C++ static initialization refers to the process by which static and global variables are initialized before the program's execution begins, ensuring they have defined values before use.
Here's a simple example:
#include <iostream>
static int staticVar = 10; // Static initialization
int main() {
std::cout << "Static Variable: " << staticVar << std::endl;
return 0;
}
What is Static Initialization in C++?
C++ static initialization refers to the initialization of variables that are declared with the `static` keyword. This means that they retain their value between function calls and persist for the lifetime of the program. Understanding static initialization is crucial as it affects how data is managed in C++.
Key takeaway: Static data persists for the duration of the program, providing a way to keep track of state across multiple function invocations.
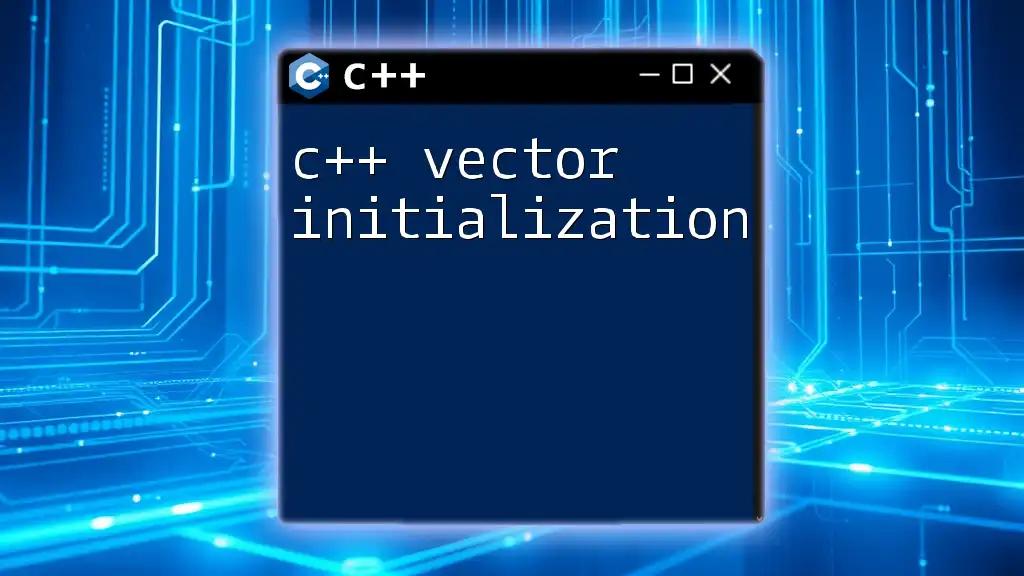
Types of Static Initialization
Static Initialization of Local Variables
When a local variable is declared as static, it is initialized only once, the first time that the function is called. Its lifetime extends beyond the function call, preserving its value for the next invocation.
Consider the following code example:
void function() {
static int count = 0; // initialized only once
count++;
std::cout << count << std::endl;
}
In this example, each time `function()` is called, the value of `count` persists. The output will increment with each call, demonstrating how static local variables maintain their state.
Static Initialization of Global and Namespace Variables
Global variables that are declared with the `static` keyword are initialized before entering the `main()` function. Their scope is limited to the translation unit, which means they are not visible to other files.
Here’s a simple code example:
static int globalVar = 10; // initialized before main
This variable is available throughout this translation unit but cannot be accessed from other translation units, providing encapsulation to your global state.
Static Initialization in Classes
In C++, static data members of a class belong to the class itself, rather than to any specific instance. Their initialization needs to occur outside the class definition.
For example:
class MyClass {
public:
static int staticVar;
};
// Static member initialization
int MyClass::staticVar = 100; // initialized outside the class
Here, `staticVar` is shared among all instances of `MyClass`, and it is initialized only once.
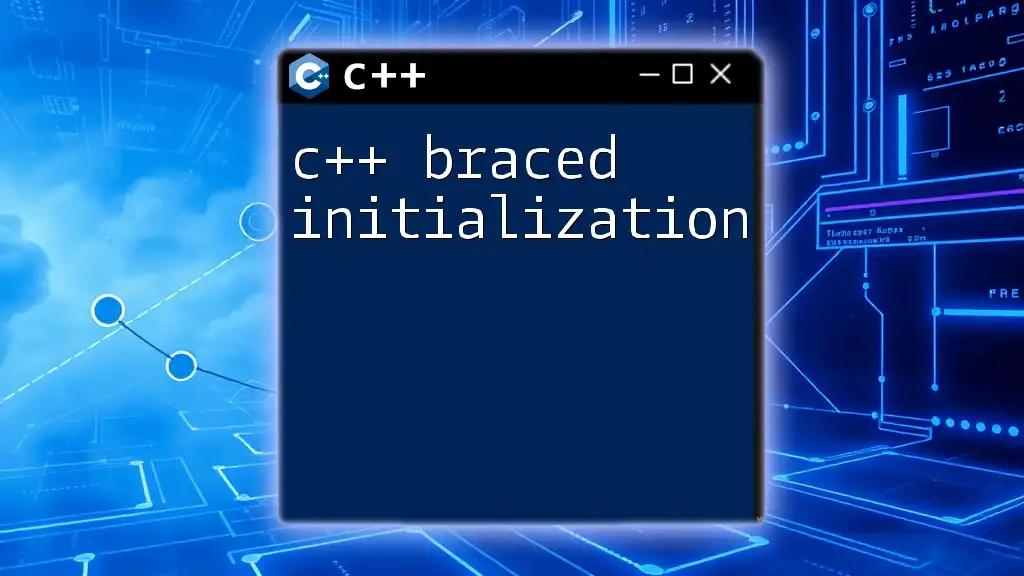
Static Initializers in C++
What is a C++ Static Initializer?
A C++ static initializer is a specific way to initialize static members or variables using various forms. Understanding these initializers is vital for effectively managing your program's state.
Different Forms of Static Initializers
Zero Initialization
Static variables that aren’t explicitly initialized get automatically zero-initialized by default. This ensures that all bits are set to zero.
Example:
static int count{}; // all bits set to zero
In this case, `count` will start at 0.
Constant Initialization
For constant static variables, you can provide a fixed value during declaration. This value is then immutable throughout the program.
Example:
static const int MAX_SIZE = 100; // constant static initialization
Using `const` here ensures that `MAX_SIZE` cannot be altered.
Aggregate Initialization
This type refers to initializing arrays or structures with defined initial values. It is straightforward and practical for ensuring arrays start with a specific set of data.
Example:
static int arr[5] = {1, 2, 3, 4, 5}; // static array initialization
This guarantees that all elements of `arr` are set upon initialization.

How Static Initialization Works Under the Hood
Static variables are initialized only once, and their initialization order is defined by their translation unit. This means that C++ guarantees static members are initialized before any function that refers to them is executed.
Key takeaway: All global and static variables within a translation unit are initialized before the execution of `main()`. However, care must be taken with dependencies between different translation units, as this can lead to a phenomenon known as the "Static Initialization Order Fiasco." This situation occurs when the initialization order breaks some expectations, potentially allowing a static variable to be used before it is initialized.

Best Practices for Static Initialization
Avoid Global Mutable State
While static variables can provide convenience, relying on them can create mutable global states that can lead to bugs. Immutable data structures or encapsulated classes are often safer alternatives, especially in multi-threaded environments.
Order of Initialization Concerns
To mitigate issues arising from initialization order across translation units, consider grouping related static variables in the same file or using a function to encapsulate the initialization.
Use of Singleton Pattern
The Singleton pattern allows you to create a single instance of a class and provides a global point of access to that instance. This pattern effectively relies on static initialization for its implementation.
Example:
class Singleton {
public:
static Singleton& getInstance() {
static Singleton instance; // Guaranteed to be destroyed.
return instance;
}
private:
Singleton() {} // Constructor is private
};
Here, the static instance of `Singleton` is initialized the first time `getInstance()` is called, ensuring thread safety and single instance creation.
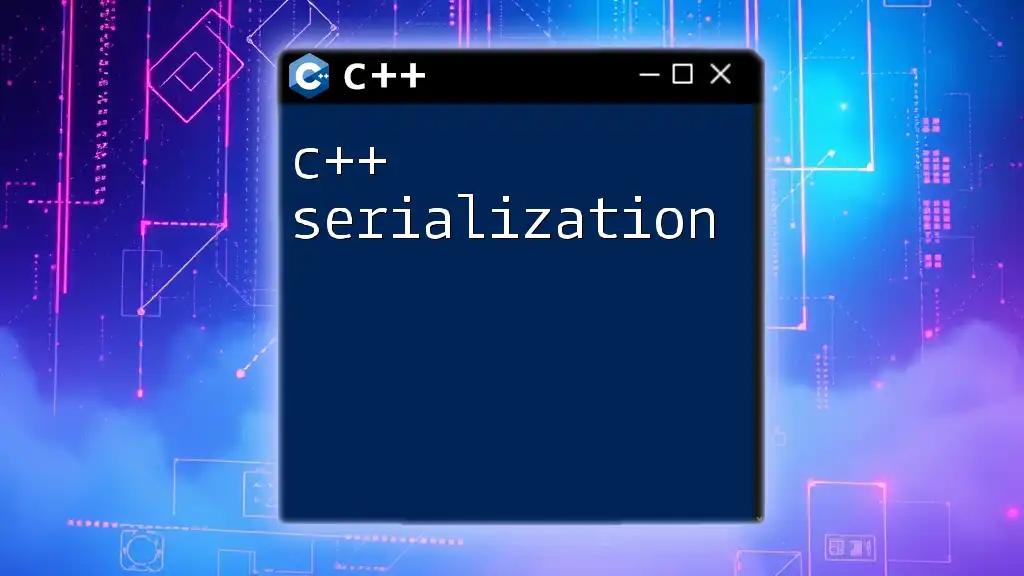
Common Pitfalls in Static Initialization
Uninitialized Static Variables
If you fail to initialize a static variable, its value will be indeterminate, leading to undefined behavior.
Example:
static int uninitializedVar; // May contain garbage value.
Always ensure that static variables are explicitly initialized to avoid such pitfalls.
Dependency on Global State
Static variables can inadvertently depend on other global variables for initialization. This can lead to unpredictable behavior, especially if the order of initialization is not controlled. It’s advisable to design with fewer dependencies on global variables whenever possible.

Conclusion
Understanding C++ static initialization is essential for managing state efficiently in your programs. By leveraging static variables, initializing data correctly, and following best practices, you can write more robust and maintainable code. As you continue to explore C++, consider how these concepts can fit into your larger designs. Incorporate these principles to enhance your programming practices and improve your solutions.