The `getcwd` function in C++ retrieves the current working directory of the calling process, returning it as a string.
#include <iostream>
#include <unistd.h>
int main() {
char cwd[256];
if (getcwd(cwd, sizeof(cwd)) != nullptr) {
std::cout << "Current working directory: " << cwd << std::endl;
} else {
perror("getcwd() error");
}
return 0;
}
What is getcwd in C++?
The `getcwd` function is a crucial part of C++ that allows developers to retrieve the current working directory of a process. This function stands for "get current working directory" and is often used in file management routines to ensure that file paths are constructed correctly based on the current context of the application.
While there are other directory-related functions like `chdir` (change directory) and `opendir` (open directory), `getcwd` plays a unique role by providing a means to inquire about the present location of the process within the filesystem.
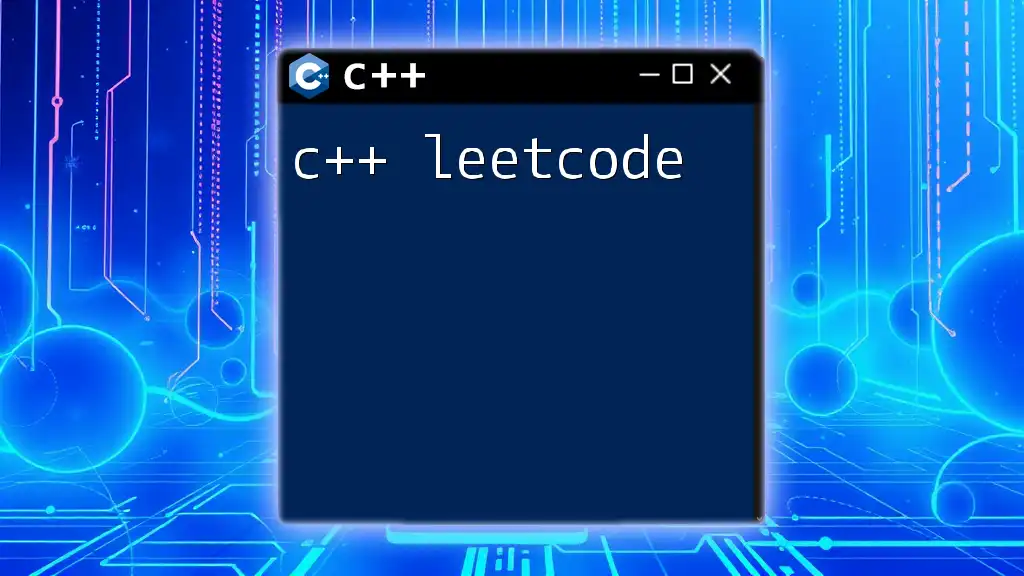
How to Use getcwd in C++
Syntax of getcwd
The syntax of the `getcwd` function is as follows:
char* getcwd(char* buf, size_t size);
- First Parameter: `buf` – A pointer to a character array that will store the current working directory.
- Second Parameter: `size` – The size of the buffer. It determines how much space is available in `buf` for storing the path.
Including Necessary Headers
To use `getcwd`, it's essential to include the appropriate header files:
- For UNIX-like systems, you must include `<unistd.h>`.
- For Windows systems, include `<direct.h>`.
Both headers contain the necessary declarations needed to call the `getcwd` function safely.
Example Code Snippet
Here is a simple example demonstrating the use of `getcwd`:
#include <iostream>
#include <unistd.h>
int main() {
char buffer[256];
char* cwd = getcwd(buffer, sizeof(buffer));
if (cwd) {
std::cout << "Current working directory: " << cwd << std::endl;
} else {
perror("getcwd() error");
}
return 0;
}
This code snippet does the following:
- Initialization of the Buffer: It prepares a character array `buffer` to hold the current working directory path.
- Function Call to getcwd: It invokes `getcwd` and stores the result in `cwd`.
- Error Handling: If `getcwd` returns `nullptr`, it indicates an error, and the code uses `perror` to display a relevant error message.
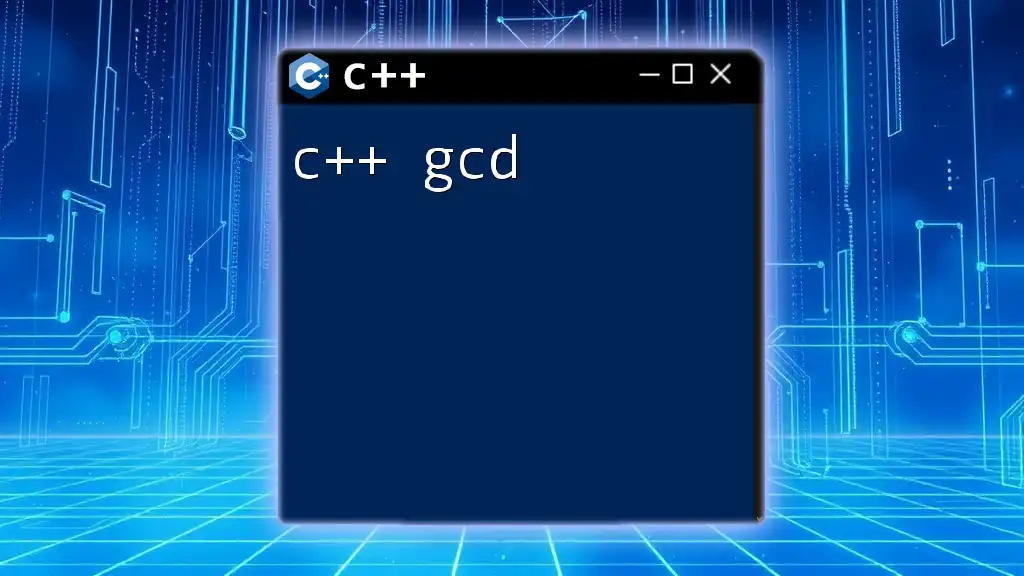
Detailed Breakdown of getcwd Parameters
First Parameter: buffer
The `buffer` acts as the storage location for the current working directory. It's critical to choose an appropriate buffer size to ensure that it can accommodate the full path. If the buffer runs out of space, `getcwd` will return `nullptr`, potentially leading to a loss of information and debugging headaches.
Second Parameter: size
The `size` is an important factor that dictates how much data can be safely written to the buffer. When this size is too small, not only can it prevent the correct path from being returned, but it can also lead to undefined behavior in your application if you attempt to write more data than the buffer can hold.
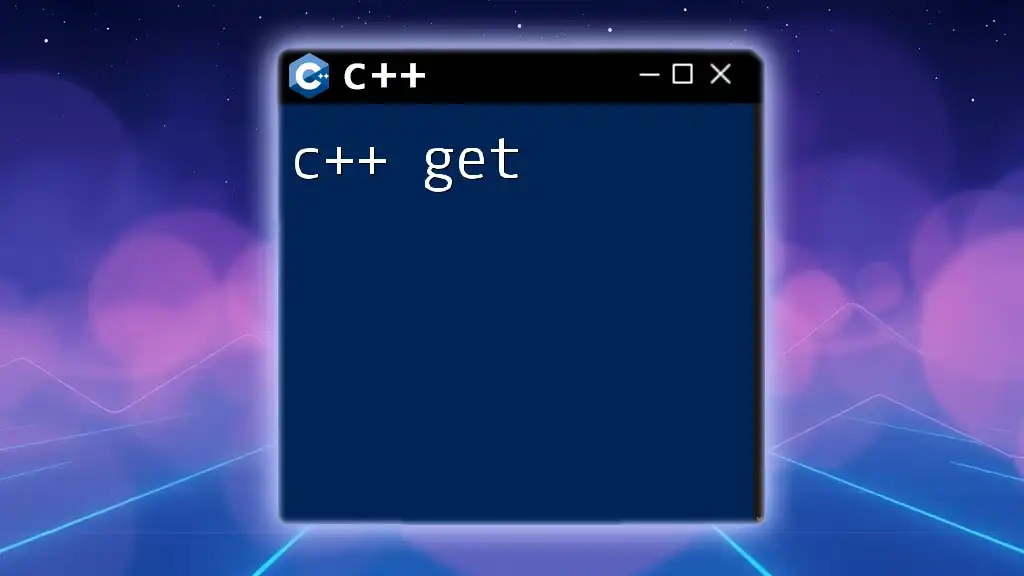
Handling Errors with getcwd
Using `getcwd` may present various errors that developers need to consider. Common errors include insufficient buffer size, invalid directory paths, or other system-level issues such as permission problems.
Often, you'll want to implement error handling to make your application robust. By checking the return value of `getcwd`, you can diagnose issues effectively:
if (getcwd(buffer, sizeof(buffer)) == nullptr) {
std::cerr << "Error Getting Current Working Directory: " << strerror(errno) << std::endl;
}
In this code, you check if `getcwd` returns `nullptr` and, if so, you utilize `strerror(errno)` to output the specific error associated with the failure.
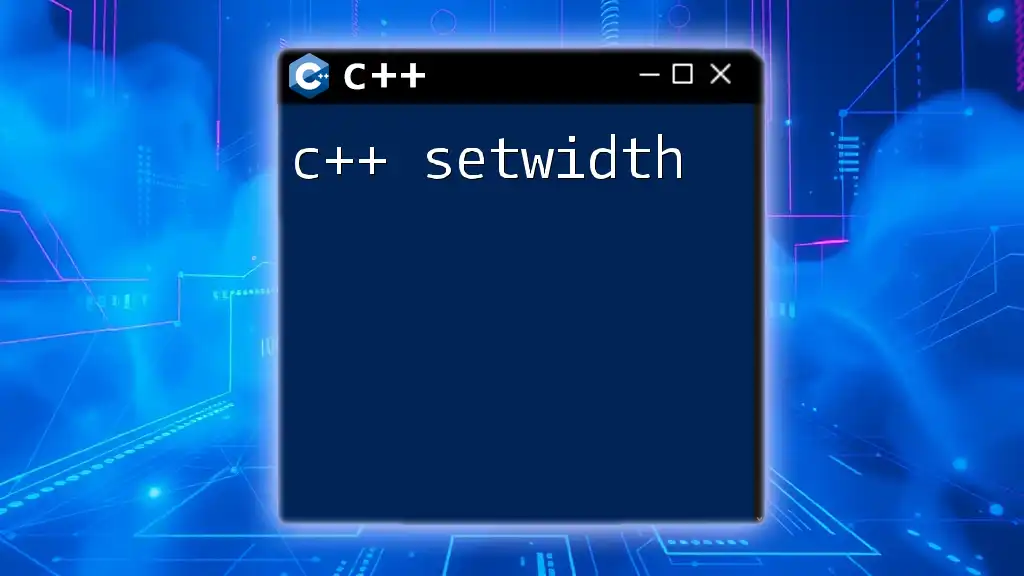
Cross-Platform Considerations
When using `getcwd`, it's important to recognize that implementations may vary between operating systems. The function works seamlessly on UNIX-like systems, including Linux and macOS, but may face nuances when used on Windows.
For instance, the path formats and directory structures differ, which can introduce compatibility issues. Testing your code on various operating systems ensures that your applications remain functional and reliable across platforms.
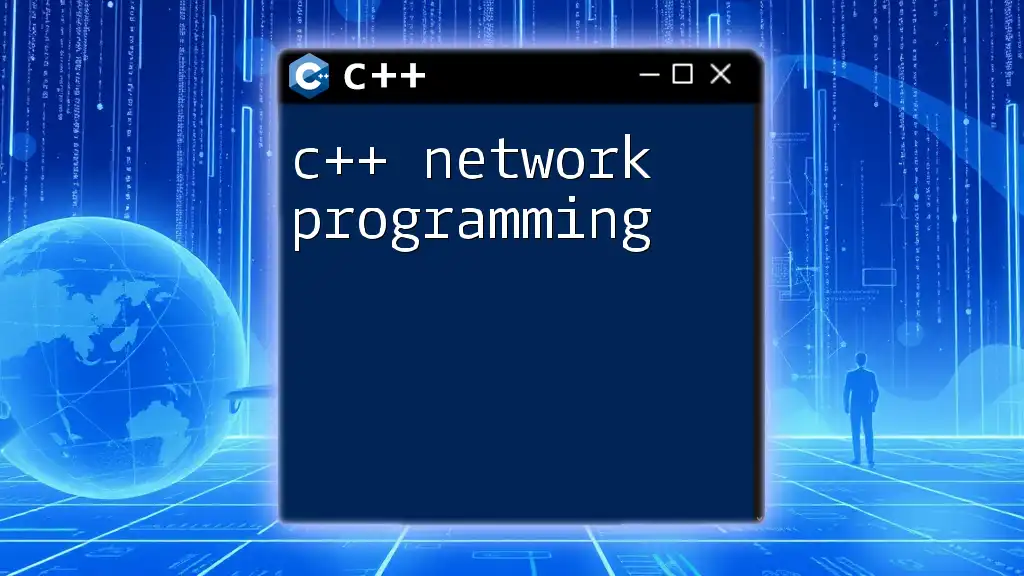
Using getcwd with Other File Operations
Combining getcwd with file I/O operations
One of the significant advantages of `getcwd` is that it allows developers to construct absolute paths dynamically. This capability is particularly useful when you need to access files located in the same directory as your executable or script.
For example:
std::string fullPath = std::string(cwd) + "/example.txt";
In this case, you're concatenating the current working directory with a filename to obtain the full path necessary for file operations.
Practical Use Cases
Knowing the current working directory is especially crucial when developing command-line applications, file managers, or tools that manipulate files within the filesystem. It enhances the user experience by allowing actions that are contextually aware of the user's present location.
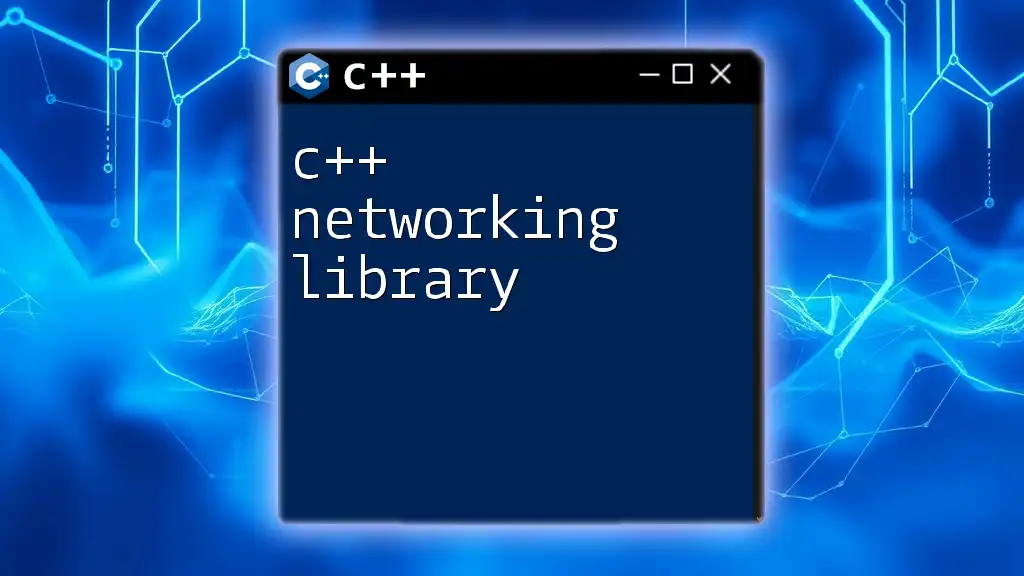
Best Practices for Using getcwd
When working with `getcwd`, keep these best practices in mind to ensure safe and effective use:
- Buffer Size: Always allocate an adequately sized buffer to minimize the risk of overflow.
- Error Handling: Implement robust error handling to capture and respond to potential issues effectively.
- Cross-Platform Testing: Ensure your code runs correctly on various systems to avoid compatibility problems in file path handling.
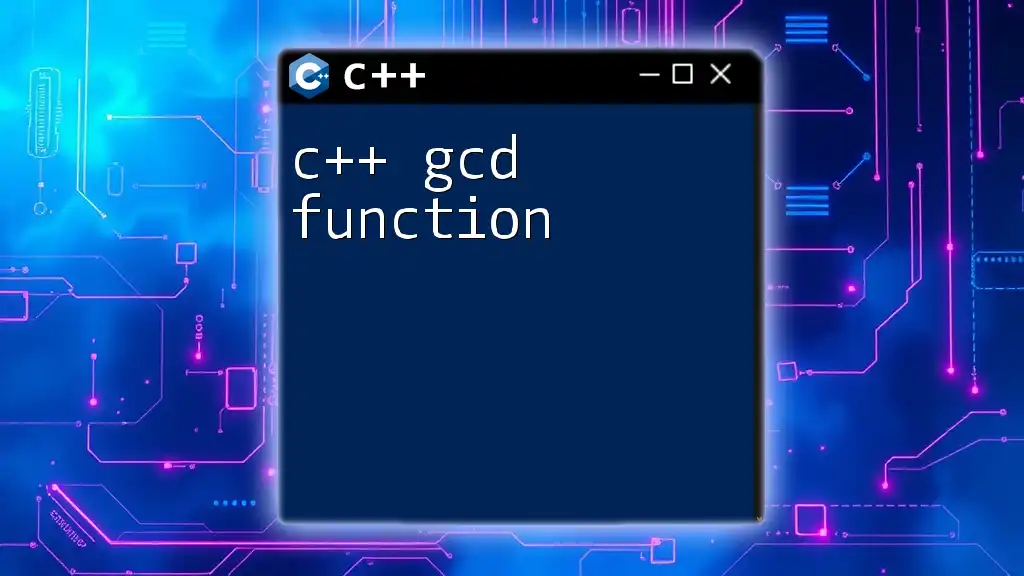
Conclusion
The `c++ getcwd` function is a powerful tool for managing current directories and file paths in your applications. It's essential for creating context-aware file manipulation processes, enhancing overall user experience. Make sure to practice with the examples provided and integrate `getcwd` into your projects!
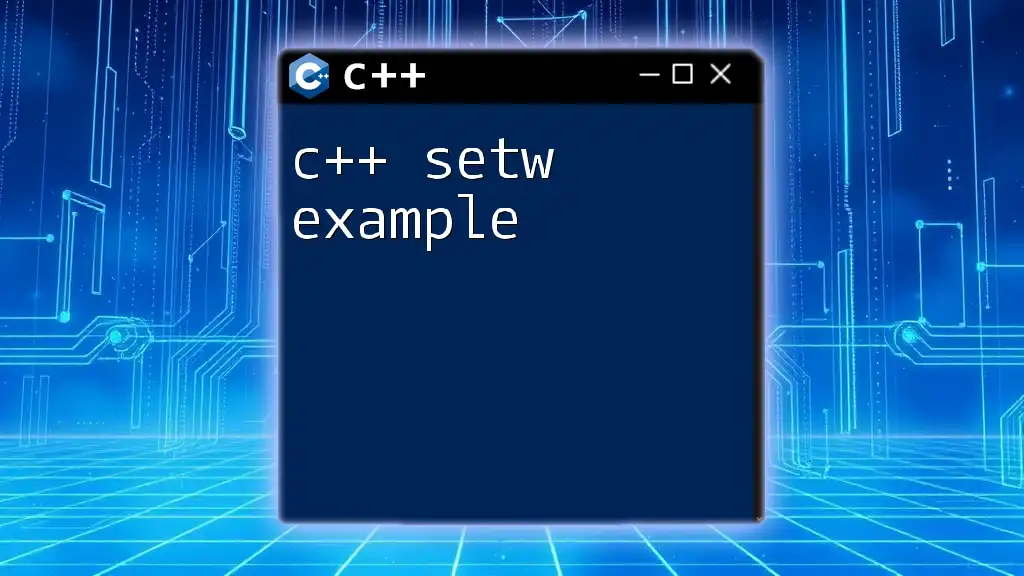
Additional Resources
For further exploration, consider reviewing official documentation, taking C++ programming courses, and participating in coding communities to deepen your understanding and enhance your skills.
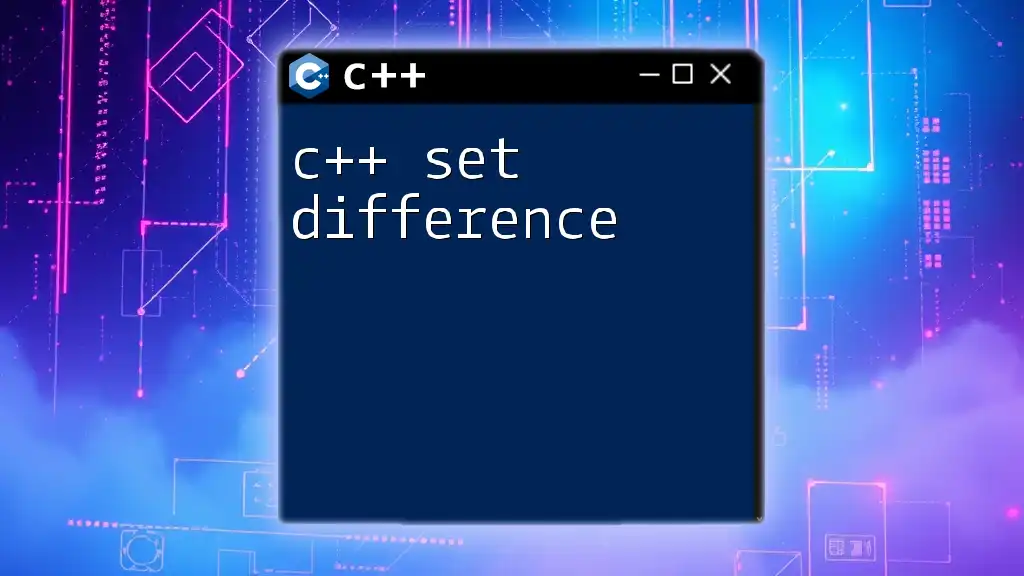
Call to Action
Now that you understand how to use `getcwd` effectively, try integrating it into your current projects. Share your experiences or any unique use cases in the comments below or on your social media, and let's learn from each other!