In C++, you can check if a value is within a specified range using a simple conditional statement.
int value = 10;
if (value >= 1 && value <= 20) {
// Value is in range
}
Understanding the Concept of Range Checking
What is Range Checking?
Range checking is a crucial programming practice that involves determining whether a given value falls within a specified range. The significance lies in ensuring that values adhere to expected boundaries, which helps in maintaining program stability and integrity. Failing to perform range checks can lead to unexpected behavior, crashes, or vulnerabilities within the software.
Common Scenarios for Range Checking
Range checking is indispensable in various scenarios, including:
- User Input Validation: When accepting inputs, especially from users, it's vital to ensure that the data conforms to expected limits. This prevents incorrect data from causing runtime errors.
- Array Indices Management: In programming, accessing data structures like arrays demands strict adherence to boundaries. A mistake here can result in out-of-bound errors, potentially leading to data corruption.
- Business Logic Constraints: Applications often have rules governing acceptable values. For example, a financial application may restrict certain transactions based on budget limits.
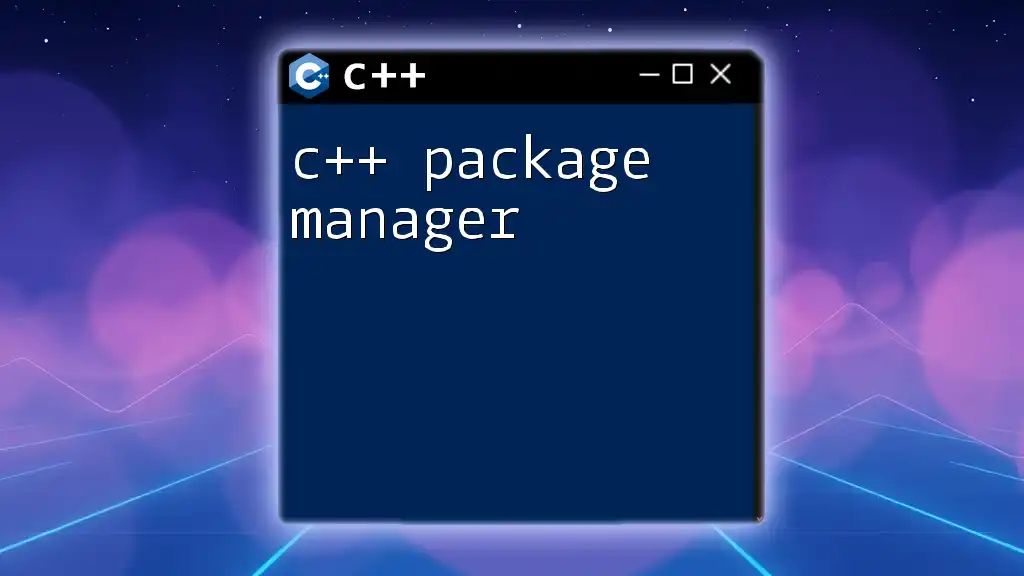
How to Check if a Value is Within a Range in C++
Basic Syntax for Range Checking
Checking if a value is within a range generally involves comparison operators, specifically `<`, `>`, `<=`, and `>=`. These operators allow you to establish if a value lies within the defined boundaries.
Using Conditionals to Check Range
The `if` statement is the primary tool for implementing range checks. Here is a straightforward example:
int value = 10;
if (value >= 1 && value <= 100) {
cout << "Value is within range." << endl;
} else {
cout << "Value is out of range." << endl;
}
In the example above, the value `10` is checked against the range of `1` to `100`. The output confirms that the value is indeed within the specified range.
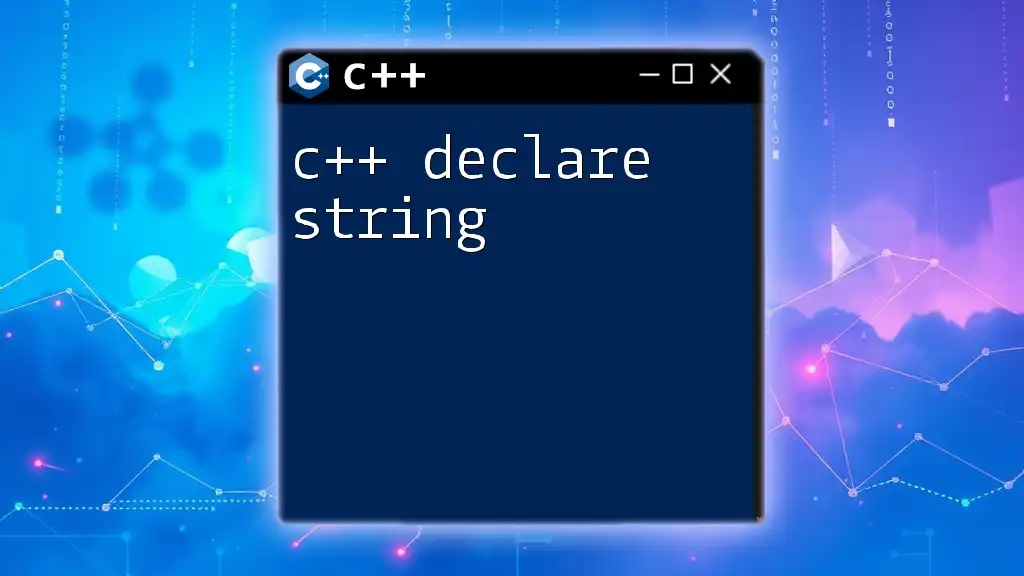
Advanced Techniques for Range Checking
Creating a Function for Reusability
To promote reuse and enhance code organization, it is beneficial to abstract range checking into a function. This modular approach can help streamline your main logic. Here’s an example function:
bool isInRange(int value, int min, int max) {
return (value >= min && value <= max);
}
int main() {
cout << isInRange(10, 1, 100) << endl; // Output: 1 (true)
}
This function, `isInRange`, takes three parameters: the value to check, the minimum, and the maximum. It returns a boolean indicating whether the value falls within the specified range.
Using Ternary Operator for Conciseness
For scenarios where brevity is essential, you can employ the ternary operator, which condenses if-else logic into a single line. Here’s an example:
int value = 50;
string result = (value >= 1 && value <= 100) ? "In range" : "Out of range";
cout << result << endl; // Output: In range
This approach not only saves space but also improves readability when used judiciously.
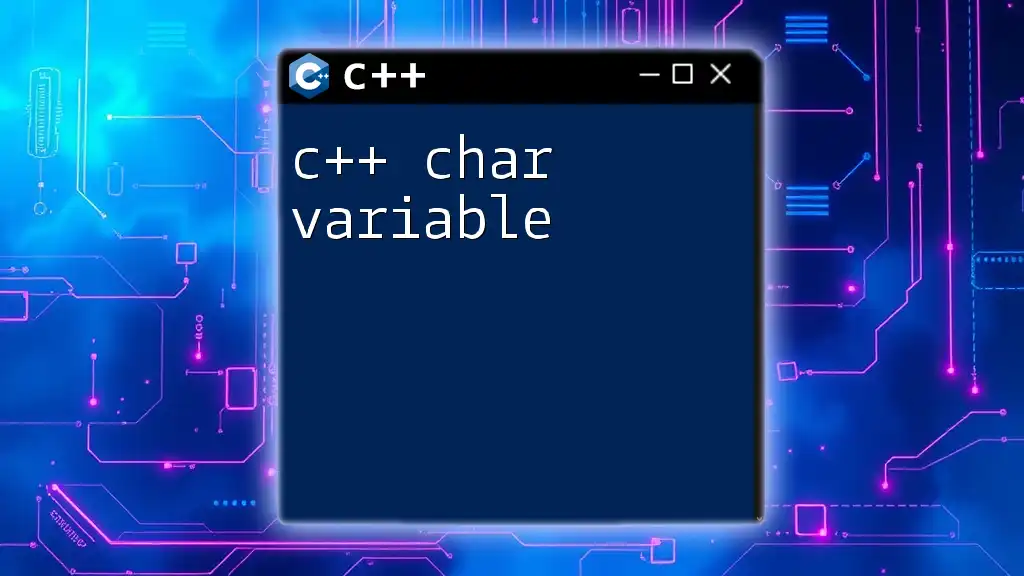
Handling Edge Cases in Range Checking
Boundary Conditions
Validating edge cases—especially boundary values—is critical in programming. Often scenarios arise where the exact minimum or maximum needs special handling.
For example, consider a case where zero is the lower boundary:
int value = 0;
if (value >= 0 && value <= 100) {
cout << "Value is within range." << endl; // Output: Value is within range.
}
Handling Negative Values
It is common for applications to require range checks that include negative values. Say you're working with temperatures, where acceptable values can dip below zero. You might implement a check like this:
int value = -5;
if (value >= -10 && value <= 0) {
cout << "Value is within acceptable negative range." << endl; // Output: Value is within acceptable negative range.
}
This ensures that your program accounts for all potential scenarios and ranges effectively.
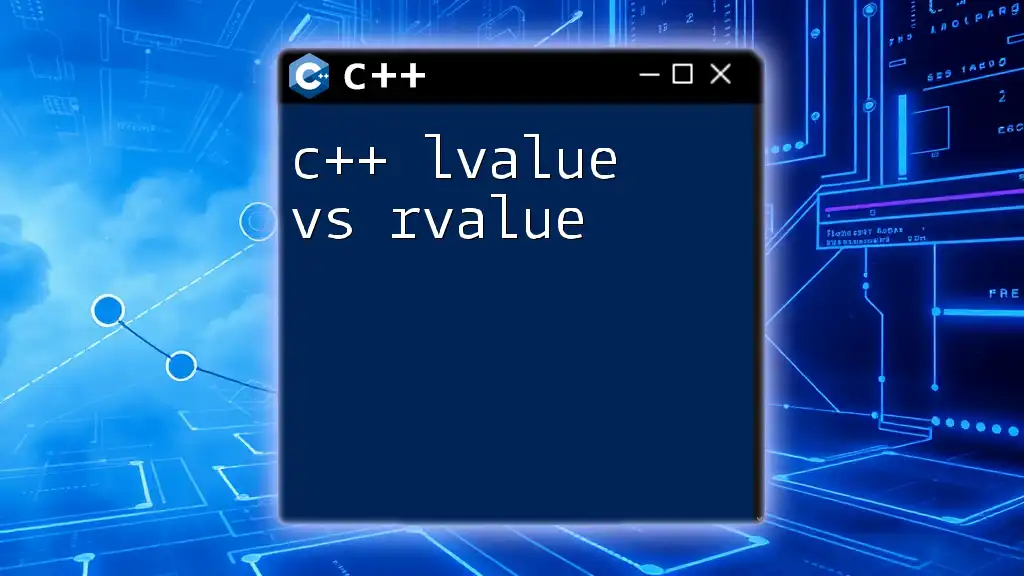
Utilizing STL for Range Checking
Introduction to Standard Template Library (STL)
The Standard Template Library (STL) provides robust methods and algorithms that facilitate range checks. A particularly handy function is `std::find()`, which can be used to verify the existence of an element within a specific range.
Using `std::clamp()` for Containing Values
Another powerful tool introduced in C++17 is `std::clamp()`, which ensures that a given value matches specified constraints by either capping it or reducing it to the nearest edge. Here’s an example:
#include <algorithm> // Include this for std::clamp
int value = 150;
int clampedValue = std::clamp(value, 1, 100);
cout << "Clamped Value: " << clampedValue << endl; // Output: Clamped Value: 100
This approach simplifies the control of value ranges and reduces the need for repetitive range checks.
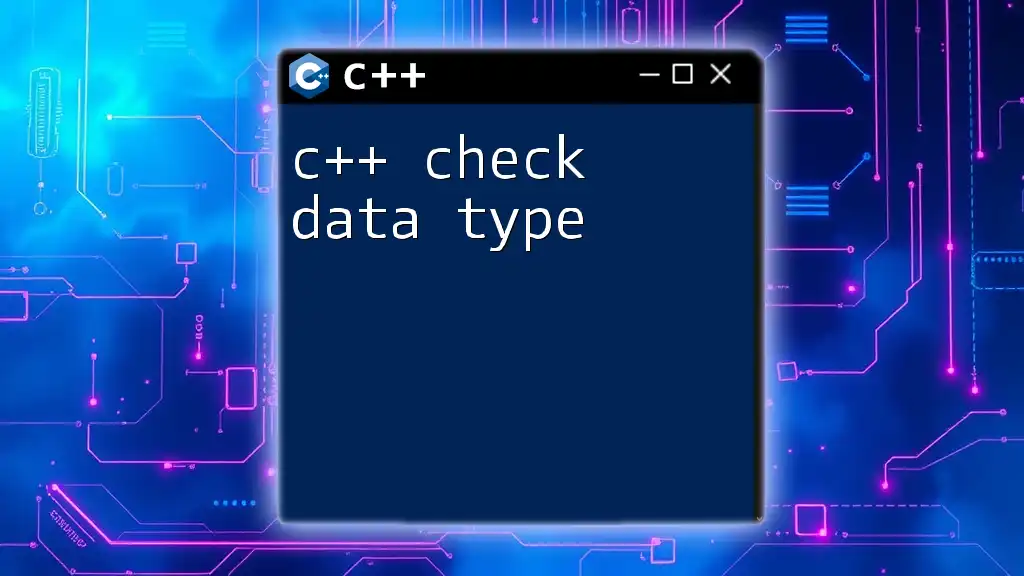
Best Practices for Checking Ranges
Consistent and Clear Coding Style
When dealing with range checks, maintaining a clear coding style is vital. Readable code not only aids future maintenance but also helps when collaborating with others. Use meaningful variable names and provide comments when necessary to clarify intent.
Error Handling and Logging
Incorporating robust error handling can significantly enhance the user experience. Logging invalid inputs allows developers to trace issues promptly. For example:
if (!isInRange(value, 1, 100)) {
cerr << "Error: Value out of range: " << value << endl;
}
This style of error reporting aids in debugging and maintaining application integrity.
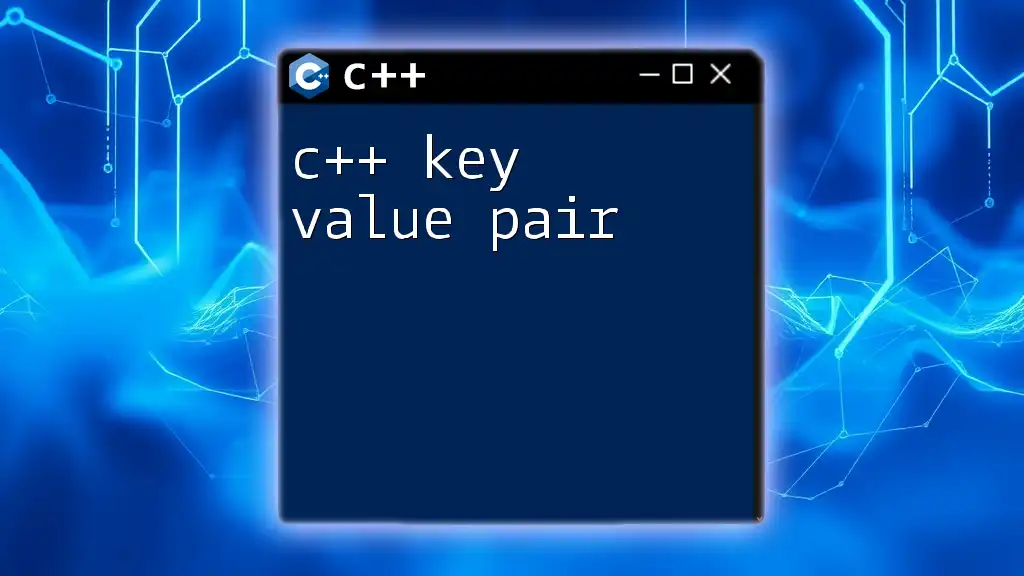
Conclusion
Range checking in C++ is a foundational skill that every programmer should master. Ensuring values are within defined limits protects your code from unexpected behavior while enhancing reliability. By employing various techniques outlined in this guide—from straightforward conditional checks to utilizing STL functions—you can create robust applications that handle a myriad of scenarios effectively. For further learning, explore additional C++ resources and delve into best practices that elevate your coding skills. Stay curious, and happy coding!