A `ListNode` in C++ is a basic structure used to represent a node in a linked list, typically containing data and a pointer to the next node.
Here's a simple code snippet demonstrating a `ListNode` structure:
struct ListNode {
int data; // Value of the node
ListNode* next; // Pointer to the next node in the list
ListNode(int val) : data(val), next(nullptr) {} // Constructor
};
Understanding ListNode
What is a ListNode?
A ListNode is a fundamental component in linked lists, which are versatile data structures used in various programming scenarios. Unlike arrays, which allocate a contiguous block of memory, a linked list utilizes nodes that can be scattered in memory. Each node in a linked list is composed of two parts: the data it holds and a pointer (or reference) to the next node in the sequence. This structure enables dynamic memory allocation and efficient element addition or removal compared to traditional arrays.
Properties of a ListNode
A basic ListNode consists of two properties:
- Data: This holds the actual value stored in the node (e.g., an integer, string, etc.).
- Next Pointer: This points to the subsequent node in the list or is set to `nullptr` if it's the last node.
Here is a code snippet demonstrating the basic structure of a ListNode:
struct ListNode {
int data; // Data stored in the node
ListNode* next; // Pointer to the next node
};
This simple definition forms the building block for more complex data structures.
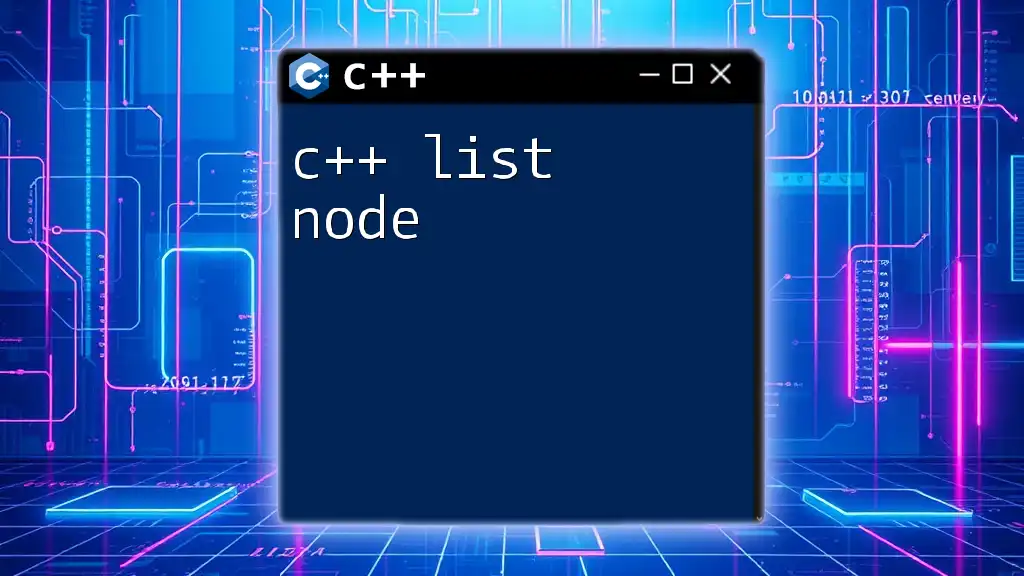
Implementing ListNode in C++
Creating a ListNode
Creating a ListNode involves initiating a new object of the ListNode structure and setting its initial values. This can be done as follows:
ListNode* newNode = new ListNode();
newNode->data = 10; // Set the data
newNode->next = nullptr; // Initialize the next pointer
Here, we allocate memory for a new ListNode, assign it a value, and ensure that the next pointer points to `nullptr`, indicating that it does not link to any other node yet.
Modifying ListNode
Modification of ListNodes typically involves changing the data it holds or adjusting its links. For instance, if we want to create a second ListNode and link it to the first, we can do the following:
ListNode* secondNode = new ListNode();
secondNode->data = 20;
newNode->next = secondNode; // Link the first node to the second
This operation effectively forms a chain of nodes, with `newNode` pointing to `secondNode`.
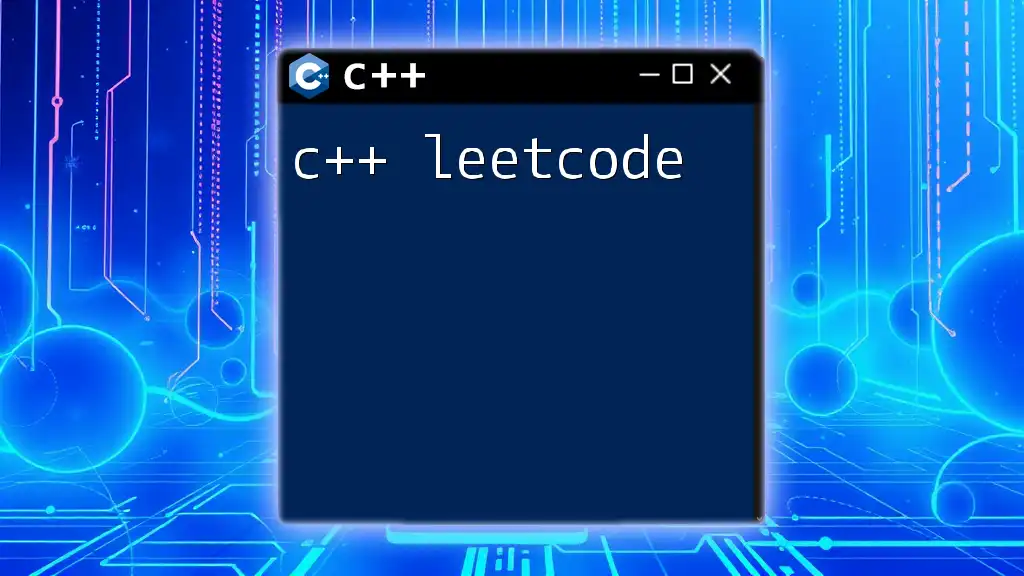
Using ListNode in Linked Lists
Singly Linked List
A singly linked list is a simple data structure where each node contains a value and a pointer to the next node, making it easy to traverse from the head to the end of the list. The following code demonstrates how to insert values into a singly linked list:
void insert(ListNode*& head, int data) {
ListNode* newNode = new ListNode();
newNode->data = data;
newNode->next = head; // Insert at the beginning
head = newNode;
}
In this example, we create a new node and insert it at the beginning of the list. As a result, the head of the list is updated to this new node.
Doubly Linked List
A doubly linked list is a more advanced structure where each node contains two pointers: one pointing to the next node and another pointing to the previous node. This allows traversal in both directions. Here’s how you might define a doubly linked node:
struct DoublyListNode {
int data;
DoublyListNode* next; // Pointer to the next node
DoublyListNode* prev; // Pointer to the previous node
};
The inclusion of the `prev` pointer allows for more flexible navigation through the list, a critical feature in many applications.
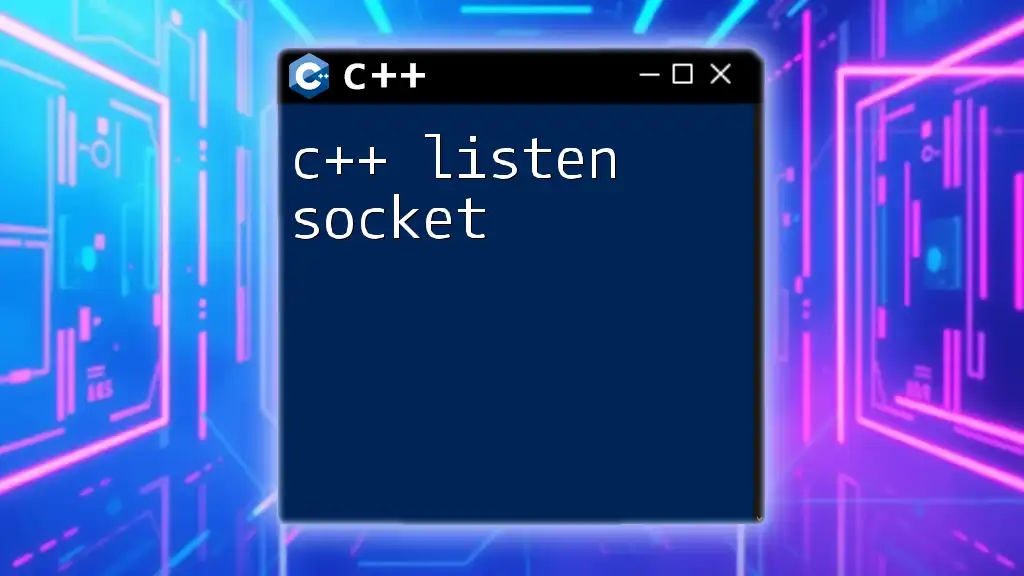
Operations on ListNode
Traversing a Linked List
Traversing a linked list means visiting each node in sequence, which can be efficiently accomplished with a loop. Here is a simple traversal implementation:
void traverse(ListNode* head) {
ListNode* current = head;
while (current != nullptr) {
std::cout << current->data << " -> ";
current = current->next;
}
std::cout << "nullptr" << std::endl;
}
This function iterates through the list, printing the data in each node until it reaches a node that points to `nullptr`, indicating the end of the list.
Searching for a Value
Searching through a linked list requires iterating through each node until the desired value is found or the end of the list is reached. Here’s a code example that demonstrates this process:
ListNode* search(ListNode* head, int value) {
ListNode* current = head;
while (current != nullptr) {
if (current->data == value) {
return current; // Found the value
}
current = current->next;
}
return nullptr; // Not found
}
In this example, the function returns a pointer to the node that contains the searched value or `nullptr` if it is not found.
Deleting a Node
Deleting a node from a linked list involves several steps: locating the node, adjusting pointers, and freeing the memory. Here’s an implementation for deleting a node by its value:
void deleteNode(ListNode*& head, int value) {
ListNode* current = head;
ListNode* previous = nullptr;
while (current != nullptr && current->data != value) {
previous = current;
current = current->next;
}
if (current == nullptr) return; // Value not found
if (previous == nullptr) {
head = head->next; // Node to delete is the head
} else {
previous->next = current->next; // Bypass the node to delete
}
delete current; // Free the memory
}
This function looks for the node to delete, modifying pointers accordingly until the node is successfully removed from the linked list.
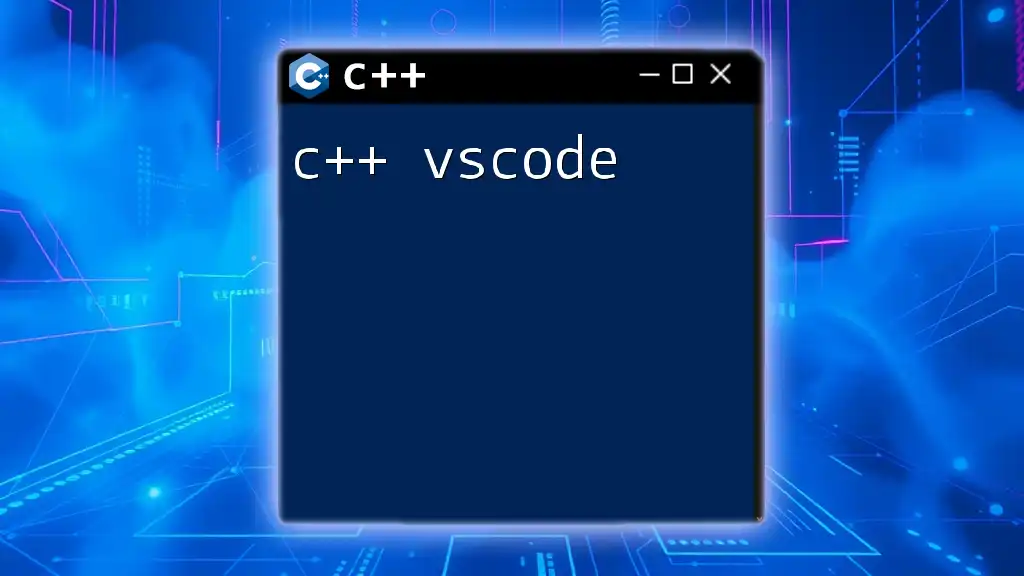
Common Use Cases for ListNode
Implementing Stacks and Queues
ListNodes can be used to implement stacks and queues, which are fundamental data structures for data management and processing tasks. For instance, a stack can be implemented as a singly linked list where insertion and deletion occur at the head. A queue can similarly be implemented, utilizing both ends of a linked list (head for removal and tail for insertion) to maintain the order of elements.
Real-world Applications of Linked Lists
Linked lists, built from ListNode structures, have myriad applications. They are commonly used for:
- Dynamic memory management: Where the size of the data structure isn’t known in advance.
- Implementing complex data types: Such as adjacency lists in graphs or hash tables with separate chaining.
- Efficient insertions/deletions: Where data structure elements frequently change during execution.
Understanding and leveraging the power of ListNode in C++ can significantly enhance your coding efficiency and capability to solve complex problems.