The `&` operator in C++ is used to obtain the address of a variable (address-of operator) or to declare a reference type, which allows an alias for an existing variable.
Here’s a code snippet demonstrating both uses of the `&` operator:
#include <iostream>
int main() {
int x = 10;
int* ptr = &x; // Using & to get the address of x
int& ref = x; // Using & to declare a reference to x
std::cout << "Value of x: " << x << std::endl;
std::cout << "Address of x: " << ptr << std::endl;
std::cout << "Value via reference: " << ref << std::endl;
return 0;
}
Understanding the & Operator in C++
What is the & Operator?
The & operator in C++ serves multiple roles, primarily acting as the address-of operator and the bitwise AND operator. These functionalities make it an indispensable tool in any C++ programmer's toolkit.
Address-of Operator: When placed before a variable, it retrieves the memory address of that variable. This is crucial for working with pointers and memory management.
Bitwise AND Operator: When applied between two integral types, it performs a bitwise AND operation on their binary representations, returning a new integral type reflecting the result.
Importance of the & Operator in C++
The & operator holds significant value in C++, particularly in memory management and low-level programming. Its ability to manipulate memory addresses allows developers to create efficient applications. Understanding this operator is vital for comprehending concepts like pointers and references, which further enhance a programmer's scalability in building sophisticated systems.
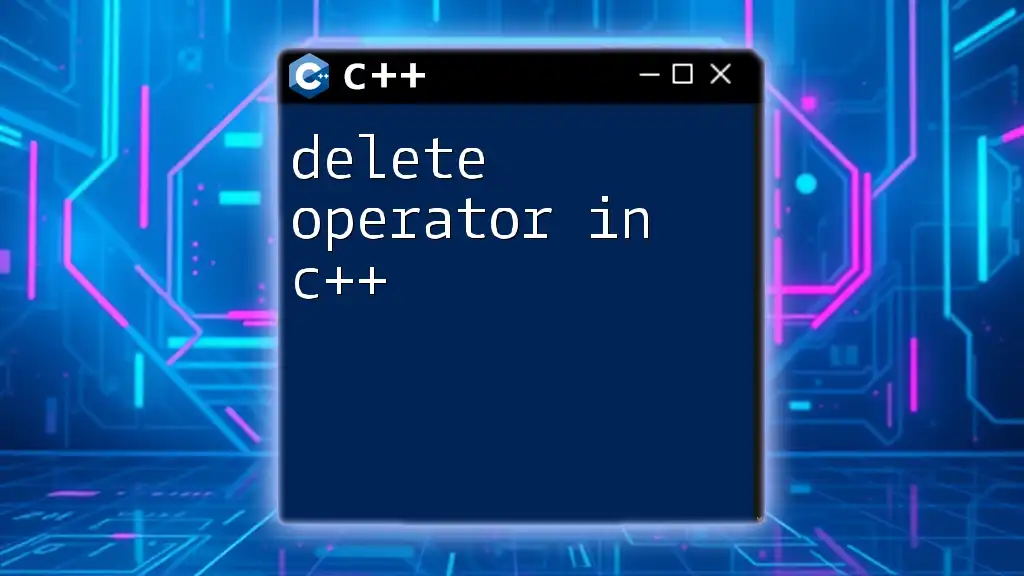
The Address-of Operator
Definition and Usage
The address-of operator allows you to obtain the memory address of a variable seamlessly. This is essential for pointer manipulation.
Example: To get the address of an integer variable, you can do the following:
int num = 42;
int *ptr = # // ptr now holds the address of num
Here, `ptr` will point to the memory location where `num` is stored in memory.
Practical Applications
The address-of operator is particularly useful in functions where you want to pass the address rather than the actual value. This is vital for memory management.
Example: Consider the following function designed to display the address of an integer:
void displayAddress(int *ptr) {
std::cout << "Address: " << ptr << std::endl;
}
When calling this function with `ptr`, you effectively let it display the address stored in `ptr`, showcasing one of the many applications of the address-of operator.
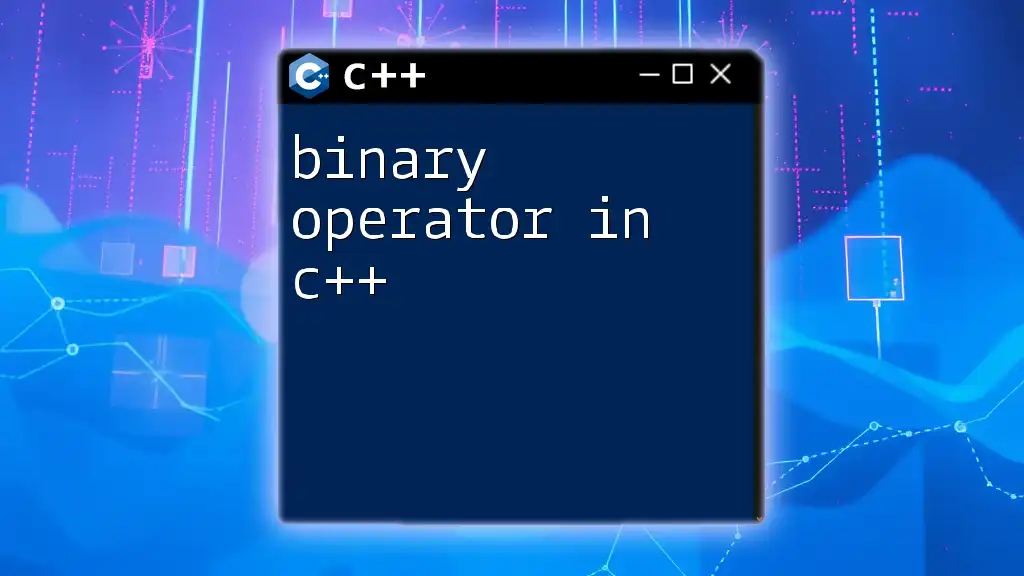
The Bitwise AND Operator
Definition and Usage
The bitwise AND operator works on the bits of its operands. Each corresponding bit in the two numbers is compared; if both bits are 1, the resulting bit is set to 1; otherwise, it is set to 0.
Example: A simplistic calculation using the bitwise AND operator is as follows:
int a = 5; // 0101 in binary
int b = 3; // 0011 in binary
int result = a & b; // result is 1 (0001 in binary)
The result here demonstrates the fundamental operation of the bitwise AND, yielding a new integer based on the combined bit patterns.
Bitwise Operations Explained
Delving deeper into bitwise operations, we can observe how the bitwise AND operator contrasts with others like OR (|) and NOT (~). Each operates based on differing logic, allowing developers to manipulate binary data efficiently.
For instance, consider this comparison between bitwise operations:
- AND (`a & b`): Both bits must be 1 to yield 1.
- OR (`a | b`): At least one bit must be 1 to result in 1.
- NOT (`~a`): Flips all bits in the number.
Effective use of these operators can lead to optimized performance in programs that require direct manipulation of binary data.
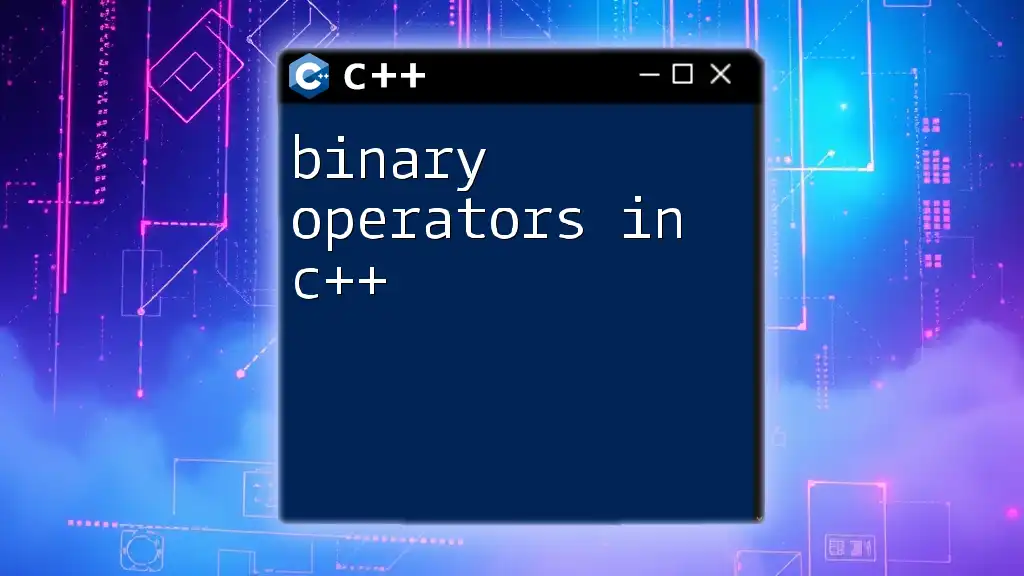
The & Operator in Function Parameters
Pass by Reference
The & operator is crucial when we want to manipulate the original argument passed into a function without creating a copy. This is achieved through pass by reference.
Example: A straightforward function that increments an integer can be illustrated as follows:
void increment(int &value) {
value++;
}
In this case, calling `increment(num)` will directly affect `num`, increasing its value without creating an extra copy.
Benefits of Pass by Reference
Using pass by reference offers several advantages:
- Performance Improvement: No extra copies of variables are created, which is especially beneficial for large data structures.
- Direct Modification: The ability to change the actual variable within the function allows for tidy and efficient coding practices, reducing potential errors.
Understanding and implementing the & operator for pass by reference can significantly enhance program design.
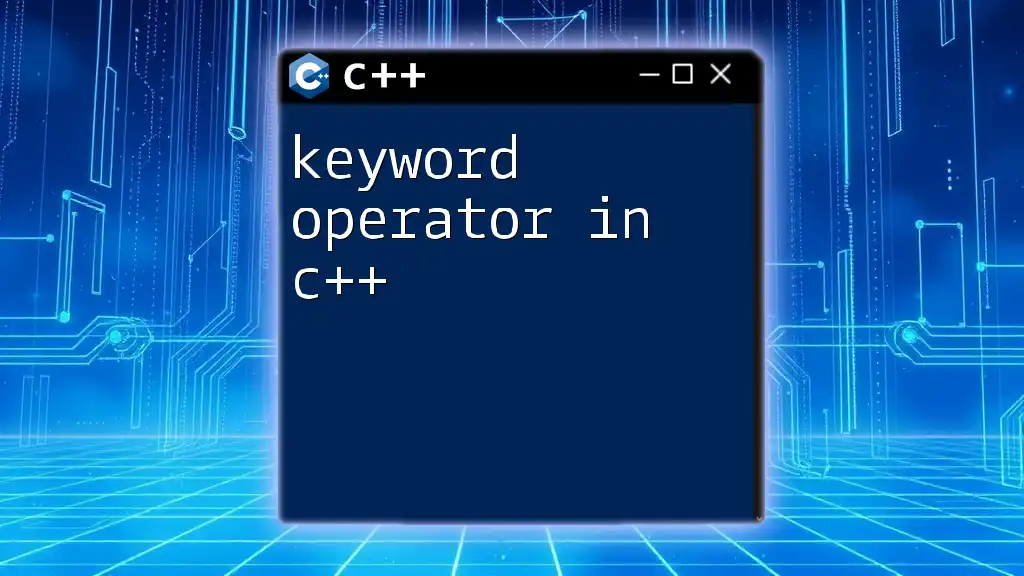
Common Mistakes with the & Operator
Misunderstandings in Usage
Many newcomers to C++ often confuse the & operator with the * operator. While the address-of operator retrieves a variable's address, the * operator dereferences a pointer to access the value at that address.
Example: Here's a common mistake:
int num = 10;
int *ptr = #
std::cout << *ptr; // Correct: Outputs 10
std::cout << &ptr; // Potential Misunderstanding: Outputs the address of ptr
Debugging Tips
Debugging issues with the & operator can be eased by understanding its implications and using compiler warnings effectively. Tools like static analysis or memory checkers can also help identify unexpected behaviors linked to the misuse of this operator.
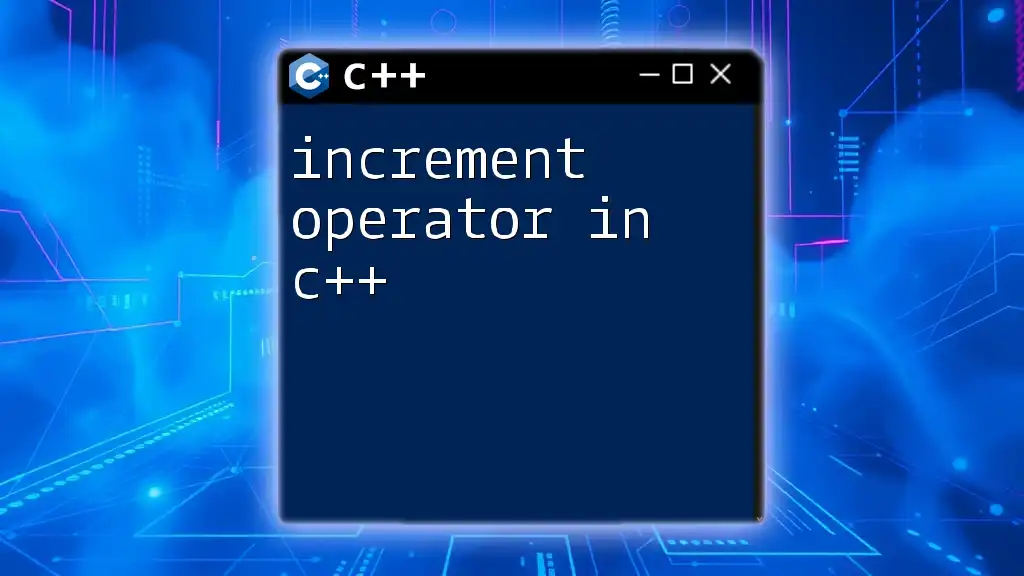
Advanced Topics
References vs. Pointers
A crucial distinction in C++ programming is between references and pointers, both of which utilize the & operator.
- References: Must be initialized and cannot be changed to refer to another object.
- Pointers: Can be reassigned and have more flexible memory allocation capabilities.
Example:
int a = 10;
int &ref = a; // ref is a reference to a
int *ptr = &a; // ptr points to the address of a
Here, `ref` remains attached to `a`, while `ptr` can point to different addresses as needed.
Overloading Operators
In C++, we can even overload the & operator for user-defined types, enhancing object interaction. This allows for more intuitive handling of custom classes.
Example: Creating a simple custom class that overloads the & operator might look like this:
class MyClass {
public:
MyClass(int val) : value(val) {}
MyClass& operator&() { return *this; }
private:
int value;
};
This implementation allows you to define what happens when the & operator is used with instances of `MyClass`, showcasing the flexibility and power of operator overloading in C++.
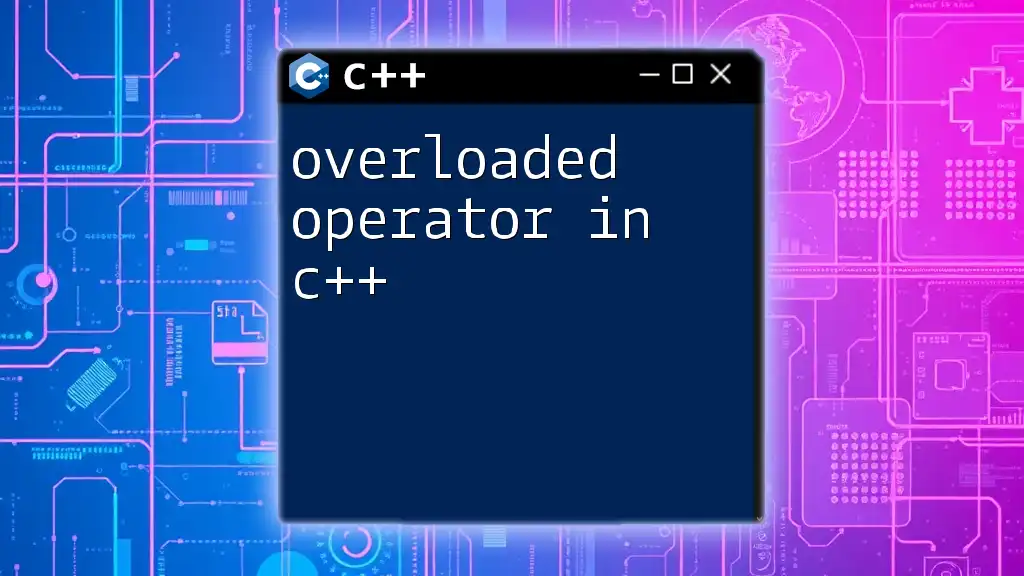
Conclusion
In summary, the & operator in C++ serves multiple essential roles: facilitating memory management through the address-of operator and manipulating binary data via the bitwise AND operator. Mastering its functionality can greatly enhance your programming skills, particularly in managing data efficiently and optimizing memory usage.
Keep exploring the nuances of the & operator and practice its applications in your projects to become fluent in the capabilities C++ offers. For deeper learning, consider following additional resources or community discussions to refine your understanding further.