting to access `instanceData` will lead to a compilation error.
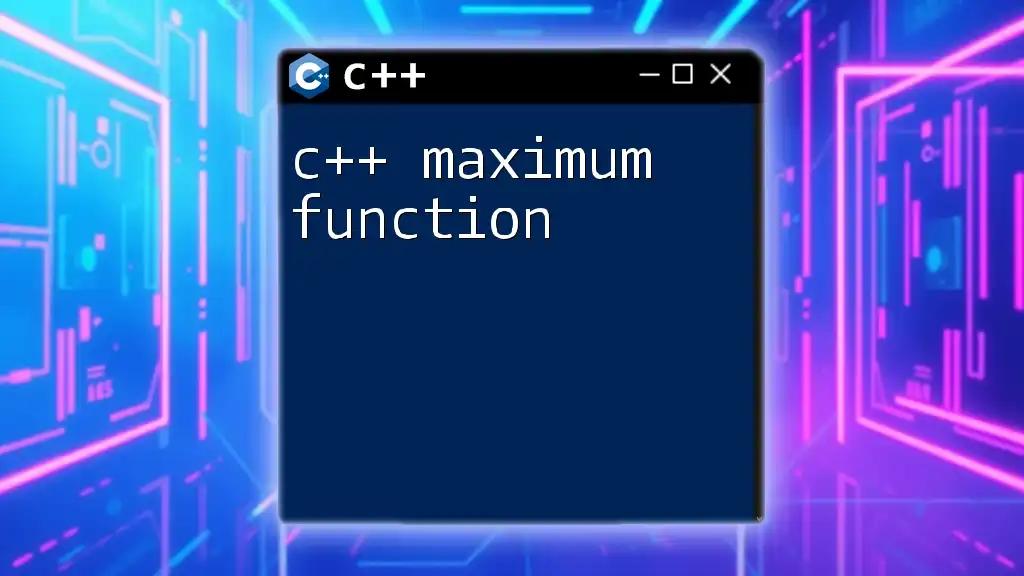
Creating and Using Static Member Functions
Step-by-step: Creating a Static Member Function
To create a static member function, follow these steps:
- Declare the Function: Use the `static` keyword in the class definition.
- Define the Function Outside the Class: This involves using the scope resolution operator to clarify that this function is a member of the class.
Here’s an example illustrating this process:
class Example {
public:
static void showMessage();
};
void Example::showMessage() {
std::cout << "Hello from static member function!" << std::endl;
}
Practical Example: A Simplified Counter Class
Consider a simple counter that tracks how many times a function has been called. Here’s how you could implement this using static member functions:
class Counter {
public:
static int count; // Static data member
static void increment() {
count++;
std::cout << "Count is now: " << count << std::endl;
}
};
// Initialization of static member
int Counter::count = 0;
int main() {
Counter::increment(); // Output: Count is now: 1
Counter::increment(); // Output: Count is now: 2
return 0;
}
In this example, `increment` is a static member function that modifies the static data member `count`. Each call to `increment` updates the shared count across all calls, demonstrating the utility of static members.
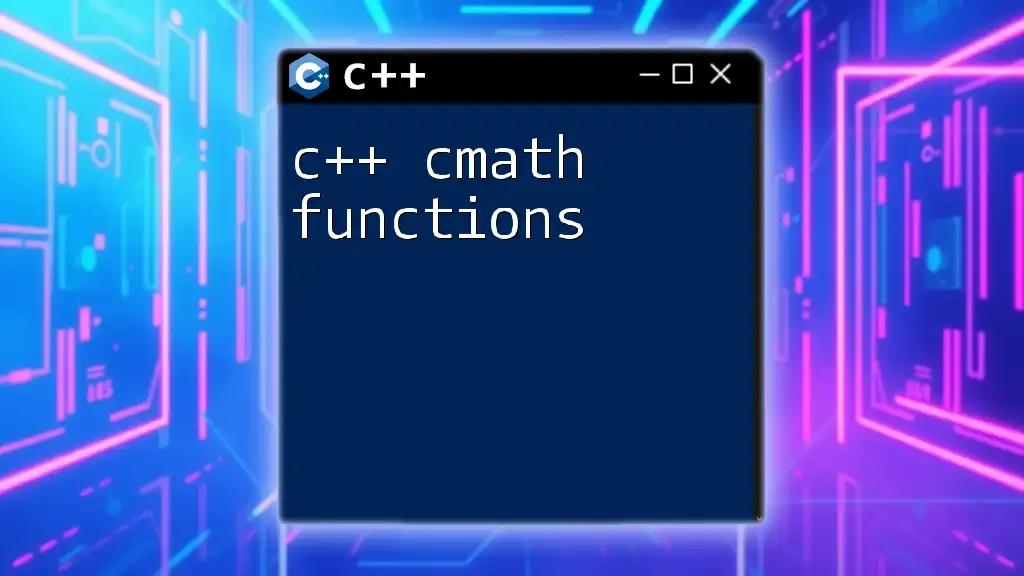
Best Practices for Static Member Functions
When to Use Static Member Functions
Use static member functions in the following cases:
- When you need to provide functionalities that do not depend on object state.
- When creating utility functions that require no instance data.
- When maintaining shared data, like a counter or configuration settings.
Common Pitfalls to Avoid
While static member functions are powerful, they can lead to complications if misused. Some common mistakes include:
- Overusing Static Members: This can lead to tightly coupled code and make unit testing difficult.
- Neglecting Object-Oriented Principles: Static member functions should not replace the polymorphism and encapsulation provided by instance methods.
Attention to these pitfalls helps maintain good coding practices while utilizing static member functions effectively.
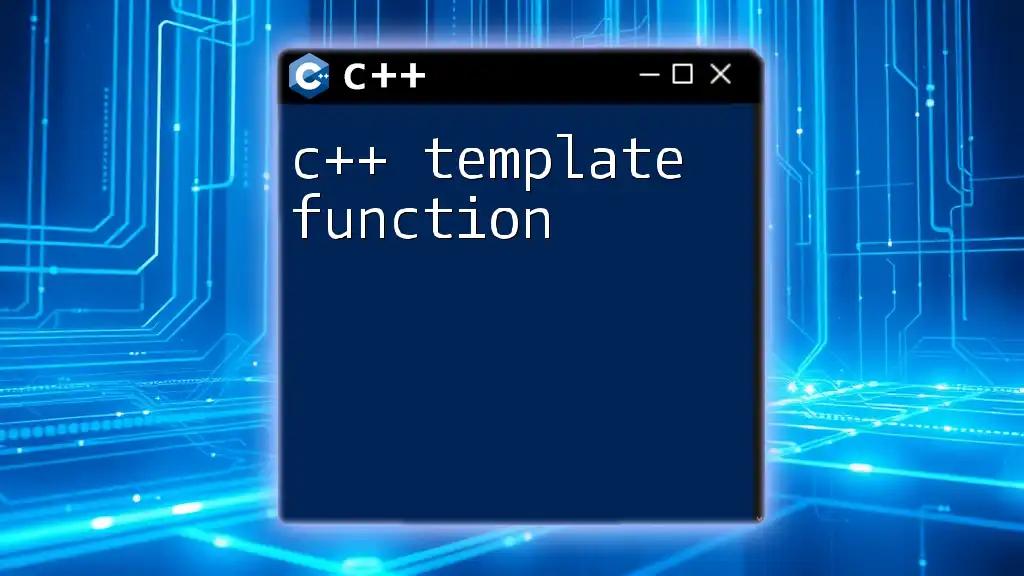
Static Member Functions in Inheritance
Static Functions in Derived Classes
Static member functions behave consistently across inheritance hierarchies. A static function declared in a base class can be accessed from a derived class, but it does not exhibit polymorphic behavior. Here’s an example:
class Base {
public:
static void show() {
std::cout << "Base show function." << std::endl;
}
};
class Derived : public Base {
public:
static void showDerived() {
std::cout << "Derived show function." << std::endl;
}
};
// Accessing static member functions
int main() {
Base::show(); // Output: Base show function.
Derived::showDerived(); // Output: Derived show function.
return 0;
}
Polymorphism and Static Functions
It’s important to note that static member functions cannot be virtual. This means that overriding a static member function in a derived class will not exhibit polymorphic behavior, which can be a source of confusion.
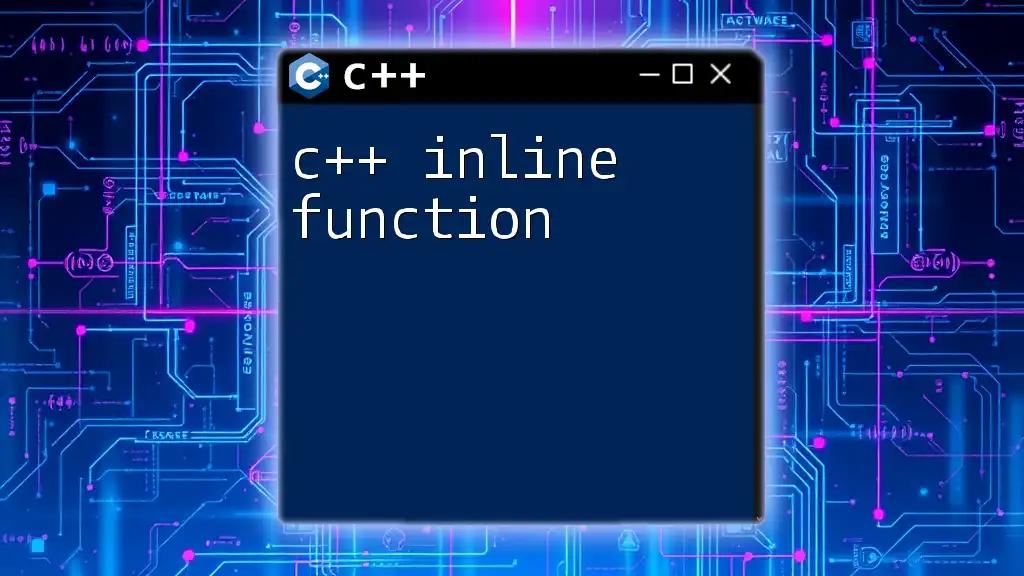
Conclusion
In summary, C++ static member functions offer a robust way to handle shared data and utility functions. They operate independently of class instances, providing versatility in the way functionalities are structured within a class. While they are a valuable addition to C++ programming, careful consideration should be given to their use to avoid pitfalls associated with rigid design and poor testability.
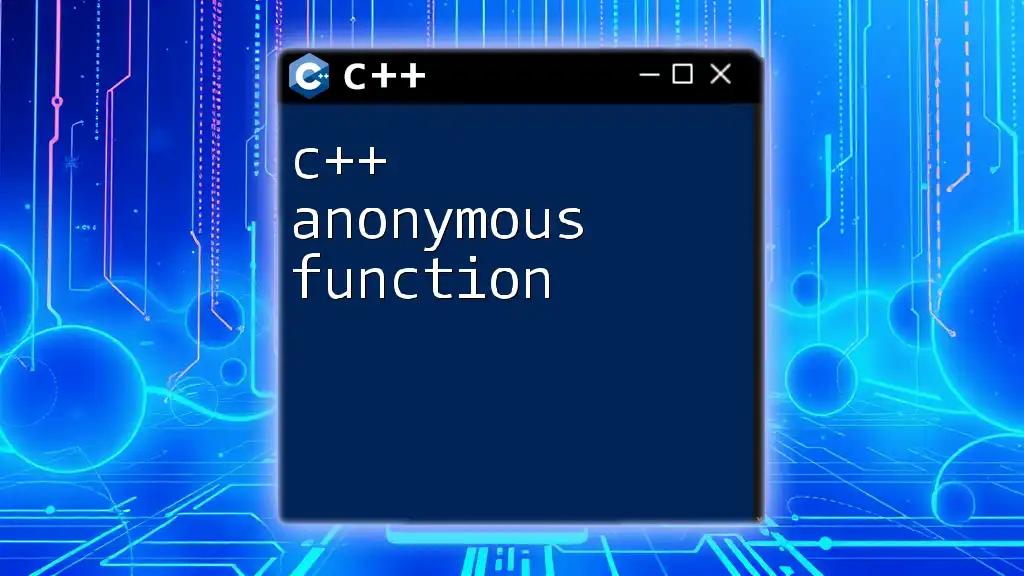
FAQs about C++ Static Member Functions
What is the difference between a static member function and a regular member function?
Regular member functions require an instance of the class to be called, while static member functions can be called using the class name without any instance.
Can static member functions be virtual?
No, static member functions cannot be virtual because they do not operate on specific instances, and thus cannot participate in polymorphism.
Are there any limitations to using static member functions?
Static member functions cannot access non-static data members directly and can lead to less flexible designs when overused, making it essential to evaluate their necessity as per project requirements.

Call to Action
We encourage readers to dive deeper into the world of static member functions by creating their own examples and experimenting with different scenarios. Share your insights and any queries in the comments section; we’re eager to learn about your experiences with C++ static member functions!