In C++, `typename` is used to declare a type for templates, enabling the compiler to distinguish between types and non-type parameters within template definitions.
template <typename T>
void displayType(T value) {
std::cout << "The type of value is: " << typeid(value).name() << std::endl;
}
What is typename in C++?
The keyword typename in C++ serves a crucial function in both template programming and in defining type names in contexts where type dependent statements require them. It clarifies when a particular name in a template context should be treated as a type rather than a value or function. Understanding the nuances of typename is essential for effective and efficient coding in modern C++.
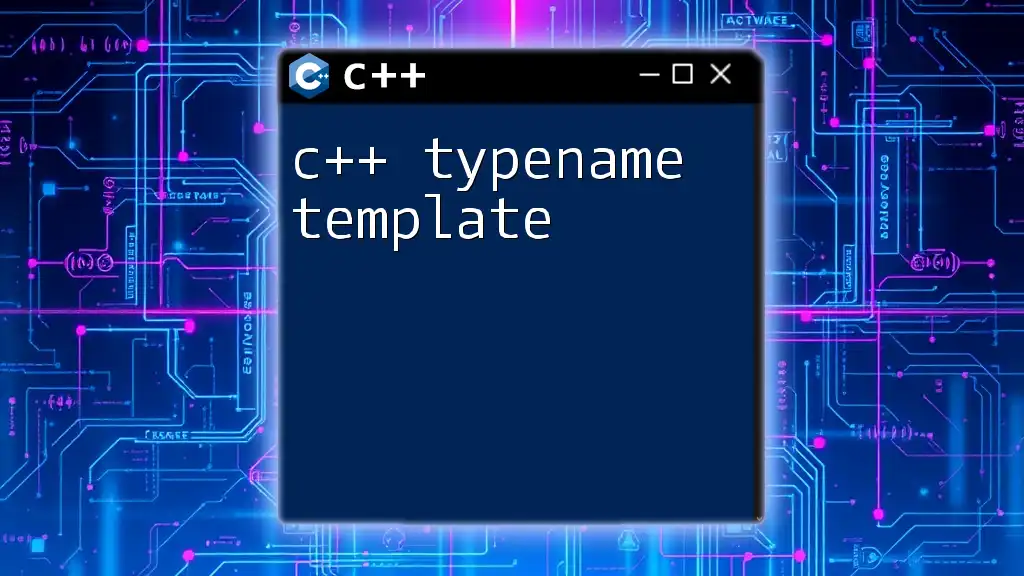
Understanding the Basics of C++ Typename
What is a Type Name in C++?
In C++, a type name refers to a name that identifies a type, which could be a fundamental type (like `int` or `float`) or a user-defined type (like a class or struct). The term is essential when dealing with generic programming, especially in templates.
The major distinction involves the recognition of the context in which a name appears so the compiler can correctly resolve it. For instance, the keyword typename helps the compiler understand that a specified name corresponds to a type.
Syntax of typename c++
The syntax for using typename is straightforward. You precede the name of the type with typename when it exists within a template declaration.
Here's a simple illustration:
template <typename T>
class Wrapper {
public:
T value;
Wrapper(T val) : value(val) {}
};
In this example, T is a type parameter defined using typename that can be replaced with any data type when an instance of the class is created.
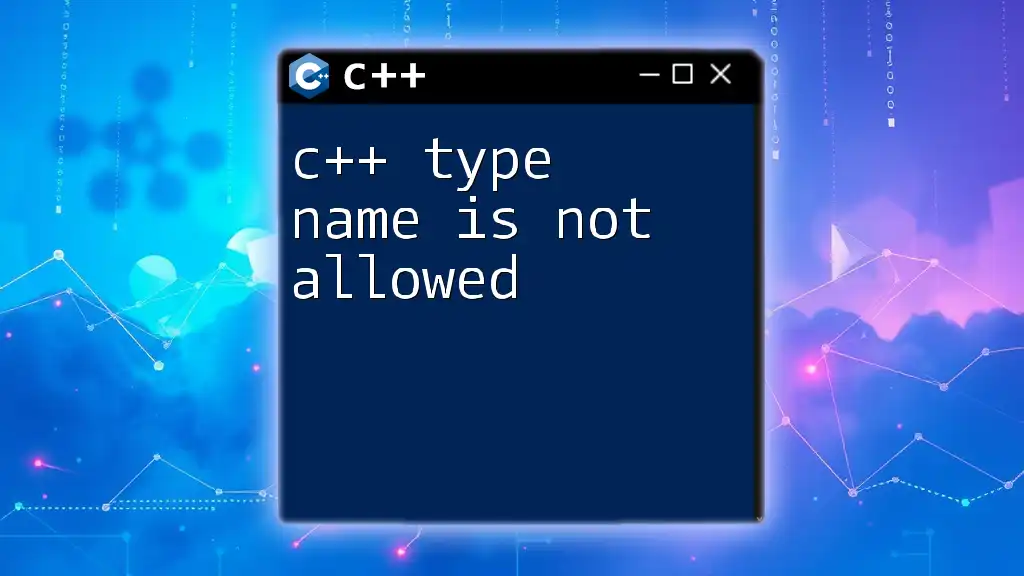
When to Use C++ typename
Template Programming
One of the primary uses of typename is in template programming. When you create a function or class template, you often want to define a placeholder for a type parameter that can be instantiated later with an actual type.
For example:
template <typename T>
void printValue(T value) {
std::cout << value << std::endl;
}
In this function template, T can be any type, such as `int`, `double`, or even user-defined types. The typename keyword indicates to the compiler that T should be treated as a type.
Dependent Types
When working with templates, you may encounter what are known as dependent types. These are types that depend on a template parameter. Here, using typename allows the compiler to understand that a specific name refers to a type within a template context.
Consider the following example:
template <typename T>
class MyClass {
public:
typename T::NestedType value; // T::NestedType is a dependent type
MyClass() : value() {}
};
In the code snippet above, typename specifies that T::NestedType is indeed a type, resolving ambiguity for the compiler.
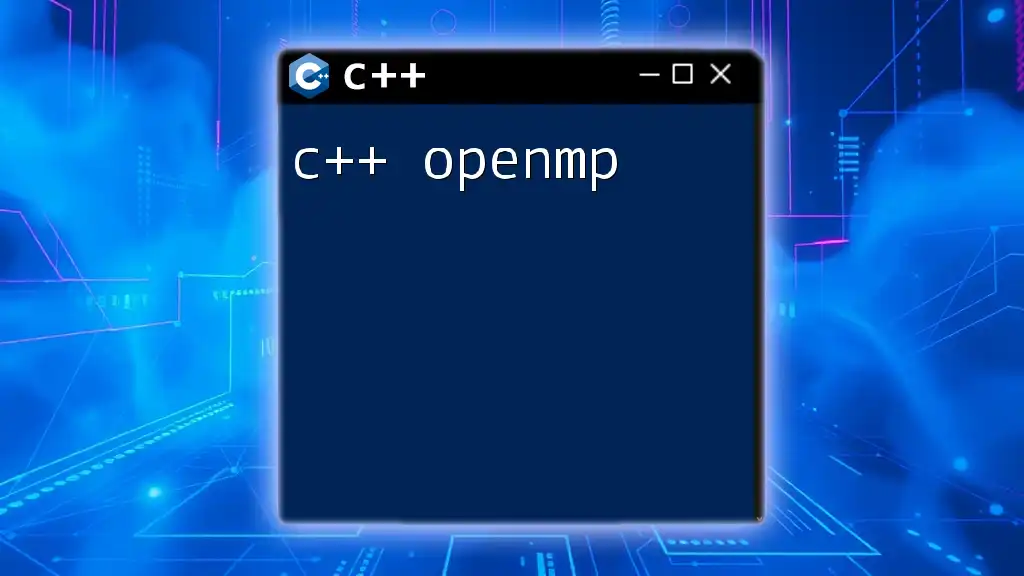
Examples of C++ Typename in Action
Example 1: Basic Usage of typename
template <typename T>
class Pair {
public:
T first;
T second;
Pair(T a, T b) : first(a), second(b) {}
};
In this example, typename allows the `Pair` class to hold two variables of any specified type. You can instantiate it as follows:
Pair<int> intPair(1, 2);
Pair<std::string> strPair("Hello", "World");
Example 2: Using typename with Standard Library
The typename keyword is also prevalent within C++ Standard Library templates, such as `std::vector`. Here’s a code snippet demonstrating its use:
#include <vector>
#include <iostream>
template <typename T>
void printVector(const std::vector<T>& vec) {
for (const auto& elem : vec) {
std::cout << elem << " ";
}
std::cout << std::endl;
}
This function template utilizes typename to indicate that T can represent any type within a `std::vector`.
Example 3: Nested Types and typename
Nested types within class templates can create additional complexity. Here’s how to handle it:
class Outer {
public:
class Inner {
public:
using Type = int;
};
};
template <typename T>
void func() {
typename T::Inner::Type value = 42; // Resolves ambiguity
}
In the above example, typename ensures that the compiler knows to treat T::Inner::Type as a type.
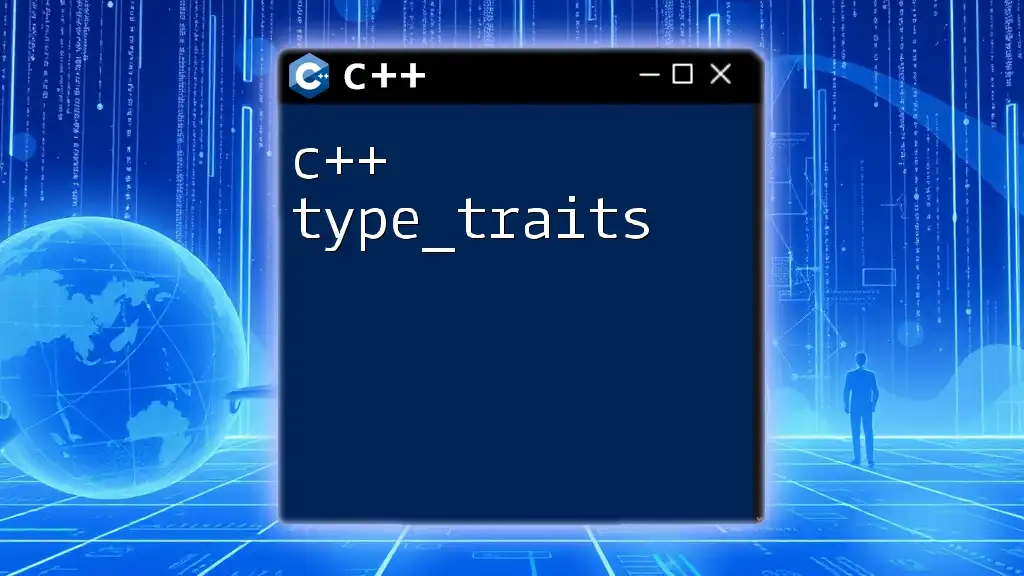
Advanced Uses of C++ Typename
typename vs class in Templates
C++ allows both typename and class to be used interchangeably when declaring template parameters. However, there are some nuanced differences. For instance:
template <class T>
class Example {};
template <typename U>
class Demo {};
Both snippets accomplish the same goal. However, typename is preferred when defining type parameters because it emphasizes that a type is factored into the generic definition, whereas class can imply something more.
C++11 and Beyond: Enhancements in Typename Usage
The introduction of C++11 added several new features that impact how we use typename. One noteworthy addition is decltype, which works with typename to deduce types dynamically.
Example:
template <typename T>
void useDecltype(T t) {
typename std::remove_pointer<decltype(t)>::type newObj; // Removes pointer to type
}
This example demonstrates how you can integrate decltype with typename for advanced type manipulation.
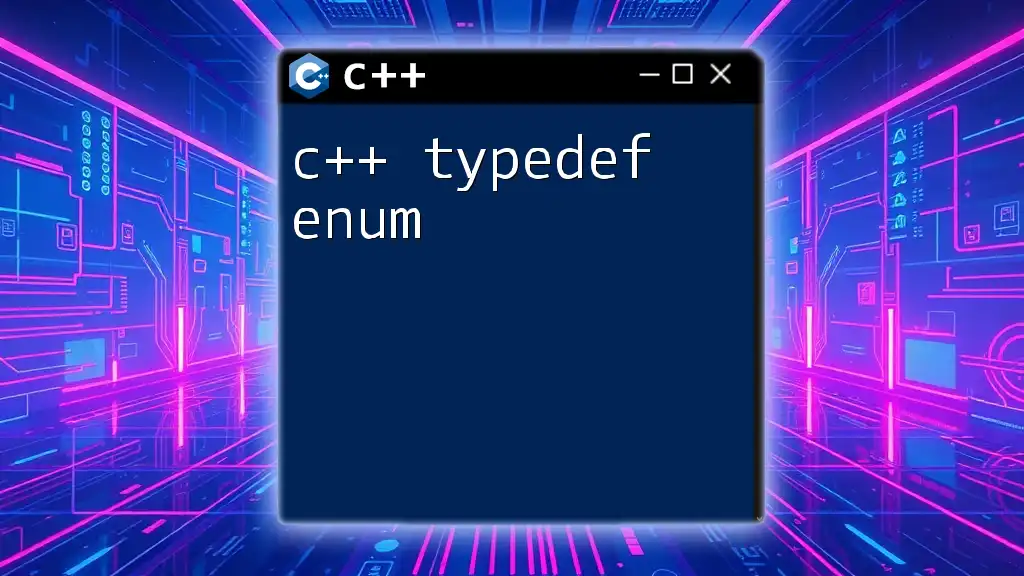
Common Pitfalls When Using C++ typename
Misunderstanding Dependent Contexts
One frequent mistake programmers make is failing to use typename in contexts requiring it, leading to compilation errors. For instance, not using typename when referring to dependent types can cause ambiguity.
Tip: Always remember to use typename when you're unsure if a name needs clarification for the compiler — this prevents confusion and errors down the line.
Performance Considerations
While the use of typename itself does not have significant performance implications, improper use can lead to non-optimized code due to misunderstanding template instantiation. Always ensure you are using typename correctly to maintain efficiency.
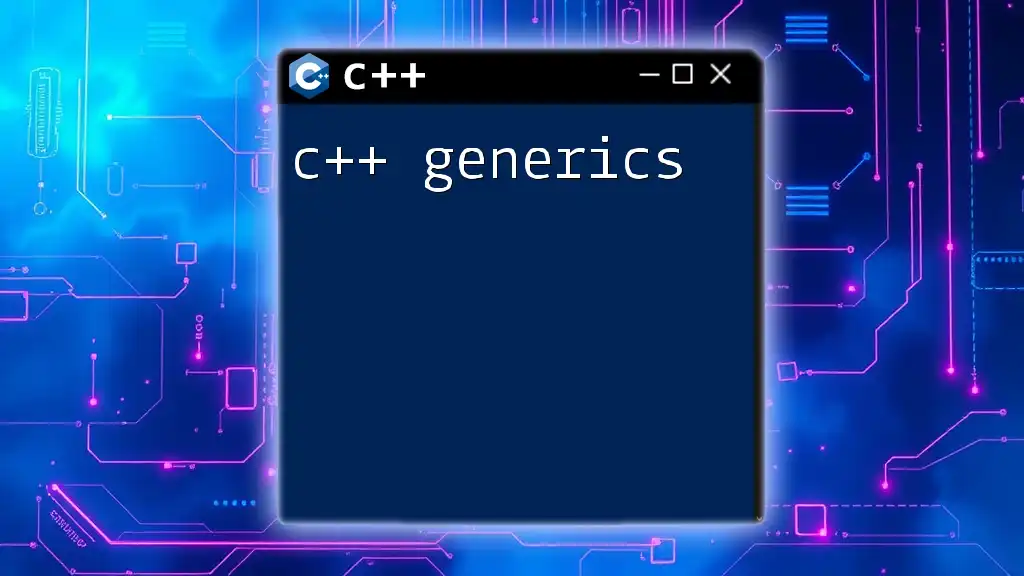
Conclusion
Understanding the role of typename in C++ is fundamental for writing robust and generic code. It not only clarifies the nature of types in different contexts but also enhances the flexibility and reusability of your code through templates. By mastering the use of typename, you can become proficient in leveraging C++’s powerful template features, making a significant improvement in your programming skills.
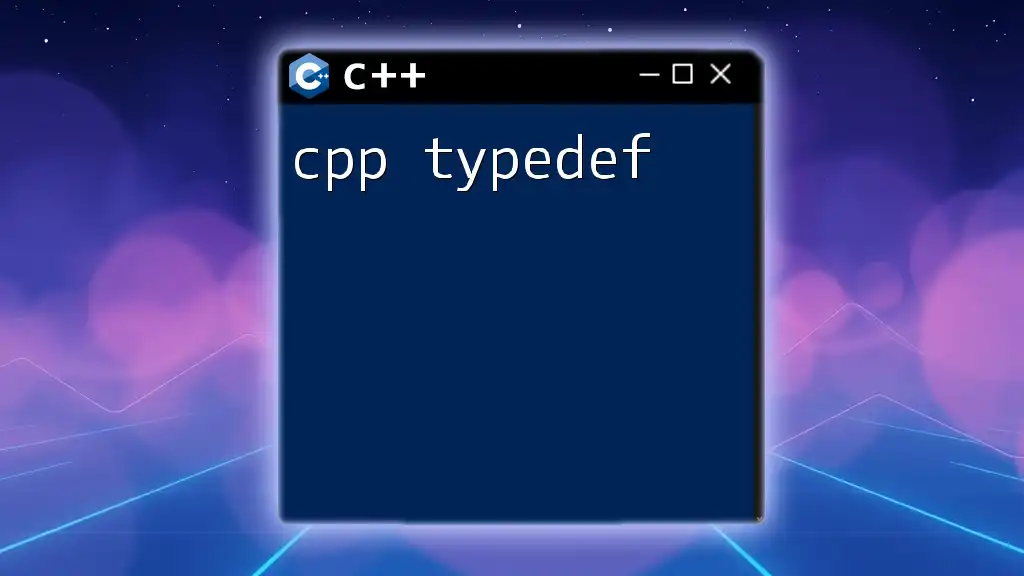
Additional Resources
To expand your knowledge further, consider exploring additional resources such as C++ textbooks, online coding platforms, or video tutorials on advanced template techniques, providing deeper insights and practical exposure to using typename effectively.