The C++ address operator (`&`) is used to obtain the memory address of a variable, allowing you to work with pointers effectively.
Here's a simple code snippet to illustrate its usage:
#include <iostream>
using namespace std;
int main() {
int variable = 42;
int* ptr = &variable; // Using the address operator to get the address of 'variable'
cout << "The address of variable is: " << ptr << endl;
return 0;
}
What is the Address Operator?
The C++ address operator, represented by the symbol `&`, is a crucial tool in the C++ programming language, used primarily to retrieve the memory address of a variable. Understanding this operator is essential not just for managing memory effectively, but also for manipulating variables in a more granular manner through pointers and references.
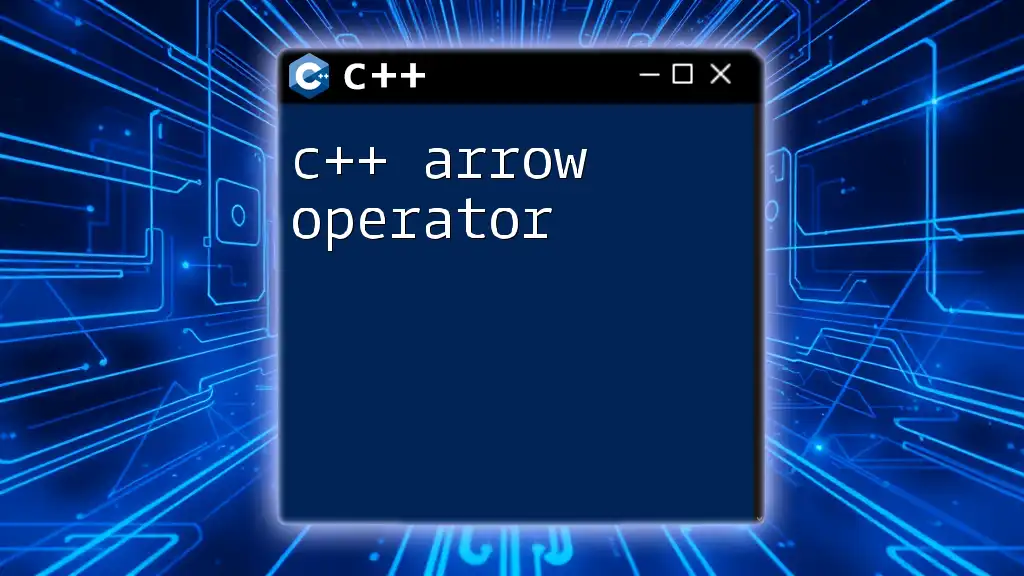
Importance of Understanding Address Operator in C++
Mastering the address operator enhances your capabilities as a C++ programmer. It allows for efficient memory management and optimization, making your programs faster and more efficient. Furthermore, many advanced programming concepts—such as pointers, dynamic memory allocation, and references—rely on a solid understanding of the address operator.
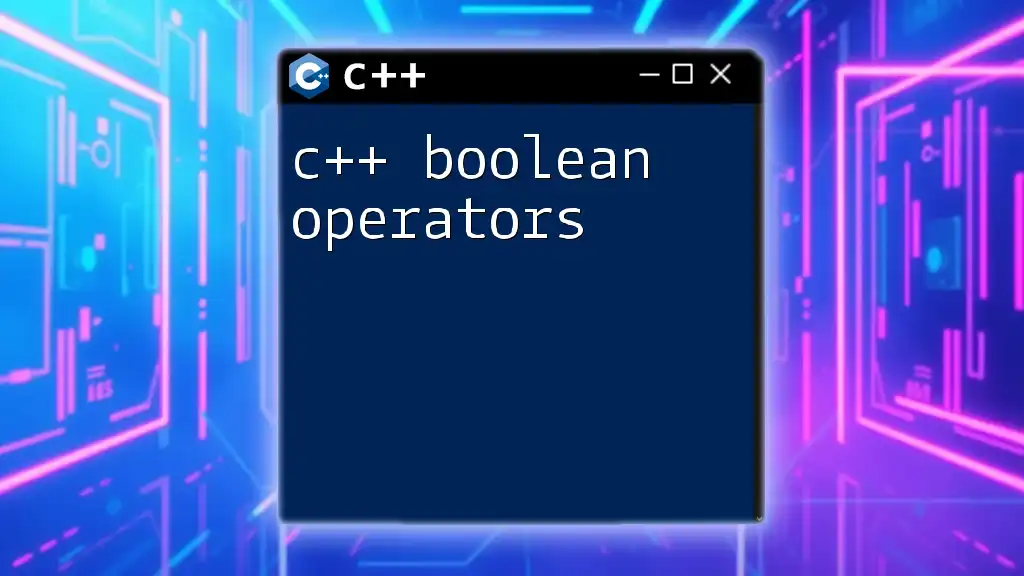
How the Address Operator Works
Basic Operation
At its core, the address operator is used to obtain the precise memory location of a variable. The syntax might look simplistic but carries significant weight in how variables are accessed and manipulated in C++.
Here's a basic code snippet demonstrating its fundamental use:
int main() {
int a = 10;
int* ptr = &a; // Using the address operator
std::cout << "Address of a: " << ptr << std::endl;
return 0;
}
In this example, the variable `a` is initialized with a value of `10`. By using `&a`, we retrieve the address where `a` is stored in memory, and store that address in a pointer called `ptr`. When we print `ptr`, we see the memory address of `a`.
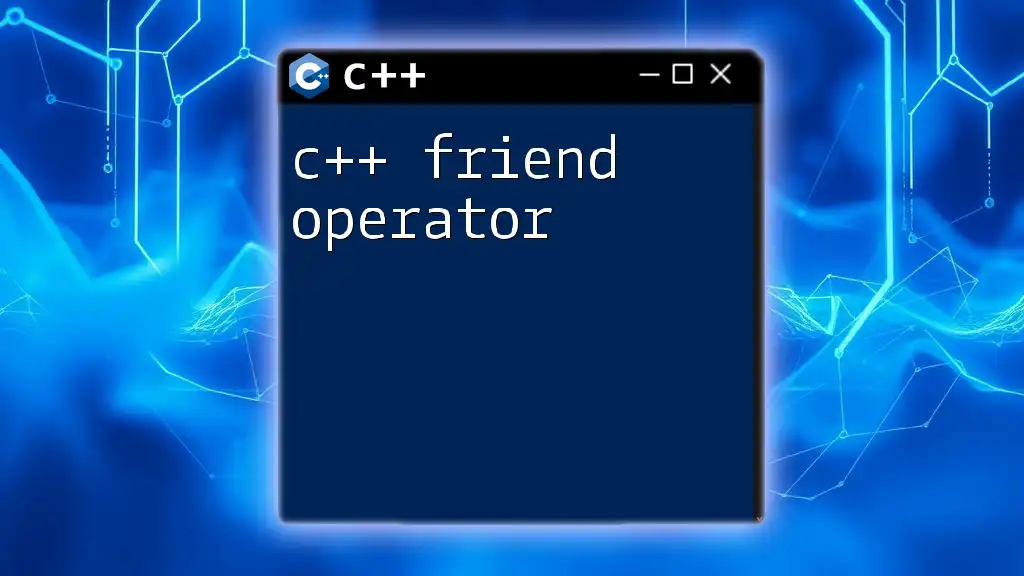
Practical Applications of the Address Operator
Working with Pointers
Pointers are variables that hold memory addresses. They allow for efficient data manipulation and dynamics in managing resources. The address operator plays a pivotal role in pointer creation and usage.
Here's an example:
int main() {
int var = 20;
int* pVar = &var; // Address of var
std::cout << "Value of var: " << *pVar << "\n"; // Dereferencing
return 0;
}
In this snippet, `pVar` holds the address of `var`, and by using the dereference operator `*`, we can access the value stored at that address (in this case, `20`). This is a foundational concept in C++ programming that enables direct interaction with memory.
Dynamic Memory Allocation
The address operator is also essential in dynamic memory allocation, where memory can be allocated at runtime using the `new` keyword. This approach gives programmers flexibility but demands careful management of memory resources.
Consider the following example of dynamic memory allocation:
#include <iostream>
using namespace std;
int main() {
int* arr = new int[5]; // Dynamically allocating memory for 5 integers
for(int i = 0; i < 5; i++) {
arr[i] = i + 1; // Assigning values
}
cout << "First element's address: " << &arr[0] << endl; // Using address operator
delete[] arr; // Freeing allocated memory
return 0;
}
In this code, `new int[5]` allocates memory for an array of 5 integers. We can access the address of the first element in this dynamically allocated array with `&arr[0]`. It’s crucial to remember to `delete` the allocated memory when you’re done using it to prevent memory leaks.
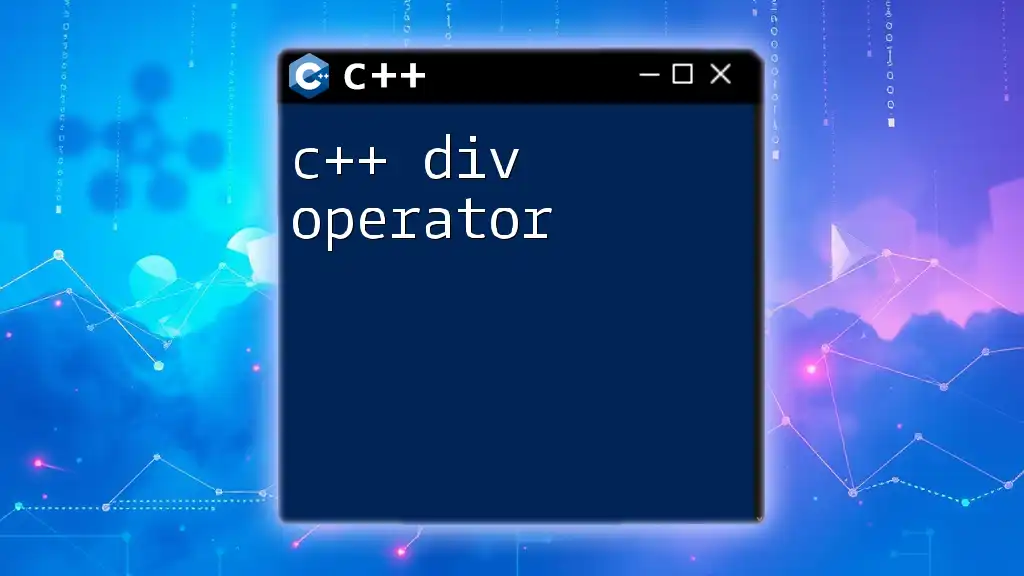
Address Operator in Function Parameters
Passing by Reference
Another powerful feature of the C++ address operator is its role in passing variables to functions by reference. This technique allows the function to modify the original variable directly without creating a copy, which can improve performance.
Here’s an illustrative example:
void increment(int &num) {
num++; // Modifies the original value
}
int main() {
int x = 5;
increment(x); // Passing x by reference
std::cout << "Incremented value: " << x << std::endl; // Outputs 6
return 0;
}
In this case, `increment(x)` is called with `x` passed by reference. Thus, any changes made within the `increment` function directly affect `x`. This method is highly efficient and beneficial when dealing with large data structures.
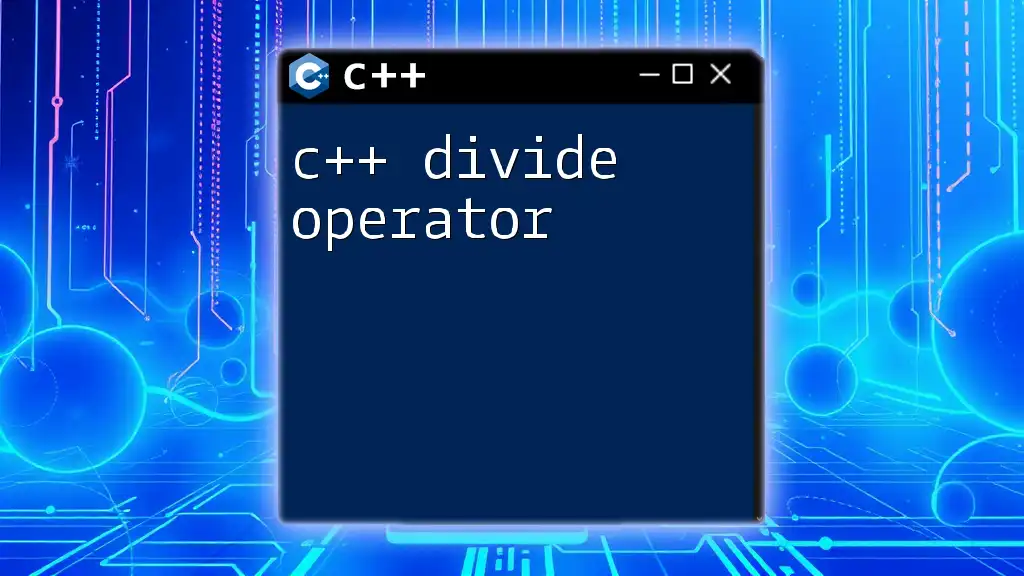
Differences Between Address Operator and Dereference Operator
Understanding Dereference Operator (`*`)
While the address operator retrieves the memory address, the dereference operator (`*`) allows you to access the value at that memory address. Understanding how to use both operators effectively is vital for manipulating the memory directly.
Consider the following example:
int main() {
int var = 30;
int* pVar = &var; // Get address of var
std::cout << "Using dereference operator: " << *pVar << std::endl; // Accessing value at address
return 0;
}
Here, `pVar` points to `var`, and using `*pVar` retrieves the value stored at that address (in this case, `30`).
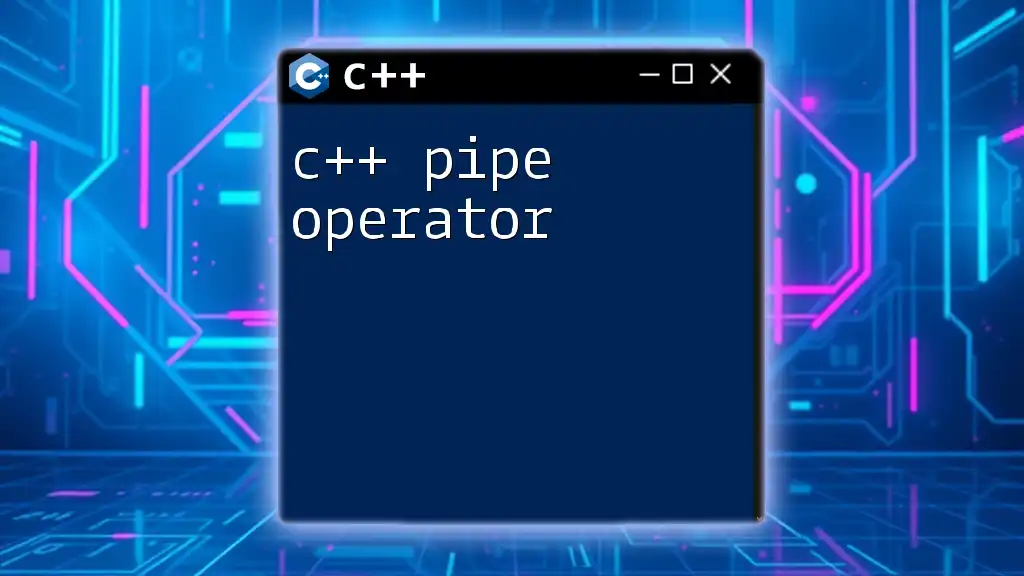
Common Mistakes with Address Operator
Uninitialized Pointers
One of the most prevalent mistakes when dealing with the address operator is using it with uninitialized pointers. Attempting to dereference such pointers can lead to undefined behavior or program crashes. Always ensure that your pointers are initialized before use.
Dangling Pointers
Another issue to be aware of is dangling pointers—pointers that refer to memory that has already been freed. This can happen if you use the address operator on a variable that has gone out of scope or has been deleted.
To prevent these issues, you should set pointers to `nullptr` after freeing their associated memory.
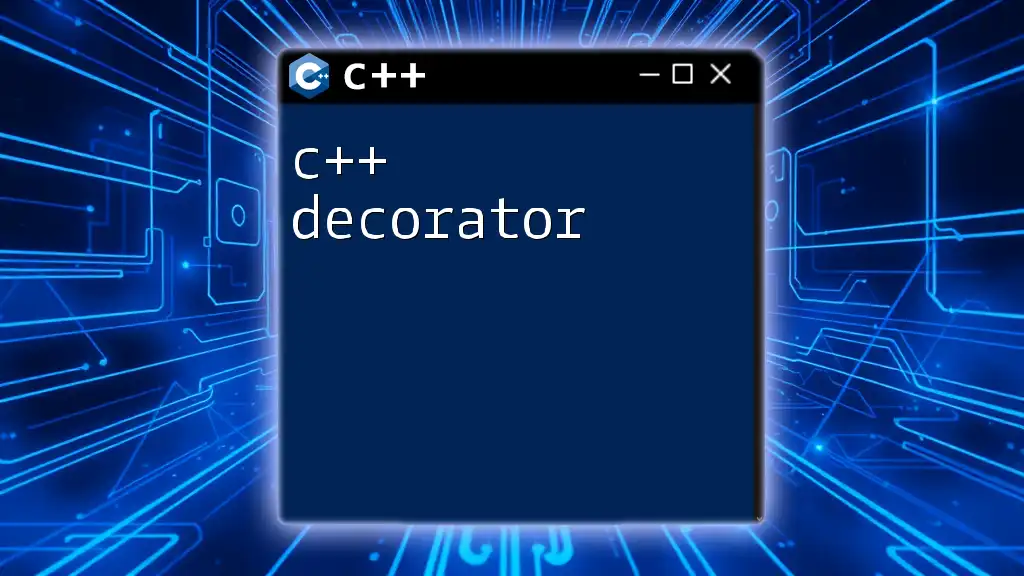
Best Practices for Using the Address Operator
Always Initialize Pointers
When declaring pointers, always initialize them to either a memory address or `nullptr`. This helps avoid accidental dereferencing of uninitialized pointers, which is a common source of bugs.
Memory Management
Be vigilant about memory management. When using dynamic allocation, always pair `new` with `delete` to ensure that allocated memory is properly freed. Failing to do so can lead to memory leaks that can degrade program performance over time.
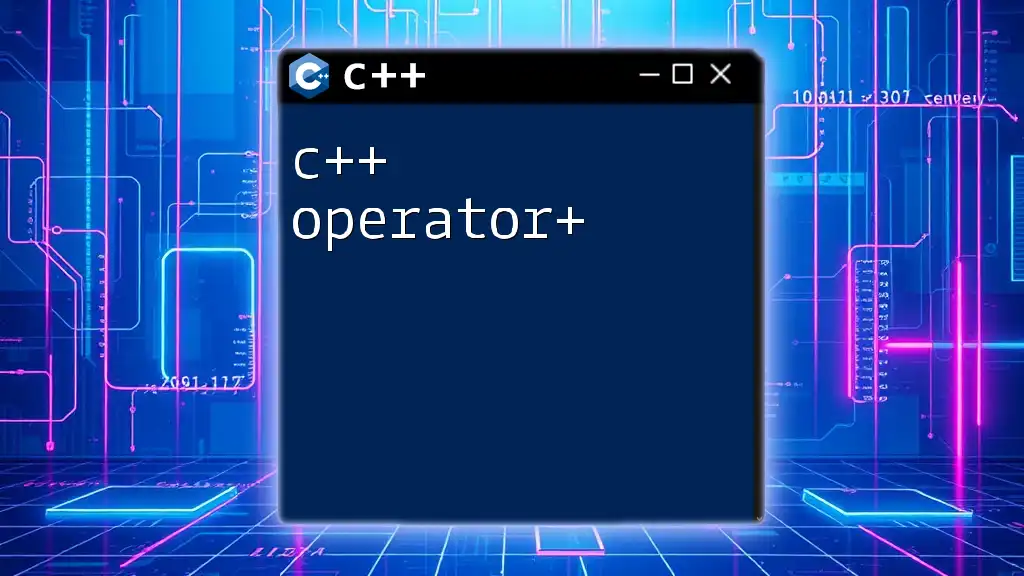
Conclusion
Understanding the C++ address operator is essential for effective programming in C++. By grasping how it works and applying it in practical scenarios—such as with pointers, references, and dynamic memory—you can write more efficient and powerful C++ code. I encourage you to practice using the address operator in your projects, as hands-on experience reinforces learning and enhances your programming skills.
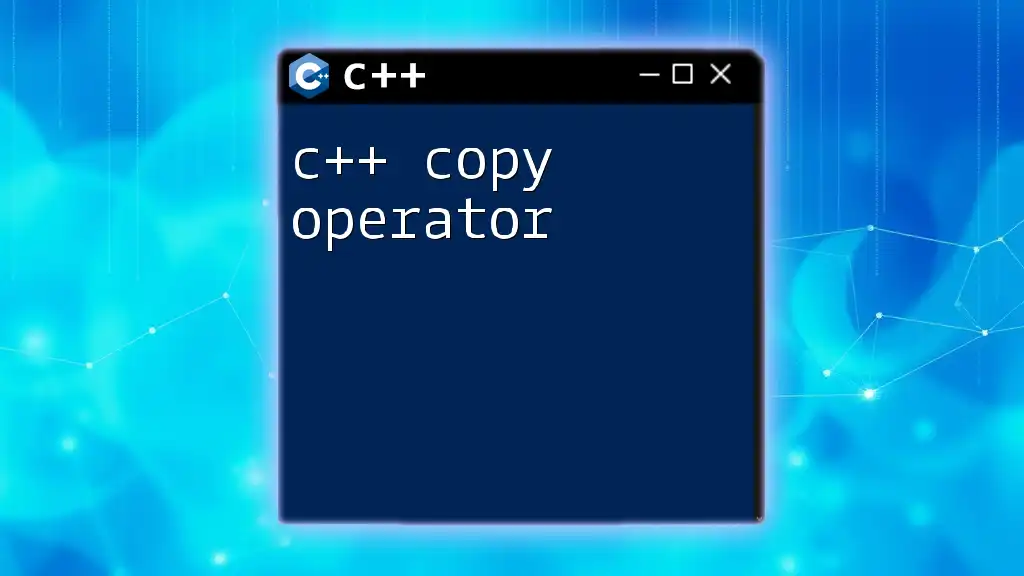
Additional Resources
To further enhance your understanding of the C++ address operator and related concepts, consider exploring additional reading materials focused on pointers, references, and memory allocation strategies in C++.