C++ games leverage the power of the C++ programming language for performance and efficiency, enabling developers to create high-quality, interactive experiences.
Here's a simple code snippet to create a basic C++ game loop:
#include <iostream>
int main() {
bool isRunning = true;
while (isRunning) {
std::cout << "Game is running... (Press Ctrl+C to exit)" << std::endl;
}
return 0;
}
Understanding C++ in Game Development
What is C++?
C++ is a high-performance programming language widely recognized for its flexibility and power, originally developed by Bjarne Stroustrup in the early 1980s. It is an extension of the C programming language, bringing with it features like object-oriented programming (OOP). These features allow developers to create complex systems and applications, making it an ideal choice for game development.
Why Choose C++ for Game Development?
C++ stands out in game development for several reasons. First, its performance is a significant advantage; C++ gives programmers tight control over memory and system resources, which is crucial for creating fast and efficient games. This control translates into improved frame rates and smoother gameplay experiences.
Second, C++ gives developers the ability to manage memory directly, which can lead to optimized performance when done correctly. Indeed, this manual memory management allows for finer control of resources, helping developers make the most out of limited hardware capabilities.
Finally, C++ is a cross-platform language, meaning games developed in C++ can run on various operating systems, including Windows, macOS, and Linux, without needing extensive rework.

Setting Up Your C++ Development Environment
Essential Tools and Software
To begin developing C++ games, you first need to set up your development environment. Integrated Development Environments (IDEs) such as Visual Studio, Code::Blocks, and CLion are popular choices, providing tools that streamline the coding process.
Understanding your compiler is also critical. Compilers like GCC, clang, and MSVC convert your C++ code into executable programs, each with unique capabilities and advantages. Choosing an appropriate compiler can significantly impact your game development experience.
Installing Required Libraries
For most game projects, using external libraries can save time and effort. Popular game graphics libraries include SDL (Simple DirectMedia Layer), SFML (Simple and Fast Multimedia Library), and OpenGL. Here’s how you can set up a simple SDL project:
#include <SDL.h>
// Initialize SDL
int main(int argc, char* args[]) {
SDL_Init(SDL_INIT_VIDEO);
SDL_Window* window = SDL_CreateWindow("Game", SDL_WINDOWPOS_UNDEFINED, SDL_WINDOWPOS_UNDEFINED, 640, 480, SDL_WINDOW_SHOWN);
// Main loop
SDL_Delay(3000); // Keep window open for 3 seconds
SDL_DestroyWindow(window);
SDL_Quit();
return 0;
}
This snippet shows the basic structure needed to create a window using SDL, establishing a starting point for a C++ game.
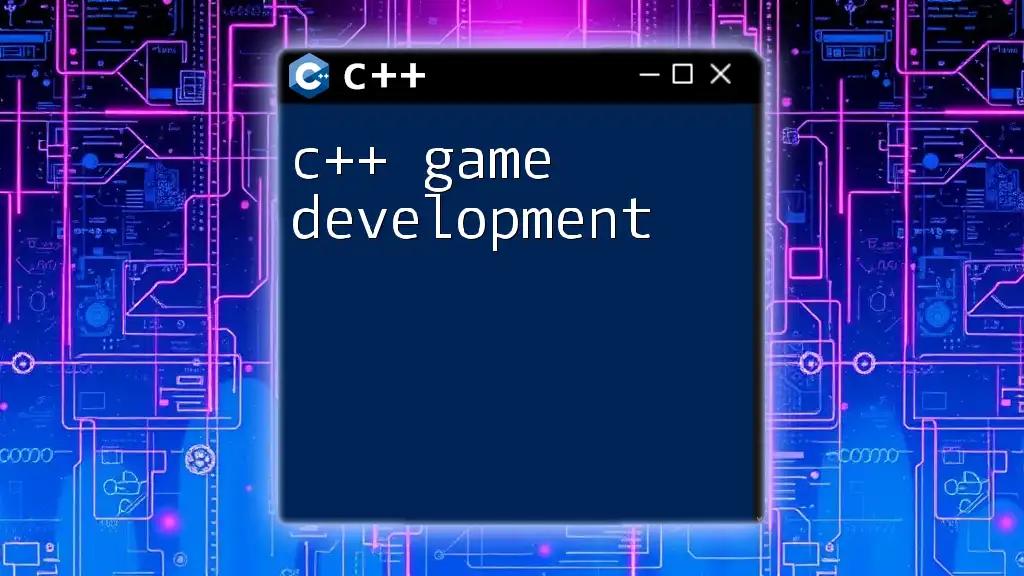
Game Development Concepts in C++
Game Loops
A game loop is the backbone of any game, constantly running to update and render the game state. This loop handles user input, processes game logic, and updates graphical output. Understanding how to implement a game loop is pivotal for any C++ game developer.
Here's a simplified view of what a game loop might look like:
while (isRunning) {
processInput();
updateGameLogic();
renderGraphics();
}
In this structure, `processInput` handles user commands, `updateGameLogic` calculates frame updates, and `renderGraphics` displays the game state, creating a continuous cycle that brings your game to life.
Object-Oriented Programming in Game Design
Object-Oriented Programming (OOP) allows developers to create complex systems by defining objects that represent various entities in a game. Using OOP principles like encapsulation and inheritance helps organize code efficiently.
For example, here's a basic class representing a game object:
class GameObject {
public:
void update();
void render();
private:
int positionX;
int positionY;
};
This class encapsulates the properties and methods of a game object, making it easy to instantiate various items in your game world while maintaining clean and modular code.
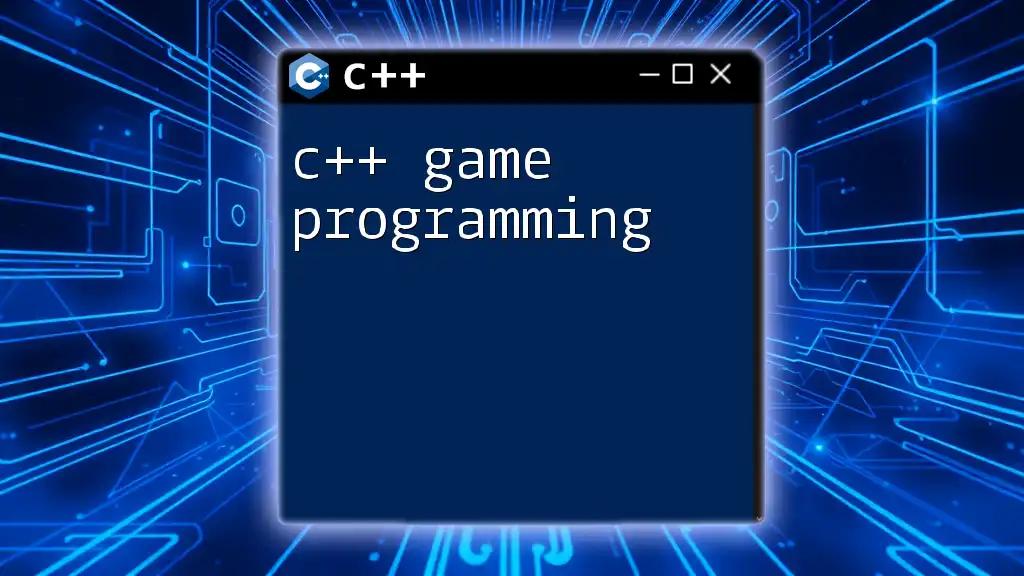
Building a Simple C++ Game
Game Concept
To exemplify what we’ve discussed, let’s consider building a simple Snake Game. This will help illustrate the application of C++ in a game development scenario.
Coding the Game
First, we’ll need to set up the game environment, initialize the snake, manage its movements, and detect collisions with the walls and itself.
When implementing game logic, focusing on movement and collision detection is critical. For example, using a simple conditional to move the snake might look like this:
if (keyboardInput == UP) {
snake.moveUp();
} else if (keyboardInput == DOWN) {
snake.moveDown();
}
This code checks keyboard input to adjust the direction of the snake accordingly.
Rendering Graphics
To make the game visually appealing, you can use SDL or SFML to render graphics. This process involves drawing the snake and any other game elements on the screen.
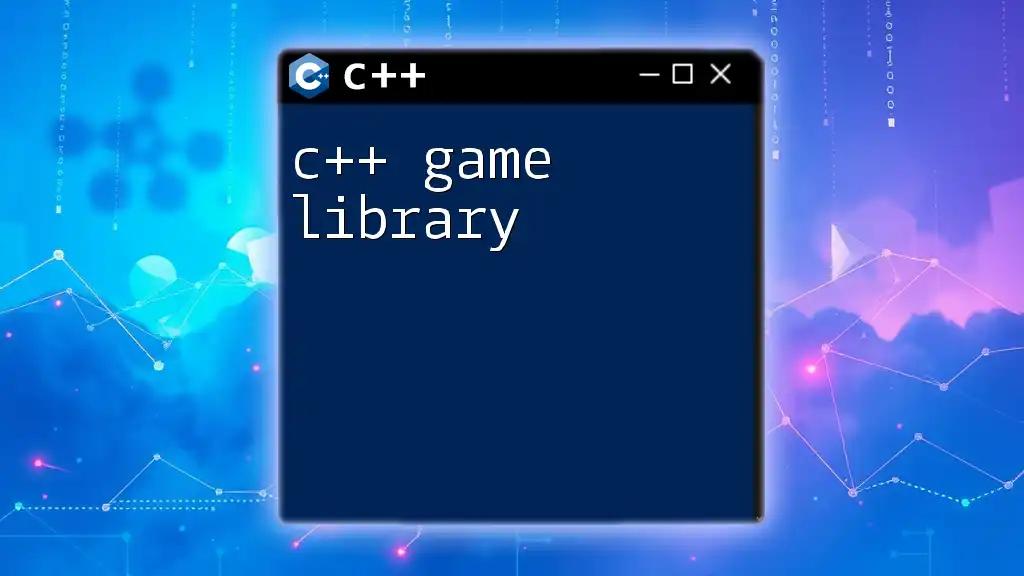
Extending Your C++ Game
Adding Audio and Sound Effects
Enhancing your game with audio can significantly improve user experience. Libraries like SDL_mixer allow games to play sound effects and background music easily.
Here’s an example of how to play a sound using SDL_mixer:
Mix_OpenAudio(44100, MIX_DEFAULT_FORMAT, 2, 2048);
Mix_Chunk* sound = Mix_LoadWAV("sound.wav");
Mix_PlayChannel(-1, sound, 0);
This code initializes audio, loads a sound file, and then plays it whenever needed.
Implementing User Interface
User interfaces (UIs) are crucial in providing players with the information they need. This could include score, health, or options. Implementing a basic menu might involve checking if a menu is active and rendering its options accordingly:
if (isMenuActive) {
// Render menu options
}
Creating an inviting UI can enhance gameplay and keep players engaged.
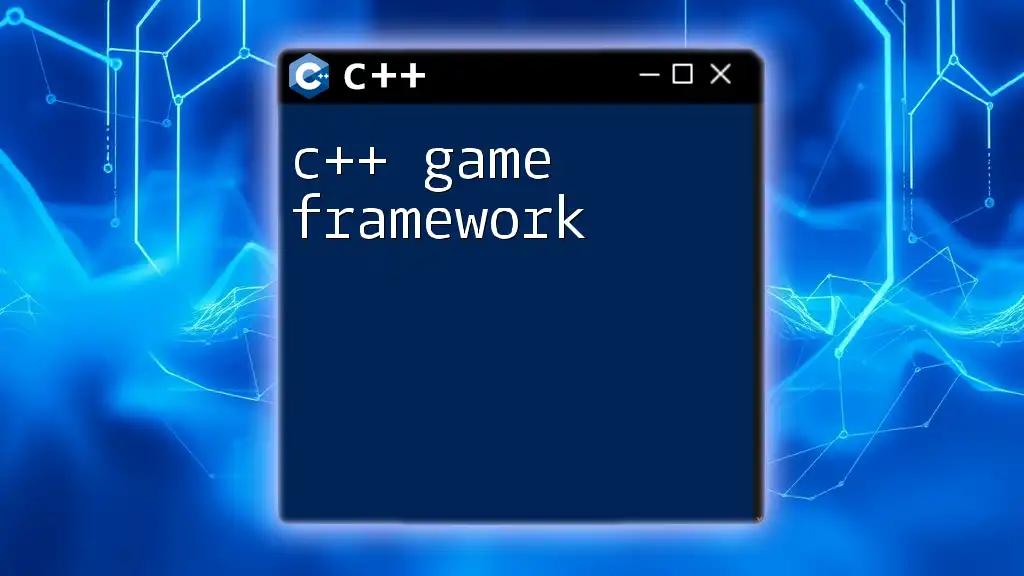
Debugging and Optimizing Your Game
Common Debugging Techniques
Debugging is an essential skill in software development. Embracing tools like gdb or built-in IDE debugging tools is fundamental for identifying and resolving issues within your code.
Logging is another valuable practice, allowing developers to track the game's behavior over time. Log significant events to understand how your game operates, facilitating easier troubleshooting and debugging.
Performance Optimization
Once the game is functioning, optimization becomes necessary. Profiling your C++ code is critical for identifying bottlenecks. Utilizing tools like Valgrind or Visual Studio Profiler can help in discerning where improvements can be made.
Optimization techniques may involve improving memory management and refining algorithms to make your game run smoother and faster. Effective optimization can greatly enhance gameplay and player satisfaction.
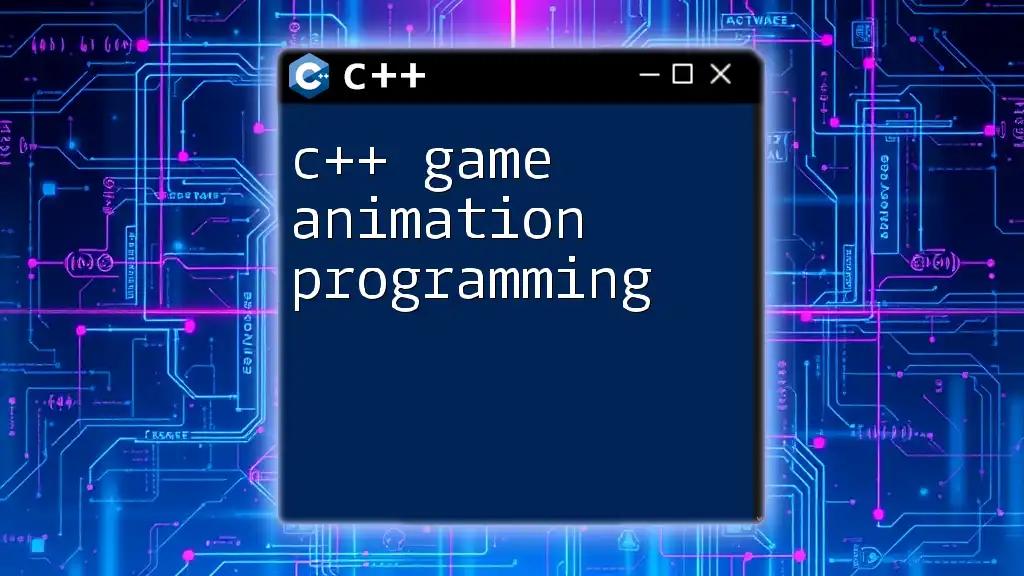
Resources for Continued Learning
Recommended Books
Expanding your knowledge is vital for mastering C++ game development. Some valuable reads include:
- “Game Programming Patterns” by Robert Nystrom: Focuses on design patterns specific to game development.
- “Beginning C++ Through Game Programming” by Michael Dawson: An excellent resource for beginners looking to learn C++ through practical game projects.
Online Courses and Tutorials
Numerous online platforms provide comprehensive courses on game development. Websites like Udemy, Coursera, and various YouTube channels can help facilitate your journey into C++ gaming.

Conclusion
In conclusion, programming C++ games requires a blend of creativity, technical skills, and knowledge of best practices. By harnessing the power of C++, developers can create engaging and high-performing games suited for various platforms. Continuing to learn and experiment with new techniques will set you on the path to becoming a successful game developer.

Call to Action
We encourage you to explore further into C++ game development. Sign up for our upcoming courses and tutorials to deepen your understanding and enhance your skills in C++ commands and game design!