The C++ copy operator, also known as the copy assignment operator, allows you to assign the values of one object to another existing object of the same class type.
Here’s a simple code snippet to illustrate its usage:
class MyClass {
public:
int value;
// Copy assignment operator
MyClass& operator=(const MyClass& other) {
if (this != &other) {
value = other.value;
}
return *this;
}
};
Understanding the Copy Operator in C++
The C++ copy operator is a fundamental concept in C++, allowing developers to create copies of objects. It serves as a bridge between the original object and its duplicate, managing how data is transferred from one instance to another. Understanding this mechanism is vital for effectively managing resources and ensuring efficient program execution.
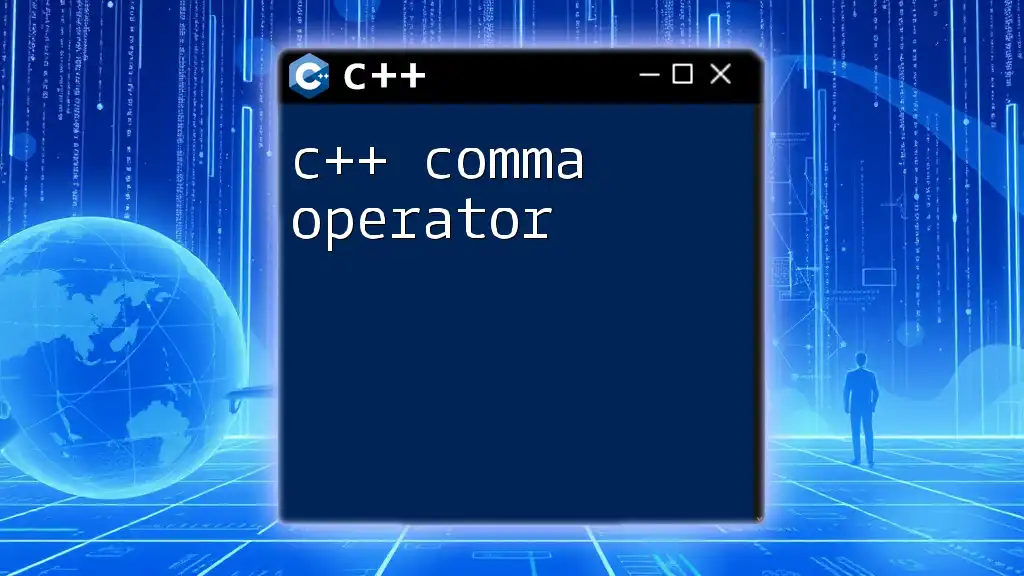
The Copy Assignment Operator in C++
What is the Copy Assignment Operator?
The copy assignment operator is a special member function defined in a class that facilitates the copying of objects after their construction. Unlike the copy constructor, which initializes a new object from an existing one, the copy assignment operator allows an already initialized object to take on the value of another existing object.
Why Use a Copy Assignment Operator?
C++ supports two different kinds of semantics for object handling: value semantics and reference semantics. The copy assignment operator embodies value semantics, allowing for independent objects that can be modified without affecting one another.
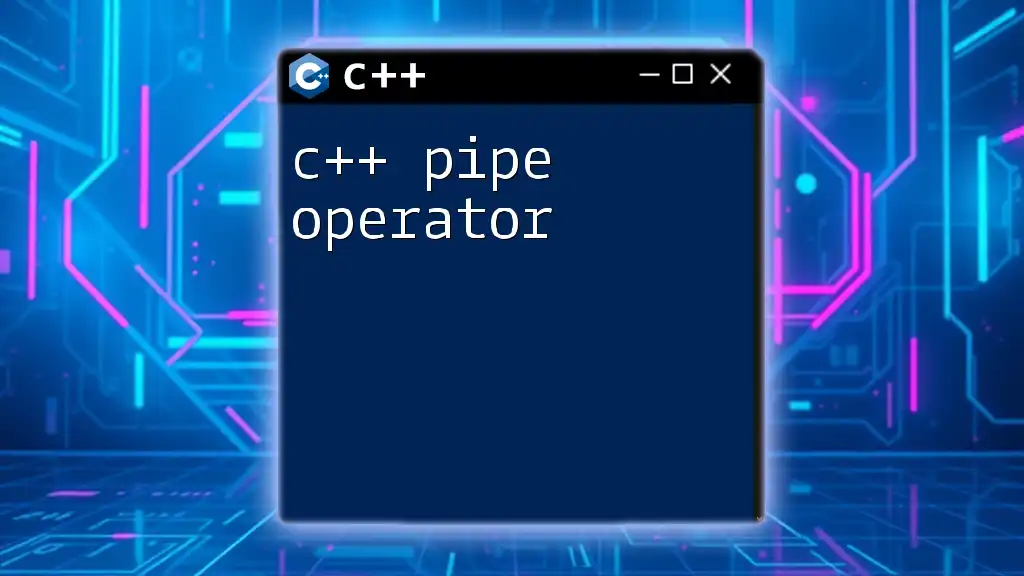
Implementing the Copy Assignment Operator
Creating a Copy Assignment Operator
Here’s how to construct a copy assignment operator in a C++ class:
- Define the operator: This operator should have a return type of the class itself.
- Input parameter: The parameter should be a constant reference to another object of the same class to prevent unnecessary copies.
- Self-assignment check: It's crucial to verify that the object isn't being assigned to itself, which would lead to resource conflicts.
- Copy member variables: Finally, copy the member variables from the source object to the current object.
Example: Implementing a Simple Class
class Example {
public:
int data;
// Copy Assignment Operator
Example& operator=(const Example& other) {
if (this != &other) { // Self-assignment check
this->data = other.data;
}
return *this; // Return the current object
}
};
In this example, the `operator=` function first checks if `this` is the same as `other`. If they are different, it copies the `data` member variable. Finally, it returns a reference to the current object, enabling chained assignments.
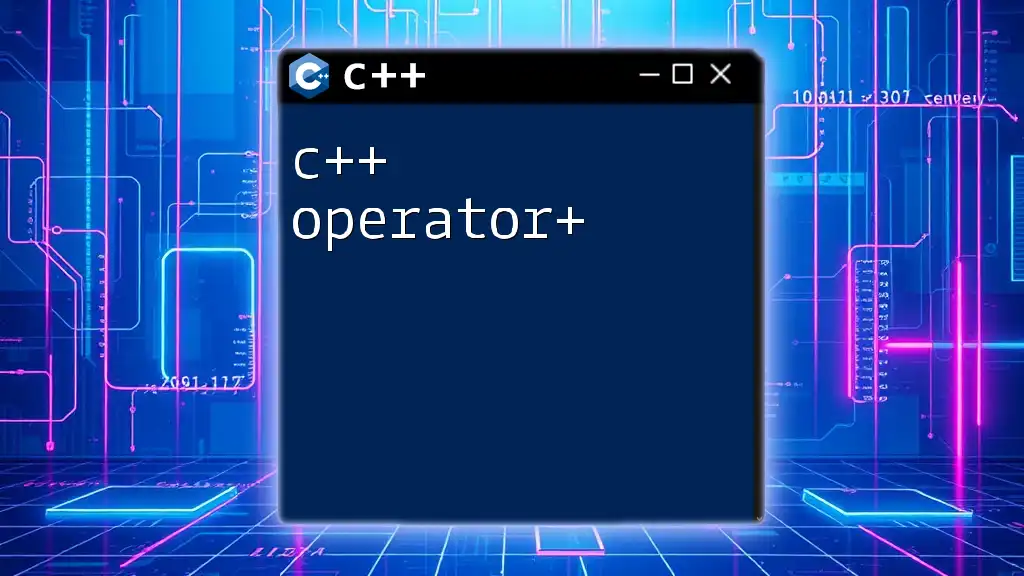
Best Practices for Copy Assignment Operators
Implementing the Rule of Three
In C++, The Rule of Three states that if a class requires a user-defined destructor, copy constructor, or copy assignment operator, it likely requires all three. This rule emphasizes the need for managing resources properly, especially when dealing with dynamic memory.
Const and Reference Parameters
Passing by constant reference is a best practice that avoids unnecessary copying and enhances performance.
Avoiding Common Mistakes
It's essential to implement a self-assignment check to prevent potential errors during the copy assignment. Returning `*this` is crucial to enable chaining assignments, making the operator more functional.
![Unlocking the C++ [] Operator: A Step-by-Step Guide](/images/posts/c/cpp-operator.webp)
Copy Operator C++: Dealing with Dynamic Memory
When to Use Dynamic Memory
Dynamic memory allocation is often necessary when the size of the data structure (like arrays) isn't known at compile time. This necessitates careful management to prevent memory leaks.
Implementing Copy Assignment with Dynamic Memory
When dealing with dynamic memory, the copy assignment operator must ensure the following:
- Free existing memory: Before allocating new memory, it's essential to release any previously allocated memory to prevent leaks.
- Allocate new memory: Allocate fresh space for the new object’s data.
- Copy data: Copy the contents from the source to the newly allocated space.
This is how it can be implemented:
class DynamicArray {
private:
int* arr;
size_t size;
public:
DynamicArray(size_t s) : size(s), arr(new int[s]) {}
// Copy Assignment Operator
DynamicArray& operator=(const DynamicArray& other) {
if (this != &other) {
delete[] arr; // Free existing resource
size = other.size;
arr = new int[size]; // Allocate new resource
for (size_t i = 0; i < size; ++i) {
arr[i] = other.arr[i]; // Copy data
}
}
return *this;
}
~DynamicArray() {
delete[] arr; // Destructor to clean up
}
};
In this example, `delete[] arr` is used to free the memory previously held by the object before allocating new memory based on `other.size`. This careful management of memory ensures that the program runs efficiently without resource leaks.
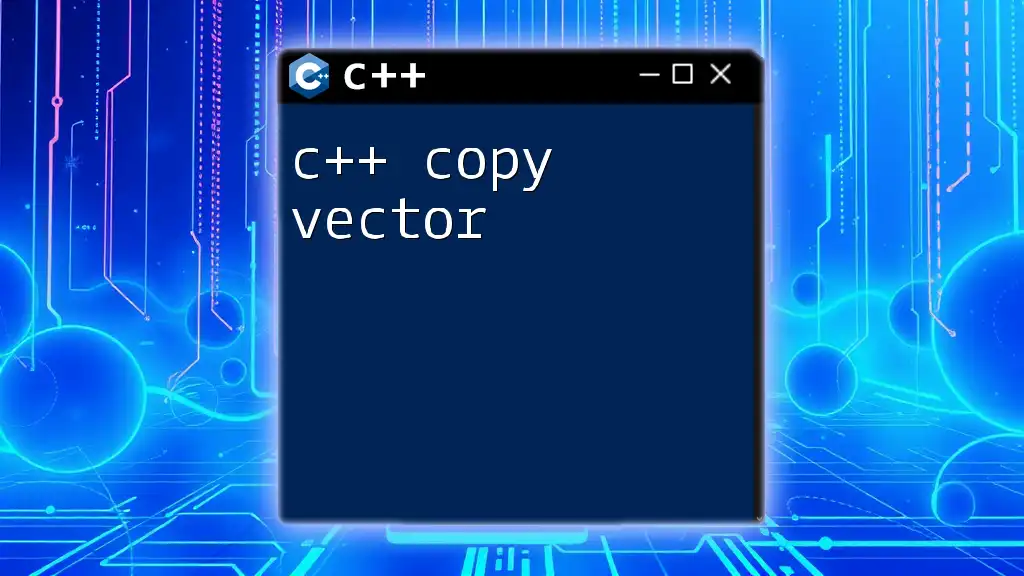
Performance Considerations
Copy Elision and Return Value Optimization (RVO)
Copy elision is an optimization technique that eliminates unnecessary copying of objects during assignments. This results in performance gains by allowing the compiler to construct objects directly in the location where they will be used.
When to Avoid Copy Assignment
In scenarios where copying is redundant or inefficient, such as when objects are large or frequently used in multi-threaded contexts, it’s advisable to disable copying by declaring the copy assignment operator as deleted:
class NoCopy {
public:
NoCopy(const NoCopy&) = delete;
NoCopy& operator=(const NoCopy&) = delete;
};
This will prevent inadvertent copies, enforcing better use of resources and minimizing overhead.
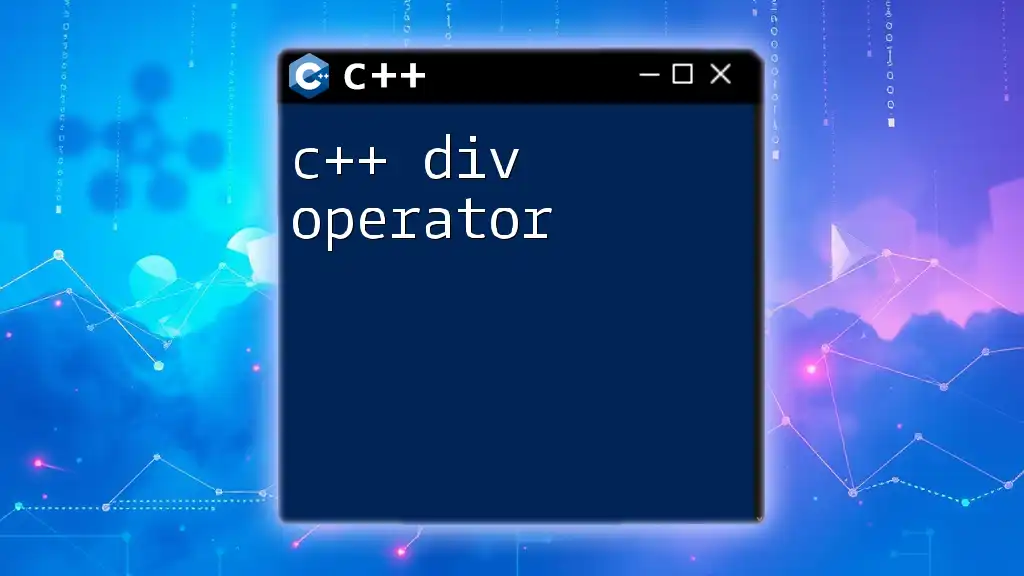
Conclusion
In summary, understanding the C++ copy operator is imperative for effective C++ programming. By mastering the copy assignment operator, developers can write more efficient, error-free code while managing resources deftly. When you follow best practices—like the Rule of Three and self-assignment checks—you’ll create robust applications that minimize memory leaks and enhance performance.
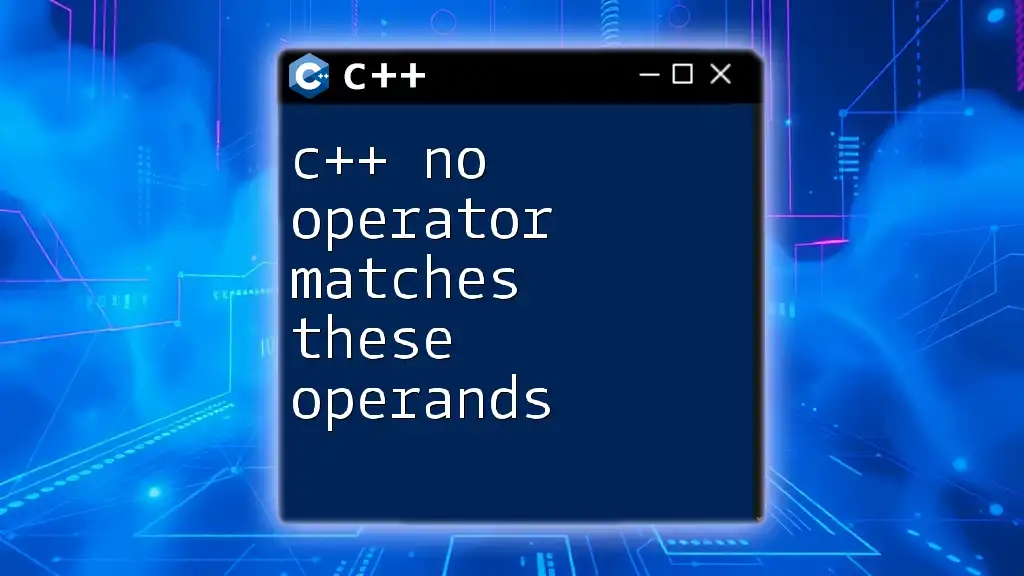
FAQ Section
-
What happens if I don’t define a Copy Assignment Operator?
If not defined, the compiler will provide a default implementation. However, this may not handle dynamic memory correctly, leading to double deletions or memory leaks. -
What are the implications of copied objects in multithreaded applications?
Careful synchronization is needed to avoid data races, especially if objects are shared across threads. Using smart pointers might alleviate some concerns associated with memory management and object ownership.