In C++, you can obtain the name of a class at runtime using the `typeid` operator along with the `name()` method from the `type_info` class, as shown in the following code snippet:
#include <iostream>
#include <typeinfo>
class MyClass {};
int main() {
MyClass obj;
std::cout << "Class name: " << typeid(obj).name() << std::endl;
return 0;
}
Understanding Class Names in C++
What are Class Names?
In C++, class names serve as identifiers for classes defined in your programs. These names are essential as they allow programmers to instantiate objects and define types in their code. Understanding how to manage and retrieve class names can enhance your coding efficiency and provide clarity when debugging or logging.
The Role of RTTI (Run-Time Type Information)
Run-Time Type Information (RTTI) is a powerful feature in C++ that allows a program to determine the type of an object at runtime. This is particularly important in the context of polymorphism, where the specific type of an object may need to be known to perform type-safe operations. RTTI provides two primary utilities: `typeid` for type identification and dynamic casting.
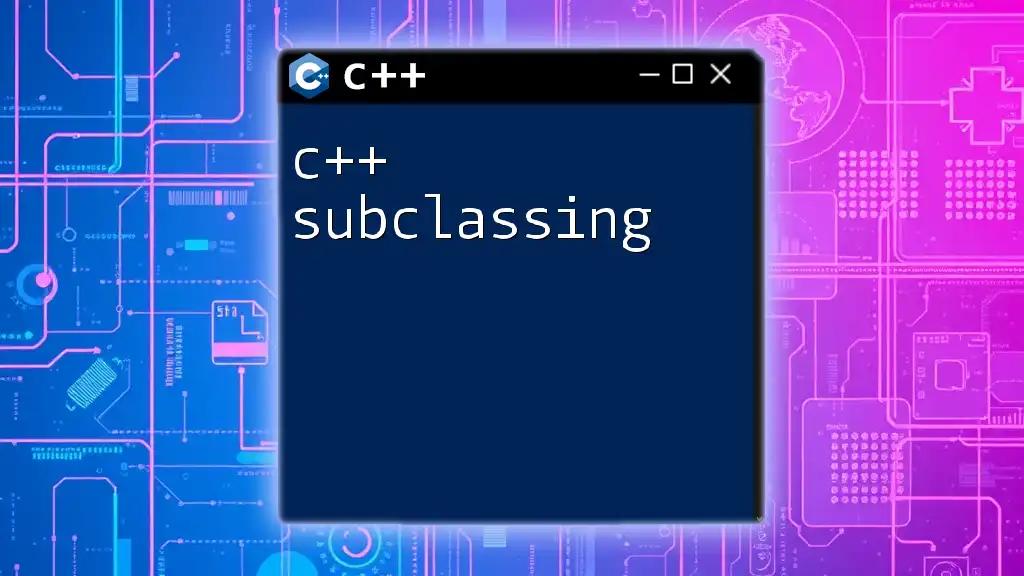
Methods to Retrieve Class Names in C++
Using `typeid`
Overview of typeid The `typeid` operator is a fundamental feature of C++ that retrieves the type information of an expression. This makes it straightforward to access the class name of an object at runtime.
Syntax and Usage Here’s how you can use `typeid` to get a class name:
#include <iostream>
#include <typeinfo>
class MyClass {};
int main() {
MyClass obj;
std::cout << "Class Name: " << typeid(obj).name() << std::endl;
return 0;
}
When you run the above code, it prints the class name to the console. However, it's important to note that the output may not be human-readable right away, as the name returned can be mangled depending on the compiler used.
Using `type_info`
Introduction to type_info The `type_info` class encapsulates the information provided by `typeid`. This class contains methods and data to access type information, including the class name.
Accessing Class Names You can access the class name directly using `type_info` like this:
class MyClass {};
int main() {
MyClass obj;
const std::type_info& ti = typeid(obj);
std::cout << "Class Name: " << ti.name() << std::endl;
return 0;
}
The advantage of using `type_info` is that it exposes more functionality, such as comparing types, which can be beneficial in complex applications.
Custom Function for Class Name Retrieval
Creating a Template Function While `typeid` and `type_info` provide a way to retrieve class names, creating a custom function can enhance your code's readability and reusability.
Code Example Let's create a template function that provides class name retrieval:
#include <iostream>
#include <typeinfo>
template <typename T>
std::string getClassName(const T& obj) {
return typeid(obj).name();
}
class MyClass {};
int main() {
MyClass obj;
std::cout << "Class Name: " << getClassName(obj) << std::endl;
return 0;
}
This function allows you to use it with any object type, making it a versatile tool for your C++ applications.
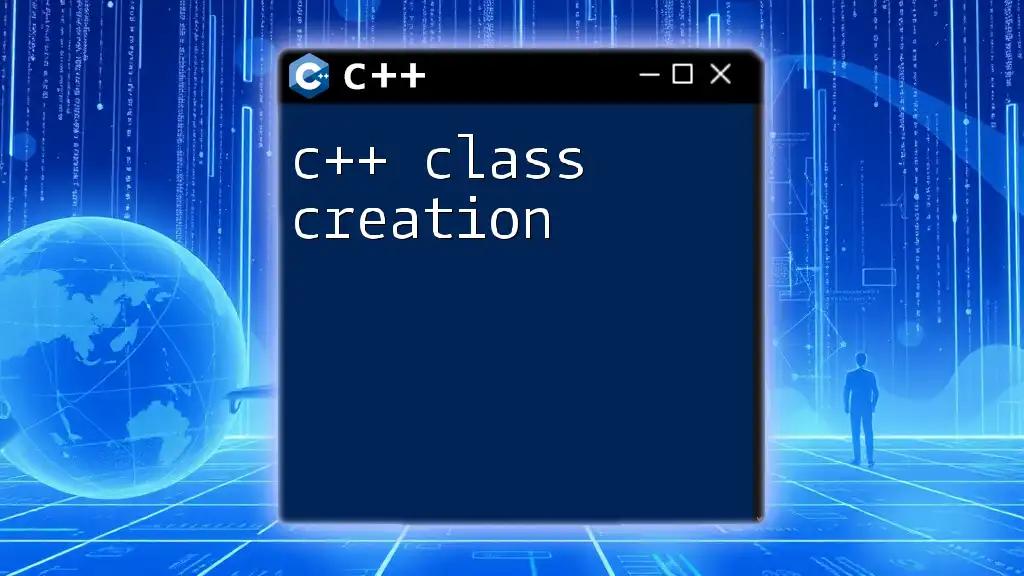
Limitations and Caveats
Compiler Differences
One challenge when using `typeid` is that the output can vary across different compilers. For example, the `gcc` compiler may produce a different representation of the class name compared to `MSVC`. Always test and be aware of how each compiler formats the output to ensure consistency in your applications.
Handling Inheritance
When dealing with inheritance, retrieving class names can yield different results depending on whether you're looking at the base or derived class.
Example:
class Base {};
class Derived : public Base {};
Base* b = new Derived();
std::cout << "Derived Class Name: " << typeid(*b).name() << std::endl;
delete b;
In this case, using `typeid(*b)` will return the name of `Derived`, highlighting the power of polymorphism. Understanding this behavior is crucial for accurately interpreting class names in a hierarchy.

Practical Applications of Getting Class Names
Debugging
Retrieving class names can significantly simplify the debugging process. When exceptions occur or unexpected behaviors are detected, knowing the specific class can help diagnose the issue quickly. For instance, if you log the class names when debugging, it can give insights into what objects are at play, leading to a faster resolution of issues.
Logging
Integrating class names into log statements enhances the informational value of logs, allowing developers to track the flow of the application more effectively. Here’s how you could log class names during object instantiation:
#include <iostream>
#include <typeinfo>
class MyClass {
public:
MyClass() {
std::cout << "Created instance of: " << typeid(*this).name() << std::endl;
}
};
int main() {
MyClass obj;
return 0;
}
This auto-logging feature can be quite beneficial for monitoring application behavior in production environments.

Conclusion
In this guide, we explored various techniques to c++ get class name, including utilizing `typeid`, `type_info`, and creating custom functions. The importance of class names is underscored in debugging and logging scenarios, highlighting the practical advantages of retrieving type information at runtime. With the knowledge gained, you are encouraged to implement these concepts in your C++ applications, enhancing clarity and efficiency in your coding practices.

Additional Resources
To deepen your understanding, consider exploring official C++ documentation, such as [cppreference](https://en.cppreference.com), and recommended C++ books or online courses that delve into RTTI and other advanced C++ features.