In C++, a "barrier" refers to a synchronization point where threads must wait for each other to reach before any can proceed, ensuring that certain tasks are completed before continuing execution.
Here's a simple example using the `std::barrier` class introduced in C++20:
#include <iostream>
#include <thread>
#include <barrier>
#include <vector>
void task(std::barrier<> &b) {
std::cout << "Thread " << std::this_thread::get_id() << " is performing some work.\n";
// Simulate work with a sleep
std::this_thread::sleep_for(std::chrono::milliseconds(100));
b.arrive_and_wait(); // Wait for other threads
std::cout << "Thread " << std::this_thread::get_id() << " has passed the barrier.\n";
}
int main() {
const int num_threads = 3;
std::barrier<> b(num_threads); // Create a barrier for 3 threads
std::vector<std::thread> threads;
for (int i = 0; i < num_threads; ++i) {
threads.emplace_back(task, std::ref(b));
}
for (auto &t : threads) {
t.join();
}
return 0;
}
Understanding the Concept of Barrier
What is a Barrier in C++?
In C++, a barrier is a synchronization primitive used primarily in multithreaded programming. It serves as a point where multiple threads can wait until a specified number of threads have reached that point. This helps in coordinating actions across different threads, preventing situations known as race conditions, where the outcome of operations may depend on the sequence or timing of uncontrollable events.
The primary function of a barrier is to ensure that multiple threads can work independently on their tasks and are only allowed to proceed when all participating threads reach the barrier point. Understanding barriers is essential for promoting data integrity and ensuring smooth communication and collaboration between multiple threads.
Types of Barriers
Synchronization Barriers
Synchronization barriers are designed to help manage coordination among threads. They allow all threads to arrive at a certain point in their execution before any of them can proceed. This is crucial in circumstances where threads rely on the results of others before continuing their process.
Example: In a parallel computation where different threads calculate parts of a matrix, the final results may depend on all threads completing their calculations before moving onto the aggregation step.
Memory Barriers
Memory barriers deal with the visibility and ordering of operations performed by threads. On muli-core processors, the compiler may reorder instructions for optimization purposes, leading to situations where one thread's changes to shared data aren't visible to others immediately.
Memory barriers ensure proper visibility of memory operations by introducing constraints on how memory reads and writes can be reordered. This is critical in maintaining the consistency of data being shared.
Example: If Thread A writes to a shared variable and Thread B reads from it, without appropriate memory barriers, Thread B may read stale data if Thread A’s write hasn’t been completed or propagated yet.

How to Implement Barriers in C++
Using std::barrier in C++20
Starting with C++20, the std::barrier class was introduced, making it easier to implement a barrier in applications. This feature simplifies multithreading as it manages the lifecycle of the barrier and the synchronization of threads.
Here’s a basic example of how to use `std::barrier` in a multithreaded application:
#include <iostream>
#include <thread>
#include <barrier>
const int numThreads = 3;
std::barrier syncPoint(numThreads);
void worker(int id) {
std::cout << "Worker " << id << " is doing some work.\n";
// Simulate doing work
syncPoint.arrive_and_wait(); // Wait for all threads
std::cout << "Worker " << id << " has reached the barrier.\n";
}
int main() {
std::thread threads[numThreads];
for (int i = 0; i < numThreads; ++i) {
threads[i] = std::thread(worker, i);
}
for (auto& th : threads) {
th.join();
}
return 0;
}
In this example, `syncPoint` is a barrier that waits for multiple threads to reach the same point of execution. Each thread calls `arrive_and_wait`, and if the number of arriving threads matches `numThreads`, then all of them are allowed to continue past the barrier.
Custom Implementation of Barriers
Creating a Simple Barrier Class
If you're working in an environment that doesn’t support C++20 features, you can create your own custom barrier using fundamental threading constructs such as mutexes and condition variables.
Here’s an implementation of a simple barrier:
#include <iostream>
#include <thread>
#include <mutex>
#include <condition_variable>
class SimpleBarrier {
public:
SimpleBarrier(int count) : thread_count(count), count(0) {}
void wait() {
std::unique_lock<std::mutex> lock(mtx);
if (++count == thread_count) {
cv.notify_all(); // Notify all waiting threads
} else {
cv.wait(lock); // Wait until notified
}
}
private:
std::mutex mtx;
std::condition_variable cv;
int thread_count;
int count;
};
void worker(int id, SimpleBarrier& barrier) {
std::cout << "Worker " << id << " is working.\n";
barrier.wait(); // Wait at the barrier
std::cout << "Worker " << id << " has crossed the barrier.\n";
}
int main() {
const int numThreads = 3;
SimpleBarrier barrier(numThreads);
std::thread threads[numThreads];
for (int i = 0; i < numThreads; ++i) {
threads[i] = std::thread(worker, i, std::ref(barrier));
}
for (auto& th : threads) {
th.join();
}
return 0;
}
The `SimpleBarrier` class counts the number of threads that have reached the barrier using a mutex for synchronization and a condition variable to block the threads until all threads arrive. Once all threads are at the barrier, they are notified to continue execution.
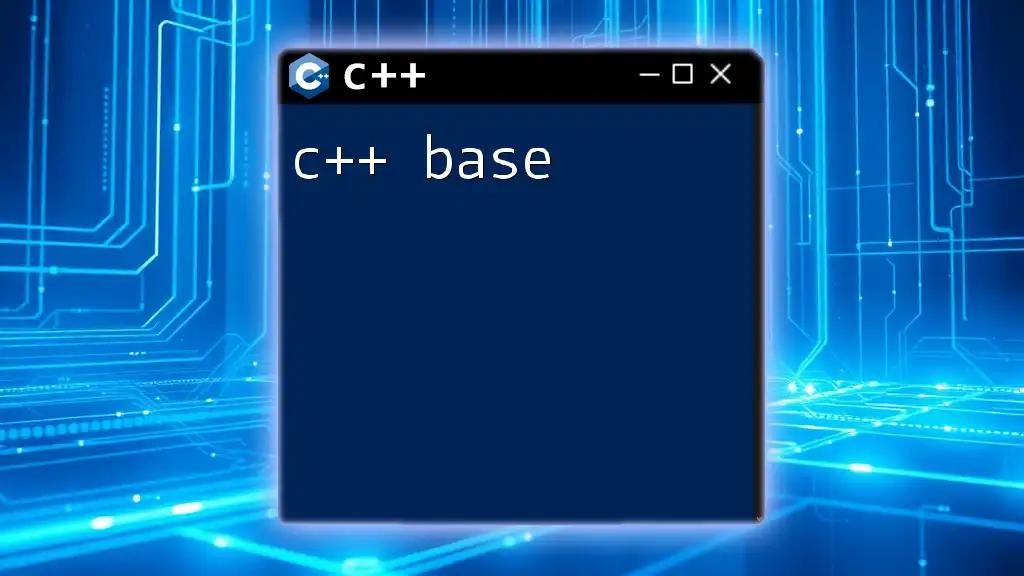
Use Cases of Barriers in C++
Real-World Applications
Parallel Computing
Barriers are extensively used in parallel computing to ensure that all computations from different threads reach a synchronization point. For instance, many numerical algorithms in scientific computing can benefit from this coordination. Libraries such as OpenMP and Intel TBB provide built-in support for barriers to help in managing complex dependencies in concurrent algorithms.
Game Development
In game development, it’s crucial to synchronize game state updates across multiple systems executing on different threads. For example, one thread may handle game logic, another for rendering, and yet another for input handling. Using barriers, developers ensure that the game state is consistent before rendering frames to the player, thus preventing glitches that might occur due to asynchronicity among threads.
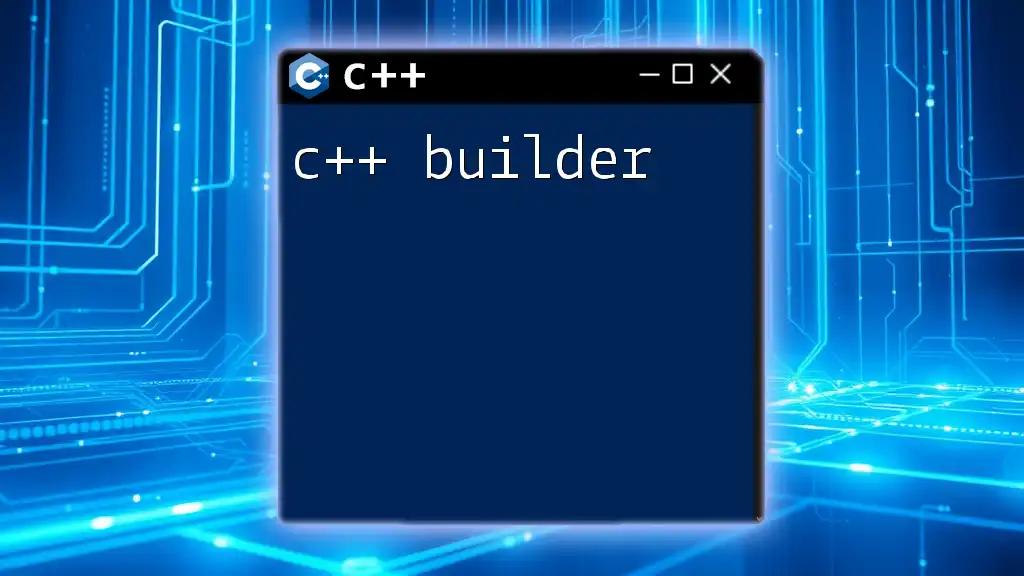
Best Practices for Using Barriers
Avoiding Common Pitfalls
When implementing barriers, be cautious about possible deadlock scenarios. This can happen if the threads are not effectively managed, leading to situations where threads indefinitely wait for conditions that will never be met. To prevent deadlocks, ensure the barrier is well-defined and that threads do not leave the wait state unexpectedly.
Performance Considerations
While barriers are useful, they come with performance overhead. It's important to minimize the number of times threads must synchronize since waiting introduces latency. When design choices can allow threads to complete their tasks independently, it’s often better to avoid barriers where possible. Analyze the trade-offs in your application to find the optimal balance between concurrency and synchronization.
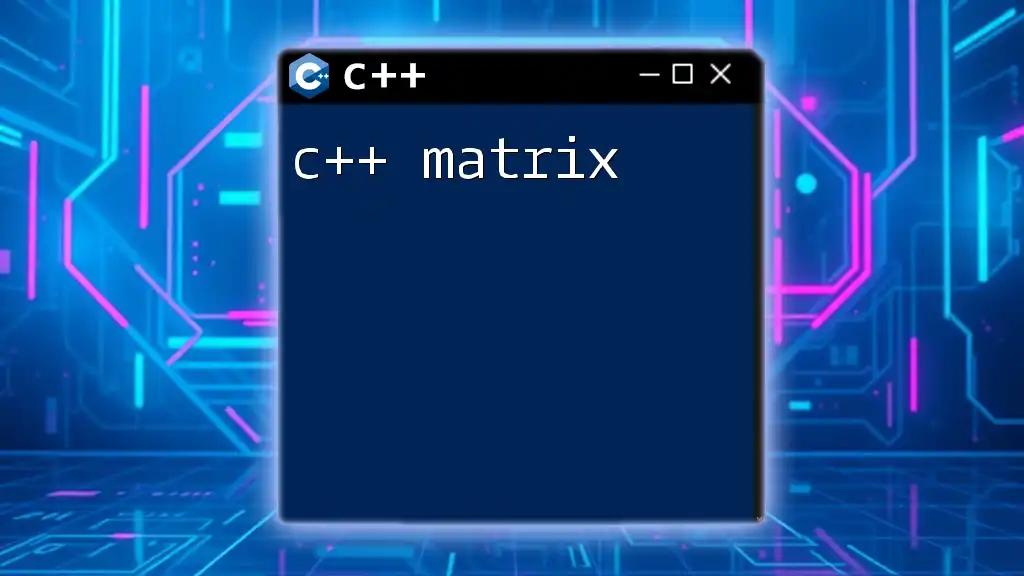
Conclusion
In conclusion, the C++ barrier is a vital tool in effective multithreaded programming. Mastery of barriers enhances your ability to manage threads, thus ensuring safety and correctness in concurrent applications. As you work with barriers, practice the provided examples to solidify your understanding and learn how to implement them in real-world scenarios. By following best practices and being mindful of performance, you can successfully leverage the power of barriers in your C++ applications.

Additional Resources
For further learning, explore the official documentation for C++ concurrency features, and dive into advanced topics on multithreading through comprehensive books and tutorials that focus on practical implementations and optimization techniques.