Initialization in C++ refers to the process of assigning an initial value to a variable at the time of its declaration, which can be done using various methods such as direct initialization, copy initialization, and uniform initialization.
Here’s a code snippet demonstrating different initialization methods:
#include <iostream>
int main() {
int a = 5; // Direct initialization
int b(10); // Copy initialization
int c{15}; // Uniform initialization (C++11 and later)
std::cout << "a: " << a << ", b: " << b << ", c: " << c << std::endl;
return 0;
}
What is Variable Initialization?
Initialization refers to the process of assigning a specific value to a variable at the time of its creation. This is a critical step in ensuring that variables hold defined values, thus avoiding unpredictable behaviors in a program. It’s also important to differentiate declaration from initialization—declaring a variable just tells the compiler about its existence, while initialization assigns a value to it.
Why is Variable Initialization Important?
Without proper initialization, variables may contain indeterminate values, leading to undefined behavior in your program. For instance, trying to use an uninitialized variable in arithmetic operations could yield unpredictable results, making debugging a nightmare.
When you initialize variables correctly, you ensure predictable program behavior, which is vital for building reliable, maintainable software.
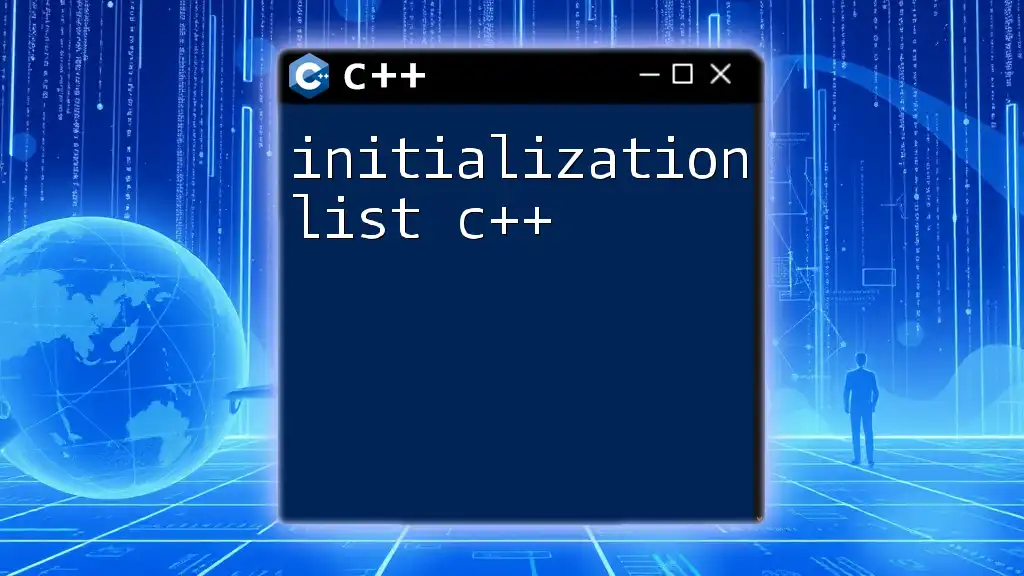
Types of Initialization in C++
C++ offers several ways to initialize variables:
Default Initialization
Default initialization occurs when a variable is declared but not explicitly initialized. The resulting value is typically indeterminate. For example:
int a; // Default initialized (value is indeterminate)
It’s essential to avoid using such variables before assigning them a defined value.
Value Initialization
Value initialization assigns a default value to a variable when using braces `{}` or `()` with no arguments. In the case of fundamental data types, it will initialize them to zero. For example:
int b{}; // Value initialized (value is 0)
This technique can improve code clarity and safety.
Copy Initialization
In copy initialization, a variable is initialized using the `=` operator. The right-hand side value is copied into the variable. This is common and straightforward:
int c = 5; // Copy initialized with the value 5
This form is versatile and often seen in standard coding practices.
Direct Initialization
Direct initialization involves using parentheses to initialize a variable directly with a specified value. This method avoids potential ambiguity with types:
int d(10); // Directly initialized with 10
Direct initialization is particularly useful when creating objects of user-defined types.
Aggregate Initialization
C++ also supports aggregate initialization for arrays and structs, where multiple elements can be initialized simultaneously. This is often accomplished using curly braces `{}`:
int arr[3] = {1, 2, 3}; // Aggregate initialized array
This approach can help maintain the clarity of data structures, especially when dealing with collections.
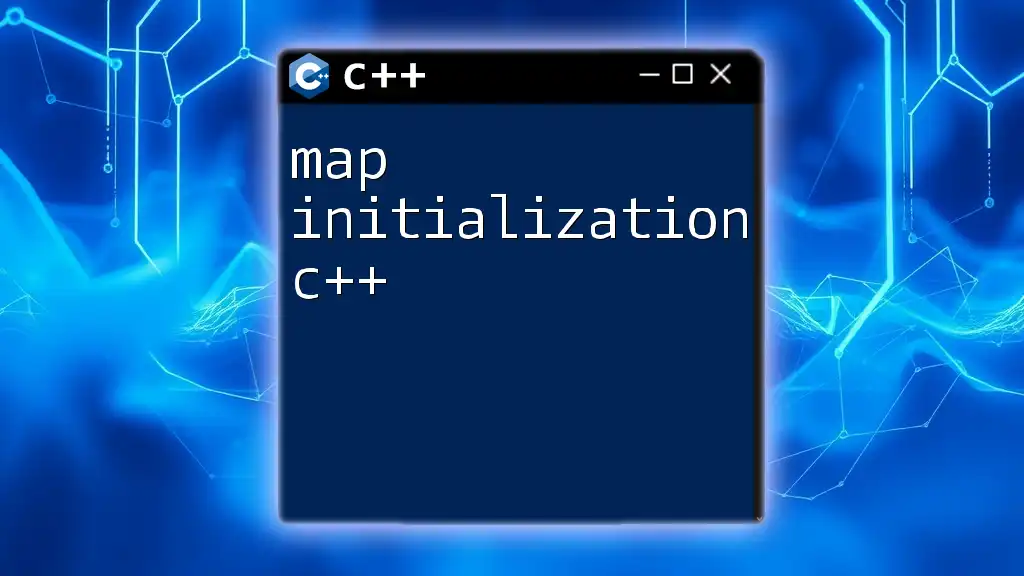
How to Initialize Variables in C++
Using Constructors with Classes
When working with user-defined types (classes), constructors are commonly used for initialization. Constructors are special functions that can take initial parameters and set class member variables upon instantiation. For example:
class MyClass {
public:
int x;
MyClass(int val) : x(val) {} // Constructor initializing 'x'
};
MyClass obj(42);
This approach enables you to enforce initialization logic, ensuring that an object is correctly set up.
Using `std::vector` for Initialization
C++ Standard Template Library (STL) provides flexible data structures such as `std::vector`. You can initialize vectors directly using a list of values:
#include <vector>
std::vector<int> vec = {1, 2, 3, 4}; // Vector initialization
Vectors automatically manage memory and provide an easy way to initialize a dynamic array.
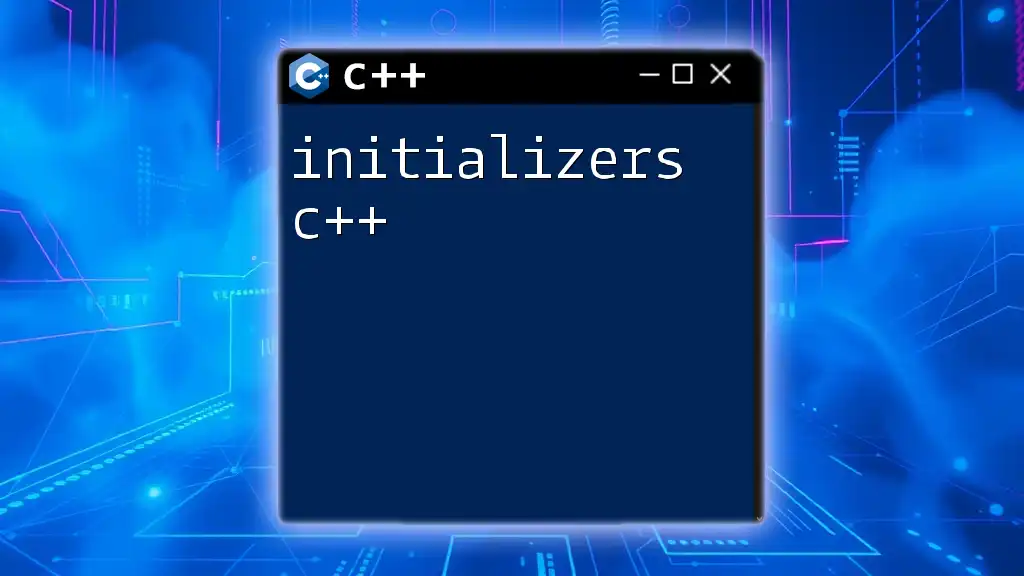
Initialization Best Practices
What to Avoid: Uninitialized Variables
One of the most critical aspects of programming in C++ is to avoid uninitialized variables. Using an uninitialized variable can lead to undefined behavior and cause errors that are difficult to diagnose. Consider the following example:
int e; // Uninitialized variable leads to undefined behavior
Always initialize your variables before use.
Use of `nullptr` in Pointer Initialization
Using `nullptr` when dealing with pointers is a good practice to ensure that your pointers are initialized to a known state. This signifies that the pointer is not currently pointing to a valid location:
int* ptr = nullptr; // Pointer initialized to nullptr
This helps prevent dereferencing a garbage pointer, making your code safer.
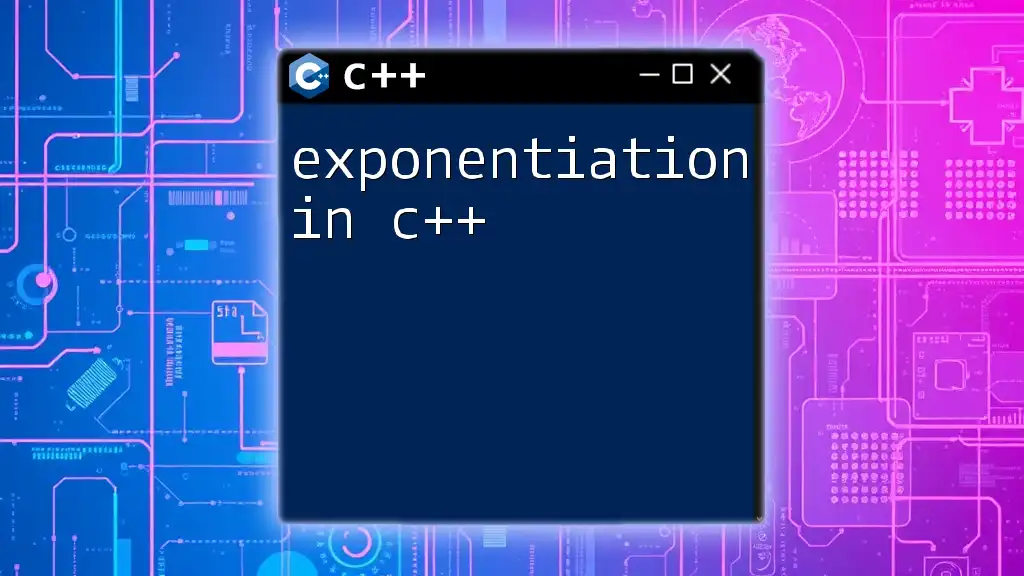
Conclusion
In conclusion, understanding initialization in C++ is fundamental for any developer. The various types of initialization techniques—from default and value initialization to constructors and STL container initialization—provide you with powerful methods to ensure your variables and objects are set up correctly.
By following best practices such as avoiding uninitialized variables and utilizing smart pointer initialization, you will significantly enhance the reliability of your software. Mastering initialization techniques will not only improve code clarity but also reinforce safer programming patterns.