In C++, operator overloading allows you to define custom behavior for operators (like +, -, *, etc.) when they are applied to user-defined types (classes or structs).
Here's a simple example of overloading the '+' operator for a `Point` class:
#include <iostream>
class Point {
public:
int x, y;
Point(int x, int y) : x(x), y(y) {}
// Overloading the '+' operator
Point operator+(const Point& other) {
return Point(x + other.x, y + other.y);
}
};
int main() {
Point p1(1, 2);
Point p2(3, 4);
Point p3 = p1 + p2; // Using the overloaded operator
std::cout << "p3: (" << p3.x << ", " << p3.y << ")" << std::endl; // Output: p3: (4, 6)
return 0;
}
Understanding Operator Overloading in C++
Operator Overloading is a powerful feature in C++ that allows you to define custom behavior for operators (like +, -, *, etc.) when they are applied to user-defined types (like classes). This means you can make your own classes behave like basic types, enhancing the expressiveness and usability of your code.
Importance of Operator Overloading
When you overload operators, you can write code that is not only more intuitive but also more readable, making it easier for others (and your future self) to understand. For example, instead of calling an add function to add two `Vector` objects, you can simply use `vector1 + vector2`, which is clearer and more in line with how we think mathematically.
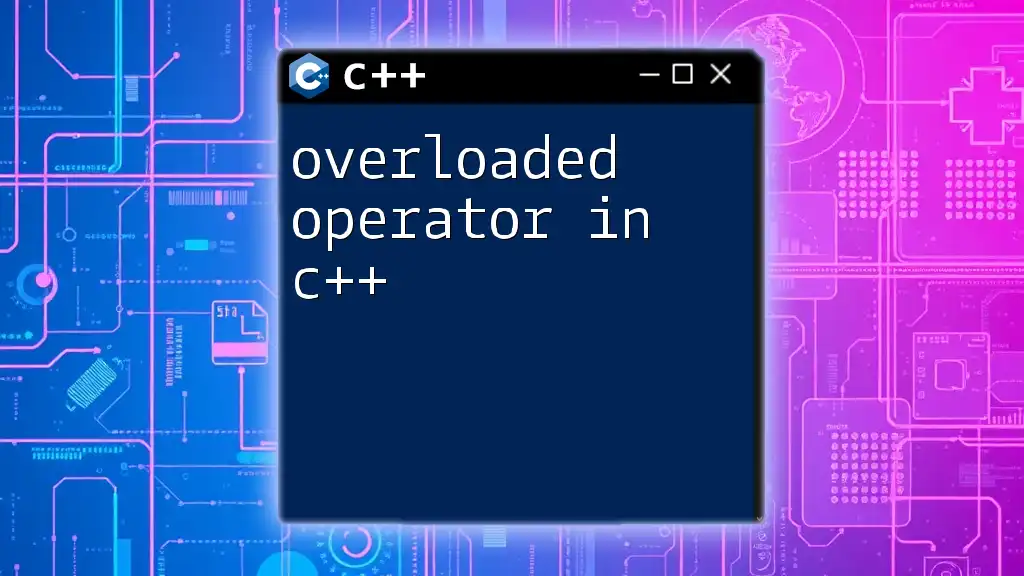
Key Concepts of Operator Overloading
What is an Operator in C++?
Operators in C++ are special symbols that perform operations on one or more operands. They are category-wise classified as follows:
- Arithmetic Operators: +, -, *, /
- Relational Operators: ==, !=, <, >
- Logical Operators: &&, ||, !
For example, using the arithmetic operator `+` with integers performs addition:
int a = 5;
int b = 3;
int sum = a + b; // sum is 8
Syntax of Operator Overloading
The syntax for overloading an operator generally consists of a function that has the keyword `operator` followed by the symbol of the operator being overloaded. This function can be a member of a class or a non-member function.
Here’s a basic example of overloading the `+` operator as a member function:
class Box {
public:
int length;
Box(int l) : length(l) {}
Box operator+(const Box& b) {
return Box(length + b.length);
}
};
In this example, the `+` operator is overloaded to add two `Box` objects by adding their lengths.
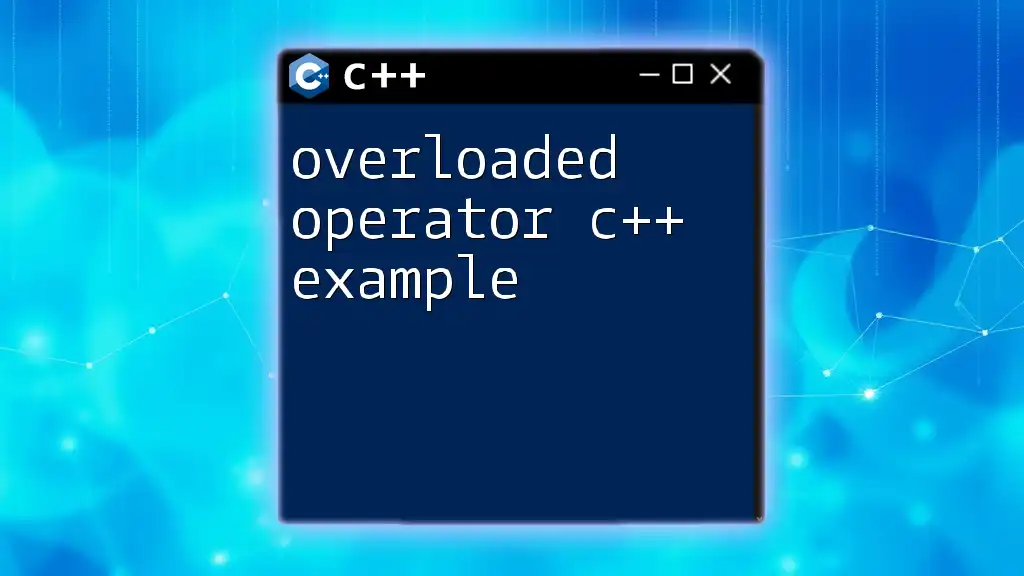
Common Operators to Overload
Arithmetic Operators
Overloading arithmetic operators allows custom data types to support mathematical operations. For instance, imagine you are working with a `Vector` class.
class Vector {
public:
float x, y;
Vector(float x, float y) : x(x), y(y) {}
Vector operator+(const Vector& v) {
return Vector(x + v.x, y + v.y);
}
};
Here, `Vector` objects can now be added together using the `+` operator.
Comparison Operators
Comparison operators help define how objects are compared with one another. For example, to overload the `==` operator:
class Point {
public:
int x, y;
Point(int x, int y) : x(x), y(y) {}
bool operator==(const Point& p) {
return (x == p.x && y == p.y);
}
};
This allows you to compare `Point` objects directly, making your code cleaner and easier to read.
Other Operators
Assignment Operator
The assignment operator (`=`) can also be overloaded to manage copying of complex objects effectively:
class DynamicArray {
private:
int* arr;
int size;
public:
DynamicArray(int s) : size(s) {
arr = new int[size];
}
DynamicArray& operator=(const DynamicArray& other) {
if (this == &other)
return *this; // Self-assignment check
delete[] arr; // Free existing resource
size = other.size;
arr = new int[size];
std::copy(other.arr, other.arr + size, arr); // Copy elements
return *this;
}
~DynamicArray() {
delete[] arr; // Cleanup
}
};
This code snippet manages memory properly during assignment.
Stream Operators
The stream operators (`<<` and `>>`) can be overloaded to allow input and output of your custom classes:
#include <iostream>
class Person {
public:
std::string name;
int age;
friend std::ostream& operator<<(std::ostream& os, const Person& p) {
os << "Name: " << p.name << ", Age: " << p.age;
return os;
}
friend std::istream& operator>>(std::istream& is, Person& p) {
is >> p.name >> p.age;
return is;
}
};
Using `std::cout << person` will output more meaningful information about the `Person`.
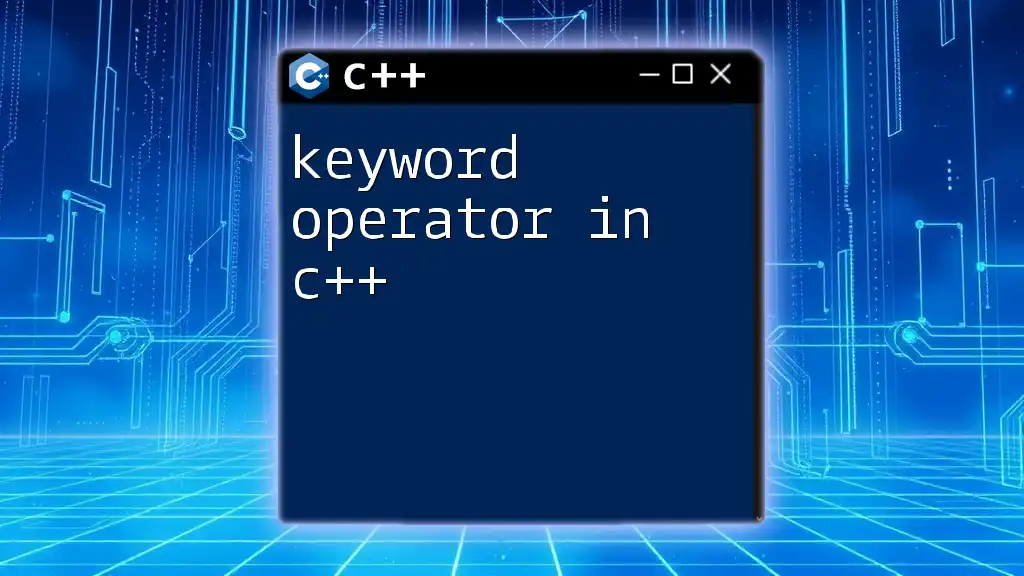
How to Overload Operators in C++
Member Function vs. Non-Member Function
Whether to use a member function or a non-member function largely depends on the context of the operator being overloaded.
- Member Function: Overloading operators like `+` or `-` as a member function works well when the left operand is guaranteed to be your class type. However, for cases where the left operand may not be your class, it is better to use non-member functions.
Example:
Vector operator+(const Vector& v1, const Vector& v2) {
return Vector(v1.x + v2.x, v1.y + v2.y);
}
Using Friend Functions
Using friend functions is beneficial when you need access to private data members of your class without either changing the class structure or making encapsulation less effective.
class Fraction {
private:
int numerator;
int denominator;
public:
Fraction(int n, int d) : numerator(n), denominator(d) {}
friend Fraction operator+(const Fraction& f1, const Fraction& f2) {
return Fraction(f1.numerator * f2.denominator + f2.numerator * f1.denominator,
f1.denominator * f2.denominator);
}
};
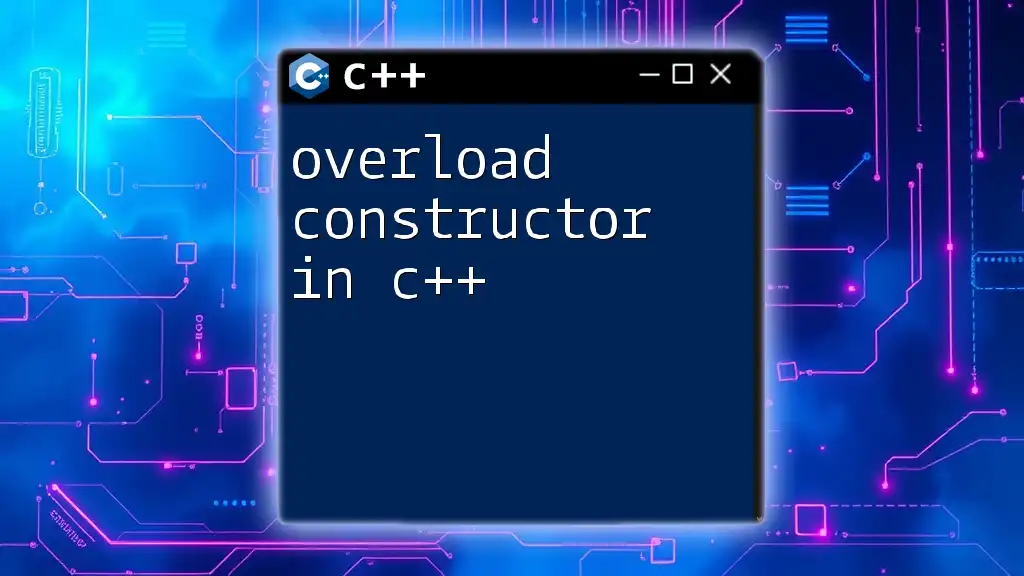
Operator Overloading Rules and Best Practices
Rules of Overloading Operators
While operator overloading provides flexibility, there are key rules to adhere to:
- You cannot create new operators; you can only overload existing ones.
- The precedence and associativity of operators cannot be changed.
- You must not alter the fundamental meaning of an operator.
Best Practices for Overloading Operators
When overloading operators, aim for readable and intuitive code. Think about the expected behavior and ensure it aligns with user's intuitive understanding of the operators.
For example, if you overload the `==` operator, it should make logical sense when compared with built-in data types. If your overloaded operator deviates from expected behavior, it may lead to code that is difficult to maintain.
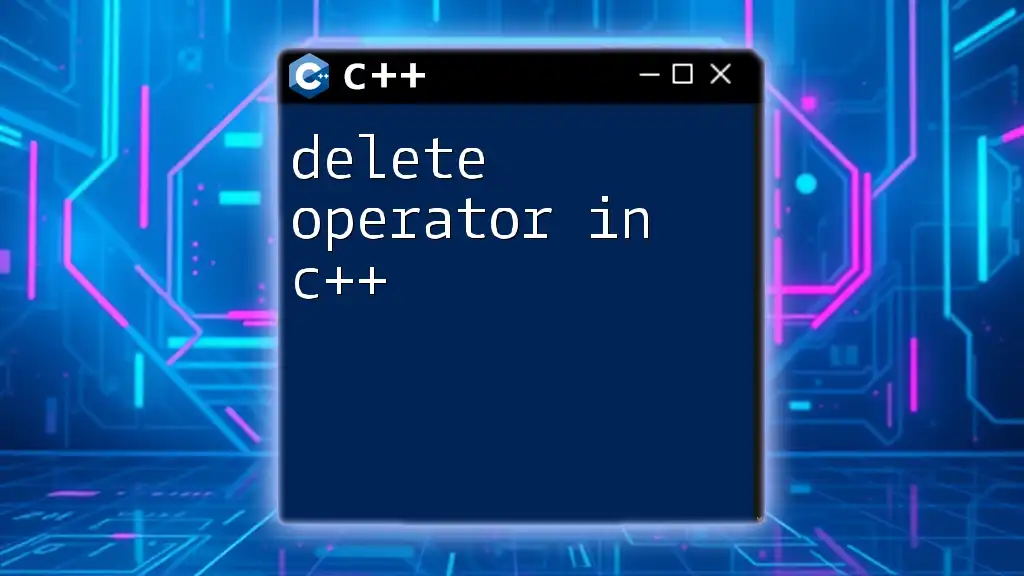
Real-world Applications of Operator Overloading
Operator overloading is incredibly useful in numerous cases. For example, if you are developing a math library, you can define elegant interfaces for addition, subtraction, or complex number manipulation.
Custom data structures that represent graphs or trees may benefit significantly from overloaded operators that simplify the manipulation logic. For instance, if you overload the `<<` operator for a `Graph` class, you can easily print the entire structure with a single command, enhancing both debugging and usability.
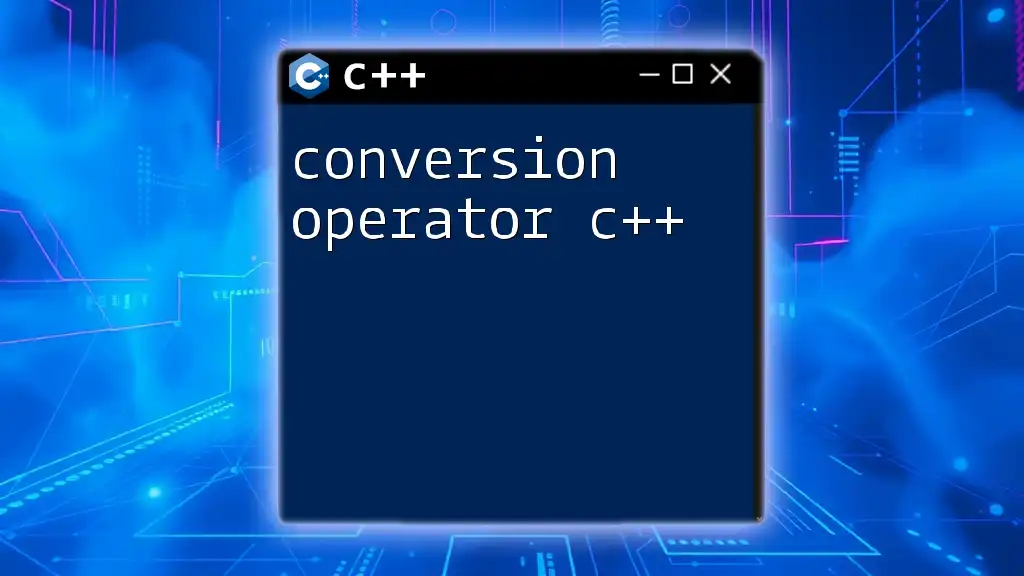
Conclusion
To overload the operator in C++ is to empower your custom types with the same intuitive syntax as built-in types. This allows your code to be more readable and align closely with how the data is conceptualized. As you continue to explore C++, practice overloading through projects to deepen your understanding and see firsthand the flexibility it brings.
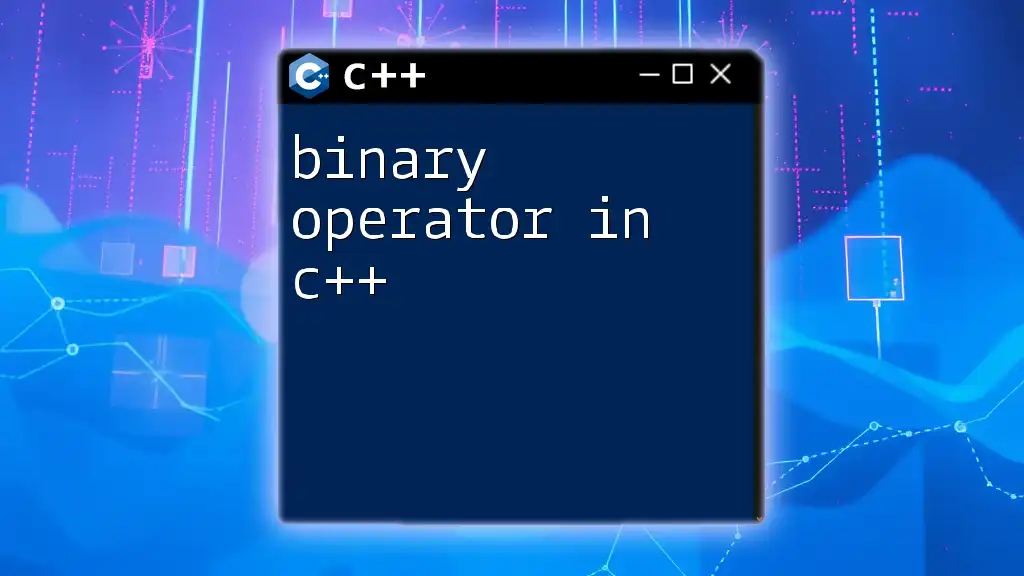
Additional Resources
For further exploration, consider delving into C++ documentation, online tutorials, or courses that cover operator overloading as well as broader concepts in C++. This will help solidify your understanding and give you practical applications to work with.
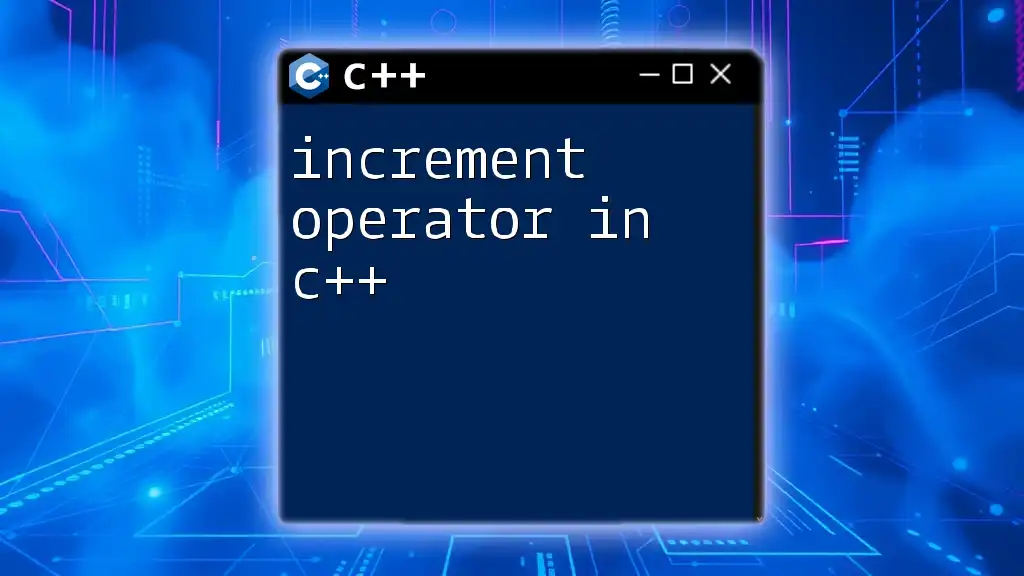
FAQs about Operator Overloading in C++
Can all operators be overloaded?
No, certain operators like `.` (member access), `.*` (pointer to member), and `::` (scope resolution) cannot be overloaded.
What are the limitations of operator overloading?
The primary limitation is that operator behavior should maintain its expected semantics and not introduce unexpected behaviors, which might confuse the users of your class.