The `this` keyword in C++ is a special pointer that refers to the current object within a class, allowing access to its members and methods.
class Example {
public:
void display() {
std::cout << "Value of this: " << this << std::endl;
}
};
What is the `this` Keyword in C++?
The `this` keyword in C++ is a special pointer that is always implicitly passed to non-static member functions. It points to the object for which the member function is called, effectively allowing you to access the instance variables and methods directly. Understanding the `this` keyword is essential in the context of object-oriented programming, as it helps maintain clarity and efficiency in your code.
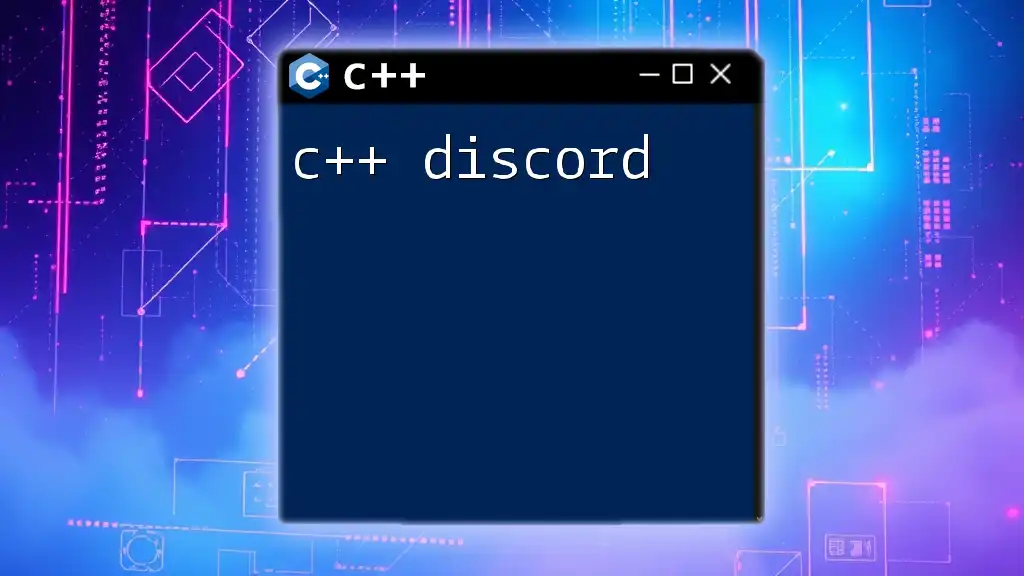
Understanding `this` Keyword Syntax
The syntax of the `this` keyword is straightforward. It is used without any special declaration when you are working inside a non-static member function:
class MyClass {
public:
void myMethod() {
// 'this' refers to the current instance of MyClass
}
};
In the above example, `this` implicitly refers to the calling object of `MyClass`. Importantly, `this` is of the type of the class being defined; hence, it allows you to access the members of the class seamlessly.
One common confusion arises when differentiating between `this` and other pointers. Unlike a regular pointer, `this` cannot be modified. It always references the current object instance, making it a reliable tool for accessing class members.
How `this` Enhances Readability
One of the ways `this` improves the readability of your code is by helping differentiate between member variables and parameters that may share the same name. Consider the following example:
class Sample {
int value;
public:
Sample(int value) {
this->value = value; // Distinguishing between parameter and member variable
}
};
Here, the constructor takes a parameter named `value`, which is the same as the class member variable. Using `this` clarifies which `value` you are referring to.
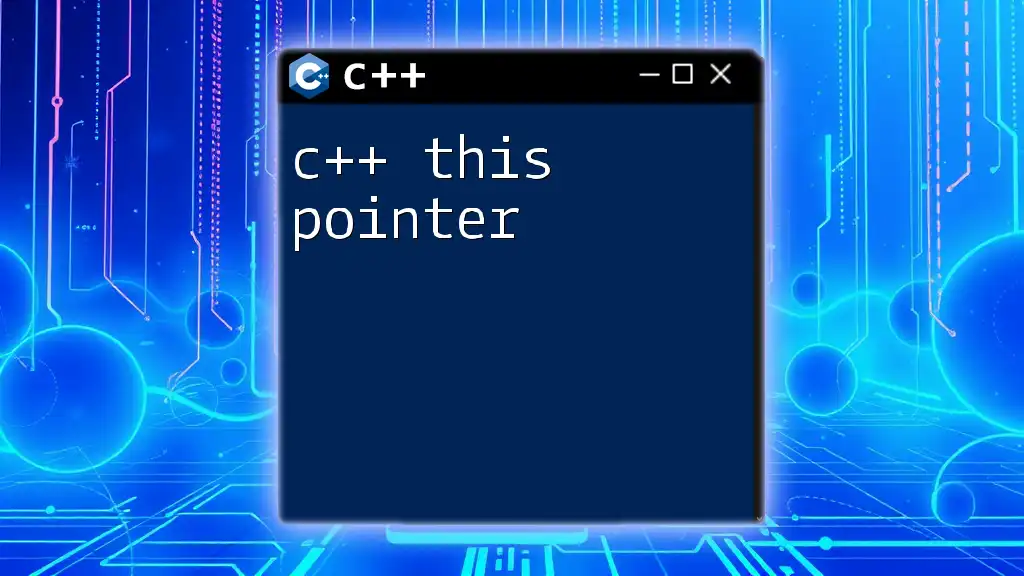
Practical Uses of the `this` Keyword in C++
Accessing Object Members
The `this` keyword can be a powerful tool when you need to access class attributes directly. For instance, consider a rectangle class where you implement its dimensions using the `this` keyword:
class Rectangle {
int width, height;
public:
Rectangle(int width, int height) {
this->width = width; // Using 'this' to avoid shadowing
this->height = height; // Using 'this' for clarity
}
};
In this example, employing `this` aids in distinguishing between the member variables `width` and `height` and the constructor parameters of the same name, thus enhancing the code's clarity.
Chaining Member Function Calls
Another practical application of `this` is in method chaining, where functions return the current object itself. This is particularly useful in creating a more fluent interface:
class Builder {
int value;
public:
Builder& setValue(int v) {
this->value = v;
return *this; // Returning current object
}
};
Using `this->value` allows for a clear assignment within the function, while returning `*this` enables the chaining of calls to `setValue`.
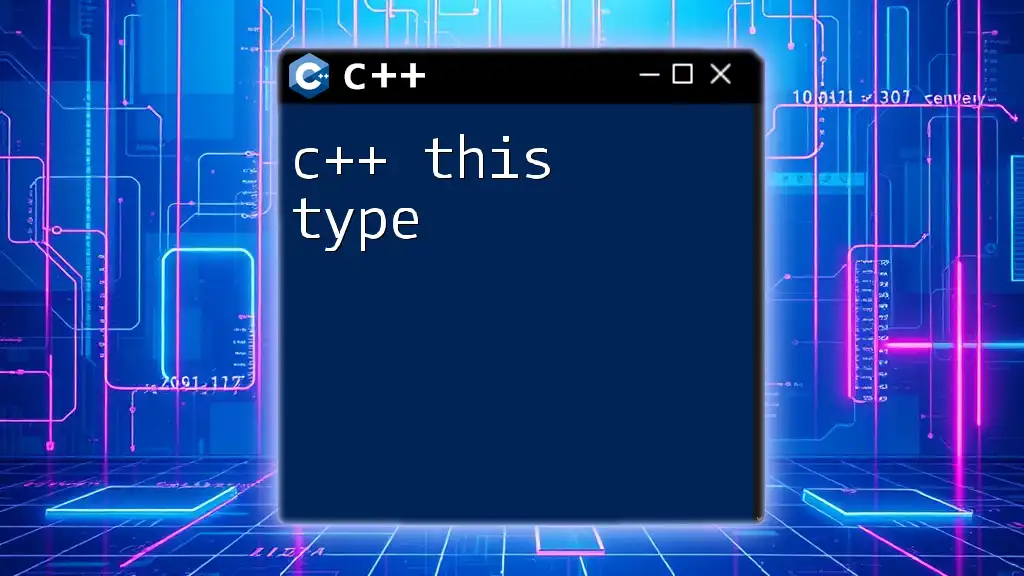
`this` Keyword in C++ and Const Member Functions
Understanding how `this` behaves in const member functions is equally important. When you declare a member function as const, the `this` pointer in that function points to a constant object:
class Container {
const int value;
public:
Container(int v) : value(v) {}
void display() const {
// 'this' is of type const Container*
const Container* constThis = this;
}
};
In the above example, any attempt to modify the member variables of `Container` through `this` will lead to a compile-time error, emphasizing the constness of the object.
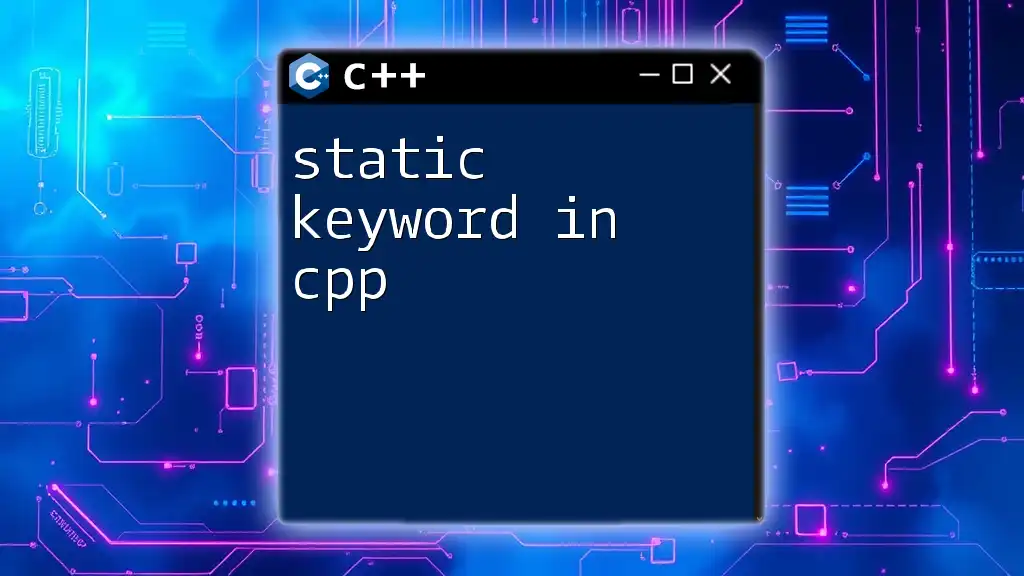
`this` Keyword in Inheritance
The Use of `this` in Derived Classes
When dealing with inheritance, `this` also plays an integral role. It can be used to refer to base class methods and attributes:
class Base {
public:
void show() { cout << "Display Base"; }
};
class Derived : public Base {
public:
void display() {
this->show(); // Calling base class method using 'this'
}
};
In this case, using `this->show()` explicitly makes it clear that you are calling a method from the base class.
Ambiguity in Base-Derived Class Relationships
In scenarios where you have methods in both the base and derived classes with the same name, `this` allows you to resolve this ambiguity effectively:
class Base {
public:
void Show() { cout << "Base"; }
};
class Derived : public Base {
public:
void Show() { cout << "Derived"; }
void display() {
this->Base::Show(); // Accessing base class Show()
}
};
Here, invoking `this->Base::Show()` clearly specifies that you want to call the show method from the base class rather than the derived class.
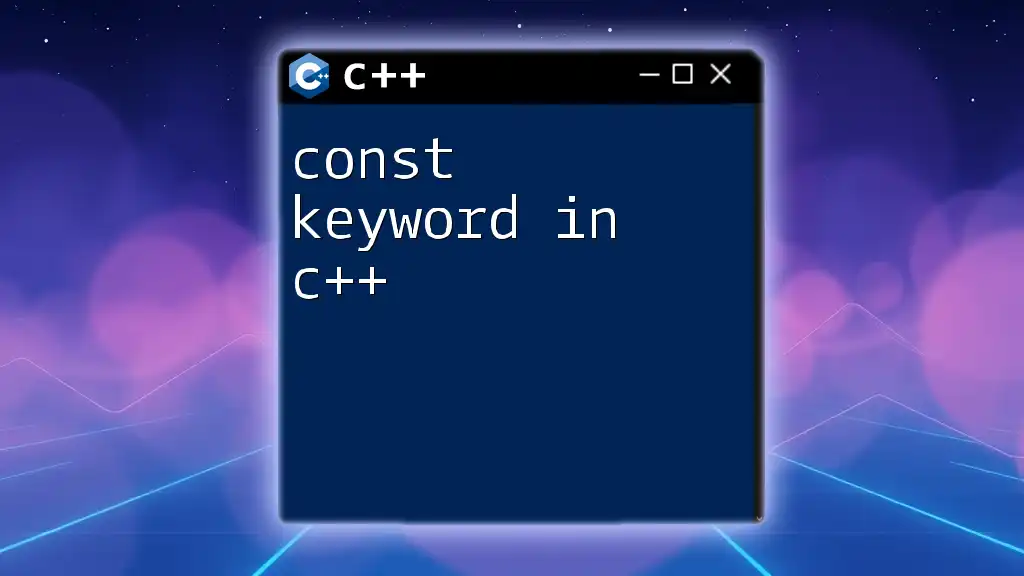
Best Practices When Using the `this` Keyword
When using the `this` keyword, here are some best practices to ensure proper usage:
- Be judicious about when to use `this`. It is generally encouraged to use it when necessary, especially in the case of variable name conflicts.
- Rely on `this` to improve clarity, but avoid overusing it in contexts where it isn't necessary. If there is no ambiguity, it's acceptable to omit it.
- Maintain consistency in your usage throughout your codebase. A uniform coding style helps other developers quickly understand your implementations.
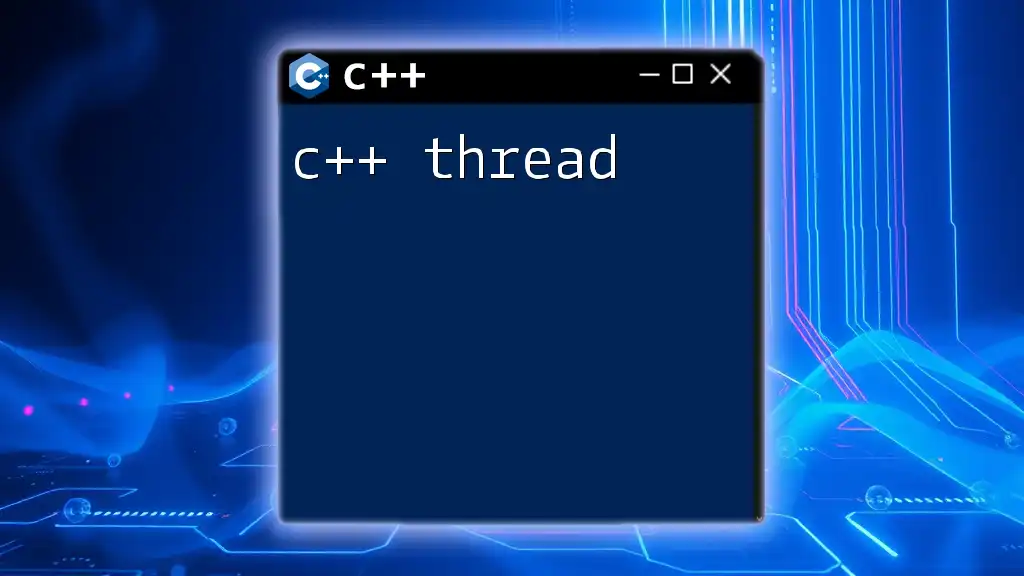
Common Pitfalls and FAQs about `this` in C++
Frequently Asked Questions
One common misconception about `this` is whether it can be null. The answer is that `this` can never be null when invoked for non-static member functions because it always points to an instance of the class. However, in the case of static member functions, `this` is not available.
Common Mistakes to Avoid
Avoid misusing `this` in constructors or destructors, as improper usage can lead to additional confusion, especially when calling other member functions or accessing attributes that have yet to be fully constructed or initialized. Additionally, overusing `this` when it's unnecessary may clutter your code and detract from its readability.
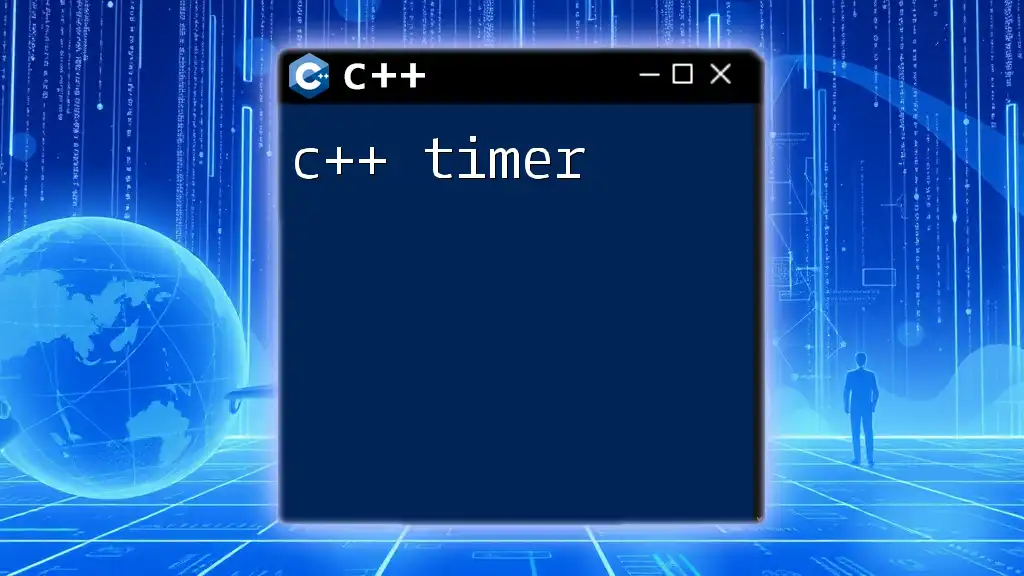
Conclusion
The c++ this keyword is a fundamental concept in C++ programming that helps manage object-oriented principles effectively. It not only aids in accessing member variables but also enhances code clarity and readability. By understanding how to use `this`, you can streamline your programming practices and improve the overall quality of your C++ code.
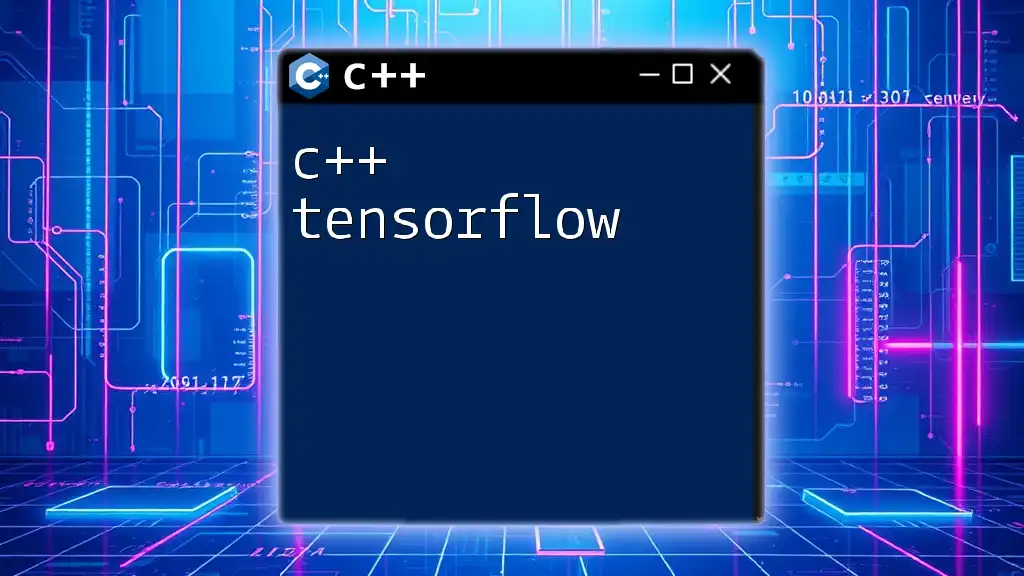
Additional Resources
For further reading, developers interested in the c++ this keyword can explore online tutorials and guides focusing on C++ object-oriented programming principles. Additionally, books that cover advanced topics in C++ can provide deeper insights into the nuances of using `this` and related concepts.
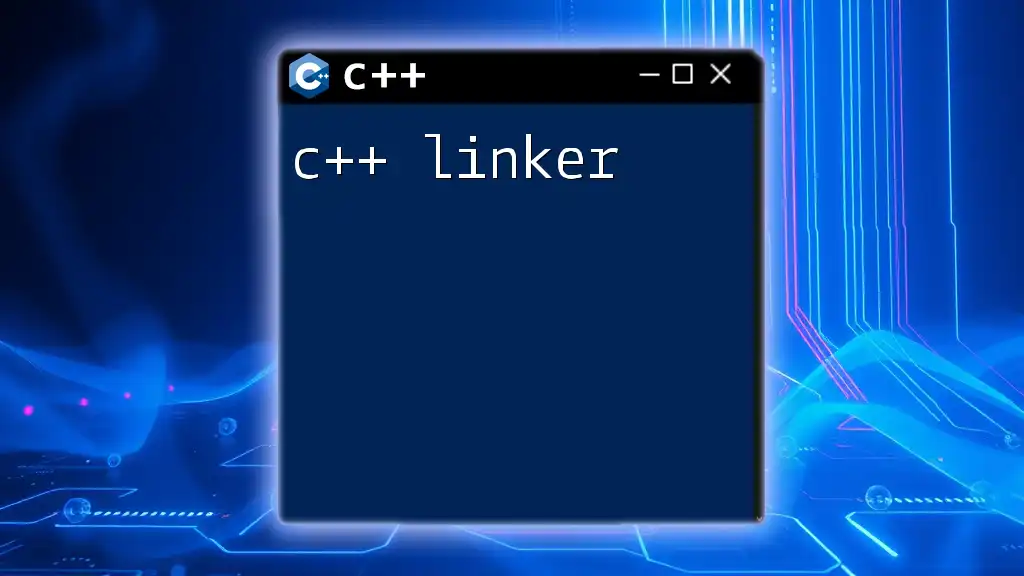
Call to Action
We encourage readers to share their experiences or challenges with the `this` keyword in their coding practices. Engaging with the community can provide valuable insights and enhance collective learning, so feel free to comment below with any thoughts or questions!