A C++ base class serves as a blueprint for derived classes, allowing them to inherit attributes and behaviors, promoting code reuse and a hierarchical relationship among classes.
Here's a simple example of a base class and a derived class in C++:
class Base {
public:
void display() {
std::cout << "Base class display function called." << std::endl;
}
};
class Derived : public Base {
public:
void show() {
std::cout << "Derived class show function called." << std::endl;
}
};
Understanding C++ Base Classes
Definition of Base Class
A base class in C++ is a class that provides common properties and behaviors that can be inherited by other classes, known as derived classes. This is a fundamental concept in object-oriented programming (OOP) that emphasizes reusability and organization of code. A base class acts as a blueprint from which other classes can derive and extend their functionality.
Importance of Base Classes
Base classes are essential because they enable:
- Code Reuse: Instead of rewriting common functionalities, developers can define them once in a base class and reuse them in derived classes.
- Polymorphism: Base classes can declare virtual functions that derived classes can override, enabling dynamic method resolution.
- Encapsulation: Base classes encapsulate data and functionalities, providing a structured approach to programming.
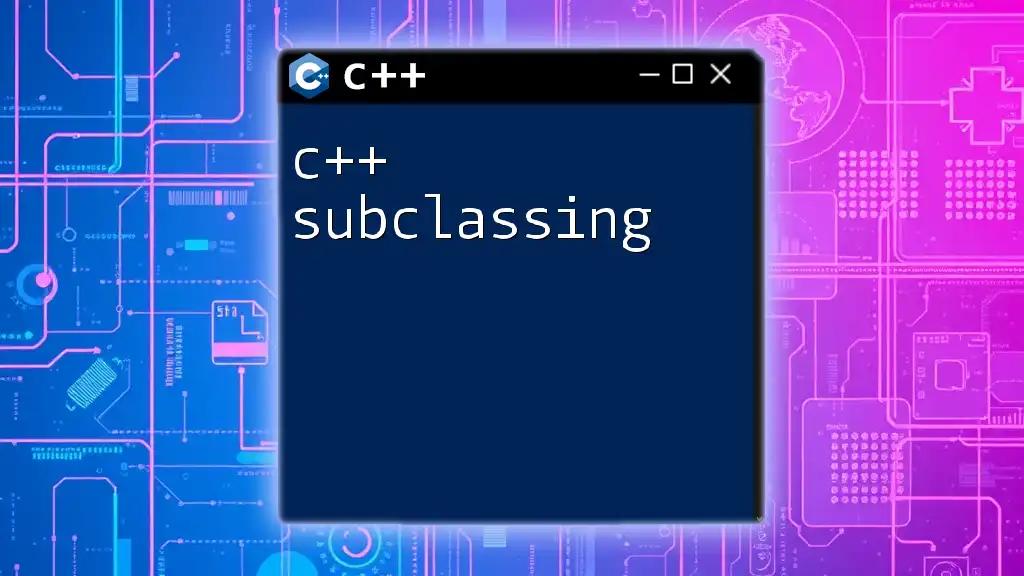
Understanding Base Class and Derived Class
What is a Derived Class?
A derived class is a class that inherits properties and behaviors from one or more base classes. Derived classes can extend or modify the functionality of the base class to fit specific needs.
Inheritance in C++
Inheritance is a key feature of OOP that allows a derived class to inherit attributes and methods from a base class. Types of inheritance in C++ include:
- Public Inheritance: Public and protected members of the base class remain accessible to the derived class. This is the most commonly used inheritance type.
- Protected Inheritance: Public and protected members of the base class become protected in the derived class.
- Private Inheritance: All members of the base class become private in the derived class, restricting access.

Creating a Base Class in C++
Syntax and Structure
Creating a base class entails defining it with the required members. For example:
class Base {
public:
void display() {
std::cout << "Base Class Display" << std::endl;
}
};
In this code snippet, we define a simple base class called `Base` with a public member function `display`. This function can be called by any derived class or any object of `Base`.
Members of a Base Class
Base classes can have different types of members:
- Member Variables: These can hold the state of an object created from the base class.
- Member Functions: These define the behaviors that the object can accomplish.
For example:
class Base {
public:
int value;
void setValue(int val) {
value = val;
}
void displayValue() {
std::cout << "Value: " << value << std::endl;
}
};
In this example, `Base` has a member variable `value` and two member functions to set and display its value.

Creating a Derived Class
Syntax for Derived Class
To create a derived class that inherits from a base class, the syntax is straightforward:
class Derived : public Base {
public:
void show() {
std::cout << "Derived Class Show" << std::endl;
}
};
Here, the `Derived` class inherits publicly from the `Base` class. This allows the derived class to access the public and protected members of the base class.
Inheritance Types
- Public Inheritance:
class Derived : public Base { };
- Protected Inheritance:
class Derived : protected Base { };
- Private Inheritance:
class Derived : private Base { };
Each inheritance type determines the accessibility of base class members in derived classes.

Accessing Base Class Members from a Derived Class
Public Members
Public members of a base class remain accessible to derived classes.
Protected Members
Protected members can be accessed directly by derived classes, promoting an object-oriented approach while still maintaining some encapsulation.
Private Members
Private members of the base class cannot be accessed directly from the derived class. If you need access, you can provide protected or public getter/setter methods in the base class.

Polymorphism and Virtual Functions
Understanding Polymorphism
Polymorphism allows objects to be treated as instances of their parent class, primarily through the use of virtual functions, which enables a class to override method implementations in derived classes.
Virtual Functions in Base Classes
To create a function that can be overridden in the derived class, the base class should declare that function as `virtual`:
class Base {
public:
virtual void display() {
std::cout << "Displaying Base Class" << std::endl;
}
};
class Derived : public Base {
public:
void display() override {
std::cout << "Displaying Derived Class" << std::endl;
}
};
In this code, both `Base` and `Derived` classes define a `display` function. The `Derived` class’s function overrides the `Base` class’s function, thus demonstrating polymorphism.
Dynamic Binding
Dynamic binding occurs when the program determines which method to invoke at runtime based on the object type. This provides flexibility in program design, allowing for efficient code execution.
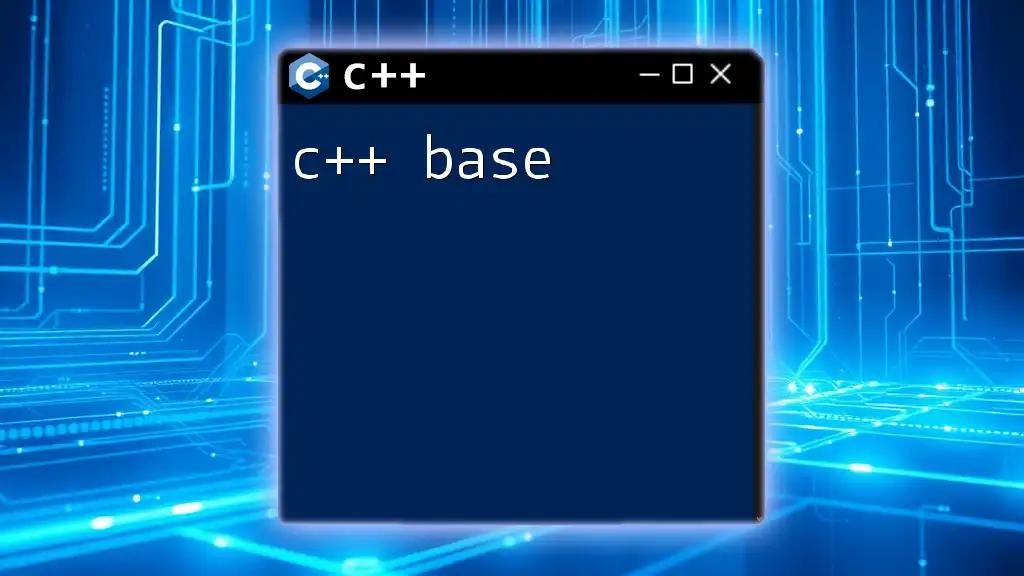
Abstract Base Classes and Interfaces
What is an Abstract Base Class?
An abstract base class is a class that cannot be instantiated directly and usually contains at least one pure virtual function. This enforces derived classes to implement certain functionalities.
Example of an abstract base class:
class AbstractBase {
public:
virtual void pureFunction() = 0; // Pure virtual function
};
Why Use Abstract Base Classes?
Abstract base classes are used to define interfaces and create a structured hierarchy in which derived classes must implement specific methods, leading to enhanced design patterns and code organization.

Best Practices in Using Base Classes
Design Principles
When working with base classes, consider following SOLID principles:
- Single Responsibility Principle: Each class should have one responsibility.
- Open/Closed Principle: Classes should be open for extension but closed for modification.
Code Reusability and Maintainability
Writing effective base classes is crucial for code reusability. Make sure:
- To define clear and concise interfaces in base classes.
- To provide documentation to help users understand the class functionalities.
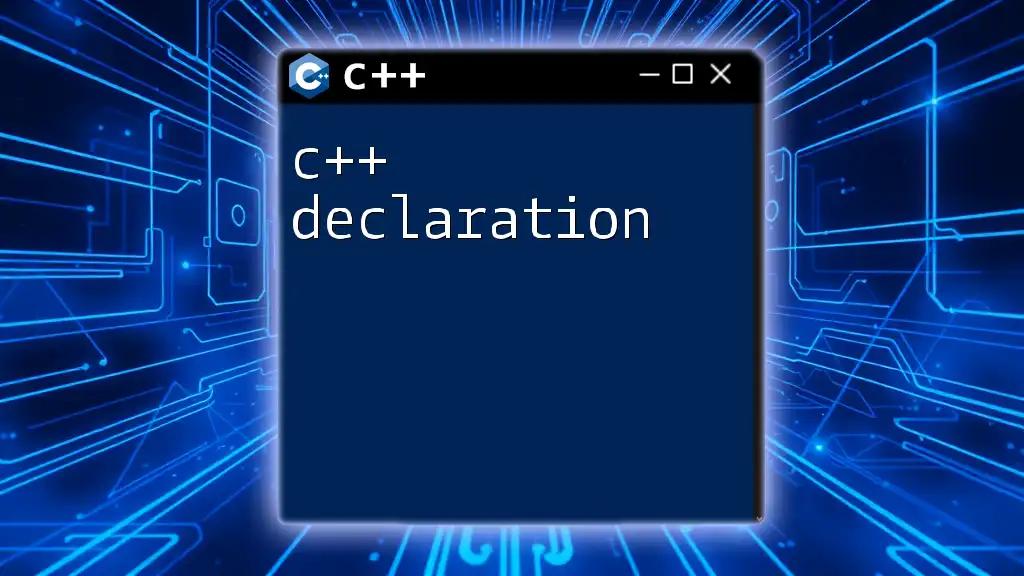
Conclusion
C++ base classes serve as the foundation of a well-structured object-oriented design, enabling code reusability, encapsulation, and polymorphism. By understanding and applying the principles discussed, developers can create more organized and maintainable code. Engaging in practical exercises will further solidify the knowledge gained on C++ base classes.
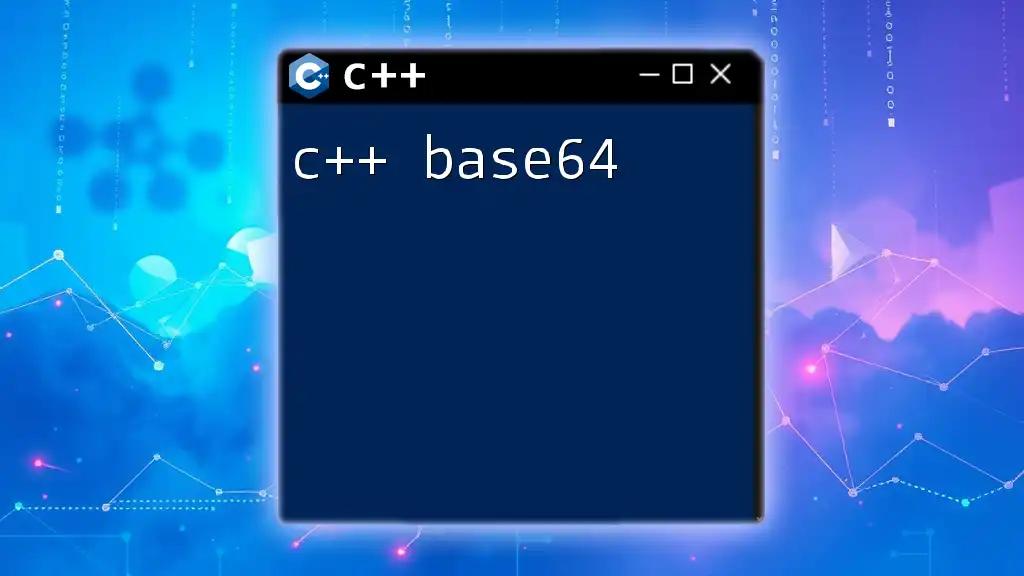
Additional Resources
For those looking to deepen their understanding of C++, consider exploring books, online tutorials, and documentation that delve into these concepts. Engaging with community resources can also provide invaluable insights and examples to enhance your skills.