`decltype` in C++ is an operator that queries the type of an expression at compile time, allowing you to declare variables with the same type as the expression without explicitly specifying it.
Here’s a code snippet demonstrating its usage:
#include <iostream>
int main() {
int a = 5;
decltype(a) b = 10; // b is of type int, same as a
std::cout << "b: " << b << std::endl; // Output: b: 10
return 0;
}
What is decltype?
decltype is a powerful feature in C++ that allows developers to query the type of an expression at compile time. It helps in determining the type of a variable or function return value based on its initialization or context, which can significantly simplify type declarations and enhance code readability.
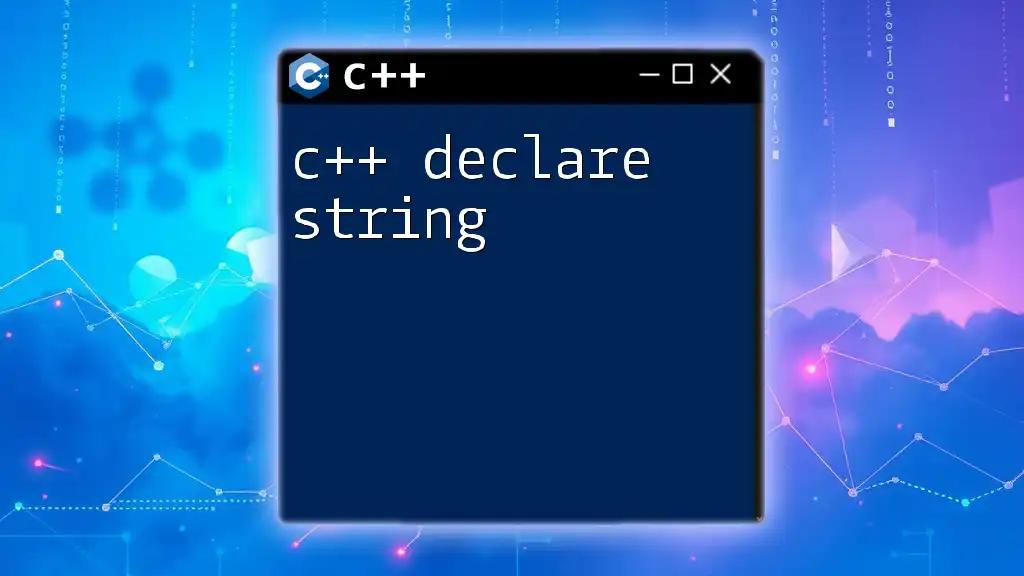
Why Use decltype in C++?
Utilizing decltype offers several advantages:
- Type Safety: It ensures that the type of the variable matches the type of the expression it is derived from, thereby reducing errors in type mismatches.
- Convenience: You can create variables without needing to manually specify their types, which is particularly useful in complex scenarios such as template programming or when using types returned from complex expressions.
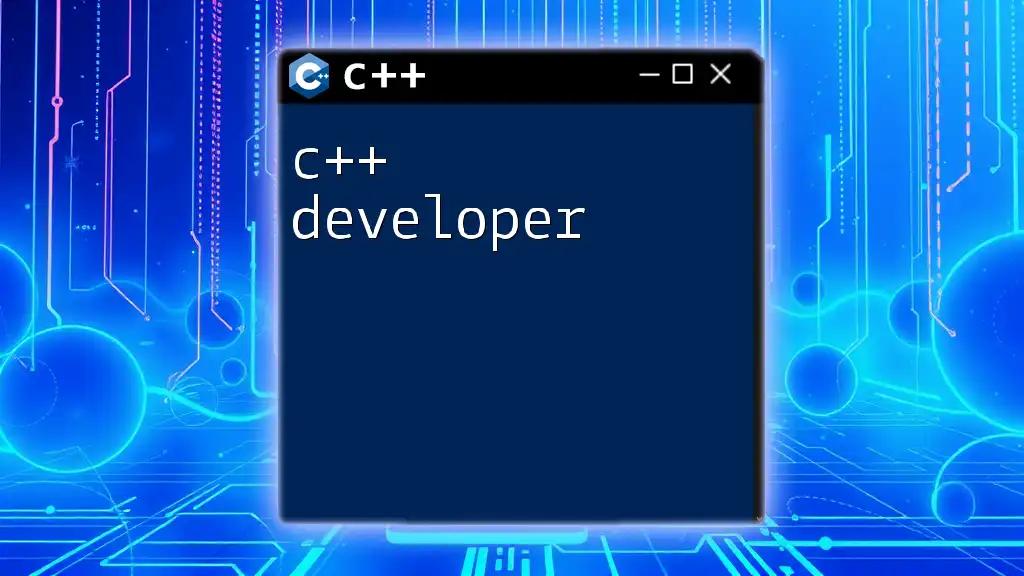
Basic Syntax of decltype
The basic syntax is straightforward:
decltype(expression)
By passing an expression inside the parentheses, decltype evaluates and returns its type.
Example of Simple Usage
Here’s a simple example showcasing how decltype deduces the type from a variable:
int a = 42;
decltype(a) b = 10; // b is of type int
In this case, the variable `b` will have the same type as `a`, which is `int`.

How decltype Works
Type Determination with decltype
The ability of decltype to deduce types based on expressions is one of its most compelling features. This is especially useful when dealing with functions where the return type might be difficult to define explicitly.
Examples of Type Deduction
Variable Declaration
Consider the following examples that demonstrate how decltype simplifies variable type declarations:
double pi = 3.14;
decltype(pi) circumference = 2 * pi * 5; // circumference is of type double
In this code snippet, `circumference` is automatically assigned the type `double` based on the type of `pi`.
Function Pointer Types
Here’s how decltype works with function pointers, illustrating its effectiveness in defining types succinctly:
void process(int);
decltype(&process) funcPtr = &process; // funcPtr is a pointer to a function taking int
By using decltype, we directly deduce the type of `funcPtr` from the function `process`, which leads to cleaner code.
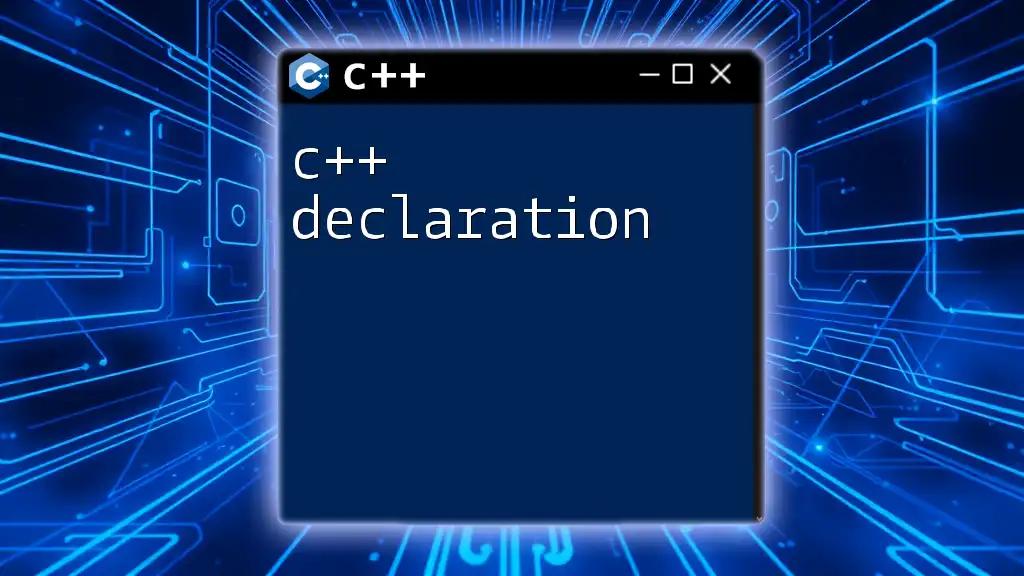
Advanced Usage of decltype
Combining decltype with auto
Combining decltype with the `auto` keyword offers exceptional flexibility in type deduction, allowing developers to write more generic and reusable code.
auto number = 5;
decltype(number) anotherNumber = 10; // both are of type int
Here, `anotherNumber` inherits its type from `number`, illustrating how decltype can elegantly handle type deduction without explicit type definitions.
Using decltype with Templates
Templates are another area where decltype shines. It allows for type deduction based on template parameters, which is crucial when writing generic functions.
template<typename T>
void showType(T arg) {
decltype(arg) val = arg; // val has the same type as arg
}
In this example, the function `showType` takes a parameter of type `T` and uses decltype to create a variable `val` that matches the type of the argument `arg`.
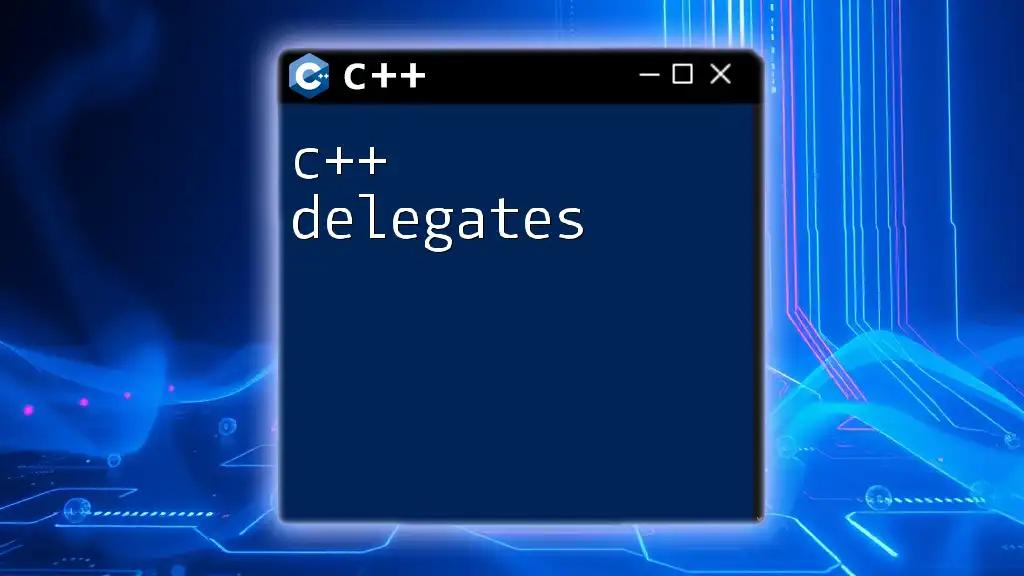
Memory Considerations with decltype
Does decltype impact memory usage? The good news is that using decltype does not lead to any additional memory overhead as it simply deduces the type of an expression at compile time. This makes it a lightweight feature that preserves efficiency in code without compromising clarity or safety.
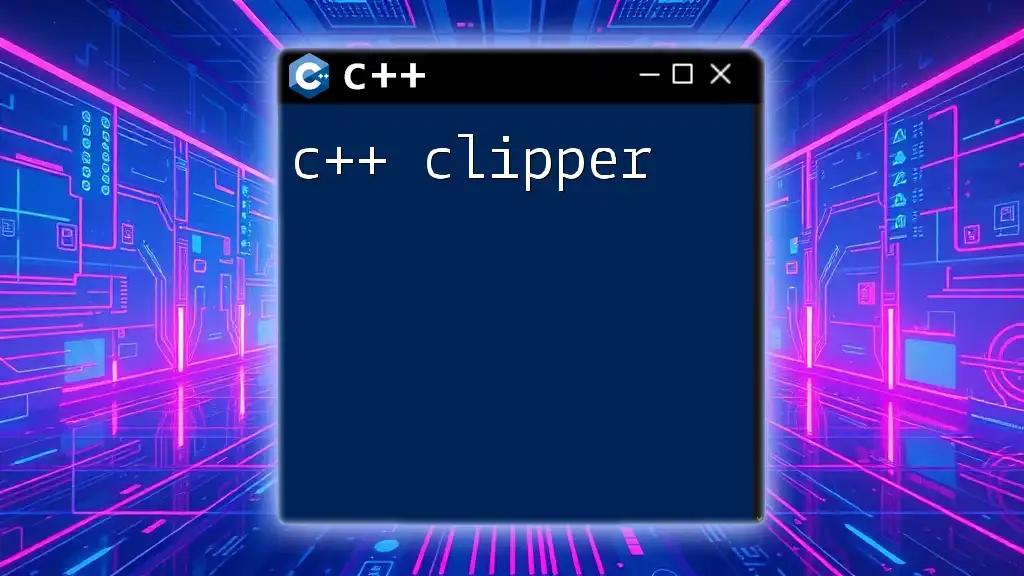
Common Pitfalls
Limitations of decltype
Despite its advantages, decltype has limitations, and developers should be mindful of cases where it may yield unexpected results. One common issue is when dealing with references or lvalues/rvalues.
int x = 5;
decltype((x)) y = x; // y is an int&, not an int
In this case, `y` becomes a reference to `x` (of type `int&`), which might not be the intended result for some developers.
Best Practices
When working with decltype, consider the following best practices:
- Use decltype in contexts where type deduction is complex: Leverage it for function return types or when interacting with libraries where type traits matter.
- Avoid excessive dependence on decltype: While it can be a powerful ally, overusing it might lead to less readable code. Maintain a balance between clarity and conciseness.

Conclusion
In wrapping up, the decltype in C++ feature offers a modern, type-safe approach to variable declarations and type deductions. Its flexibility in conjunction with keywords like `auto` and its application in templates make it an indispensable tool for any C++ programmer looking to enhance their coding practices.

Next Steps for Learning More
For those who want to dive deeper into decltype, consider exploring additional resources, including advanced C++ books, online courses focused on modern C++, and documentation on type deduction and templates. To further enhance your understanding, engage with community tutorials and forums where C++ enthusiasts share their insights and tips.