Expressions in C++ are combinations of variables, operators, and function calls that are evaluated to produce a value.
Here's a simple example:
int a = 5;
int b = 10;
int sum = a + b; // This expression calculates the sum of a and b
Understanding C++ Expressions
What is a C++ Expression?
In C++, an expression is a combination of variables, operators, and values that the compiler evaluates to produce another value. Expressions are the fundamental building blocks in programming, allowing developers to manipulate data and implement logic. For example, in the expression `5 + 3`, the compiler calculates the sum to produce the value `8`.
Expressions can involve various operations such as arithmetic, logical, and relational comparisons. They are critical for executing computations, making decisions, and controlling the flow of programs.
Types of Expressions in C++
C++ allows several types of expressions, each serving different purposes. Understanding these types can significantly enhance your programming capability. The main types of expressions include:
- Arithmetic Expressions
- Relational Expressions
- Logical Expressions
- Bitwise Expressions
- Conditional Expressions
- Assignment Expressions

Arithmetic Expressions
Introduction to Arithmetic Expressions
Arithmetic expressions involve numeric values and perform mathematical operations. The basic arithmetic operators are `+` (addition), `-` (subtraction), `*` (multiplication), `/` (division), and `%` (modulus). These operators can be combined in various ways to perform complex calculations.
Example of Arithmetic Expressions
int a = 5;
int b = 10;
int result = a + b; // result is 15
In this example, `result` holds the sum of `a` and `b`. Such expressions are frequently used in programming to perform calculations and manipulate data.
Operator Precedence and Associativity
Operator precedence determines the order in which different operations are performed in an expression. For example, multiplication and division have higher precedence than addition and subtraction, which affects the evaluation order:
int result = 5 + 3 * 2; // result is 11, not 16
Associativity defines the direction in which operators of the same precedence are evaluated. For most operators, associativity is left-to-right, while the assignment operator `=` is right-to-left.

Relational Expressions
What are Relational Expressions?
Relational expressions compare two values and yield a boolean result (true or false). They are crucial for decision-making in programs. Common relational operators include:
- `==` (equal to)
- `!=` (not equal to)
- `<` (less than)
- `>` (greater than)
- `<=` (less than or equal to)
- `>=` (greater than or equal to)
Practical Example of Relational Expressions
int x = 10;
int y = 20;
bool isGreater = (x > y); // isGreater is false
Here, `isGreater` evaluates to `false` because `x` is not greater than `y`. Relational expressions are often used in conditional statements to control the flow of execution based on certain criteria.

Logical Expressions
The Role of Logical Expressions
Logical expressions combine one or more boolean values to produce a single boolean result. These expressions are essential for constructing complex logical operations. Key logical operators include:
- `&&` (logical AND)
- `||` (logical OR)
- `!` (logical NOT)
Example of Logical Expressions
bool conditionA = true;
bool conditionB = false;
bool result = conditionA && conditionB; // result is false
In this case, `result` is `false` because both conditions must be true for the logical AND operator to yield true. Logical expressions allow for improved decision-making capabilities within programs.

Bitwise Expressions
Understanding Bitwise Operators
Bitwise expressions perform operations on the binary representations of integers. These expressions allow manipulation of data at the bit level. Common bitwise operators include:
- `&` (bitwise AND)
- `|` (bitwise OR)
- `^` (bitwise XOR)
- `~` (bitwise NOT)
- `<<` (left shift)
- `>>` (right shift)
Example of Bitwise Expressions
int bitA = 5; // 0101 in binary
int bitB = 3; // 0011 in binary
int bitwiseResult = bitA & bitB; // result is 1 (0001 in binary)
In this example, the `&` operator compares the bits of `bitA` and `bitB`, resulting in `1` because only the least significant bit is set in both numbers. Bitwise operations are often used for tasks involving low-level programming, such as device control and data compression.
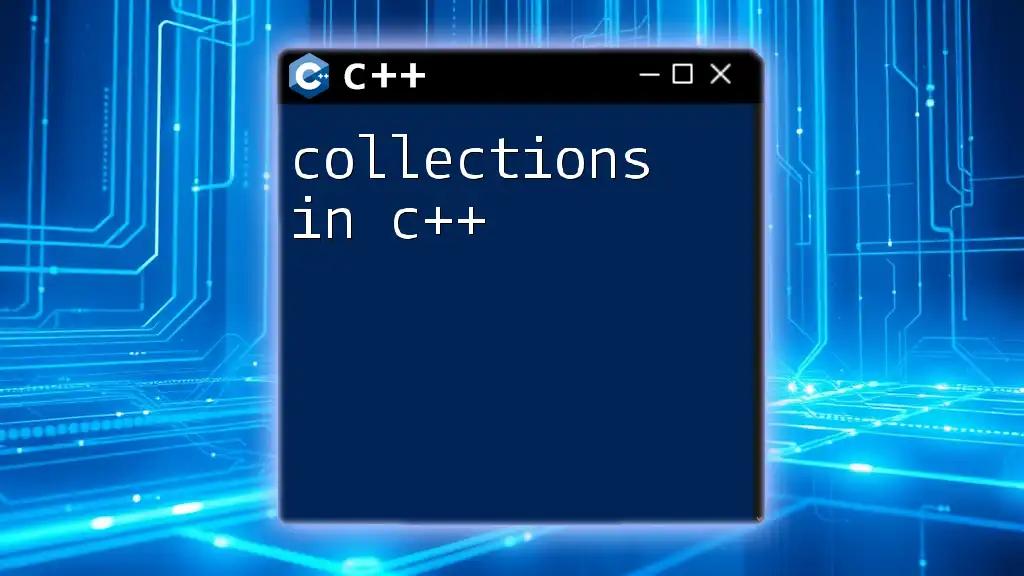
Conditional Expressions
What are Conditional Expressions?
Conditional expressions, often referred to as ternary expressions, are shorthand for if-else statements. They allow for inline conditional logic without bulky syntax. The syntax uses the form:
(condition) ? (true_expression) : (false_expression)
Example of a Conditional Expression
int age = 20;
std::string eligibility = (age >= 18) ? "Eligible" : "Not Eligible"; // Eligibility is "Eligible"
In this example, `eligibility` is set based on whether `age` meets the condition, showcasing the power of conditional expressions in making your code concise and readable.
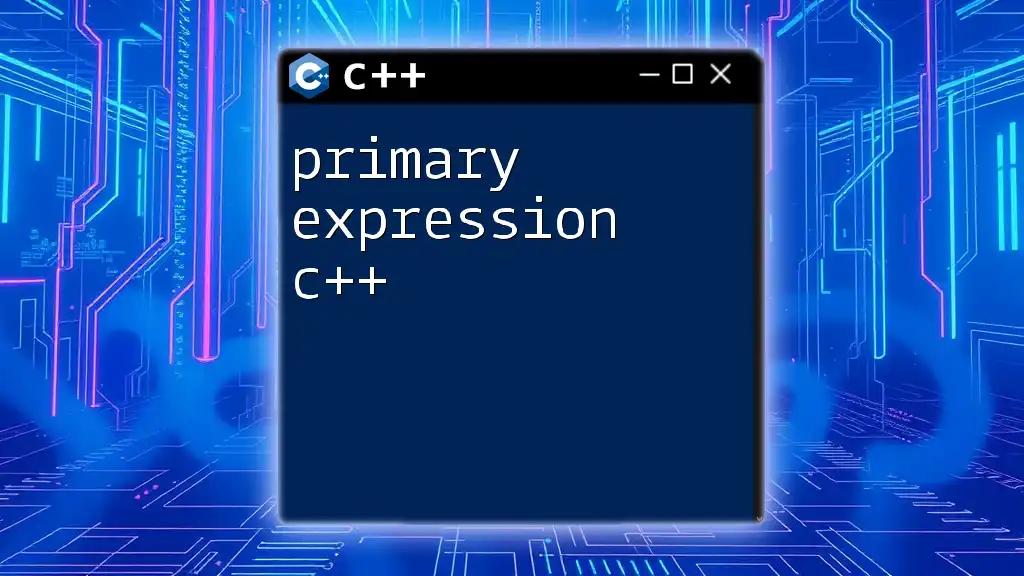
Assignment Expressions
The Role of Assignment Expressions
Assignment expressions assign values to variables using the assignment operator `=` and its derivatives. They allow variables to receive data, and compound assignment operators such as `+=`, `-=`, `*=`, and `/=` streamline this functionality.
Example of Assignment Expressions
int value = 10;
value += 5; // value is now 15
This example illustrates how `value` is updated directly with a single expression, enhancing clarity and brevity in code writing.

Using Expressions in Control Structures
How Expressions Control Flow
Expressions play a pivotal role in control structures like if statements, loops, and switch cases. They control the flow of execution based on evaluated conditions. For instance, consider the following if statement:
if (value > 0) {
std::cout << "Positive Value" << std::endl;
}
Here, the condition `(value > 0)` is a relational expression that determines whether the block of code executes, showing how expressions dictate the behavior of programs based on input values.

Conclusion
Recap of Key Concepts
In summary, expressions in C++ range from simple arithmetic to complex logical constructs, providing the necessary tools for evaluating conditions, performing computations, and designing control flows. Each type of expression is instrumental in everyday programming tasks.
Practical Applications
Mastering expressions enhances programming skills, allowing for more efficient, readable, and robust code. Areas such as game development and systems programming can significantly benefit from a strong grasp of C++ expressions, opening the door to creative solutions and effective problem-solving.
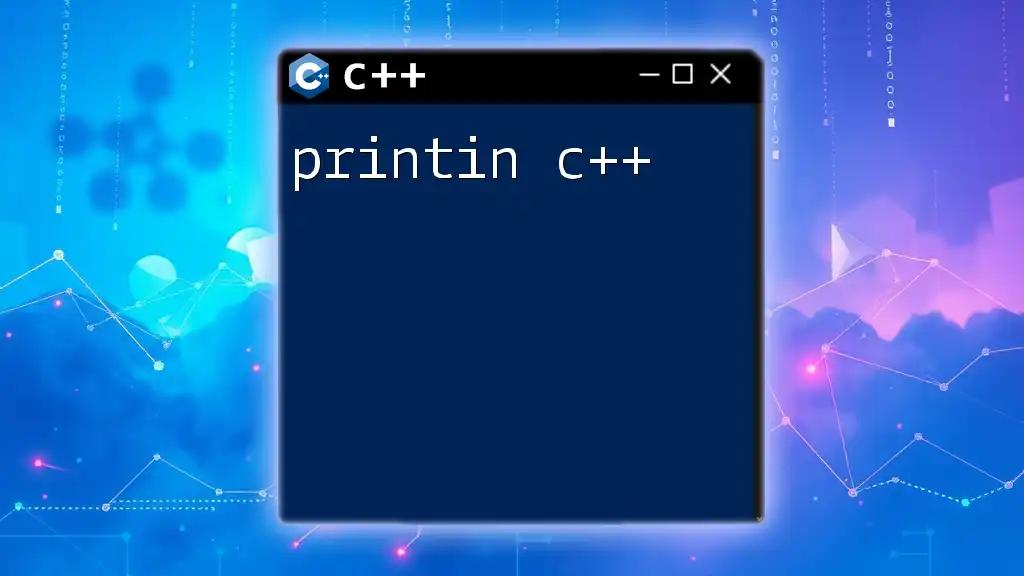
Additional Resources
Recommended Reading and References
For further exploration of expressions in C++, consider resources such as comprehensive C++ programming textbooks, online tutorials from reputable websites, and the official C++ documentation.
Practice Exercises
To reinforce your understanding, engage with sample exercises that challenge you to write and evaluate various types of C++ expressions. Practicing through real coding scenarios strengthens your grasp on utilizing expressions efficiently within your programs.