Base64 encoding in C++ is a method to convert binary data into an ASCII string format using a base 64 representation, which can be useful for data transmission over media designed to deal with text.
Here's a simple code snippet demonstrating how to encode a string to Base64 in C++:
#include <iostream>
#include <string>
#include <vector>
static const std::string BASE64_CHARS = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"
"abcdefghijklmnopqrstuvwxyz"
"0123456789+/";
std::string base64_encode(const std::string &in) {
std::string out;
int val = 0, valb = -6;
for (unsigned char c : in) {
val = (val << 8) + c;
valb += 8;
while (valb >= 0) {
out.push_back(BASE64_CHARS[(val >> valb) & 0x3F]);
valb -= 6;
}
}
if (valb > -6) out.push_back(BASE64_CHARS[((val << 8) >> valb) & 0x3F]);
while (out.size() % 4) out.push_back('=');
return out;
}
int main() {
std::string data = "Hello, World!";
std::string encoded = base64_encode(data);
std::cout << "Base64 Encoded: " << encoded << std::endl;
return 0;
}
What is Base64 Encoding?
Base64 encoding is a method of converting binary data into a text string. It employs a mechanism that uses a set of 64 different characters to represent binary data in an ASCII string format. This encoding scheme is particularly useful in situations where binary data needs to be represented in a textual format, such as embedding in HTML or transferring over protocols that only handle text.
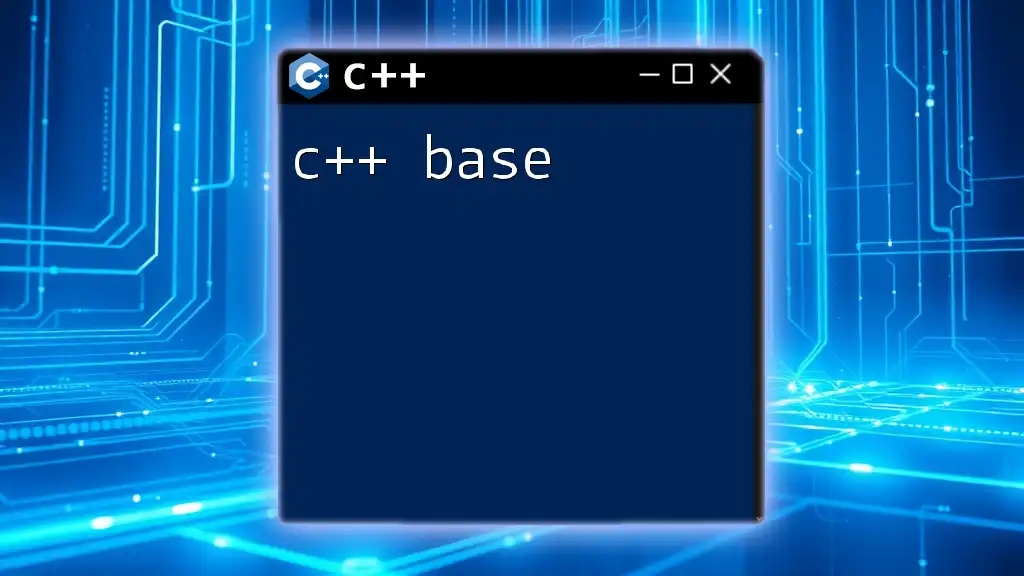
Why Use Base64 in C++?
In C++, Base64 encoding proves helpful in multiple scenarios, including but not limited to:
- Email Attachments: Most email clients support only text and may corrupt binary data. Base64 allows you to safely encode such attachments.
- Data Storage: When saving binary data in databases or files, Base64 ensures compatibility and integrity.
- Web APIs: Sending binary data in JSON objects requires encoding, making Base64 essential for API interactions.
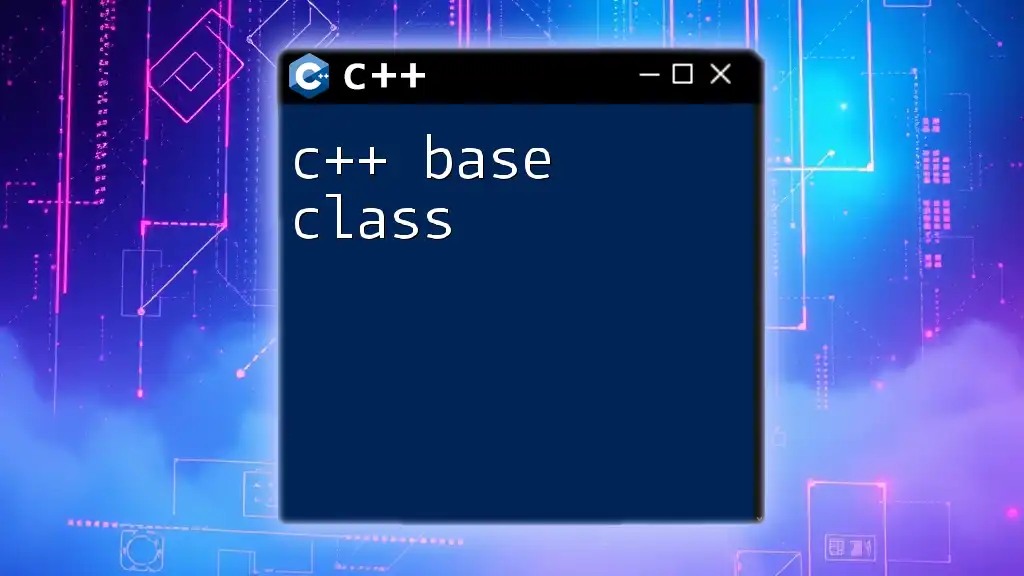
How Base64 Works
Base64 uses a range of 64 characters: `A-Z`, `a-z`, `0-9`, `+`, and `/` to represent binary data. The encoding process divides binary data into groups of 24 bits and splits each into four chunks of 6 bits. If the final group has fewer than 24 bits, it is padded using the `=` character to maintain the output's length. This ensures that the resulting string is a multiple of 4 characters.
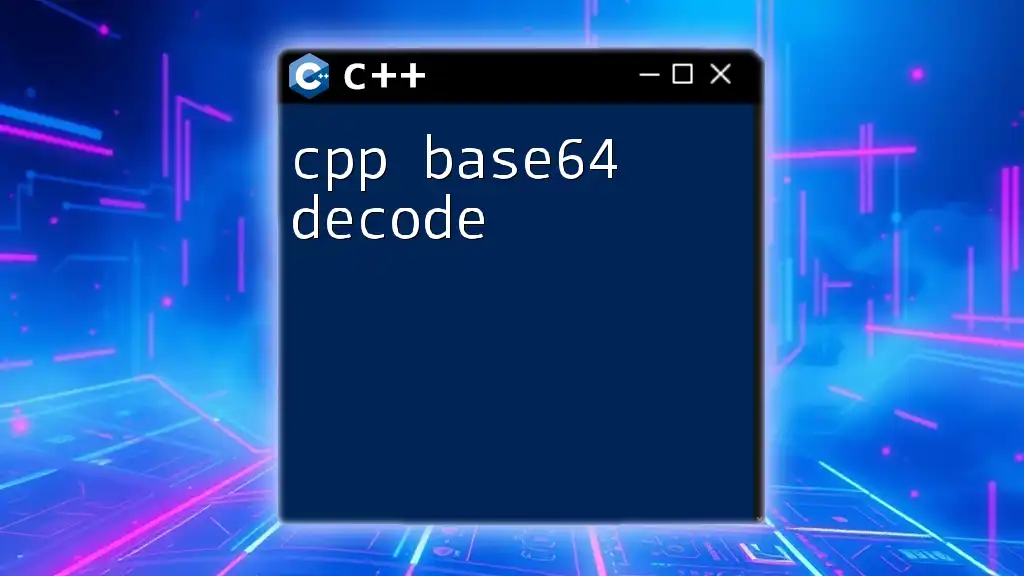
Benefits of Base64 Encoding
- Text Compatibility: Base64-encoded data is safe to transmit over text-based protocols without data loss.
- Data Integrity: Encoding ensures that binary data remains intact during conversion and transmission.
- Ease of Use: Base64 simplifies the handling and embedding of binary data in environments that prefer text.
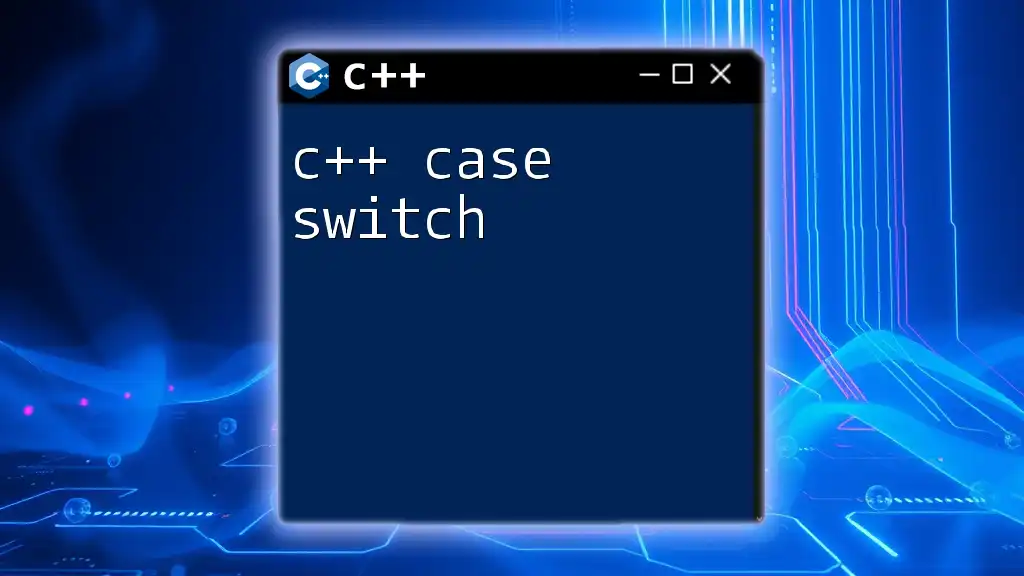
Setting Up Your C++ Environment
Prerequisites for C++ Base64 Implementation
A solid understanding of basic C++ concepts is necessary, along with familiarity with string handling and binary data processing. Being comfortable with compiling and running C++ code is essential.
Choosing the Right Libraries
While you can implement Base64 encoding and decoding manually, leveraging existing libraries can save you a significant amount of time. Some popular libraries for Base64 in C++ include:
- Base64 by René Nyffenegger: A widely cited implementation that is straightforward and easy to use.
- Boost.Beast: Part of the Boost libraries, offering powerful utilities for network programming that includes Base64 functionality.
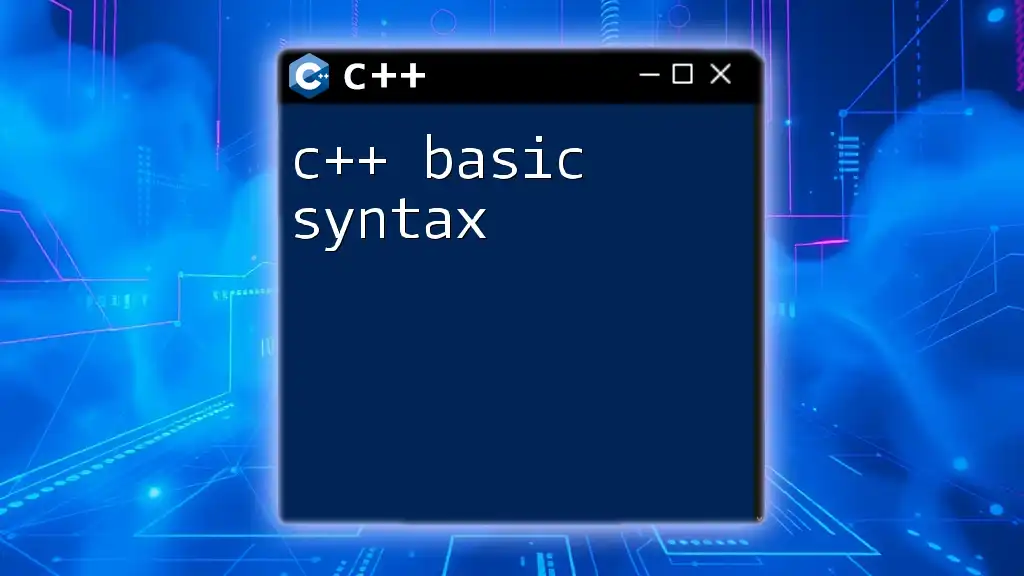
Base64 Encode in C++
Basic Concept of Base64 Encoding in C++
Base64 encoding is essential for converting binary data into a character string suitable for text-based systems. Whether you're working with images, audio files, or any binary data, you can effectively use Base64 to encode this data before transmission or storage.
Code Example: Simple Base64 Encoding
Here's a simple implementation of Base64 encoding in C++:
#include <iostream>
#include <string>
#include <vector>
static const std::string base64_chars =
"ABCDEFGHIJKLMNOPQRSTUVWXYZ"
"abcdefghijklmnopqrstuvwxyz"
"0123456789+/";
std::string base64_encode(const std::string &in) {
std::string out;
int val = 0, valb = -6;
for (unsigned char c : in) {
val = (val << 8) + c;
valb += 8;
while (valb >= 0) {
out.push_back(base64_chars[(val >> valb) & 0x3F]);
valb -= 6;
}
}
if (valb > -6) out.push_back(base64_chars[((val << 8) >> valb) & 0x3F]);
while (out.size() % 4) out.push_back('=');
return out;
}
int main() {
std::string input = "Hello, World!";
std::string encoded = base64_encode(input);
std::cout << "Encoded: " << encoded << std::endl;
return 0;
}
Explanation of the Encoding Logic
- Functionality: The `base64_encode` function takes a string input and processes it to produce a Base64-encoded string. The algorithm builds the encoded string by using bitwise operations to pack data into appropriate Base64 characters.
- Padding: If the final segment contains fewer than 24 bits, the code adds the necessary padding (`=`) to the output string to align it to a multiple of four characters.
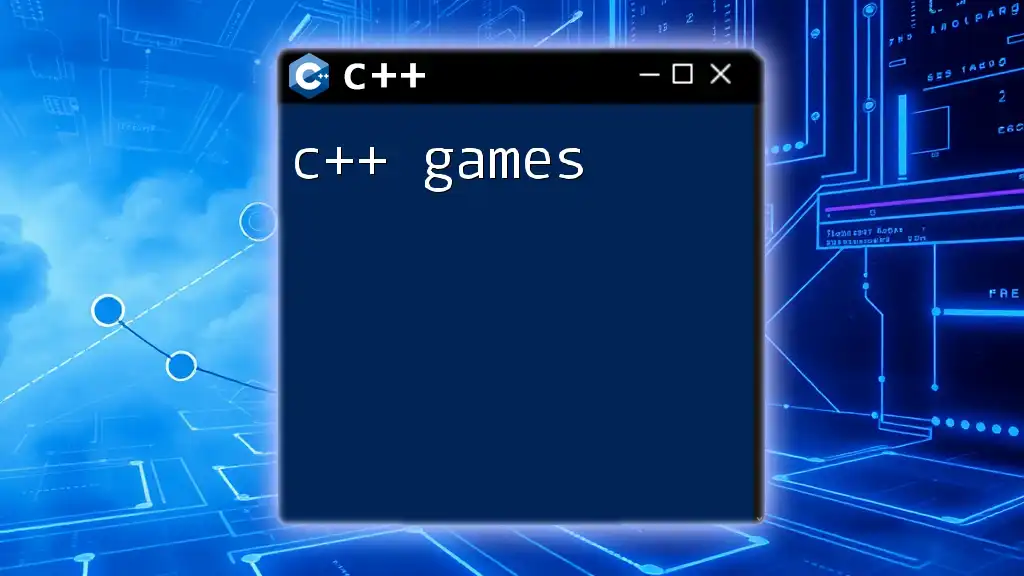
Base64 Decode in C++
Basic Concept of Base64 Decoding in C++
Decoding Base64 encoded data is critical when you need to retrieve the original binary data. This process reverses the encoding steps, converting the Base64 string back into its original form.
Code Example: Simple Base64 Decoding
The following code snippet demonstrates how to decode a Base64 string back into its original form:
#include <iostream>
#include <string>
#include <vector>
#include <stdexcept>
static const std::string base64_chars =
"ABCDEFGHIJKLMNOPQRSTUVWXYZ"
"abcdefghijklmnopqrstuvwxyz"
"0123456789+/";
std::string base64_decode(const std::string &in) {
std::vector<int> T(256, -1);
for (int i = 0; i < 64; i++) T[base64_chars[i]] = i;
int val = 0, valb = -8;
std::string out;
for (unsigned char c : in) {
if (T[c] == -1) break;
val = (val << 6) + T[c];
valb += 6;
if (valb >= 0) {
out.push_back(char((val >> valb) & 0xFF));
valb -= 8;
}
}
return out;
}
int main() {
std::string input = "SGVsbG8sIFdvcmxkIQ=="; // Base64 for "Hello, World!"
std::string decoded = base64_decode(input);
std::cout << "Decoded: " << decoded << std::endl;
return 0;
}
Explanation of the Decoding Logic
- Functionality: The `base64_decode` function decodes a Base64 string by translating each character back to its original binary form. It utilizes a lookup table to map Base64 characters back to their corresponding binary values.
- Error Handling: If any character in the input is invalid, the decoding process safely exits, returning an empty string.
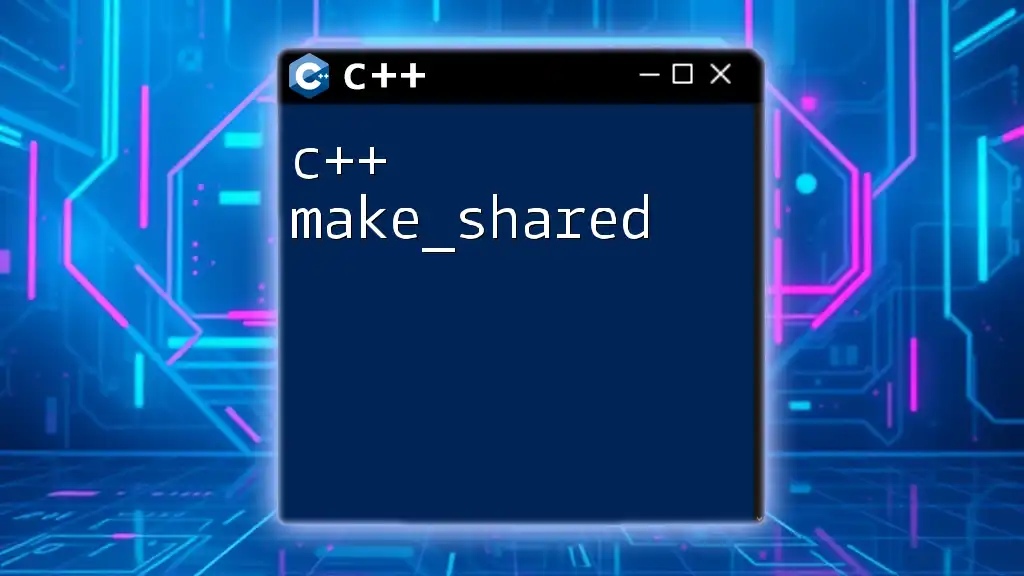
Common Use Cases for Base64 in C++
Encoding and Decoding Files
Base64 encoding and decoding are effective for file management. For example, when downloading images or audio files from a web service, you may need to receive the data in Base64 format and decode it for local storage. Best practices include preparing a robust implementation that handles larger files efficiently and manages memory effectively.
Networking: Sending and Receiving Data
In the realm of web APIs, data integrity is paramount. Base64 ensures that binary objects such as images, audio, or other binary formats remain intact during serialization and deserialization. Common frameworks and utilities, such as JSON serialization libraries, can readily handle Base64 strings in their constructs, enhancing application interoperability.
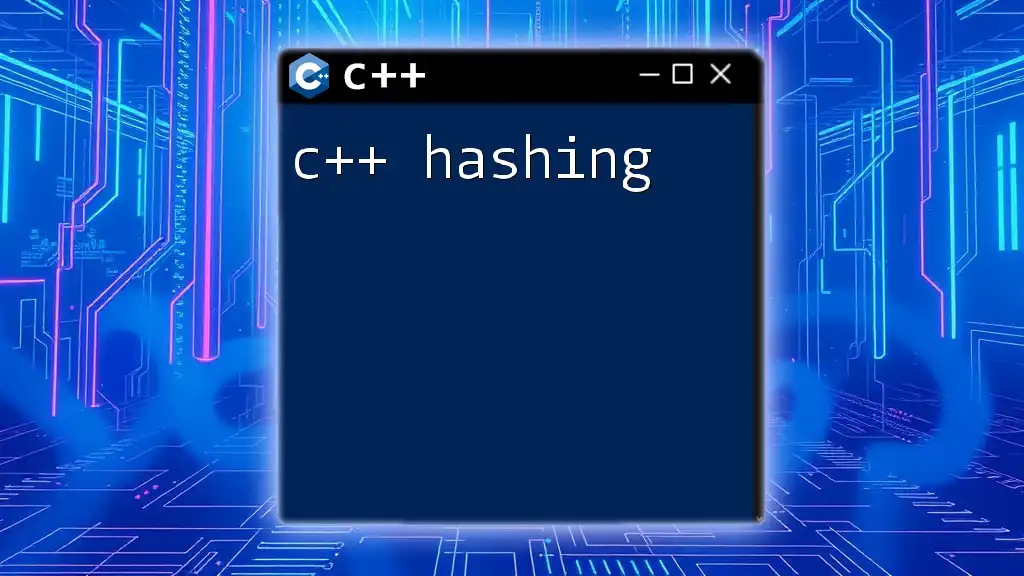
Recap of C++ Base64 Encoding and Decoding
C++ Base64 encoding and decoding are crucial skills for any developer working with binary data interchange. By understanding the mechanics behind Base64, you can ensure safe data transmission, maintain compatibility with text-processing systems, and avoid data corruption.
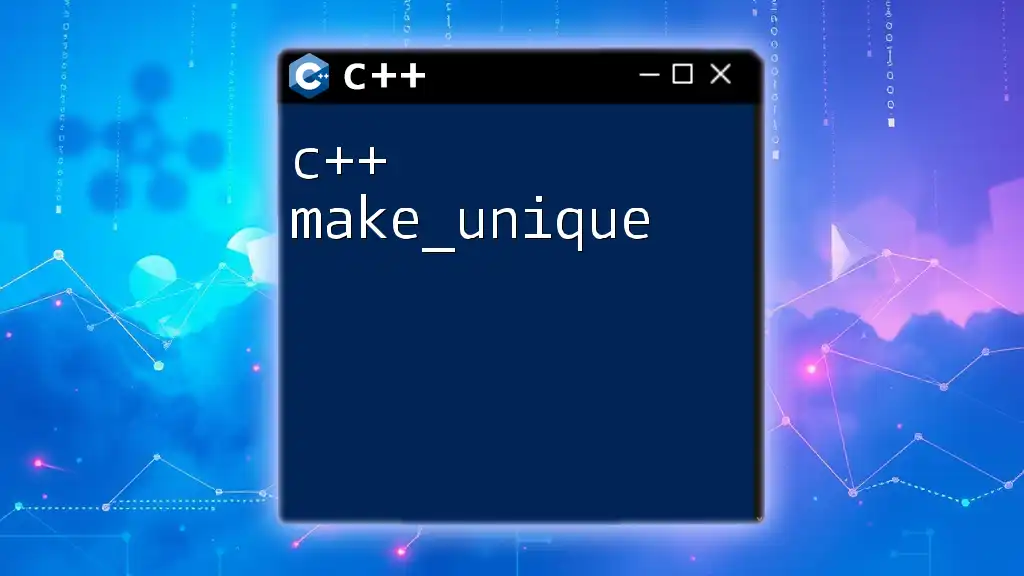
Additional Resources for Further Learning
To further deepen your understanding of Base64 encoding and decoding in C++, consider the following resources:
- RFC 4648: The complete specification about Base64 and its variants.
- C++ Standard Library Documentation: To explore additional data-handling functionalities.
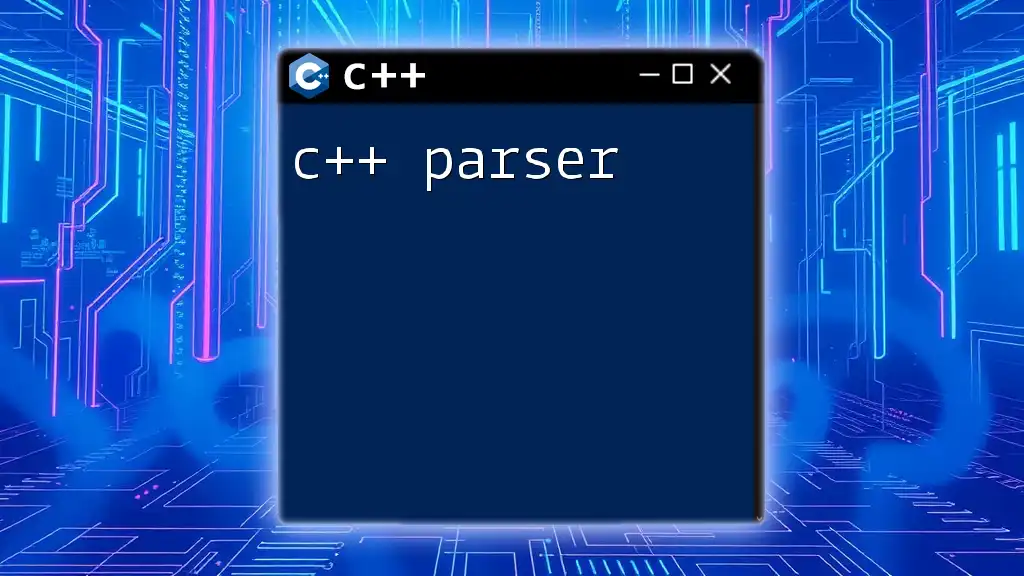
Call to Action
Try implementing the examples provided above in your own projects! Experiment with encoding various types of binary data and explore their applications in real-world scenarios. The knowledge of Base64 will prove invaluable as you enhance your C++ proficiency.