In C++, primary expressions are the simplest forms of expressions that can stand alone, including literals, identifiers, and certain operators.
Here’s a brief code snippet demonstrating some primary expressions:
#include <iostream>
int main() {
int x = 5; // Identifier
std::cout << x; // Identifier as primary expression
std::cout << 10; // Integer literal as primary expression
return 0;
}
What is a Primary Expression in C++?
Primary expressions in C++ are the simplest forms of expressions, serving as the fundamental building blocks for more complex expressions and statements. Understanding primary expressions is crucial for anyone looking to master C++, as they provide the essential components used in computations, variable assignments, function calls, and control statements.
At their core, primary expressions can be actual values (like numbers or characters), identifiers (such as variable names), or specialized keywords (like `this`). They are evaluated first in the scope of other expressions, determining how operations are conducted based on their values, types, and positions in the code.
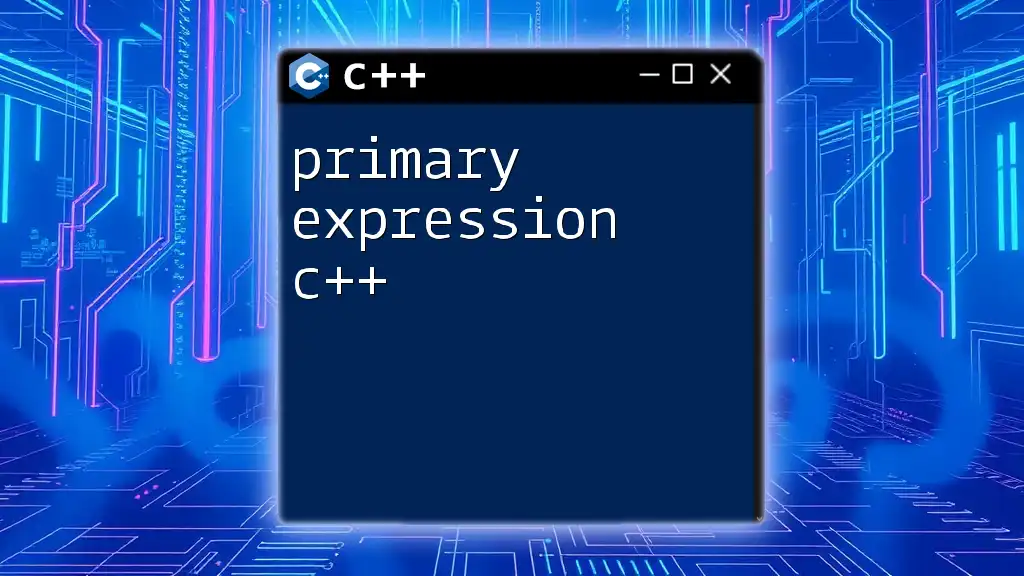
Types of Primary Expressions
Literals
Literals are fixed values directly represented in your code. They are essential for initializing variables, performing operations, or passing arguments to functions. There are several types of literals in C++, each with unique properties:
-
Integer Literals: These represent whole numbers. For example, `42` is an integer literal.
int num = 42; // Assigning an integer literal to a variable
-
Floating-point Literals: Used for representing numbers with a decimal point. For instance, `3.14` is a floating-point literal.
double pi = 3.14; // Assigning a floating-point literal
-
Character Literals: Enclosed in single quotes, these represent single characters. For example, `'A'` is a character literal.
char ch = 'A'; // Assigning a character literal to a variable
-
String Literals: These are sequences of characters enclosed in double quotes, like `"Hello"`.
std::string greeting = "Hello"; // Assigning a string literal
-
Boolean Literals: These literals represent truth values, with `true` and `false` being the two possibilities.
bool isTrue = true; // Assigning a boolean literal
Identifiers
Identifiers are names used to identify a variable, function, array, class, or any other user-defined item. Understanding how to create valid identifiers is critical, as they serve as a means for referring to these components throughout your code.
To create an identifier, you must follow these rules:
- It must start with a letter (uppercase or lowercase) or an underscore (`_`).
- Subsequent characters may include letters, digits (0-9), and underscores.
- Identifiers are case-sensitive (e.g., `myVariable` and `MyVariable` are distinct).
Examples of valid identifiers:
int myVariable;
double _number42;
Examples of invalid identifiers include:
int 2ndVariable; // Cannot start with a digit
int my-variable; // Hyphens are not allowed
This Pointer
The `this` pointer is a special pointer that points to the object that is currently being accessed within a class. Understanding its usage is vital for employing class methods effectively.
The `this` pointer is particularly useful in several scenarios:
- It differentiates between class members and parameters with the same name. For example, if a constructor's parameter shares the same name as a class member, `this` can specify the member explicitly.
class Sample {
public:
int value;
Sample(int value) {
this->value = value; // Differentiating class member from parameter
}
};
- It provides a way to return the current object from a method, enabling method chaining in fluent interfaces.
class Builder {
public:
Builder* setValue(int value) {
this->value = value;
return this; // Returning the same object for chaining
}
};
Parentheses Expressions
Parentheses expressions facilitate grouping in C++. They can prioritize evaluation, which is vital in complex calculations.
Using parentheses affects the order of operations, which can lead to different results than if the parentheses were omitted. For example:
int result = (5 + 3) * 2; // Adds 5 and 3 before multiplying by 2
Without parentheses, the multiplication would occur first, leading to a different result:
int result1 = 5 + 3 * 2; // Evaluates to 5 + 6 = 11
Typeid Expressions
The `typeid` operator allows programmers to perform runtime type identification. It is especially useful when working with polymorphism in class hierarchies, enabling you to ascertain the exact type of an object at runtime.
For example, consider a base class and a derived class:
class Base {};
class Derived : public Base {};
int main() {
Base* obj = new Derived();
std::cout << typeid(*obj).name(); // Outputs the type of the object pointed to by obj
return 0;
}
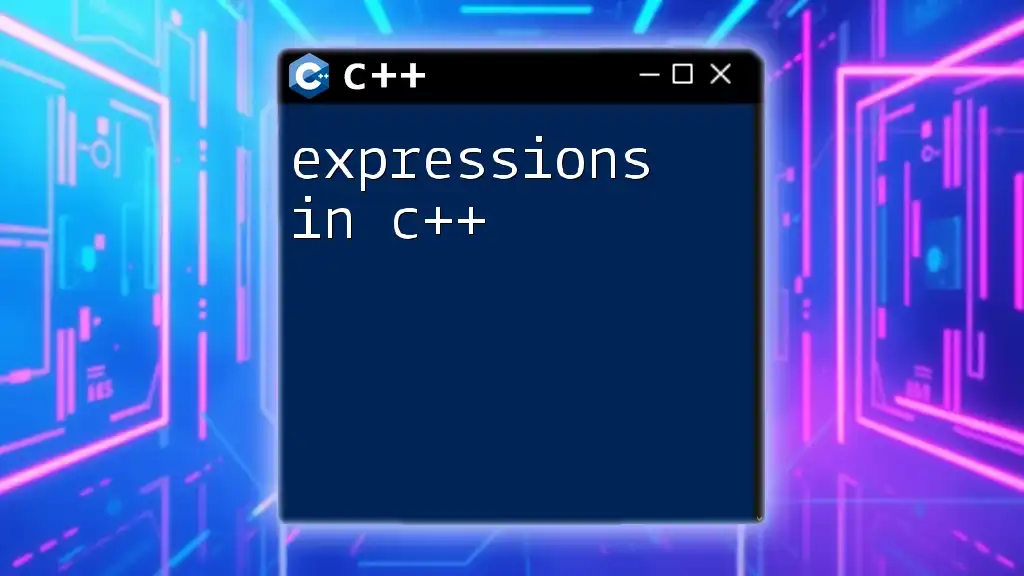
The Role of Primary Expressions in C++
Primary expressions in C++ are integral for constructing more intricate expressions. They serve as the operands in mathematical operations, condition checks, and function calls, allowing developers to build complex logic with clarity. For instance:
int x = 5;
int y = 10;
int z = x + y * 2; // Here, x and y are primary expressions used in a complex expression
In this example, `x` and `y` are primary expressions that participate in arithmetic calculations, demonstrating how foundational they are to the functionality of the program.
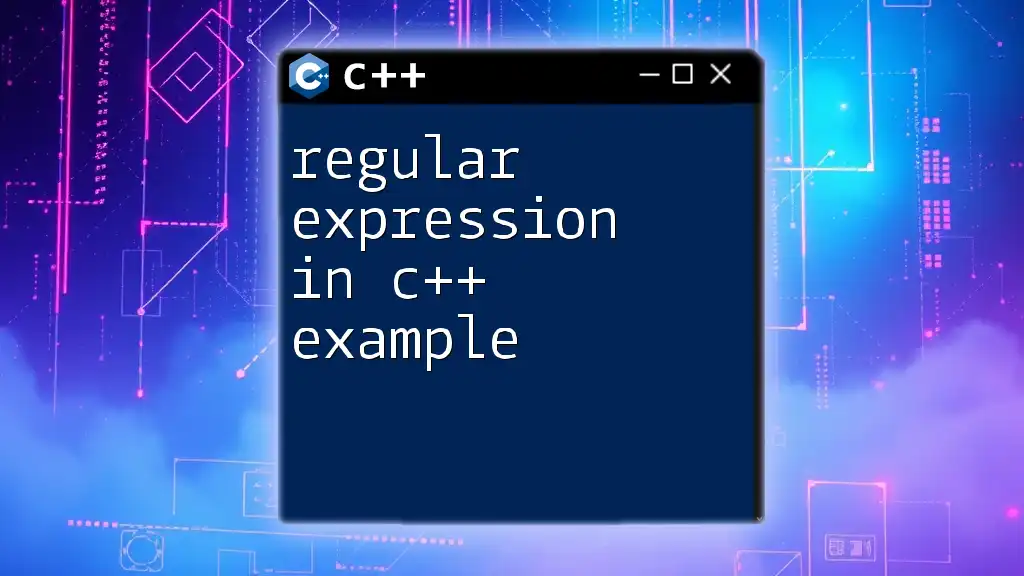
Common Mistakes with Primary Expressions
Misunderstanding Literals
One common error is misinterpreting the type of literals. For example, using an integer where a floating-point is expected can lead to type errors and bugs that are often hard to trace. Careful attention to types during development can alleviate many issues.
Identifier Rules Violations
Another frequent mistake is creating invalid identifiers, such as starting one with a digit or using unsupported characters like symbols. This can lead to compilation errors, halting the build process.
Improper Use of This Pointer
Misusing the `this` pointer, particularly in static methods (where `this` is not accessible), can yield undefined behavior. Understanding the scope and context of this keyword is essential to prevent such pitfalls.
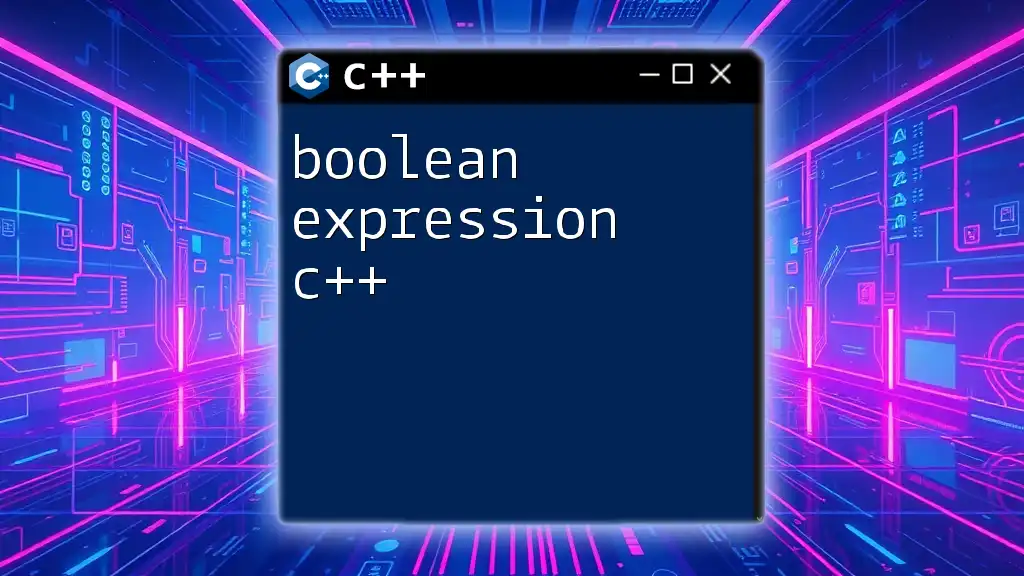
Tips for Mastering Primary Expressions
To truly master primary expressions:
- Practice creating and using different types of literals, identifiers, and pointers.
- Use comments liberally to document your code, ensuring that the purpose and function of each primary expression are clear.
- Engage in community discussions, forums, or study groups to expand your understanding and solve common issues collaboratively.
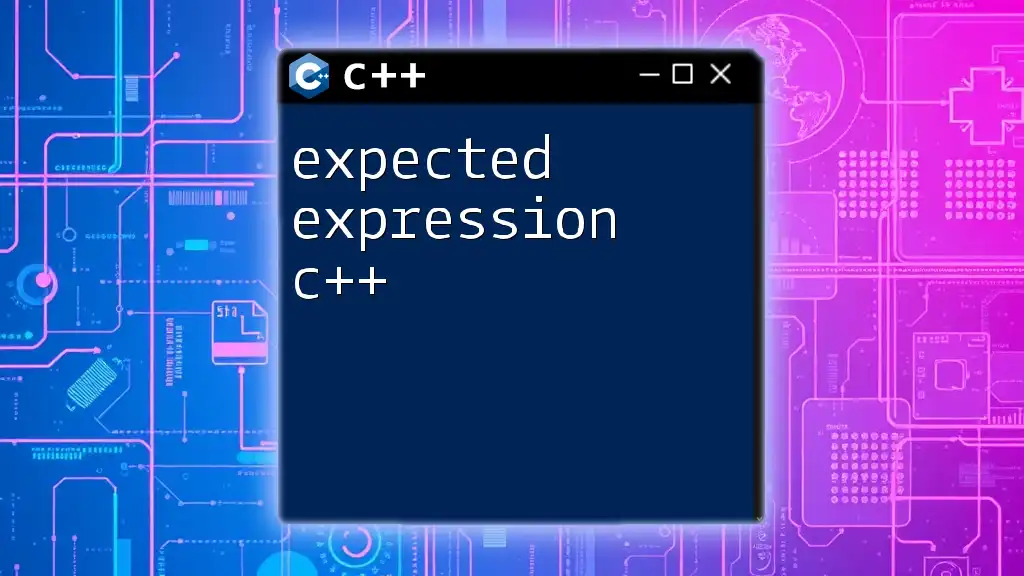
Conclusion
Understanding primary expressions in C++ is foundational to programming in this versatile language. They form the backbone of expressions, enabling operations, facilitating logic, and ensuring clear code organization. As you continue your C++ journey, delving deeper into these concepts will enhance your skill set and improve your development practice.