A C++ `char` variable is used to store a single character and is defined by placing the character within single quotes.
char myChar = 'A';
What is a Character in C++?
A character is a fundamental data type used in C++ programming that represents a single text character. Characters can be letters, digits, punctuation marks, or any other symbol represented in ASCII or Unicode. Each character is denoted by its corresponding code in the character set.
- ASCII vs Unicode Characters: ASCII (American Standard Code for Information Interchange) uses a 7-bit encoding scheme to represent characters, while Unicode can represent characters from multiple languages and symbols worldwide, providing a much larger character set.
Understanding characters and their representation is crucial for tasks such as text processing, input validation, and string manipulation.
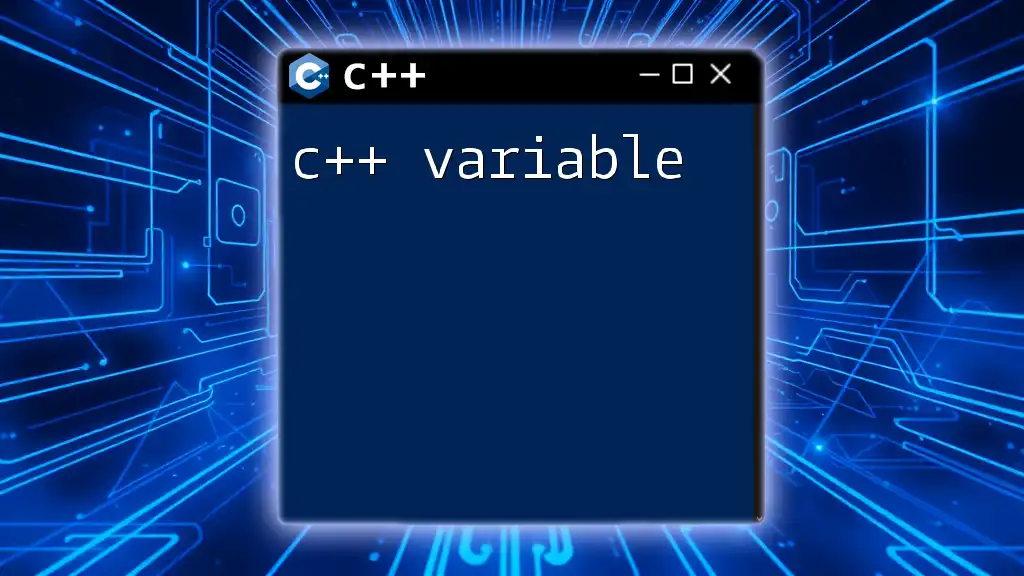
How to Use Char in C++
To effectively utilize a C++ char variable, it’s essential to understand how to declare and initialize it correctly.
Declaring a Char Variable
In C++, you can declare a char variable using the following syntax:
char myChar;
This line creates a char variable named `myChar` which can store a single character.
Initializing a Char Variable
You can initialize a char variable at the time of declaration. Here’s how to do it:
char myChar = 'A'; // Simple initialization
You can also initialize with different characters:
char digit = '1'; // A character representing the digit '1'
char symbol = '#'; // A character representing the symbol '#'
Default Values of Char Variables
If a char variable is declared but not initialized, it will contain a default value that is often undefined. It's a good practice to always initialize your char variables. Here’s an example showing default initialization:
char myChar; // Undefined value
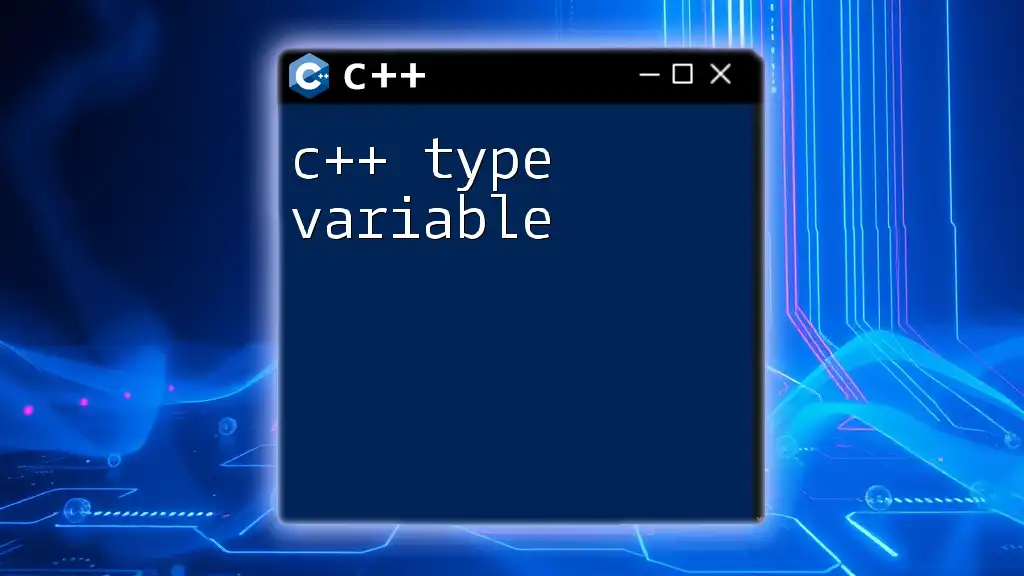
Understanding Character Variable C++
The c++ char variable has specific characteristics that make it unique among data types.
Characteristics of Char Variables
- Memory Size and Limits: Typically, a char variable takes up one byte of memory, which can store values ranging from -128 to 127 in a signed char and 0 to 255 in an unsigned char.
- Data Type Specifications: A char is implicitly convertible to integer types, allowing you to perform arithmetic operations under certain conditions.
Char Variable Storage
Characters are stored in memory using their corresponding ASCII or Unicode values:
char myA = 'A'; // Stored in memory as its ASCII value 65
In this case, the character `'A'` is stored in memory as 65, which is its ASCII representation.
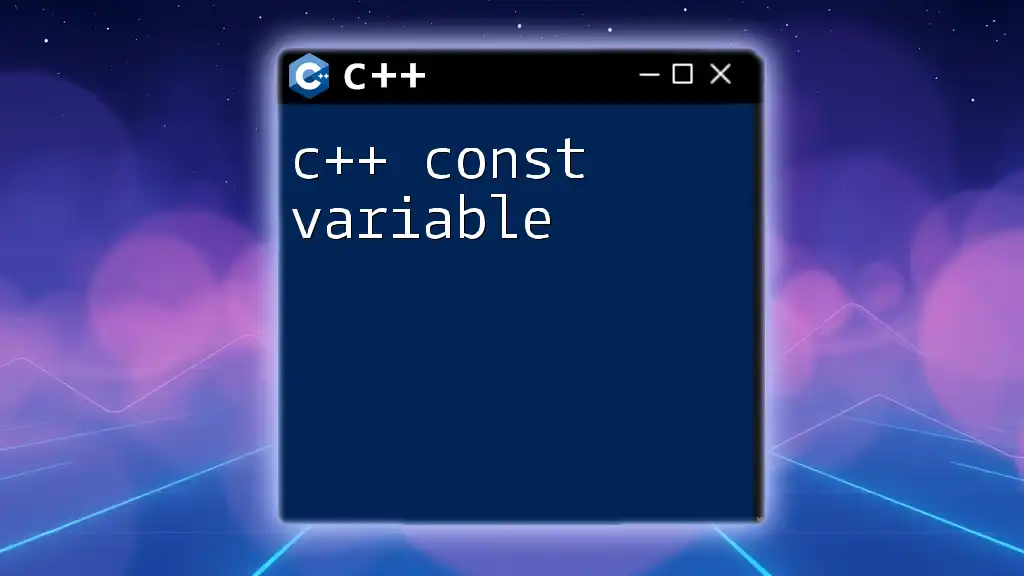
Working with Char Variables in C++
Understanding how to perform operations on char variables is essential to effectively using them in your code.
Basic Operations on Char Variables
Operations on characters often involve comparison. Here's an example of how to compare char values:
char char1 = 'A';
char char2 = 'B';
if (char1 < char2) {
// This will evaluate to true since 65 < 66
cout << char1 << " is less than " << char2;
}
Combining Char Variables
You can also build strings from char variables. Strings are essentially arrays of characters. Here’s an example:
char first = 'H';
char second = 'i';
char exclamation = '!';
char greeting[4] = { first, second, exclamation, '\0' }; // Null-terminated string
cout << greeting; // Output: Hi!
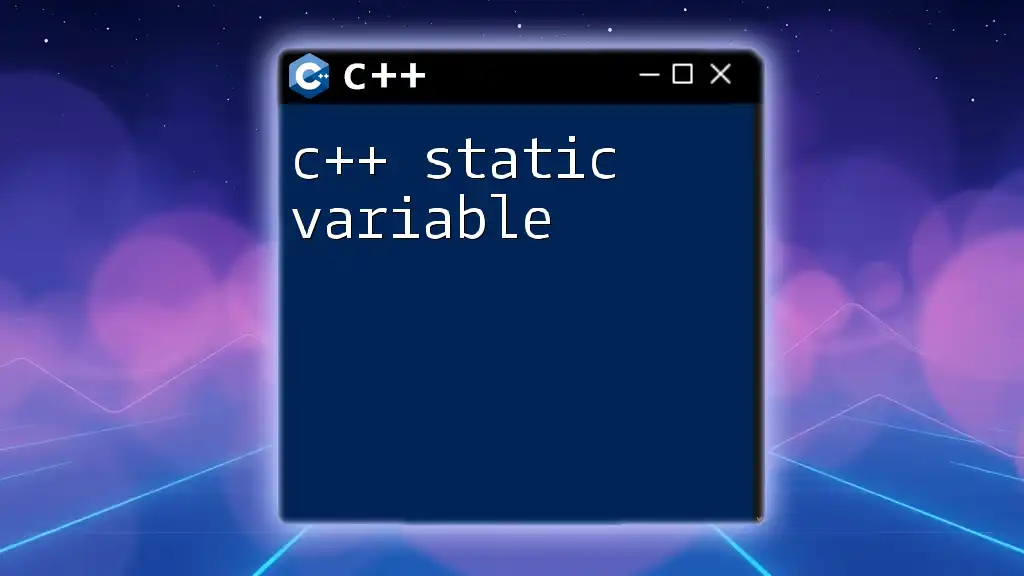
Character in C++: Advanced Topics
Once you have a solid understanding of char variables, you can dive into more advanced topics like char arrays and special characters.
Char Arrays and Strings
Char arrays are closely tied to the C++ character type, and it’s essential to distinguish between a `char` and a `string`. A char array can be treated as a string when it is null-terminated. Here’s how to declare and use char arrays:
char name[5] = "John"; // String initialization
In this case, the character array `name` stores the characters 'J', 'o', 'h', 'n', and a null terminator.
Special Characters in C++
C++ supports various escape sequences that represent special characters. For instance, `\n` represents a newline, and `\t` represents a tab. Here's how you might use escape sequences in code:
cout << "Hello, World!\n"; // New line after printing
cout << "Column 1\tColumn 2"; // Tab space between columns
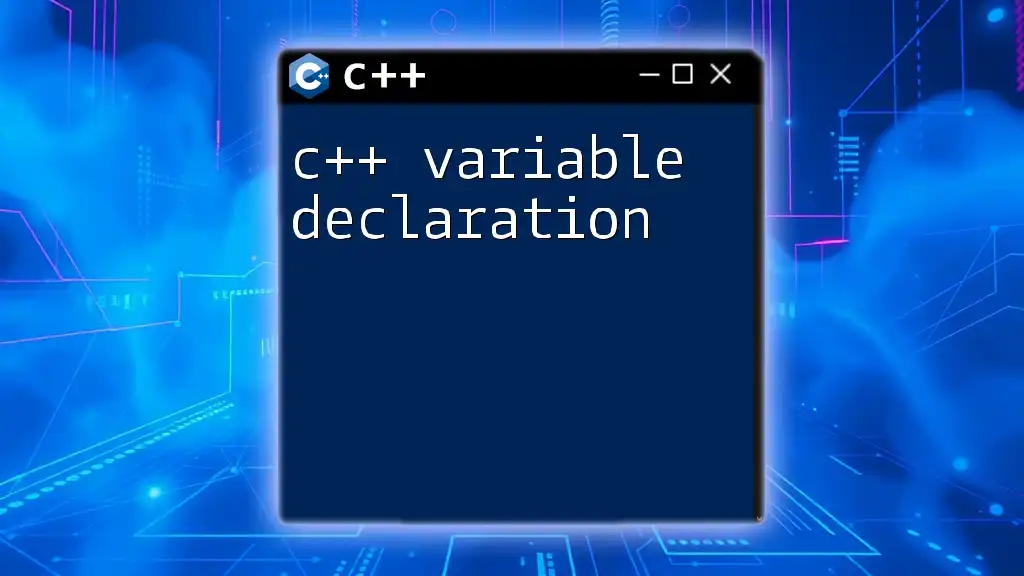
Common Pitfalls When Working with Char Variables
While working with c++ char variable, beginners often encounter several common pitfalls that can lead to unexpected behavior.
- Underestimating Char Limits: Always be mindful that `char` can hold a limited range of values; using it to store larger ASCII or Unicode values can lead to overflow issues.
- Misunderstanding Character Encoding: Since character encoding varies, ensure that you are aware of the environment you are programming in, especially when dealing with different languages or systems.
Here’s an example of a common error:
char overflowChar = 300; // This will lead to overflow
This will likely yield an unexpected character because the value exceeds the limit for a standard char variable.
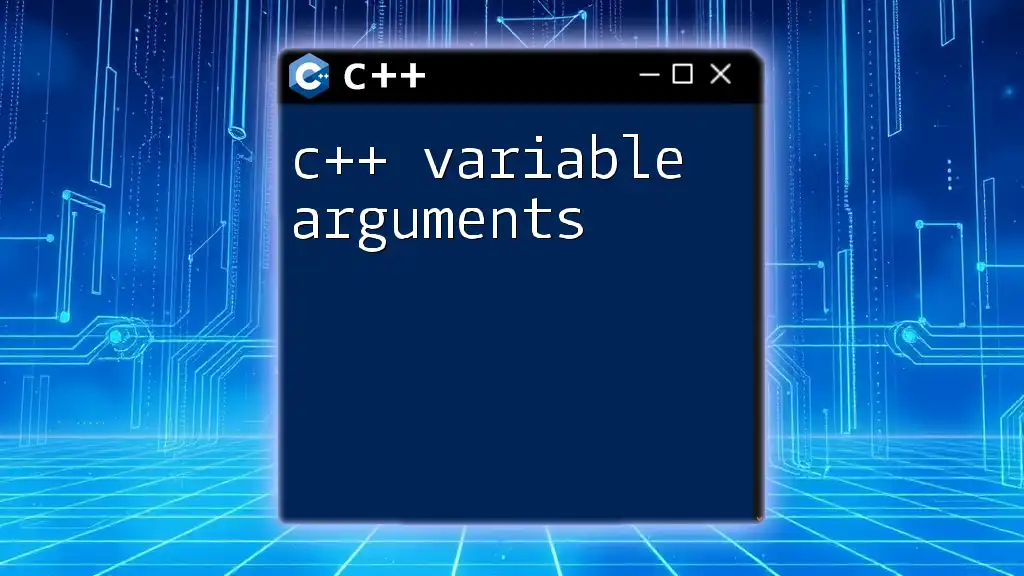
Conclusion
In summary, mastering the c++ char variable is key for any aspiring C++ developer. By understanding the declaration, initialization, and manipulation of char variables, you can enhance your programming skills significantly.
Practice using char variables in various contexts, and explore the complexities of character arrays, escape sequences, and other advanced topics. Familiarity with these concepts will not only improve your coding proficiency but also prepare you for more complex exercises in C++.
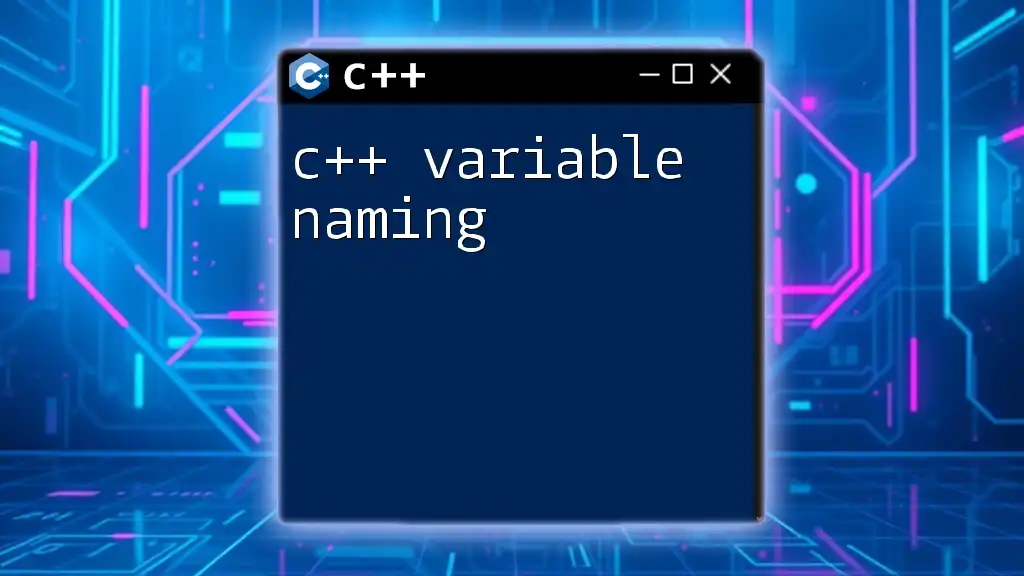
Additional Resources
For further learning, consider diving into well-reviewed books on C++ or taking comprehensive online courses that focus on data types and variables. Additionally, many online resources provide practical examples and exercises that can deepen your understanding of the C++ char variable.
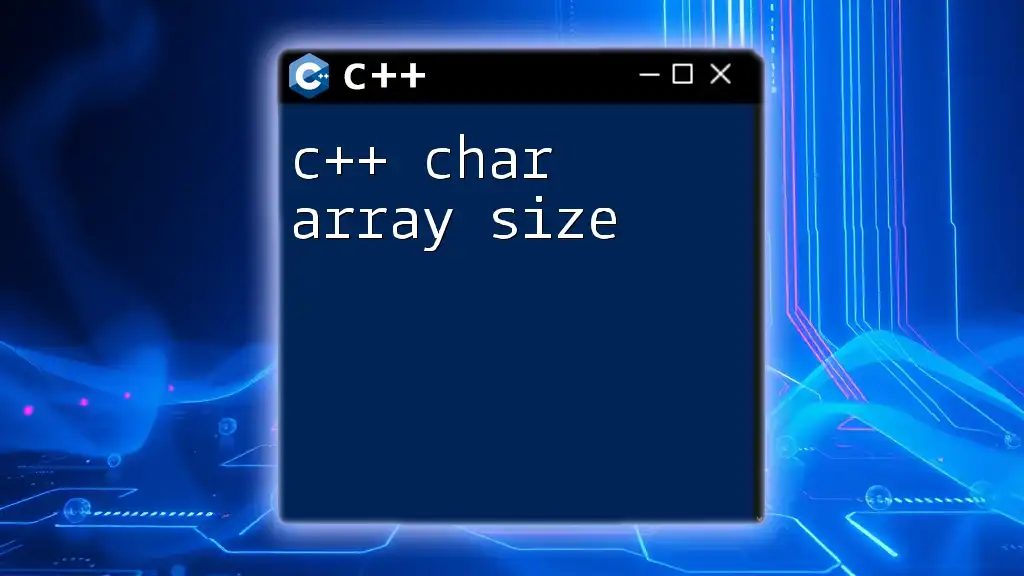
Call to Action
We invite you to share your experiences or any questions you may have regarding the use of C++ char variables. Stay tuned for our upcoming posts where we'll explore more advanced C++ programming topics!