Binary operators in C++ are operators that work on two operands to perform operations such as arithmetic, comparison, and bit manipulation.
Here's a simple example using the arithmetic binary operator:
int a = 5;
int b = 10;
int sum = a + b; // sum will hold the value 15
Understanding Binary Operators
What are Binary Operators?
Binary operators are fundamental components in C++ that operate on two operands. Unlike unary operators, which act on a single operand, binary operators take two values and return a single value based on the operation performed. The term "binary" denotes this duality, making it essential to understand their role in various calculations and comparisons in your programs.
Common Uses of Binary Operators
Binary operators serve several purposes in programming, including:
- Performing mathematical calculations
- Comparing values
- Manipulating individual bits in data types
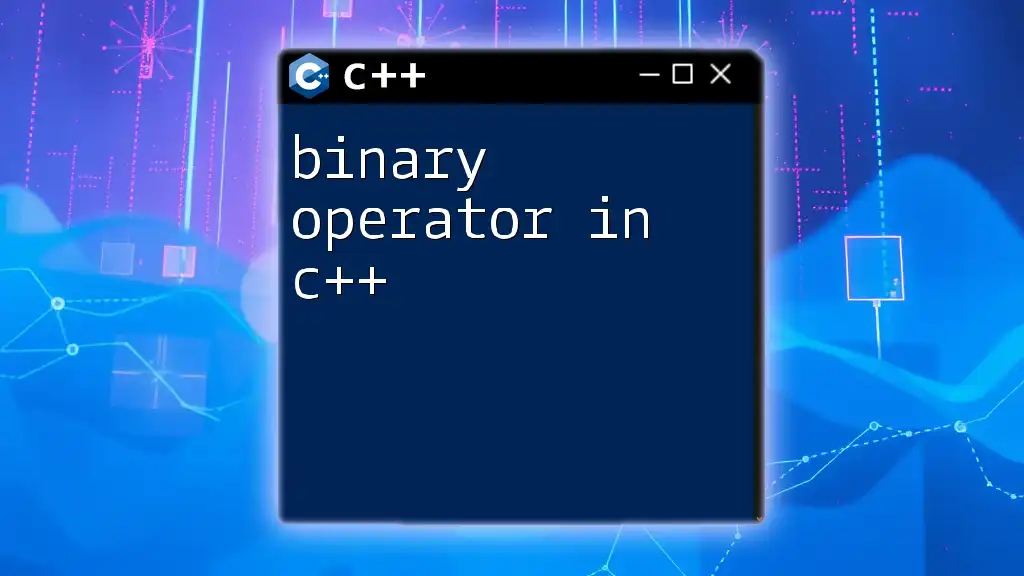
Types of Binary Operators in C++
Arithmetic Operators
Arithmetic operators are used for basic mathematical operations. These operations include addition, subtraction, multiplication, division, and modulus.
Addition and Subtraction
In C++, the `+` operator performs addition, while the `-` operator performs subtraction.
Example:
int a = 10, b = 5;
int sum = a + b; // 15
int difference = a - b; // 5
In the above example, we see how two integer variables are added and subtracted, yielding a straightforward output.
Multiplication and Division
For multiplication, C++ uses the `*` operator, while the `/` operator is used for division.
Example:
int product = a * b; // 50
int quotient = a / b; // 2
As illustrated, multiplying `a` and `b` results in `50`, while dividing `a` by `b` gives a quotient of `2`.
Modulus Operator
The modulus operator `%` determines the remainder of a division operation.
Example:
int remainder = a % b; // 0
Here, `10 % 5` results in `0` because `10` is perfectly divisible by `5`. The modulus operator is particularly useful for tasks that involve checking evenness, oddness, or cycling through a range.
Relational Operators
Relational operators are vital for comparing two values. They return a Boolean result—either `true` or `false`.
Comparison Operators
C++ provides several relational operators, including `==`, `!=`, `<`, `>`, `<=`, and `>=`, which allow you to evaluate relationships between operands.
Example:
bool isEqual = (a == b); // false
bool isLess = (a < b); // false
The comparisons reveal that `10` is not equal to `5`, and `10` is not less than `5`.
Logical Operators
Logical operators take Boolean values as operands and return a Boolean result. These include the logical AND (`&&`), logical OR (`||`), and logical NOT (`!`).
AND, OR, NOT
Logical operators are often used in conditional statements, allowing for complex decision-making processes in your code.
Example:
bool result = (a > b && a < 20); // true
In this scenario, both conditions (`a` is greater than `b` AND `a` is less than `20`) evaluate to `true`, making `result` true.
Bitwise Operators
Bitwise operators manipulate individual bits of integer values. They're essential for low-level programming operations, such as hardware interfacing or graphics programming.
Overview of Bitwise Operations
C++ supports various bitwise operators, including `&`, `|`, `^`, `~`, `<<`, and `>>`.
Example:
int bitwiseAnd = a & b; // 0
int bitwiseOr = a | b; // 15
int bitwiseNot = ~a; // -11
The bitwise AND operation results in `0`, as the corresponding bits of `10` and `5` do not match in any position. The bitwise OR operation, however, gives `15` since it combines bits, while the NOT operator inverts all bits in `10`.
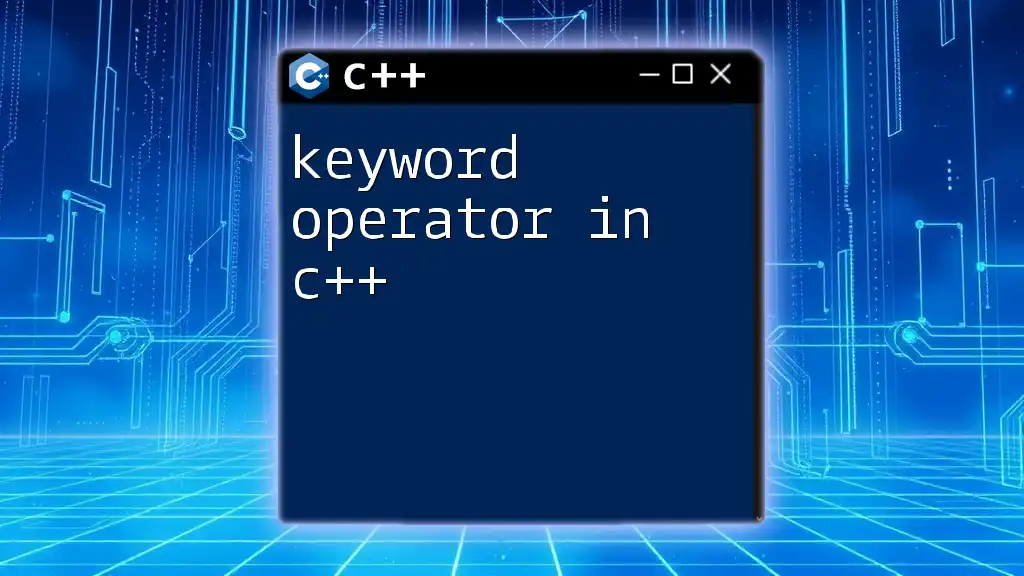
Operator Precedence and Associativity
Understanding Precedence
Operator precedence determines the order in which operations are performed in an expression. Operators with higher precedence are evaluated before those with lower precedence.
Example:
int result = a + b * 10; // 60 (multiplication has higher precedence)
In this example, multiplication happens before addition due to operator precedence, leading to a result of `60`.
Associativity of Operators
Associativity refers to the direction in which operators of the same precedence level are evaluated. C++ generally evaluates most binary operators from left to right, while certain operators like assignment (=) evaluate from right to left.
Example:
int result1 = a - b + a; // 15
int result2 = a - (b + a); // 0
The first expression adds and subtracts in sequence, while the second expression alters the outcome by changing the grouping of operations.
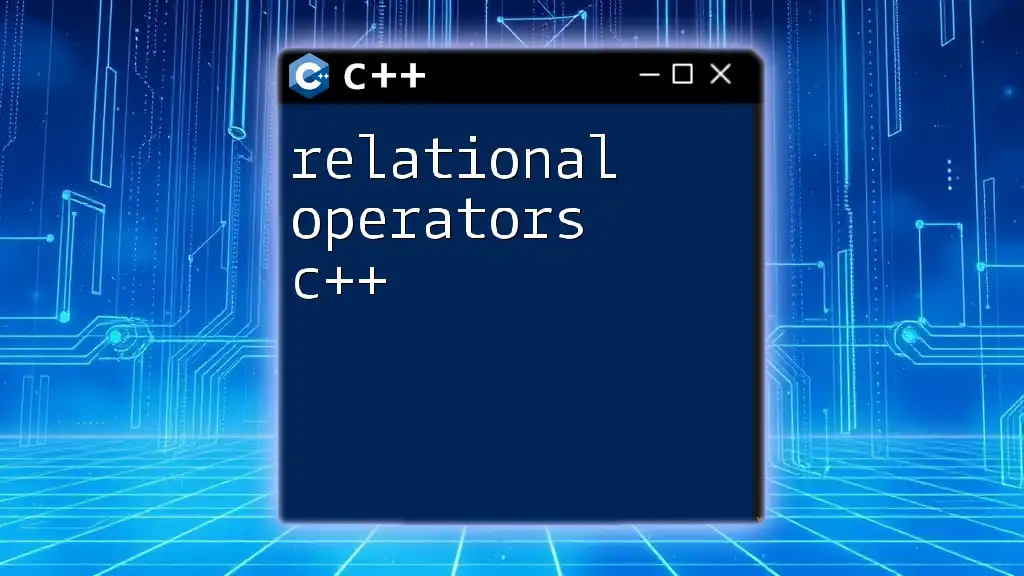
Best Practices When Using Binary Operators
Readability and Maintainability
When working with binary operators, it's crucial to maintain code readability. Ensure your expressions are not overly complex, making it easier for you and others to understand the logic at a glance. Using parentheses wisely can also help clarify priority among operations.
Avoiding Common Mistakes
Common pitfalls when using binary operators include neglecting operator precedence and failing to use parentheses, both of which can lead to unexpected results.
Example:
int mistake = a - b + a * 2; // Confusing order
int fix = a - (b + a * 2); // Clearer intention with parentheses
In the first example, the order of operations could lead to bugs, while the second expression clearly outlines intent, leading to a reliable outcome.
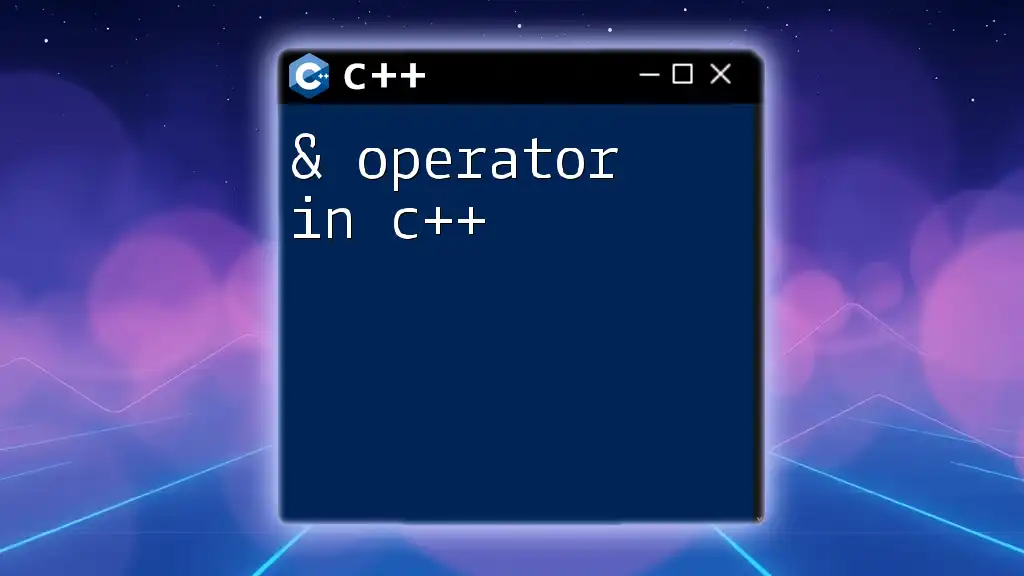
Conclusion
Understanding binary operators in C++ is fundamental for any programmer. They serve various purposes, from basic arithmetic calculations to complex logical evaluations and bit manipulations. By employing best practices for readability and avoiding common mistakes, you'll harness the full power of binary operators effectively. Now, take the time to practice these concepts in your projects and see your proficiency flourish in C++.