The increment operator in C++ (`++`) increases the value of a variable by one and can be used as either a prefix (before the variable) or a postfix (after the variable).
Here's a code snippet demonstrating both forms of the increment operator:
#include <iostream>
int main() {
int a = 5;
int b = ++a; // Prefix increment
int c = a++; // Postfix increment
std::cout << "a: " << a << ", b: " << b << ", c: " << c << std::endl; // Outputs: a: 7, b: 6, c: 6
return 0;
}
Understanding the Increment Operator
Definition of Increment Operator
The increment operator in C++ is a unary operator that increases the value of its operand by one. It can be represented in two forms:
- Pre-increment: This is written as `++variable`, where the variable is increased by one before its current value is used in any expression.
- Post-increment: This is expressed as `variable++`, where the current value of the variable is used in an expression before it is incremented.
Understanding the difference between these two forms is crucial for writing efficient and bug-free code.
How the Increment Operator Works
Pre-Increment vs Post-Increment
In the case of pre-increment, the increment occurs first, and then the updated value can be used.
Example: Pre-Increment
int x = 5;
int y = ++x; // y is 6, x is 6
Here, `x` is incremented to 6, and that value is assigned to `y`.
On the other hand, with post-increment, the current value of the variable is returned first before the variable is incremented.
Example: Post-Increment
int x = 5;
int y = x++; // y is 5, x is 6
In this case, `y` gets the original value of `x`, which is 5, while `x` becomes 6 afterward.
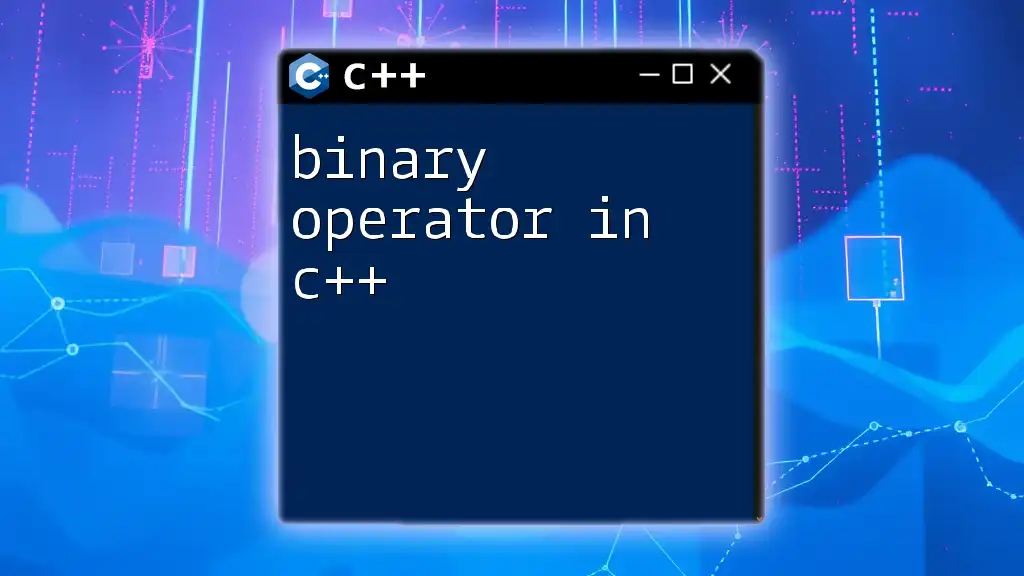
Practical Use Cases of the Increment Operator
Basic Use in Loops
One of the most common applications of the increment operator is within loops, particularly `for` loops. The increment operator serves to control loop iteration effectively.
Example: Using Increment in a Loop
for (int i = 0; i < 5; i++) {
cout << i << endl;
}
In this loop, `i` starts at 0 and increments by 1 until it reaches 5, effectively printing the numbers 0 to 4.
Increment in Conditionals
The increment operator can also be utilized within conditional statements, allowing dynamic manipulations of variables based on conditions.
Example:
int count = 0;
if (++count == 1) {
cout << "Count is now 1" << endl;
}
In this example, `count` is incremented first, and then compared against 1. Thus, this code outputs the message since `count` becomes 1.
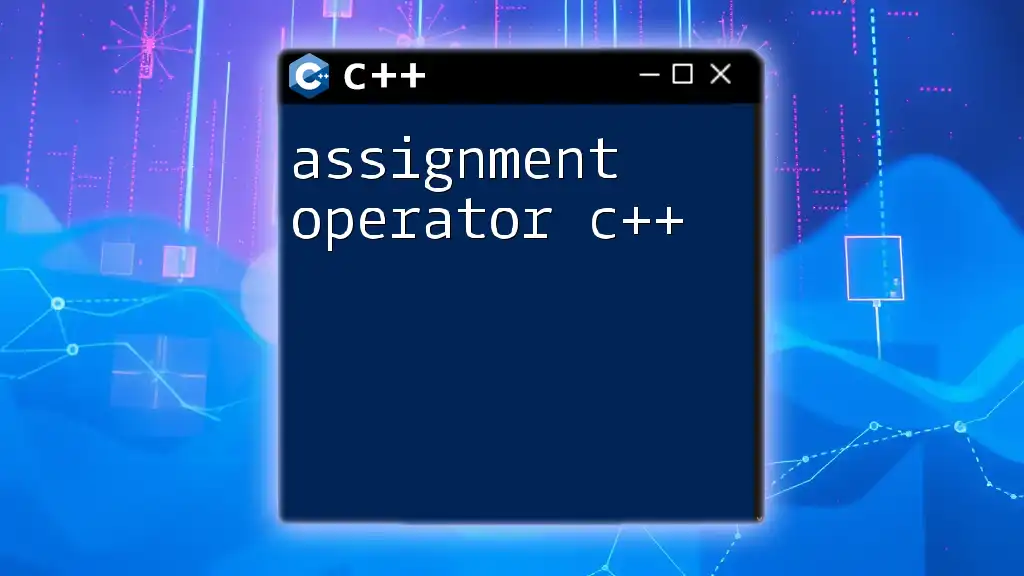
Increment and Decrement in C++
Overview of Decrement Operator
Just as crucial is the decrement operator, which is represented similarly but decreases the value by one. The syntax is `--variable` for pre-decrement and `variable--` for post-decrement.
Combining Increment and Decrement
Both operators can be used in tandem to achieve more complex functionalities in your program.
Example: Mixing Increment and Decrement
int a = 5;
int b = --a; // b is 4, a is 4
int c = a--; // c is 4, a is 3
In this example, `a` is decremented first for `b`, then the original value of `a` is stored in `c` before the variable is decremented again.
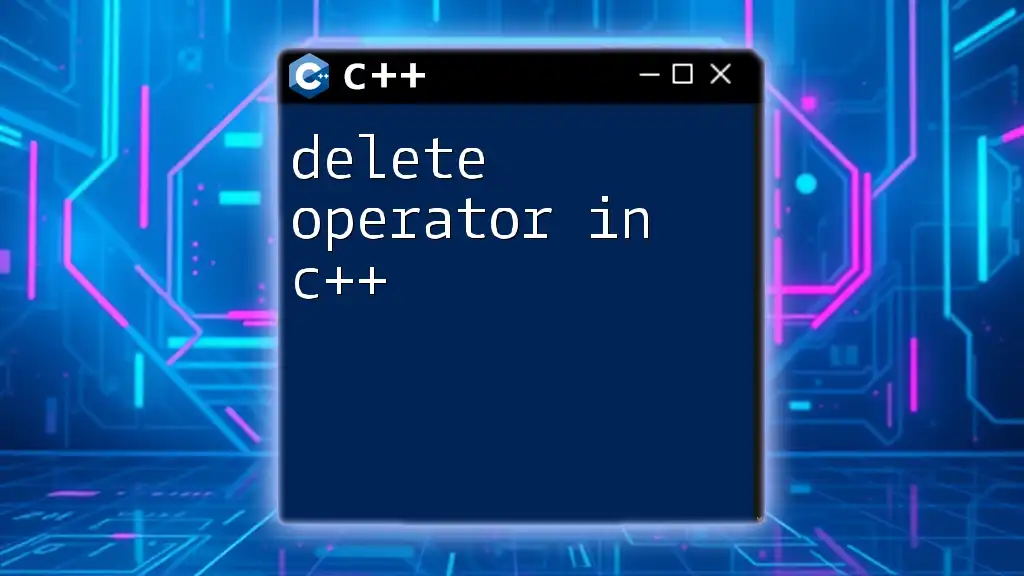
Common Errors and Pitfalls
Confusion Between Pre and Post Increment
A frequent issue among new C++ programmers is the confusion between pre-increment and post-increment, which can lead to unexpected results.
Example:
int a = 1;
int b = a++; // b is 1 not 2
In this case, `b` gets the value of `a` before the increment, which may not have been the intention.
Not Understanding Operator Precedence
C++ has a defined operator precedence, and misunderstanding this can lead to bugs. When mixed with other operations, it’s essential to use parentheses to ensure operations are performed in the desired order.
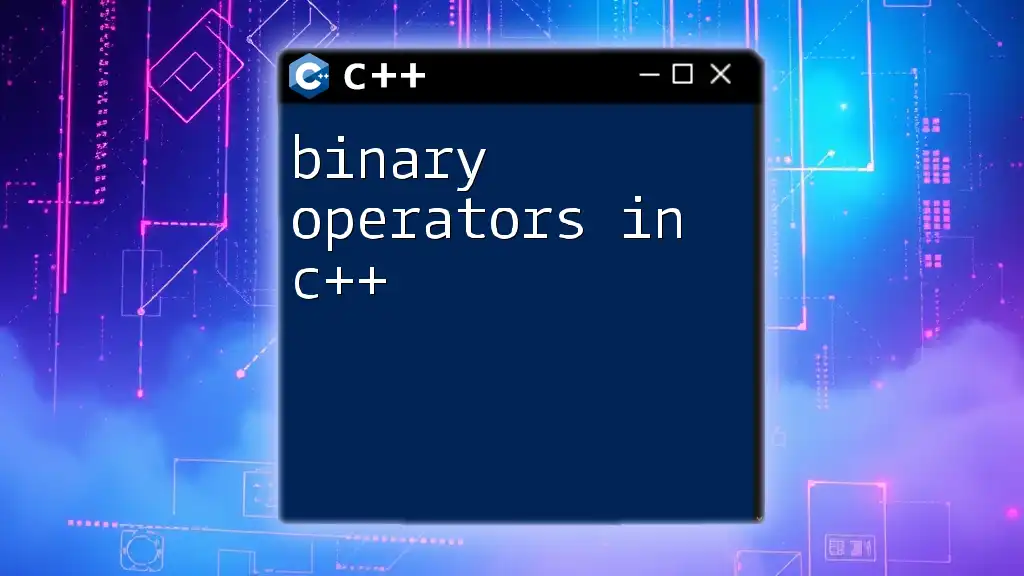
Performance Considerations
Efficiency of Increment Operators
Using the increment operator can often lead to more efficient code. This is especially true in loops or functions where the operations are minimized.
Example:
for (int i = 0; i < 1000000; ++i) { /* Do something */ }
In this scenario, using the pre-increment `++i` can technically result in more efficient code performance over an increasingly large dataset, although the difference may be negligible in smaller cases.
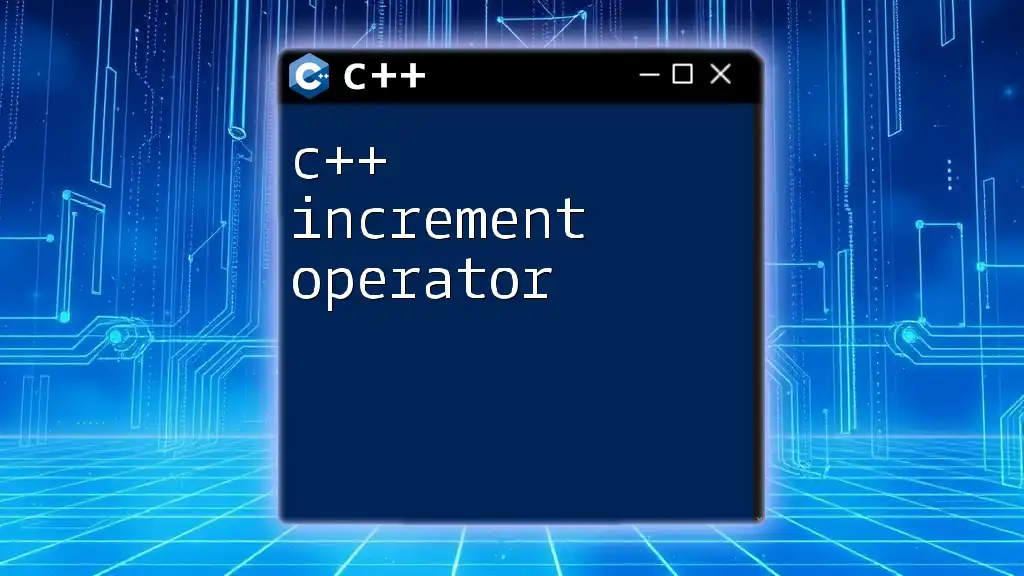
Conclusion
In conclusion, the increment operator in C++ serves as an essential tool for manipulating values in various programming scenarios. Mastering both the increment and decrement operations can significantly enhance your coding fluency and precision.
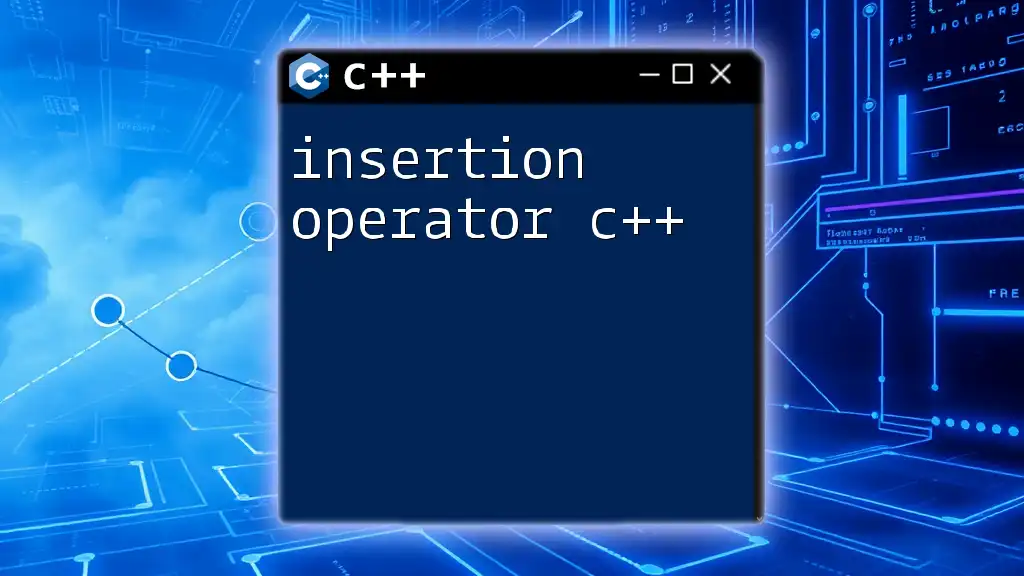
Additional Resources
For further reading, consider checking out official C++ documentation and programming tutorials that delve into practical applications and provide interactive coding environments for hands-on learning. Recommended tools include online compilers and integrated development environments (IDEs) that facilitate learning through experimentation.