In C++, you can check the type of a variable at compile time using the `typeid` operator or the `std::is_same` type trait for type comparisons.
Here’s a code snippet that demonstrates checking a variable's type using `typeid`:
#include <iostream>
#include <typeinfo>
int main() {
int x = 10;
std::cout << "The type of x is: " << typeid(x).name() << std::endl;
return 0;
}
Alternatively, you can use `std::is_same` to compare types:
#include <iostream>
#include <type_traits>
int main() {
int x = 10;
std::cout << "Is x of type int? " << std::boolalpha << std::is_same<decltype(x), int>::value << std::endl;
return 0;
}
What is Type Checking in C++?
Type checking in C++ refers to the verification of the data types involved in operations, ensuring that they are compatible. This process can occur at compile-time, allowing many errors to be caught before the program even runs, or at runtime, which can identify type mismatches during execution. Understanding how to effectively check types is crucial for writing safer and more reliable C++ code.
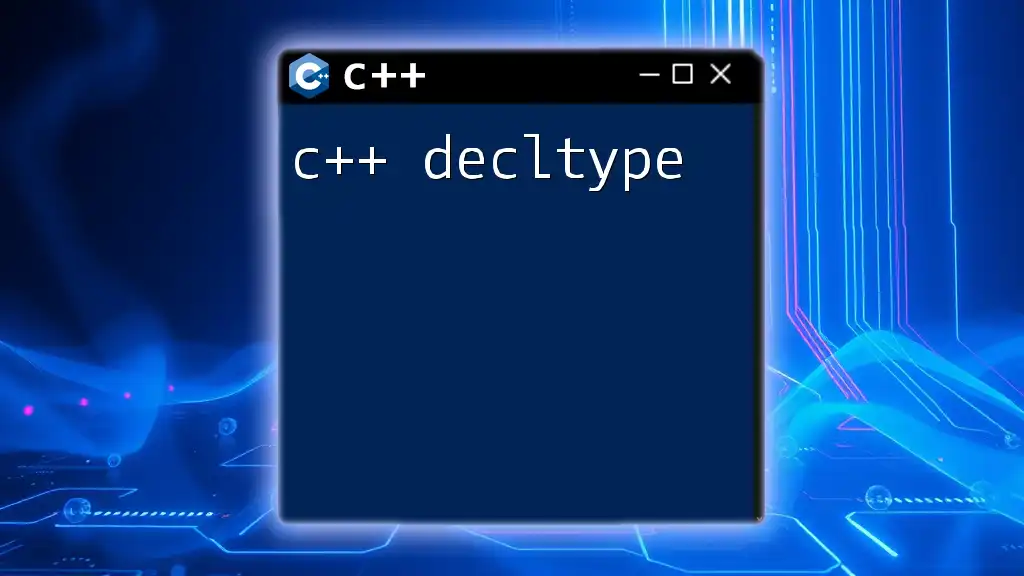
Why Understanding Type Checking is Essential for C++ Programmers
A solid understanding of type checking is essential for C++ programmers for several reasons. Firstly, it helps prevent potential runtime errors that could lead to crashes or undefined behavior in software applications. Secondly, it allows developers to write optimized and maintainable code, reducing the likelihood of bugs. Type checking also enhances code readability by explicitly communicating the intended use of variables and functions.
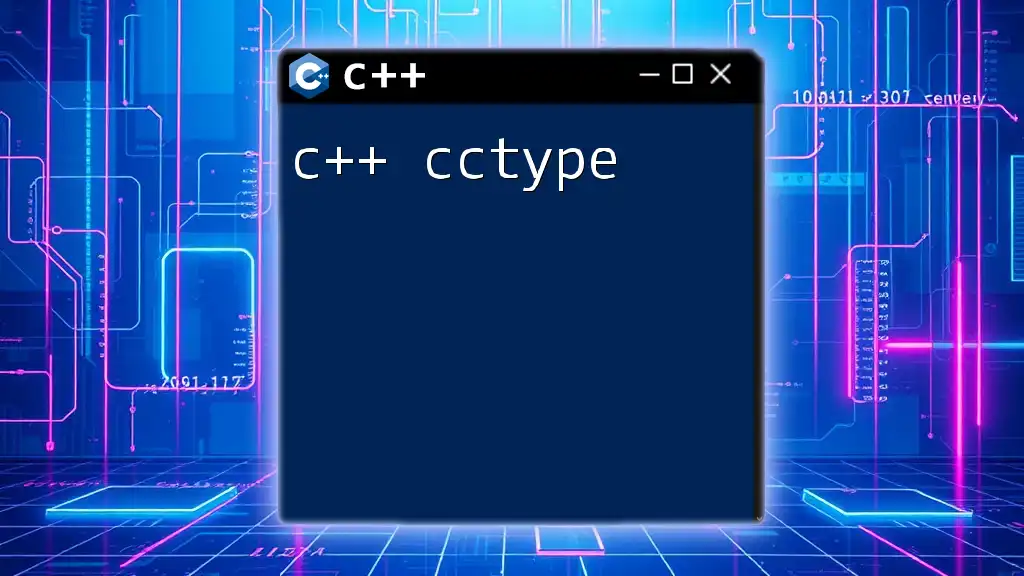
Overview of Fundamental Data Types
C++ is rich in data types that categorize the kinds of values that variables can hold. Fundamental data types include:
- int: Used for integer values.
- char: Represents single characters.
- float: Stores floating-point numbers, handling decimal values.
- double: Similar to float but with higher precision.
- bool: Represents boolean values (true or false).
Understanding these data types is vital, as they form the foundation of variable declarations and operations within C++.
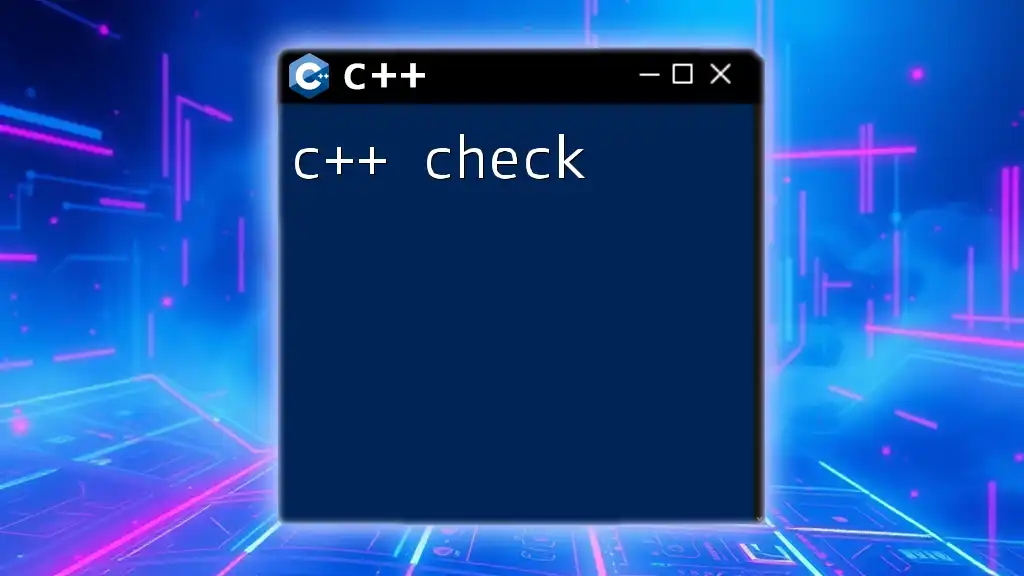
User-Defined Types
C++ also supports user-defined types, which allow developers to create types tailored to their specific needs. The most common user-defined types include:
- Classes: Create complex types encapsulating behavior and data.
- Structs: Similar to classes but typically used for simpler data structures.
- Enums: Define a collection of named integer constants.
- Typedefs: Give new names to existing data types for clarity and convenience.
By mastering user-defined types, programmers can enhance the organization of their code and better model real-world entities.
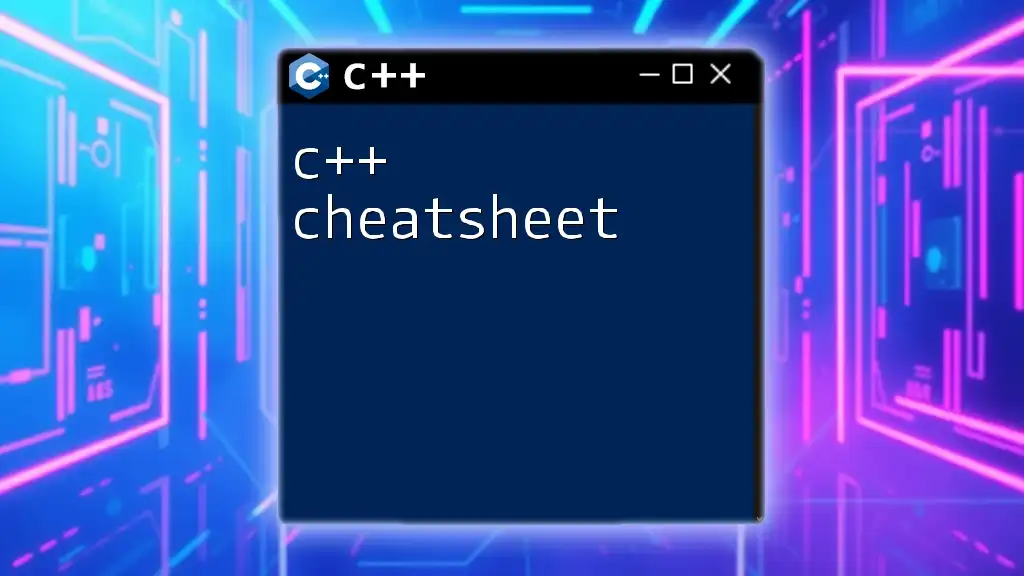
Methods for Checking Types in C++
Using `typeid`
The `typeid` operator is one of the simplest ways to check types in C++. It provides information about the object's type at runtime.
The syntax looks like this:
typeid(expression).name()
Example:
#include <iostream>
#include <typeinfo>
int main() {
int myInt = 42;
std::cout << "The type of myInt is: " << typeid(myInt).name() << std::endl;
return 0;
}
This will output something like `"int"` depending on your compiler’s implementation.
The output of `typeid` gives you a type’s name, which is a handy way to debug your code and ensure that the data types are as expected.
Utilizing `decltype`
The `decltype` specifier is a powerful feature in C++ that retrieves the type of an expression at compile-time without evaluating it.
The syntax is straightforward:
decltype(expression)
Example:
#include <iostream>
int main() {
int myVar = 10;
decltype(myVar) anotherVar = 20; // anotherVar is also int
std::cout << "The type of anotherVar is int: " << std::is_same<decltype(anotherVar), int>::value << std::endl;
return 0;
}
In this example, `decltype(myVar)` deduces the type of `myVar` as `int`, and `anotherVar` will also be of type `int`. The combination with `std::is_same` enables you to check if the deduced type matches another type.
The `std::is_same` Type Trait
C++ offers a suite of type traits, with `std::is_same` serving a critical role in validating type equivalence at compile-time. This allows for a cleaner design, especially when using templates.
The syntax involves:
std::is_same<T, U>::value
Example:
#include <iostream>
#include <type_traits>
int main() {
std::cout << std::is_same<int, int>::value << std::endl; // Outputs 1 (true)
std::cout << std::is_same<int, float>::value << std::endl; // Outputs 0 (false)
return 0;
}
In the output, `1` denotes that `int` is the same as `int`, and `0` indicates that `int` is not the same as `float.` This can be extremely useful in template metaprogramming, where you may want to enforce type safety or adapt functionality based on specific types.
Template Specialization for Type Checking
Template specialization allows for providing different implementations based on specific types. This is particularly useful for type checks in generic programming.
Here’s how it works:
#include <iostream>
template<typename T>
void checkType(T var) {
std::cout << "Type: unknown" << std::endl;
}
template<>
void checkType<int>(int var) {
std::cout << "Type: int" << std::endl;
}
int main() {
checkType(10); // Outputs: Type: int
checkType(10.5); // Outputs: Type: unknown
return 0;
}
In this example, `checkType` has a generic definition for unknown types and a specialized version for `int`. The specialization enables a precise check, providing a clear distinction in handling data types.
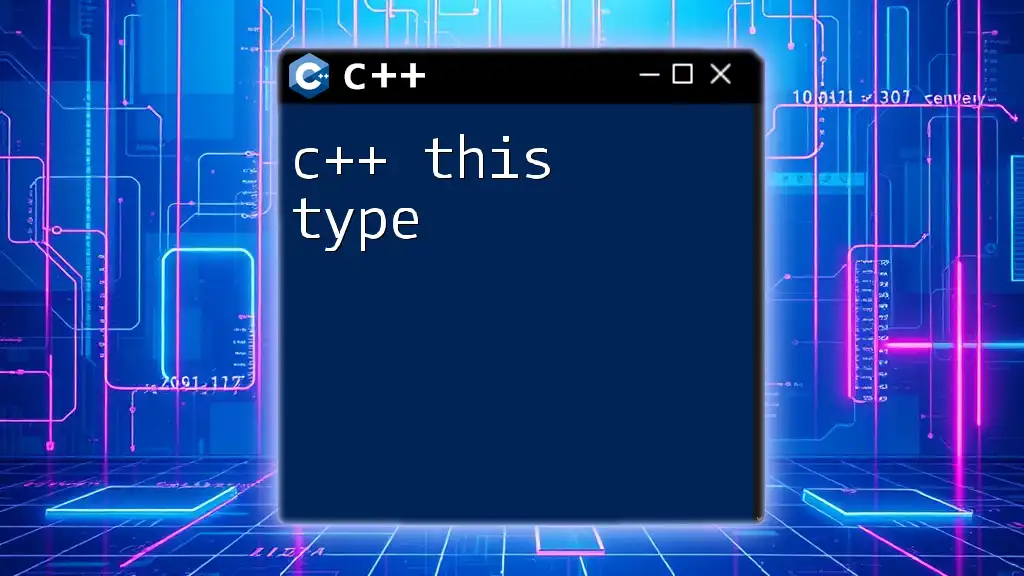
Dynamic Type Checking
RTTI (Run-time Type Information)
Runtime Type Information (RTTI) is a feature that enables the checking of object types at runtime, which is particularly useful when working with polymorphism.
One of the main functions of RTTI is `dynamic_cast`, which safely downcasts pointers and references in an inheritance hierarchy.
For example:
#include <iostream>
class Base {
virtual void func() {}
};
class Derived : public Base {};
int main() {
Base* b = new Derived();
if (Derived* d = dynamic_cast<Derived*>(b)) {
std::cout << "Successful downcast!" << std::endl;
} else {
std::cout << "Downcast failed." << std::endl;
}
delete b;
return 0;
}
This checks if the base pointer `b` can be safely downcasted to a pointer of type `Derived`. Using `dynamic_cast` can produce overhead, so it’s advisable to use it judiciously, especially in performance-critical applications.
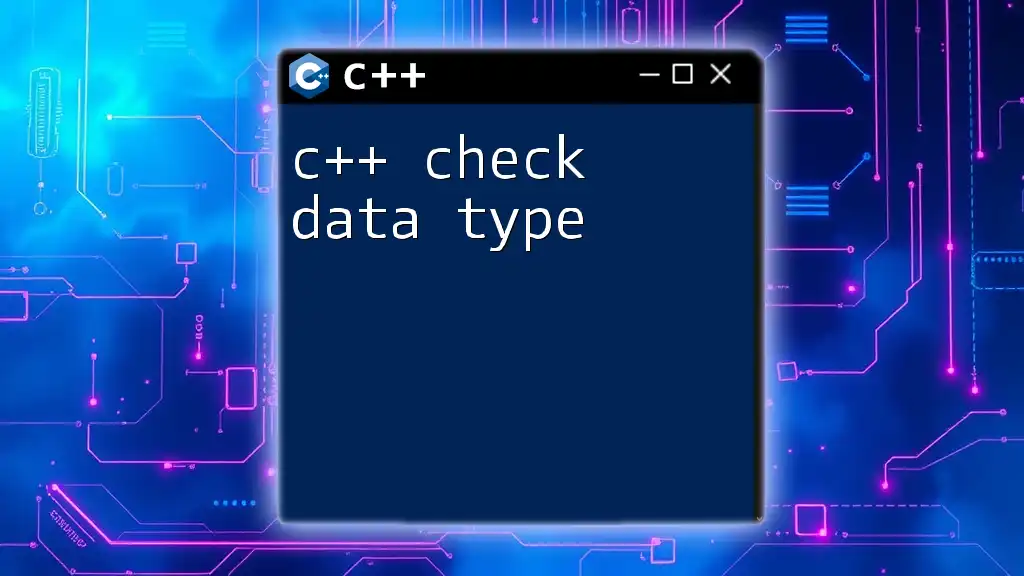
Best Practices for Type Checking in C++
Use Cases for Compile-Time vs Run-time Type Checking
Choosing between compile-time and run-time type checking depends largely on the situation. Compile-time checking, via techniques such as `typeid` or `decltype`, allows for early identification of errors and generally yields better performance. Conversely, run-time checking is beneficial when you have a flexible system where type decisions must be made during execution, such as in polymorphic code.
Performance Considerations
While type checking is essential for error prevention, excessive use can lead to performance degradation. It's crucial to balance error checking and performance, optimizing checks that are absolutely necessary while avoiding redundant ones.
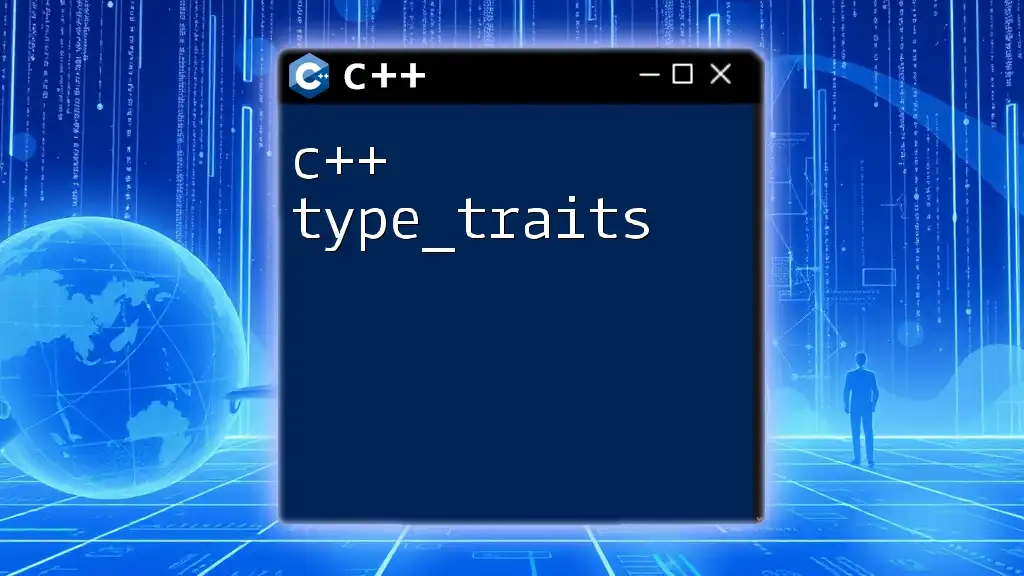
Conclusion
In summary, understanding how to check types in C++ effectively is vital for writing robust, maintainable, and error-free code. By leveraging features like `typeid`, `decltype`, `std::is_same`, and template specialization, along with insights into dynamic type checking through RTTI, programmers can enhance their proficiency in C++. The encouragement lies in applying these concepts in real-world scenarios, improving both your coding skills and your grasp of C++'s powerful type system.