The C++ increment operator `++` increases a variable's value by one and can be used in both prefix and postfix forms.
#include <iostream>
int main() {
int a = 5;
std::cout << "Prefix increment: " << ++a << std::endl; // Outputs 6
int b = 5;
std::cout << "Postfix increment: " << b++ << std::endl; // Outputs 5
std::cout << "Value after postfix increment: " << b << std::endl; // Outputs 6
return 0;
}
Understanding the Increment Operator
What is the Increment Operator? The C++ increment operator, denoted as `++`, plays a crucial role in modifying the value of variables. It is fundamental in programming, allowing developers to increase a variable's value efficiently.
Definition and Syntax
The increment operator comes in two forms: prefix and postfix. The syntax for each is:
- Prefix Increment Operator: `++variable`
- Postfix Increment Operator: `variable++`
The primary difference lies in the sequence of operations. With a prefix increment, the variable is incremented before its value is used in an expression. In contrast, with a postfix increment, the original value is used in the expression before the variable is incremented.
Types of Increment Operators
Prefix Increment Operator
The prefix increment operator (`++variable`) increments the variable's value before its value is utilized in an expression. This can be particularly useful in certain programming scenarios where immediate updates to the variable are necessary.
Consider this example:
int x = 5;
int y = ++x; // y is 6, x is 6
In this case, `x` is incremented to `6`, and then `y` is assigned the updated value.
Postfix Increment Operator
The postfix increment operator (`variable++`) performs the increment operation after the current value has been evaluated in an expression. This can lead to different outcomes in calculations.
Example:
int x = 5;
int y = x++; // y is 5, x is 6
Here, `y` takes the original value of `x` (5), while `x` is subsequently incremented to 6.
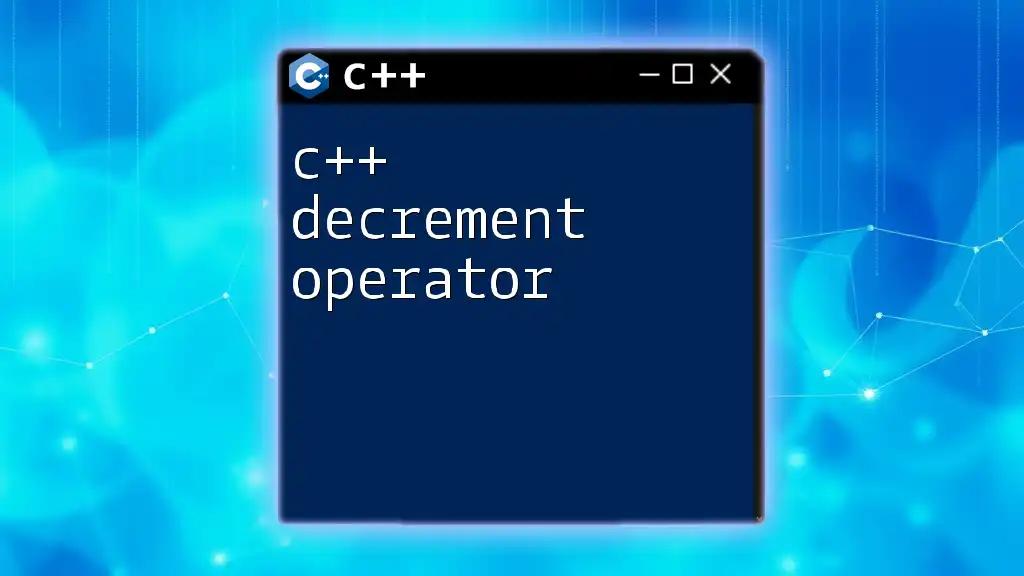
How to Use the Increment Operator in C++
Basic Examples
The simplest way to demonstrate the increment operator is through basic increments:
Prefix Increment:
int x = 5;
int y = ++x; // y is now 6, x is also 6
Postfix Increment:
int x = 5;
int y = x++; // y is now 5, x is now 6
Increment Operator in Expressions
The increment operator can also be used within larger expressions, where understanding the sequence of operations becomes crucial. For example:
int a = 5;
int b = ++a + a++; // What are the values of a and b?
In this case, `b` first receives the value from prefix increment (6) and then adds the original value of `a` (6 before increment) resulting in `b` being 12. Meanwhile, `a` ends up being 7 after the postfix increment.

Performance Considerations
Efficiency of Increment Operators
The performance of increment operators is an essential consideration for any programmer. Using the increment operator can sometimes provide better efficiency than directly modifying a variable through other means. For integer types and common data structures, increment operators are typically optimized in terms of speed and efficiency compared to other programming constructs.
For instance, utilizing `++i` within loops can be marginally faster than `i++` since it avoids unnecessary temporary variables in certain compilers, particularly in C++. This is a point worth noting, especially for performance-sensitive applications.

Common Pitfalls
Common Mistakes with the Increment Operator
Many beginners encounter common mistakes when using the increment operator due to its subtlety. One common issue arises from misunderstanding the fundamental differences between prefix and postfix increments.
For example:
int x = 3;
int result = x++ + ++x; // What's the final value of 'result'?
It's easy to miscalculate this expression, potentially leading to incorrect outcomes in larger programs.
Debugging Increment Mistakes
When debugging issues involving the increment operator, one approach is to print variable values at different stages of code execution. This can help clarify how each increment influences values, ensuring accuracy throughout your program.
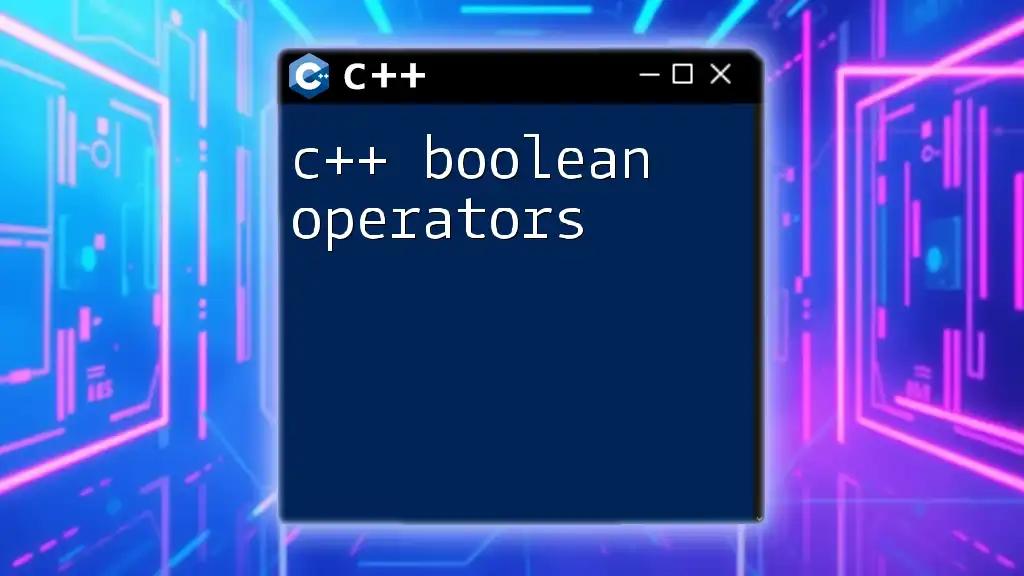
Practical Use Cases
When to Use Increment Operators
Increment operators are prevalent in loops and various algorithms. They simplify the process of iterating through a set of values or elements. For example, within a `for` loop, prefix and postfix increments can be used interchangeably, but prefix is often preferred for its slight performance advantages:
for(int i = 0; i < 10; ++i) {
// Do something with i
}
Moreover, in complex data structures like arrays or vectors, the increment operator allows for succinct iteration and clear code.
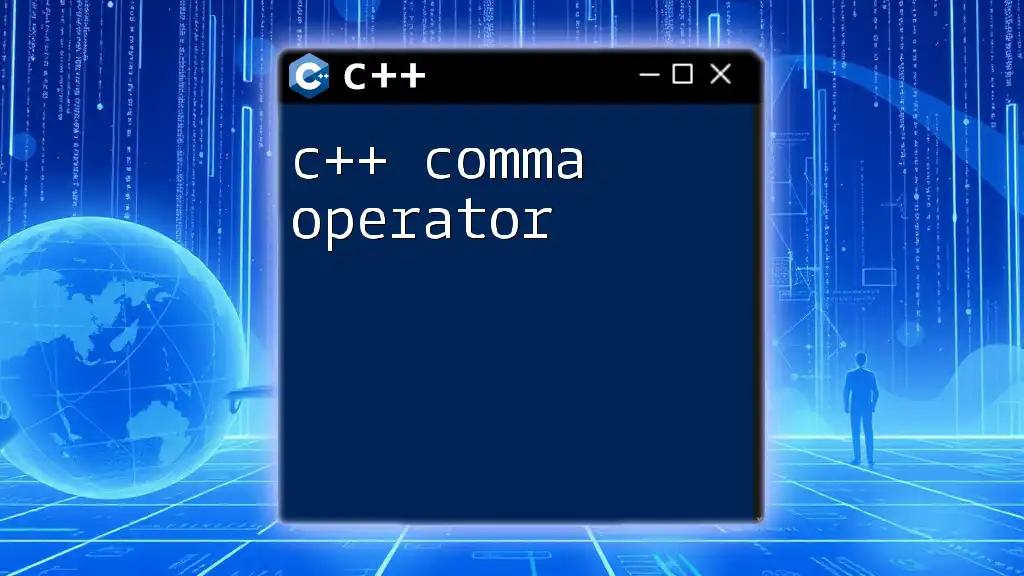
Best Practices
Choosing Between Prefix and Postfix
When deciding which increment operator to use, consider the context. If the current value is needed before incrementing, use the postfix operator. However, if you require the updated value immediately, opt for the prefix operator. This practice not only optimizes performance but also improves code readability.
Maintainability and Readability
Clarity is key in any codebase. Choose prefixes for repetitive actions or in complex expressions only when necessary. Strive for maintainable code that incorporates clear logic using increment operators. This ensures that future developers (or even you) can decipher code more readily.

Comparison with Other Operators
Increment vs Decrement Operators
The increment operator is often compared with its counterpart, the decrement operator (`--`). Similar to prefixes and postfixes, the decrement operator can be either prefix or postfix:
Prefix Decrement:
int a = 5;
int b = --a; // a is now 4, b is 4
Postfix Decrement:
int a = 5;
int b = a--; // a is now 4, b is 5
While both operators modify the value of a variable, care must be taken in their use, as they too can produce different outcomes depending on their context within expressions.
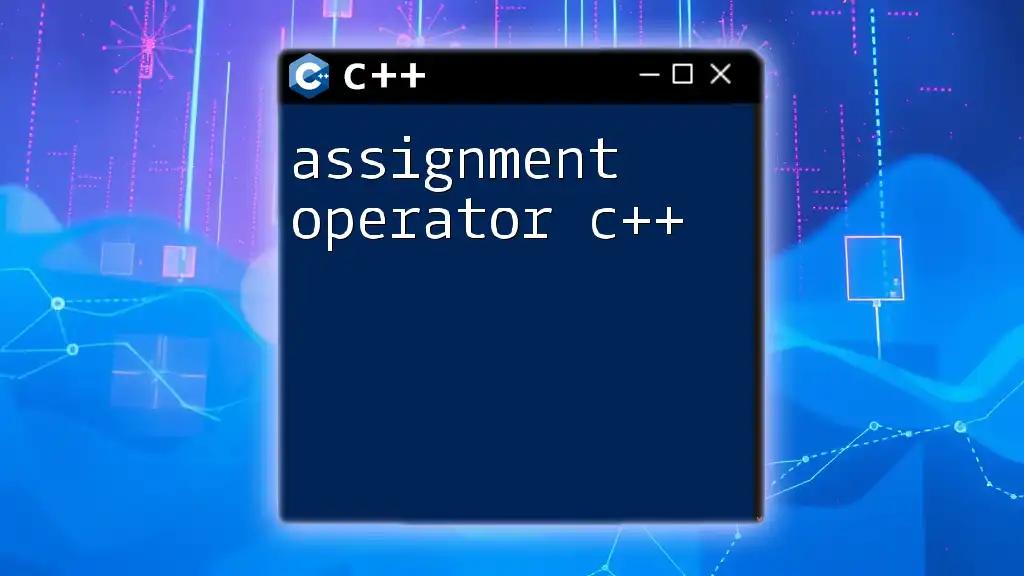
Conclusion
The C++ increment operator serves as a powerful tool in a programmer's toolkit, facilitating efficient and concise code manipulation. Understanding the nuances between prefix and postfix increment operators allows developers to make more informed decisions in their coding practices. By implementing best practices, steering clear of common pitfalls, and mastering the increment operator, you enhance both the performance and maintainability of your C++ projects.
Incorporating these lessons can lead to better coding habits and ultimately, more efficient and effective programs. Remember to practice and apply your knowledge of the increment operator in your coding journey!

Further Reading
For those looking to dive deeper into the concepts surrounding the `c++ increment operator`, consider checking out a variety of programming courses, insightful books, or comprehensive documentation available online. Engaging with these resources will further sharpen your skills and expand your programming knowledge.
If you have any experience or questions regarding the increment operator, feel free to reach out! Your feedback and insights contribute greatly to the learning community.