C++ LeetCode refers to solving competitive programming problems using C++ in an efficient and effective manner, often leveraging the Standard Template Library (STL) for improved performance.
Here’s an example of a simple C++ function to check if a string is a palindrome:
#include <iostream>
#include <algorithm>
bool isPalindrome(const std::string& str) {
std::string reversedStr = str;
std::reverse(reversedStr.begin(), reversedStr.end());
return str == reversedStr;
}
int main() {
std::string test = "racecar";
std::cout << (isPalindrome(test) ? "Palindrome!" : "Not a palindrome.") << std::endl;
return 0;
}
What is LeetCode?
LeetCode is an online platform designed for coding challenges and technical interview preparation. It offers a vast collection of problems that help users improve their coding skills and algorithmic thinking. By solving a variety of tasks, aspiring programmers can sharpen their skills, making them more competitive in job interviews, particularly in the tech industry.
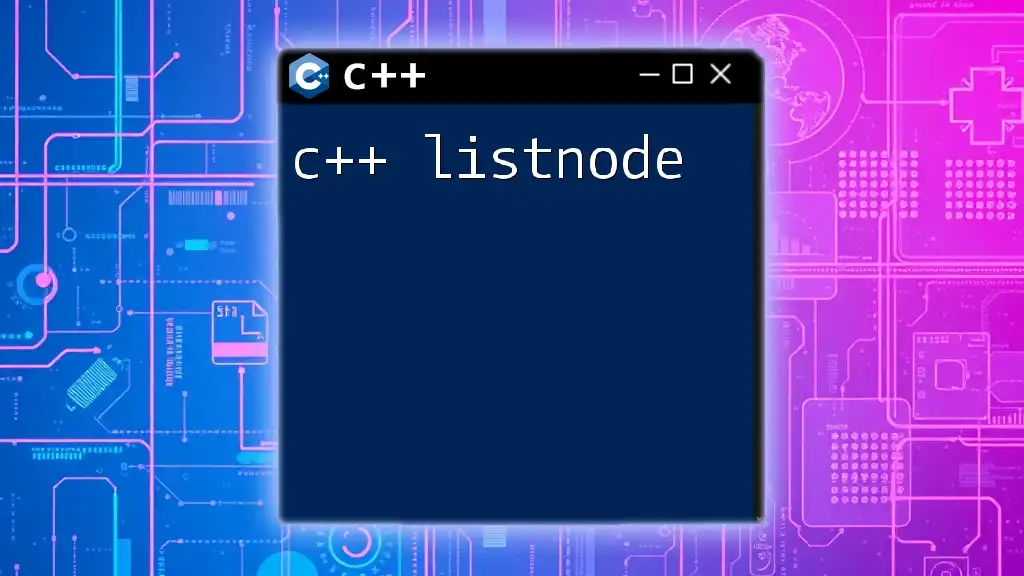
Why Choose C++ for LeetCode?
C++ is a powerful programming language favored in competitive programming due to its performance efficiency and control over system resources. Using C++ for LeetCode challenges provides several advantages:
- Performance: C++ is faster than many other high-level languages, which is crucial for time-sensitive problems.
- Robust Standard Template Library (STL): The STL offers a set of useful algorithms and data structures, which can greatly simplify the coding process.
- Memory Management: C++ allows fine-grained control over memory allocation and deallocation, providing an edge in managing resources effectively.
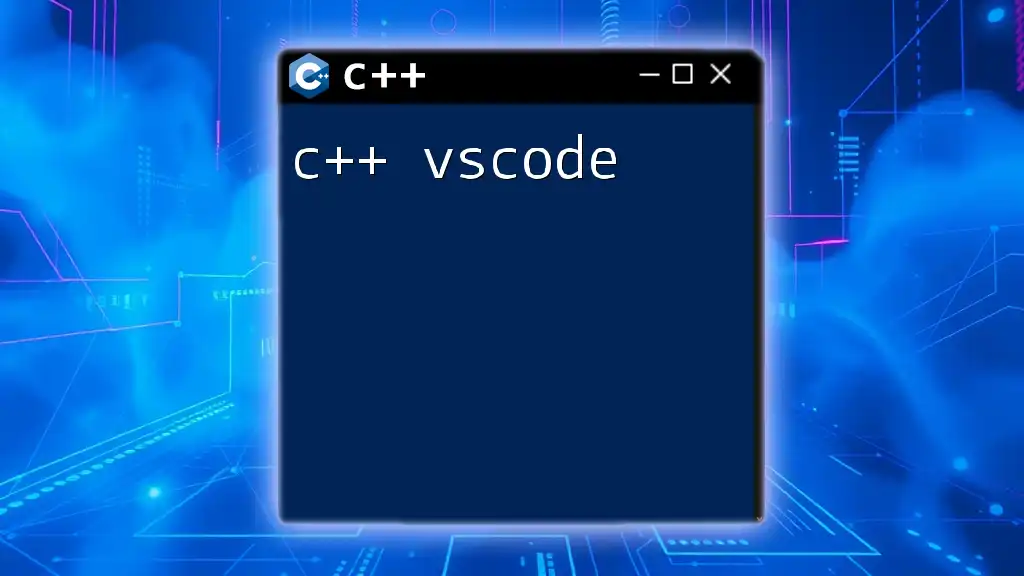
Getting Started with C++ on LeetCode
Setting Up Your Environment
To start coding for C++ on LeetCode, you'll need a development environment. Here are some recommended IDEs:
- Visual Studio: A popular choice for Windows users that provides a rich set of development tools.
- Code::Blocks: A free, open-source IDE that is user-friendly and geared towards C++ development.
- JetBrains CLion: A powerful IDE that comes with intelligent code assistance.
After installing your chosen IDE, ensure that you set it up for C++. This usually involves configuring the compiler and starting a new C++ project.
Understanding C++ Syntax and Basics
Before diving into LeetCode problems, it's essential to grasp fundamental C++ syntax and structures. Understanding variables, data types, control structures, and functions is crucial:
-
Variables and Data Types: C++ supports several data types, including integers, floats, characters, and strings.
-
Control Structures: Familiarity with conditional statements (`if`, `switch`) and loops (`for`, `while`) is vital.
-
Functions and Scope: Understanding how to declare functions and manage variable scope will help you write organized code.
Here’s a simple C++ program to demonstrate these basics:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, LeetCode!" << endl;
return 0;
}
In this example, we include the iostream library, which allows us to perform input and output operations. The `main` function is where program execution begins.
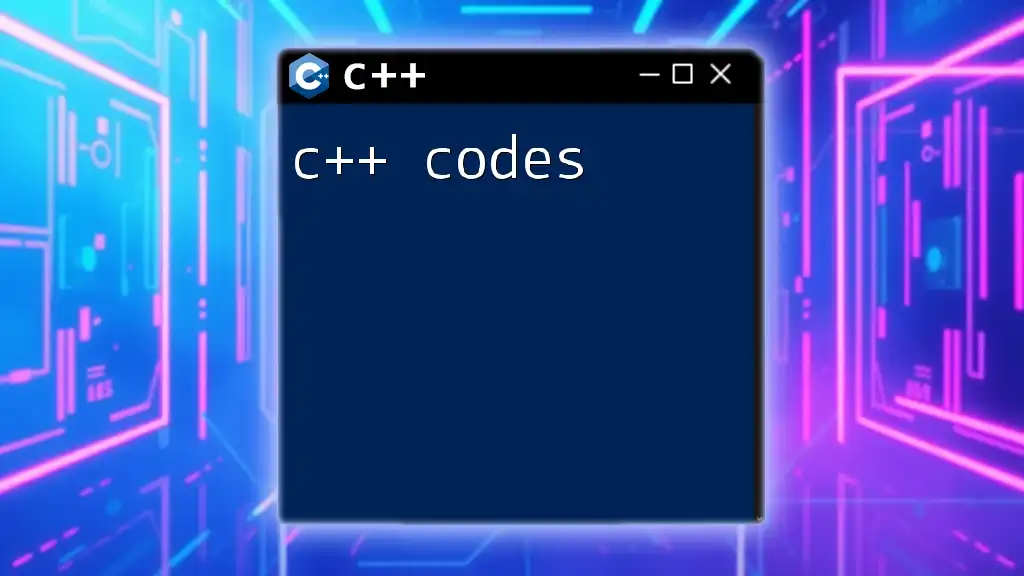
Types of Problems on LeetCode
On LeetCode, you'll encounter various categories of problems. Some common ones include:
-
Arrays and Strings: These include basic operations like searching, sorting, and manipulating elements.
-
Linked Lists: Tasks that involve the manipulation of data structures where elements are connected via pointers.
-
Trees and Graphs: Problems related to hierarchical and network-based structures requiring traversal and searching techniques.
-
Dynamic Programming: Challenges that involve breaking down complex problems into simpler subproblems.
-
Backtracking: Problems that require finding an optimal solution by exploring possible configurations.
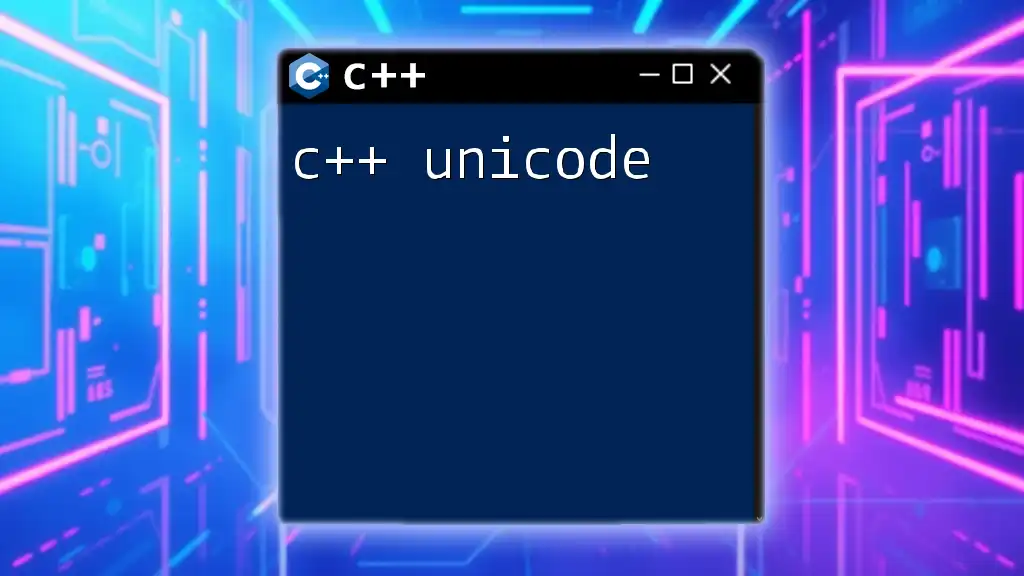
Problem-Solving Strategies in C++
Understanding Problem Statements
The key to solving any LeetCode problem is a thorough analysis of the problem statement. Extract essential details like input formats, expected outputs, and constraints. Identifying edge cases can often guide your approach.
Breaking Down the Problem
Breaking down problems into manageable parts is crucial. For this, you can employ the following techniques:
-
Top-down Approach: Start solving the main problem directly while using recursive methods to tackle subproblems.
-
Bottom-up Approach: Solve smaller subproblems first and use those solutions to build up to the desired goal.
Using pseudo-code can also be invaluable in planning your approach before diving into the actual coding phase.
Leverage C++ Standard Library
The Standard Template Library (STL) in C++ includes various components like vectors, sets, and maps, which can significantly simplify your tasks:
For example, consider using vectors for a simple problem:
#include <iostream>
#include <vector>
using namespace std;
vector<int> getEvenNumbers(const vector<int>& nums) {
vector<int> evenNumbers;
for (int num : nums) {
if (num % 2 == 0) {
evenNumbers.push_back(num);
}
}
return evenNumbers;
}
In this snippet, we iterate through a vector of integers to extract even numbers, showcasing how easy it is to manipulate arrays using the STL.
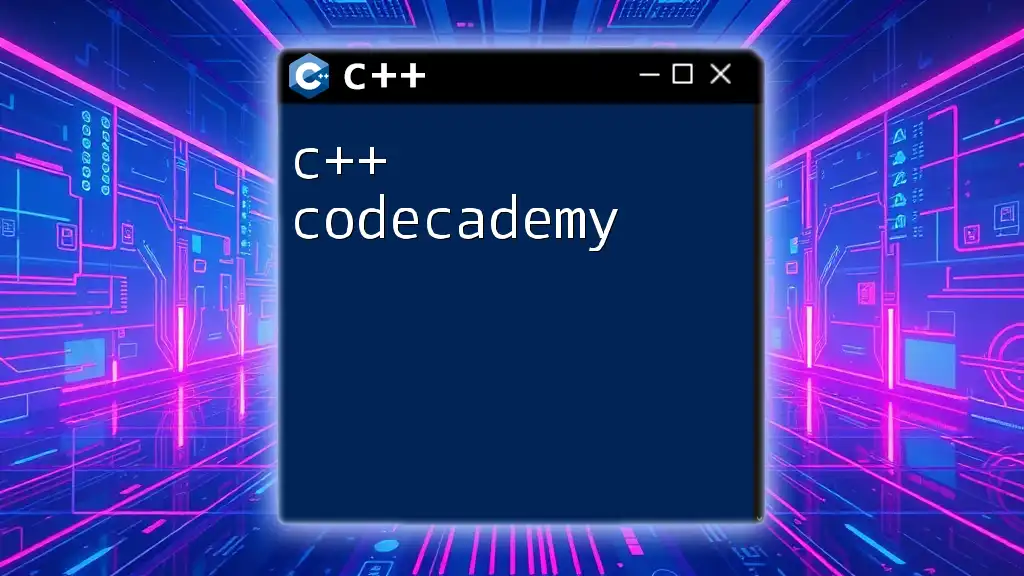
Example Problems and Solutions
Example 1: Two Sum Problem
The Two Sum problem is a classic example that asks for the indices of two numbers in an array that add up to a specific target.
Problem Statement: Given an array of integers and a target integer, return the indices of the two numbers.
Solution Breakdown:
- Use a hash map to store the visited numbers along with their indices.
- For each number, calculate the complement (target - current number) and check if it's already in the map.
Here's the C++ implementation:
#include <iostream>
#include <unordered_map>
#include <vector>
using namespace std;
vector<int> twoSum(vector<int>& nums, int target) {
unordered_map<int, int> map;
for (int i = 0; i < nums.size(); i++) {
int complement = target - nums[i];
if (map.find(complement) != map.end()) {
return {map[complement], i};
}
map[nums[i]] = i;
}
return {};
}
Example 2: Maximum Subarray Problem
The Maximum Subarray problem involves finding the contiguous subarray within a one-dimensional array that has the largest sum.
Overview: The best approach to solve this problem is Kadane’s Algorithm. The idea is to maintain two variables that store the maximum sum and the current sum at every index.
Here is how you can implement this in C++:
#include <iostream>
#include <vector>
using namespace std;
int maxSubArray(vector<int>& nums) {
int maxCurrent = nums[0], maxGlobal = nums[0];
for (int i = 1; i < nums.size(); i++) {
maxCurrent = max(nums[i], maxCurrent + nums[i]);
if (maxCurrent > maxGlobal) {
maxGlobal = maxCurrent;
}
}
return maxGlobal;
}
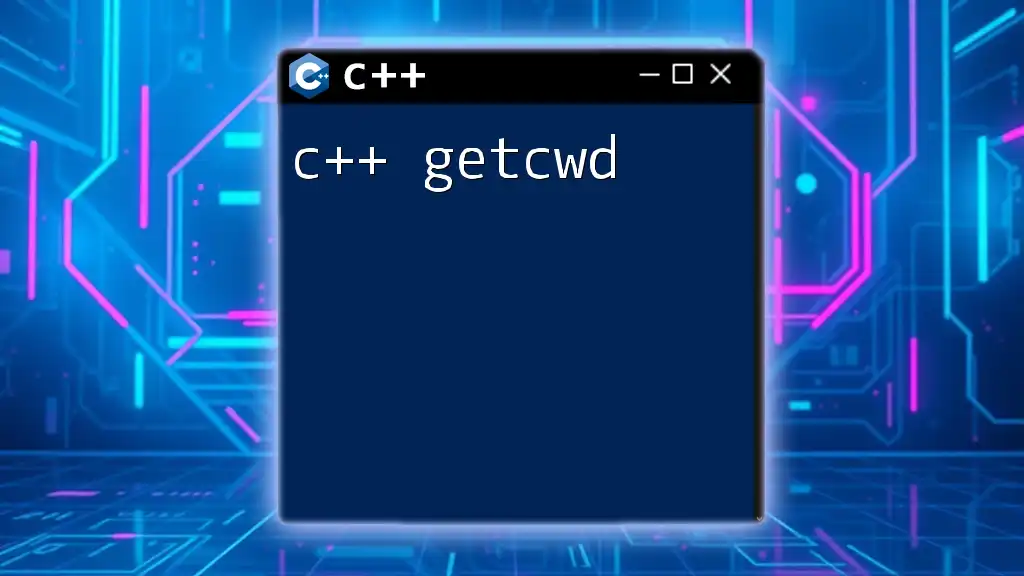
Best Practices for Solving C++ LeetCode Problems
Code Readability and Maintenance
Writing clean and maintainable code is crucial, not only for your understanding but also for others who may read it. Some practices include:
-
Use Meaningful Variable Names: Clearly convey the purpose of your variables through their names.
-
Comment Wisely: Annotations should clarify complex logic, helping others (and yourself) understand your code better in future.
Testing Your Solutions
Effective testing is essential for verifying the correctness of your solutions. In C++, you can create unit tests using frameworks like Google Test, allowing you to automate the testing process.
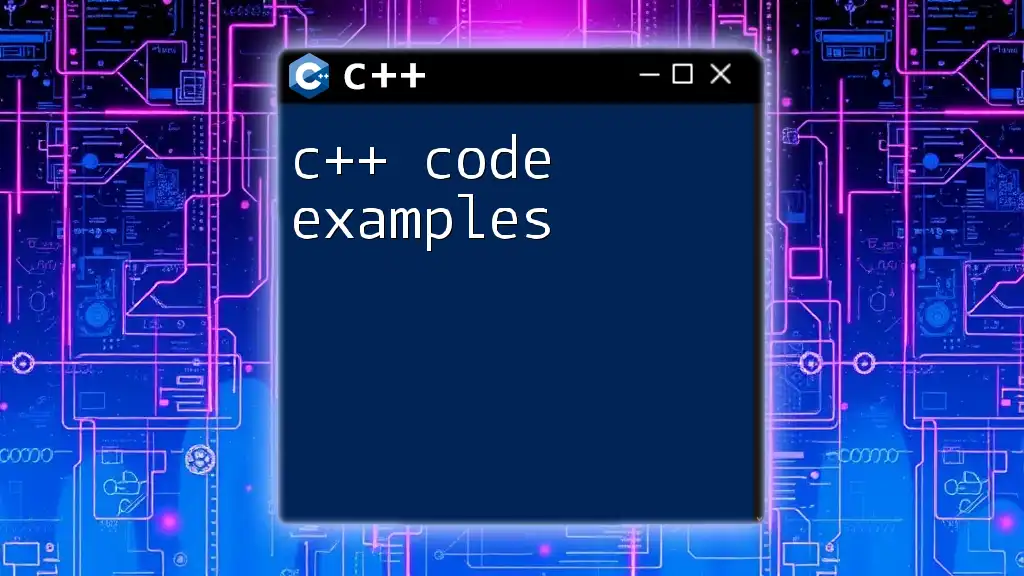
Resources for C++ LeetCode Practice
Books and Online Courses
To enhance your C++ skills and algorithm understanding, consider these resources:
- Books: Titles like "Effective C++" by Scott Meyers are excellent for learning best practices.
- Online Courses: Platforms like Coursera or Udemy offer courses targeting C++ enhancements and algorithm design.
Community and Forums
Engagement with the C++ community can provide invaluable support. Joining forums, participating in discussions, and seeking help on platforms like Stack Overflow can hugely benefit you.
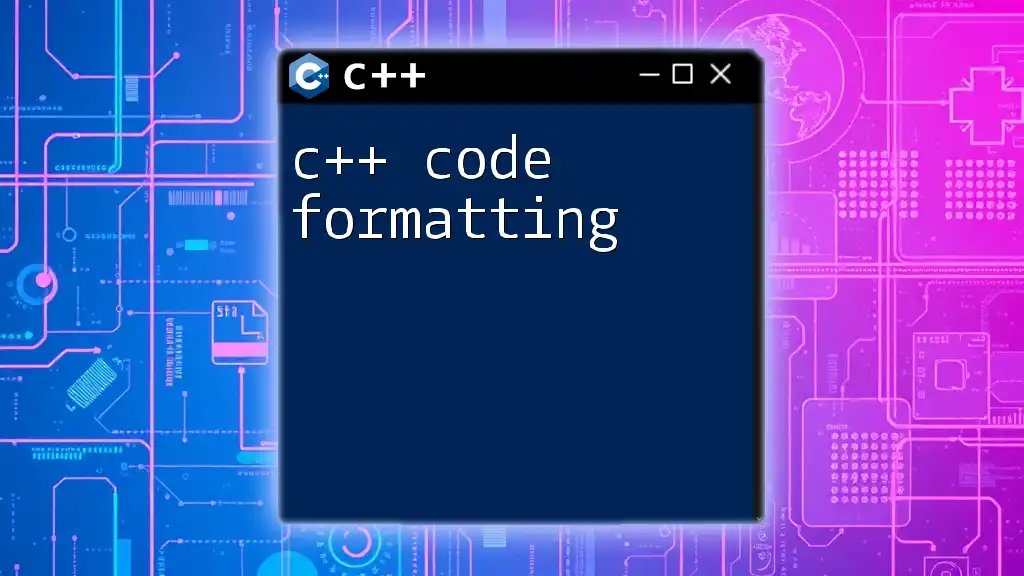
Conclusion
Using C++ on LeetCode is an excellent way to strengthen your coding skills and prepare for technical interviews. With consistent practice and the application of best coding practices, you can enhance your problem-solving abilities and boost your coding confidence.
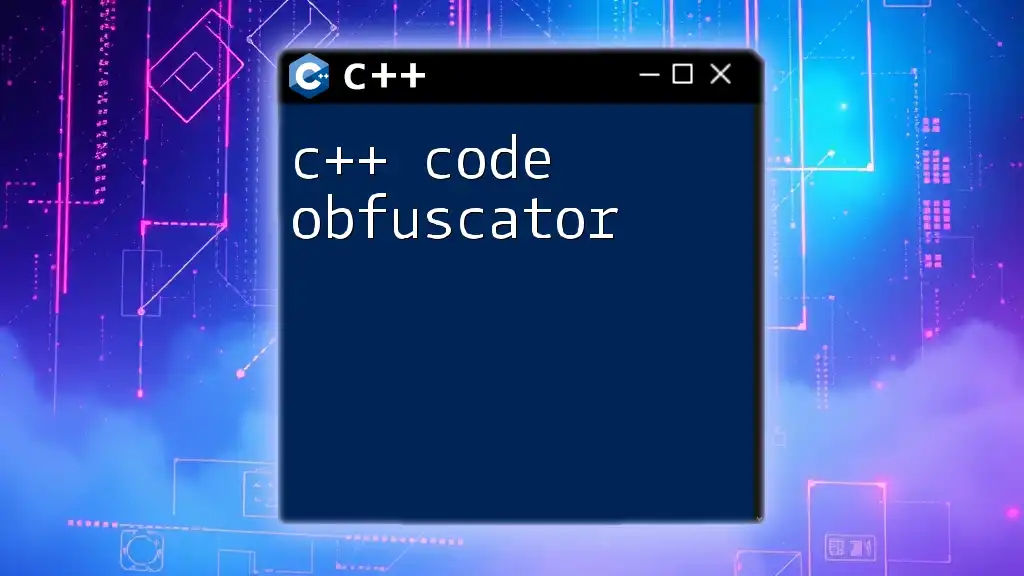
Start Your LeetCode Journey Today
Dive into LeetCode, embrace the challenges of coding with C++, and consider utilizing our company’s resources for mastering C++ commands quickly and effectively. With dedication and the right strategy, you can excel in your coding journey!