In C++, exponentiation can be performed using the `pow` function from the `<cmath>` library, which raises a base to the power of an exponent.
#include <iostream>
#include <cmath>
int main() {
double base = 2.0;
double exponent = 3.0;
double result = pow(base, exponent);
std::cout << base << " raised to the power of " << exponent << " is " << result << std::endl;
return 0;
}
Understanding Exponentiation
What is Exponentiation?
Exponentiation is a mathematical operation that involves two numbers: a base and an exponent. The base is the number you multiply, and the exponent indicates how many times to multiply the base by itself. For example, in the expression \(2^3\), 2 is the base, and 3 is the exponent, which means \(2 \times 2 \times 2 = 8\).
In programming, especially in C++, exponentiation is widely used for various calculations. Understanding this concept is fundamental to effectively implementing mathematical models and algorithms.
Real-World Applications
Exponentiation is a vital operation in many fields, including:
- Scientific calculations: Physics and chemistry often require calculations involving exponents, such as calculating forces and reaction rates.
- Financial modeling: Exponential growth is a common phenomenon in finance, particularly in areas like compound interest calculations.
- Algorithms and data structures: Certain algorithms, such as those used in sorting and searching, may require exponentiation for efficiency.
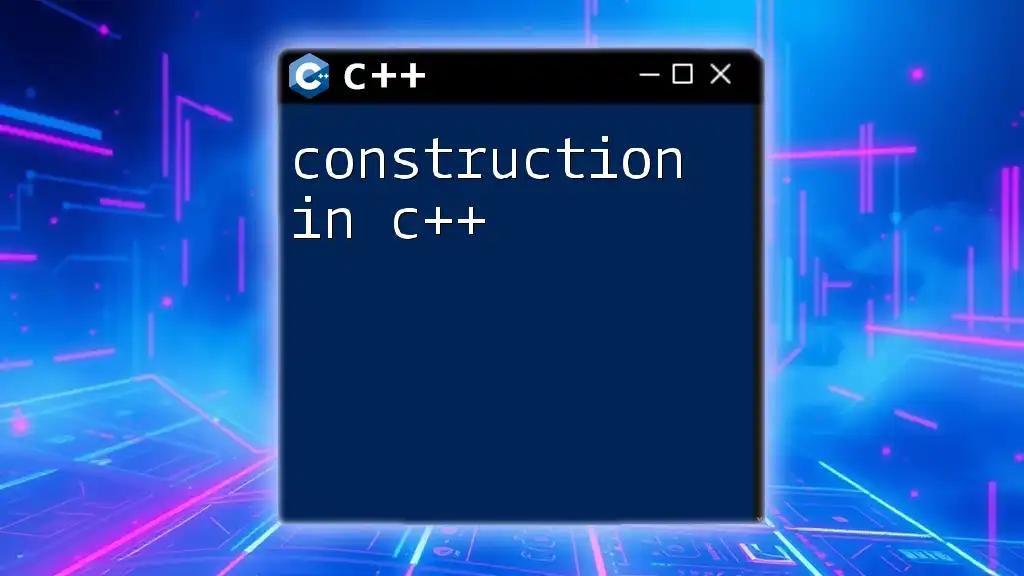
Methods for Exponentiation in C++
Using the `pow` Function
The simplest way to perform exponentiation in C++ is by utilizing the `pow` function from the standard math library.
Syntax and Parameters
The `pow` function is defined as follows:
double pow(double base, double exponent);
Here, both the base and the exponent are specified as doubles, which also allows for fractional exponents.
Example Usage
Let’s see a basic example of using the `pow` function:
#include <iostream>
#include <cmath>
int main() {
double base = 2.0;
double exponent = 3.0;
double result = pow(base, exponent);
std::cout << base << " raised to the power of " << exponent << " is " << result << std::endl;
return 0;
}
In the example above, we calculate \(2^3\) and output the result. This function handles both positive and negative exponents automatically.
Handling Different Data Types
When using `pow`, it's essential to note that it can cause implicit type conversion. If you pass integers, they will be converted to doubles. However, for integer outputs, consider using alternative methods discussed later.
Using Loops for Exponentiation
Implementing Exponentiation with a `for` Loop
Another straightforward approach to handle exponentiation in C++ is to use a loop. This is beneficial for better control over the process and can be implemented in various ways.
Example Code
Here’s an example that uses a `for` loop:
#include <iostream>
double power(double base, int exponent) {
double result = 1.0;
for (int i = 0; i < exponent; i++) {
result *= base;
}
return result;
}
int main() {
std::cout << "2 raised to the power of 4 is " << power(2.0, 4) << std::endl;
return 0;
}
In this code, the `power` function iteratively multiplies the base by itself for the number of times specified by the exponent.
Implementing Exponentiation with a `while` Loop
You can also use a `while` loop for this calculation. This provides similar functionality with a different control structure.
Example Code
Here’s how it would look:
#include <iostream>
double power(double base, int exponent) {
double result = 1.0;
while (exponent > 0) {
result *= base;
exponent--;
}
return result;
}
int main() {
std::cout << "3 raised to the power of 3 is " << power(3.0, 3) << std::endl;
return 0;
}
Optimizing Exponentiation with Recursion
Recursion is another method that can simplify the implementation and make the code cleaner when performing exponentiation.
Example Code
Here’s an example of a recursive implementation of the exponentiation function:
#include <iostream>
double power(double base, int exponent) {
if (exponent == 0) return 1; // Base case for exponent zero
return base * power(base, exponent - 1);
}
int main() {
std::cout << "5 raised to the power of 2 is " << power(5.0, 2) << std::endl;
return 0;
}
In this example, the function multiplies the base by the result of the same function called with a decremented exponent until it reaches zero.

Advanced Topics
Handling Negative Exponents
When dealing with negative exponents, remember that mathematical principles dictate that \(a^{-n} = \frac{1}{a^n}\). Thus, the implementation must consider this to avoid conceptual errors.
Example Code with `pow` Function
Consider how the `pow` function handles negative exponents:
#include <iostream>
#include <cmath>
int main() {
double base = 2.0;
double exponent = -3.0;
double result = pow(base, exponent);
std::cout << base << " raised to the power of " << exponent << " is " << result << std::endl;
return 0;
}
Integer Overflows in Exponentiation
While performing exponentiation, especially with large integers, you should be cautious of potential overflows. When the result of exponentiation exceeds the maximum value representable by the target data type, it can result in undefined behavior or unexpected results.
Example of Overflow
Here is an example to illustrate integer overflow:
#include <iostream>
int main() {
int base = 2;
int exponent = 31; // This likely causes overflow
long long result = 1;
for (int i = 0; i < exponent; i++) {
result *= base; // Potential overflow
}
std::cout << "Result (may overflow): " << result << std::endl;
return 0;
}
In this case, `result` is defined as a `long long` type to handle larger values, but this doesn’t fully prevent overflow when the exponent is significantly large.
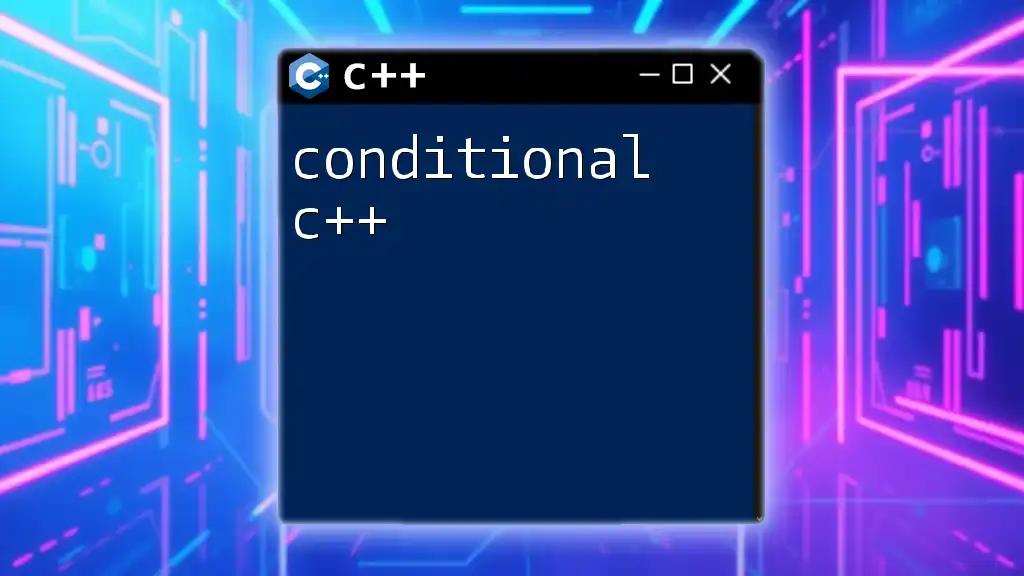
Conclusion
In this article, we explored a wide range of methods to perform exponentiation in C++. From using the `pow` function to implementing loops and recursion, each method has unique advantages and should be chosen based on the specific requirements of your project. We also discussed advanced considerations, such as handling negative exponents and the risk of integer overflow.
By grasping how exponentiation works in C++, you can maximize your programming potential, especially in fields that require intensive numerical computations. Use the provided examples and best practices to strengthen your coding skills and implement robust applications effectively.

FAQ
Can I use negative bases?
Yes, you can use negative bases in exponentiation. However, be cautious about the sign of the result based on whether the exponent is even or odd.
What library do I need for `pow`?
You need to include the `<cmath>` library to use the `pow` function in C++.
How to use exponentiation in templates?
Exponentiation can be incorporated into C++ templates, allowing for greater flexibility in terms of base and exponent types. Here’s a simple example:
#include <iostream>
template <typename T>
T power(T base, int exponent) {
T result = 1;
for (int i = 0; i < exponent; i++) {
result *= base;
}
return result;
}
int main() {
std::cout << "2 raised to the power of 3 is " << power(2.0, 3) << std::endl;
return 0;
}
This provides a versatile way to calculate powers for varying data types.