The `operator` keyword in C++ is used to define or overload operators for user-defined types, allowing instances of classes to be manipulated with standard operators like +, -, and *.
Here’s an example of how to overload the addition operator for a simple class:
#include <iostream>
class Point {
public:
int x, y;
Point(int x, int y) : x(x), y(y) {}
// Overloading the + operator
Point operator+(const Point& other) {
return Point(this->x + other.x, this->y + other.y);
}
};
int main() {
Point p1(1, 2);
Point p2(3, 4);
Point p3 = p1 + p2; // Using the overloaded + operator
std::cout << "Point p3: (" << p3.x << ", " << p3.y << ")" << std::endl; // Output: Point p3: (4, 6)
return 0;
}
What is the Operator Keyword?
The operator keyword in C++ is essential for enabling operator overloading, allowing programmers to redefine the way operators work with user-defined types. By creating custom behavior for operators such as `+`, `-`, `*`, and `==`, developers can write code that is not only clearer but also more intuitive.
Why Use Operator Overloading?
Operator overloading can vastly improve the readability and usability of your code. For instance, when working with complex numbers or custom vector types, using `+` to add them together conveys meaning far clearer than calling a method like `add()`. Overloading allows operations to be performed in a straightforward way, closely resembling how they work with fundamental data types. This clarity is particularly beneficial in applications like mathematical libraries, graphics programming, or any domain where custom types are prevalent.
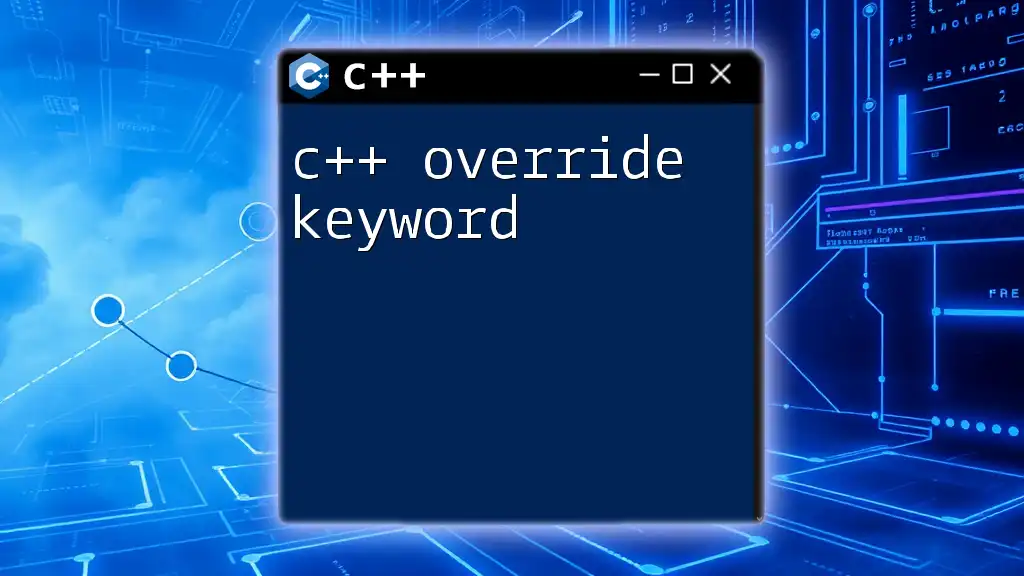
Basic Syntax of the Operator Keyword
The syntax for overloading an operator is straightforward but follows specific rules:
- The function must be a member function of a class or a friend function.
- The operator keyword precedes the function declaration.
Here’s an example of a simple operator overload for a class representing complex numbers:
class Complex {
public:
float real;
float imag;
// Overload the + operator
Complex operator+(const Complex& other) {
Complex result;
result.real = this->real + other.real;
result.imag = this->imag + other.imag;
return result;
}
};
In this example, the `operator+` function is defined to take another `Complex` object and return a new `Complex` object that represents the sum of the two complex numbers.
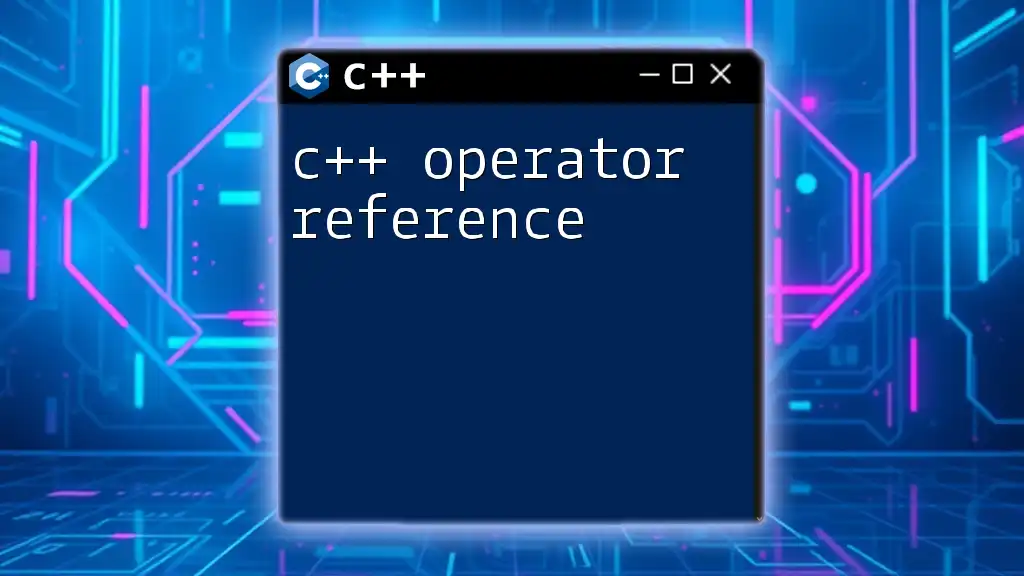
Types of Operators That Can Be Overloaded
C++ allows a wide range of operators to be overloaded, but some of the most common types include:
Arithmetic Operators
These include basic operations such as addition, subtraction, multiplication, and division. Overloading these operators can create a seamless integration of calculations with user-defined types.
Example of Overloading Arithmetic Operators
Consider a simple vector class where the addition and subtraction of vectors make sense:
class Vector {
public:
int x, y;
// Overloading +
Vector operator+(const Vector& v) {
Vector result;
result.x = this->x + v.x;
result.y = this->y + v.y;
return result;
}
// Overloading -
Vector operator-(const Vector& v) {
Vector result;
result.x = this->x - v.x;
result.y = this->y - v.y;
return result;
}
};
In this example, you can add and subtract vectors intuitively, using `+` and `-` without explicit function calls.
Comparison Operators
These operators allow for the comparison of two objects. For example, you can define how two objects are compared through overloaded operators like `==` and `>`.
Code Example for Comparison Operators
class Person {
public:
std::string name;
int age;
// Overloading ==
bool operator==(const Person& other) const {
return (this->name == other.name && this->age == other.age);
}
// Overloading >
bool operator>(const Person& other) const {
return this->age > other.age;
}
};
In this case, we can easily compare two `Person` objects directly using `==` and `>`.
Increment and Decrement Operators
These operators allow the use of incremental changes in a succinct manner. C++ allows both prefix (`++x`) and postfix (`x++`) forms to be overloaded.
Code Example for Increment and Decrement Operators
class Counter {
public:
int count;
// Overloading prefix increment
Counter& operator++() {
++count;
return *this;
}
// Overloading postfix increment
Counter operator++(int) {
Counter temp = *this;
++count;
return temp;
}
};
This allows you to increment a `Counter` object in an intuitive manner while also illustrating the difference between prefix and postfix operations.
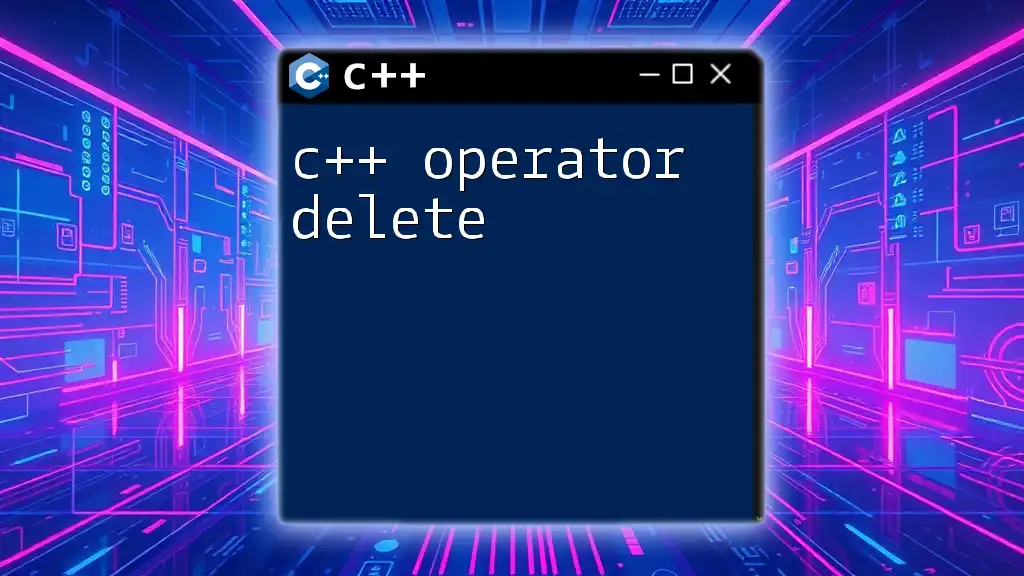
Special Member Functions Involved with Overloading
Copy Constructor
When operator overloading is used, implementing a copy constructor is critical to managing resources correctly. This ensures that copies of objects behave as expected, especially when they involve dynamic memory or complex data structures.
Assignment Operator
The assignment operator is equally important. It must be overloaded when you have resources that need to be duplicated or released correctly. Here’s how it can be done:
Vector& operator=(const Vector& v) {
if (this != &v) {
this->x = v.x;
this->y = v.y;
}
return *this;
}
This implementation protects against self-assignment and ensures the correct copying of data.
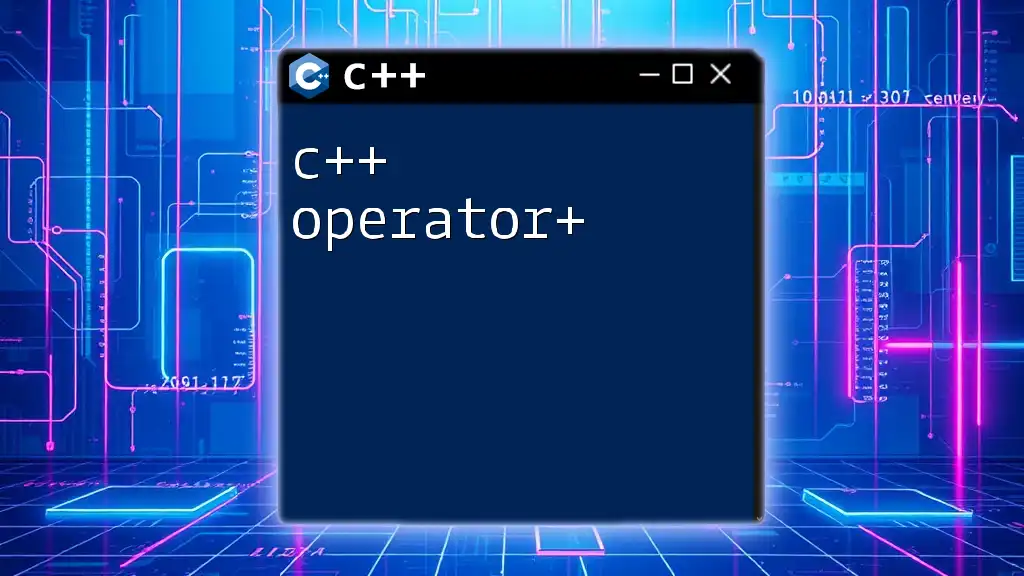
Rules and Best Practices for Using the Operator Keyword
Following best practices is essential when overloading operators:
- Maintain intuitive behavior similar to built-in types.
- Ensure that overloaded operators handle left-hand and right-hand operand situations effectively.
- Avoid overloading operators for types where the operation doesn't make sense or would confuse the code's intent.
Common Mistakes to Avoid
Avoid making incorrect assumptions about how overloaded operators function—particularly in scenarios involving const correctness and the potential for unintended side effects. Proper encapsulation must also be maintained to avoid exposing sensitive data or operations inadvertently.
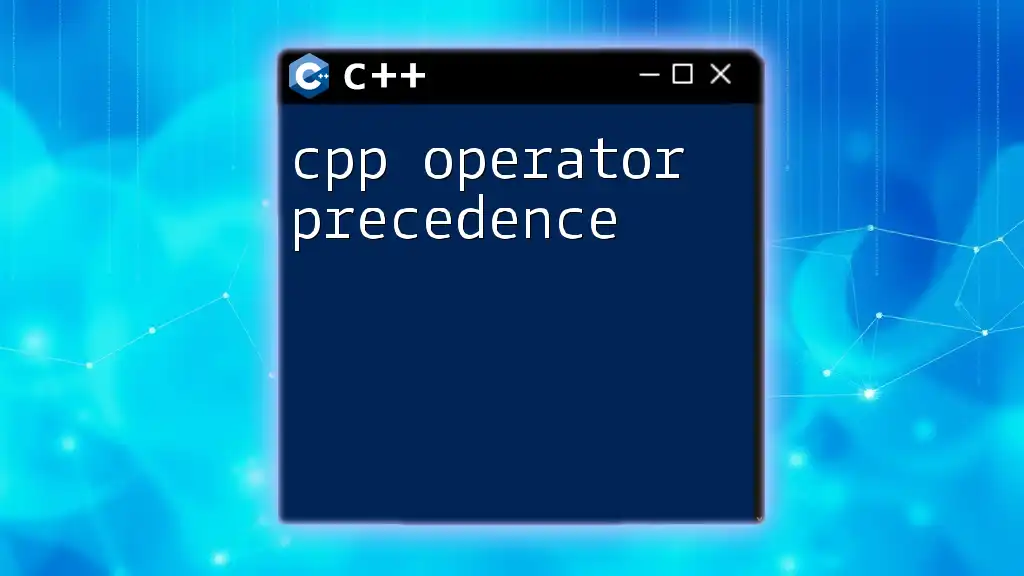
Limitations of Operator Overloading
While operator overloading is a powerful feature in C++, some operators cannot be overloaded, which includes:
- The scope resolution operator `::`
- The member access operator `.`
- The pointer-to-member operator `.*`
- The size of operator `sizeof`
Recognizing these limitations is critical in developing robust, maintainable code.
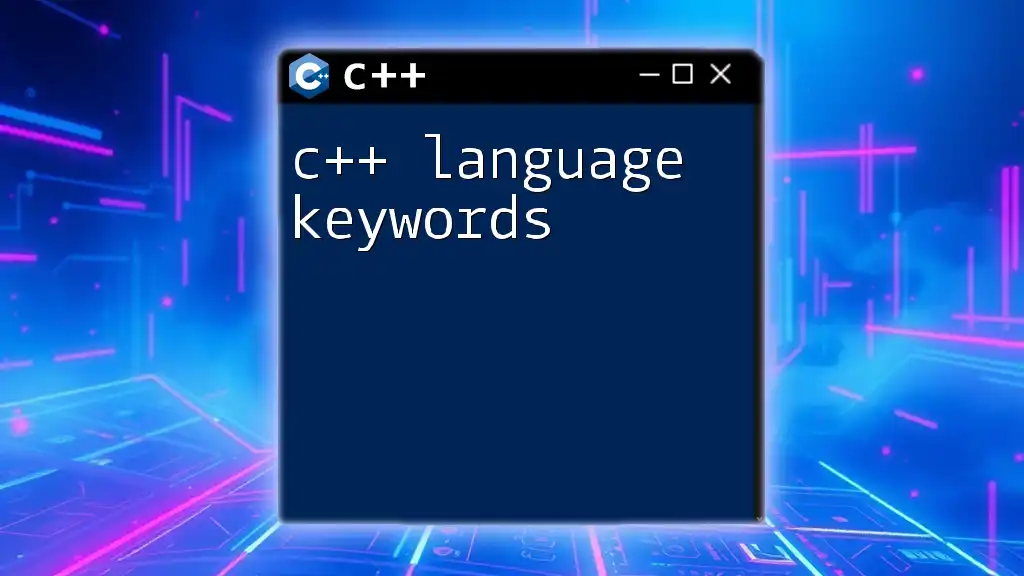
Conclusion
In summary, the C++ operator keyword plays a pivotal role in extending the functionality of operators to user-defined types. By leveraging operator overloading intelligently, you can create clearer, more expressive code that closely mimics the behavior of built-in types. Dive into experimenting with operator overloading in your projects to unlock its full potential!
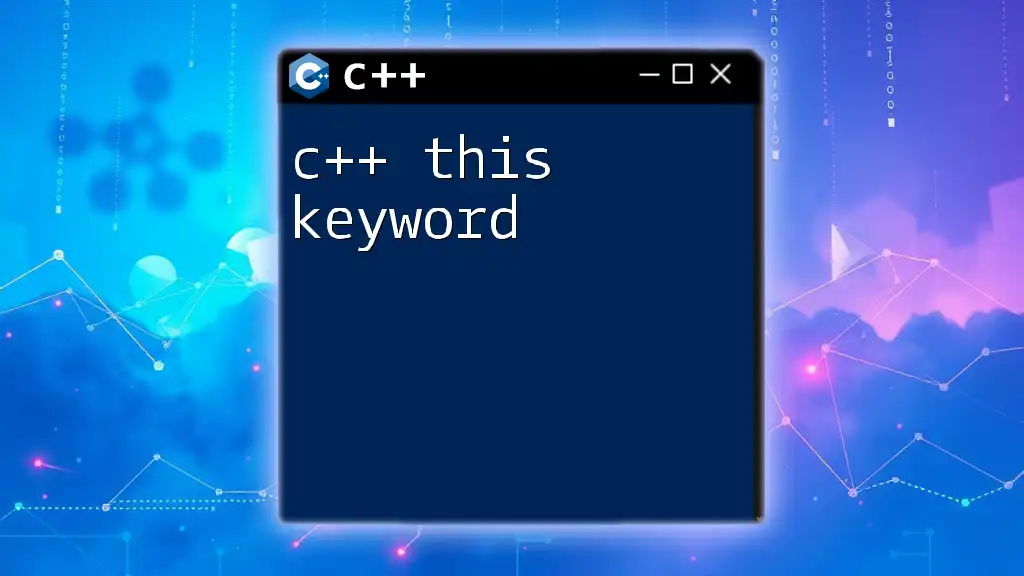
Additional Resources
Books and online courses encompassing these concepts along with community forums can help solidify your understanding of C++ and the operator keyword. Engaging with these resources can elevate your coding proficiency and practical application of C++ in everyday programming tasks.
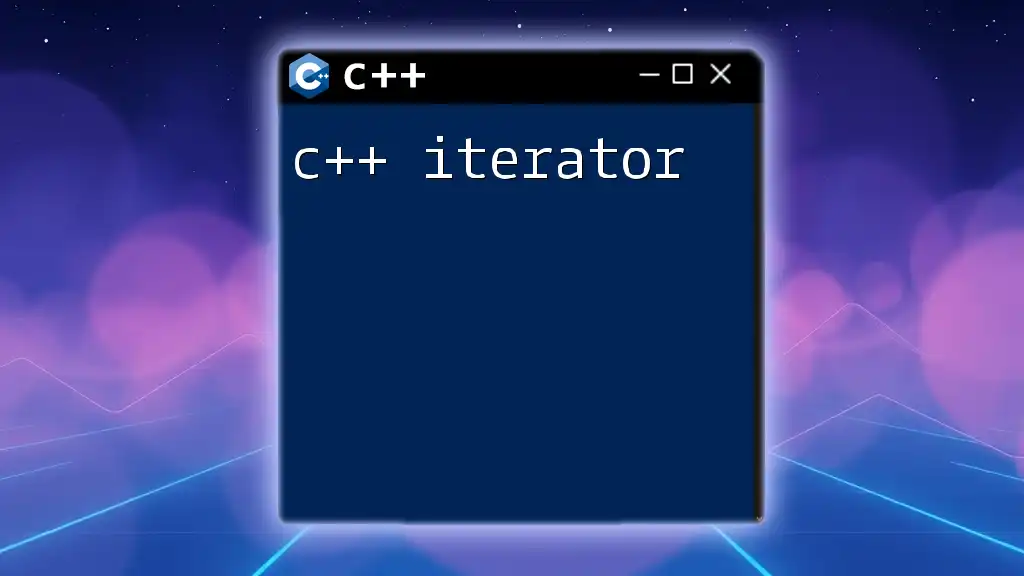
Call to Action
We encourage you to join our C++ learning community where you can gain even more insights and practical tutorials on the C++ operator keyword and various other important features of C++. Engaging with us can help foster your growth and mastery in C++ programming!