In C++, the Gaussian function is often used in mathematical computations and can be represented as `f(x) = a * exp(-((x - b) * (x - b)) / (2 * c * c))`, where `a` is the height, `b` is the position of the center of the peak, and `c` controls the width of the bell curve.
Here’s a simple code snippet illustrating how to implement a Gaussian function in C++:
#include <iostream>
#include <cmath>
double gaussian(double x, double a, double b, double c) {
return a * exp(-((x - b) * (x - b)) / (2 * c * c));
}
int main() {
double x = 0.0; // example input
std::cout << "Gaussian value: " << gaussian(x, 1.0, 0.0, 1.0) << std::endl;
return 0;
}
What is Gaussian in C++?
Gaussian functions are mathematical constructs that describe how values are distributed within a dataset. Generally, they create a normal distribution, commonly depicted as a bell curve, which is pivotal in statistics and various applications in programming. Understanding and implementing C++ Gaussian functions allows developers to harness statistical concepts in practical scenarios, making it a highly useful skill.
The Significance of Learning Gaussian in C++
Learning about C++ Gaussian functions is crucial due to their wide-ranging applications, including:
- Statistics: Essential for data analysis and forecasting.
- Image Processing: Used for filtering and noise reduction in images.
- Machine Learning: Fundamental for algorithms involving probability distributions.
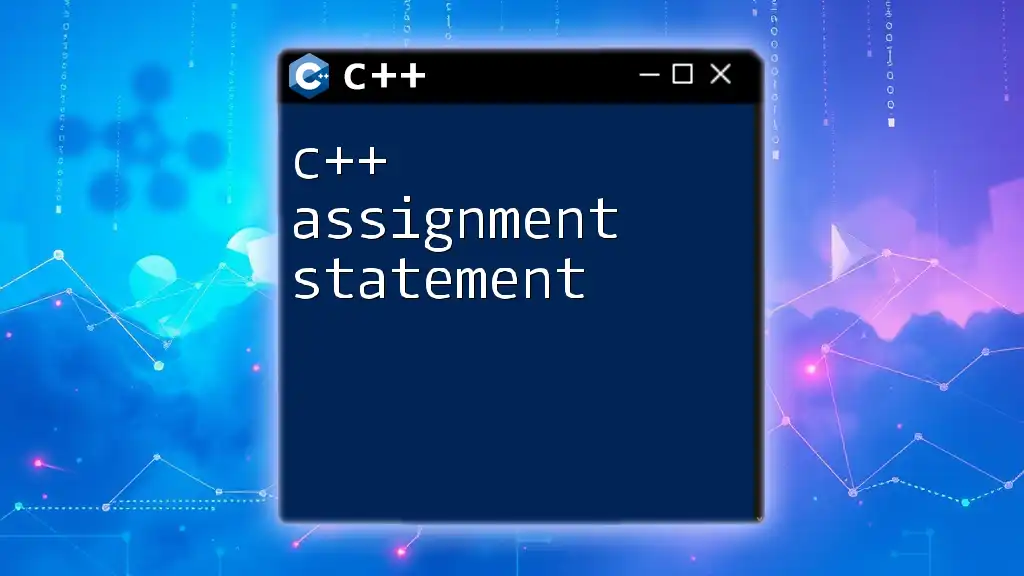
Understanding Gaussian Distribution
Definition of Gaussian Distribution
A Gaussian distribution, also known as a normal distribution, is characterized by three primary metrics: the mean, median, and mode, all of which converge at the center of the distribution curve. The distribution exhibits the following key traits:
- Most observations cluster around the central peak.
- The probabilities for values farther from the mean taper off symmetrically.
The Mathematical Representation
The mathematical representation of a Gaussian function is expressed through the equation:
f(x) = (1 / (σ * √(2π))) * e^(-0.5 * ((x - μ) / σ)^2)
Here:
- μ (mu) represents the mean of the distribution.
- σ (sigma) signifies the standard deviation, illustrating the distribution's spread.
Understanding this equation is essential when implementing Gaussian functions in C++.
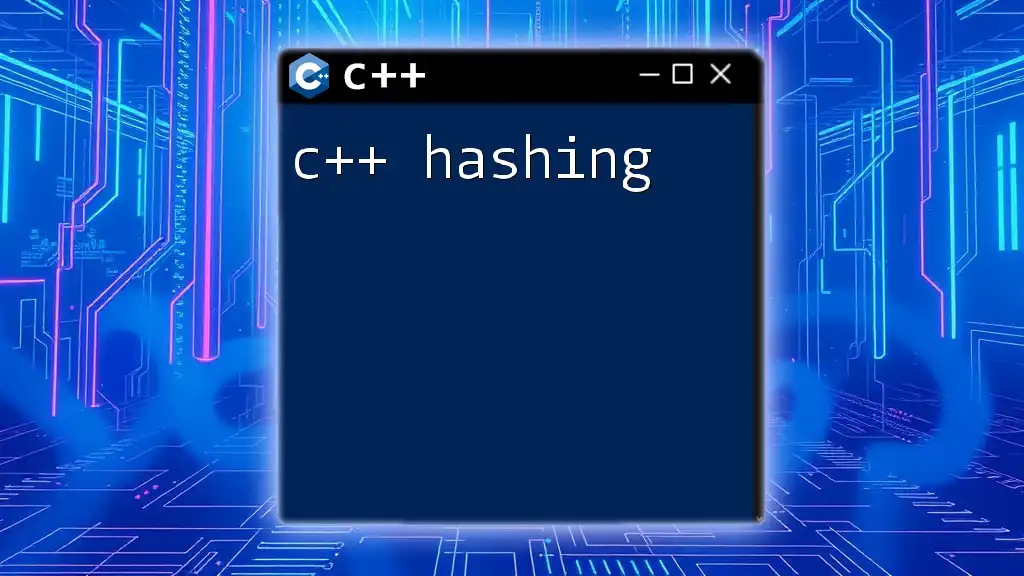
Implementing Gaussian Functions in C++
Setting Up Your C++ Environment
Before diving into the code, ensure that your C++ environment is set up correctly. You can use popular IDEs like Visual Studio or Code::Blocks, and include necessary libraries such as `<cmath>` for mathematical operations and `<random>` for random number generation.
Basic Gaussian Function Implementation
To begin with, let’s look at how to implement a simple Gaussian function in C++:
#include <iostream>
#include <cmath>
double gaussian(double x, double mean, double stddev) {
return (1 / (stddev * sqrt(2 * M_PI))) * exp(-0.5 * pow((x - mean) / stddev, 2));
}
int main() {
double x = 1.0;
double mean = 0.0;
double stddev = 1.0;
std::cout << "Gaussian value: " << gaussian(x, mean, stddev) << std::endl;
return 0;
}
In this code snippet, we define a function called `gaussian`, which calculates the Gaussian value for a given input `x`, mean, and standard deviation. The return statement computes the Gaussian value using the formula we discussed earlier.
When you compile and run the program, it prints the Gaussian value for the specified inputs.
Generating Random Numbers from a Gaussian Distribution
To generate random numbers that follow a Gaussian distribution, we can utilize the `<random>` library, which is part of C++11. The following example demonstrates this:
#include <iostream>
#include <random>
int main() {
std::default_random_engine generator;
std::normal_distribution<double> distribution(0.0, 1.0);
for (int i = 0; i < 10; ++i) {
std::cout << distribution(generator) << std::endl;
}
return 0;
}
In this snippet, we initiate a random number generator and create a normal distribution centered around 0 with a standard deviation of 1. The loop generates ten random samples from this distribution, providing insight into the behavior of Gaussian processes.
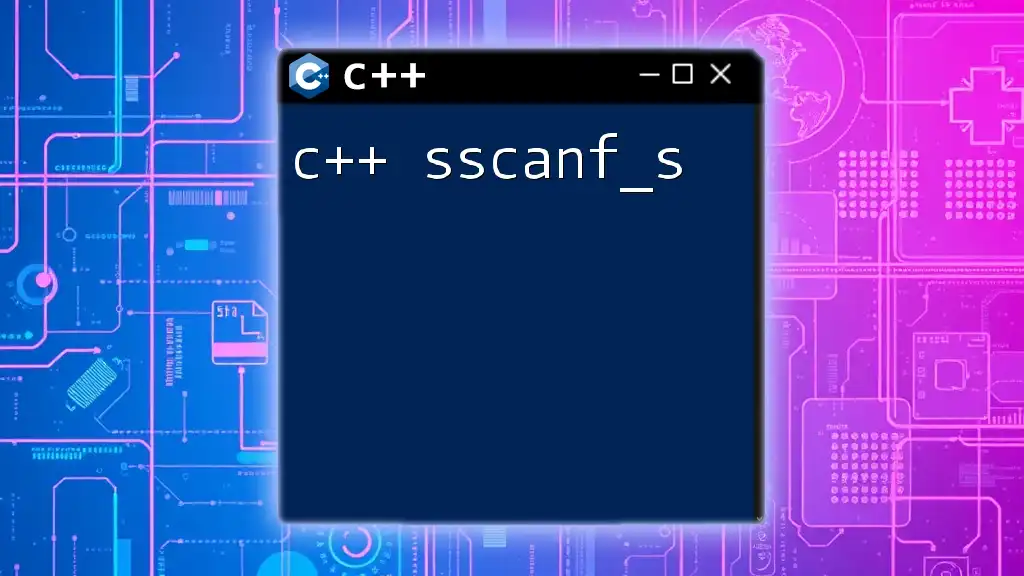
Applications of Gaussian in C++
Gaussian Blur in Image Processing
Gaussian Blur is a widely utilized technique in image processing that smooths images by reducing noise and detail. This effect enhances visual quality and assists in edge detection tasks.
Implementing Gaussian Blur
Here’s a basic implementation of Gaussian Blur using the OpenCV library:
#include <opencv2/opencv.hpp>
using namespace cv;
int main() {
Mat image = imread("input.jpg");
Mat blurred_image;
GaussianBlur(image, blurred_image, Size(5, 5), 0);
imwrite("blurred_output.jpg", blurred_image);
return 0;
}
In this code, we load an image, apply the Gaussian blur, and then save the processed image. The parameters for `GaussianBlur` include the source image, destination image, and kernel size. This code snippet demonstrates how to leverage the `C++ Gaussian` approach effectively in practical applications.
Gaussian and Machine Learning
Gaussian distributions play a critical role in numerous machine learning algorithms. For example, they are integral to clustering techniques like K-Means and Gaussian Mixture Models that rely on statistical properties to identify patterns in datasets.
Identifying how the Gaussian distribution influences machine learning models is invaluable for data scientists looking to improve algorithm accuracy.
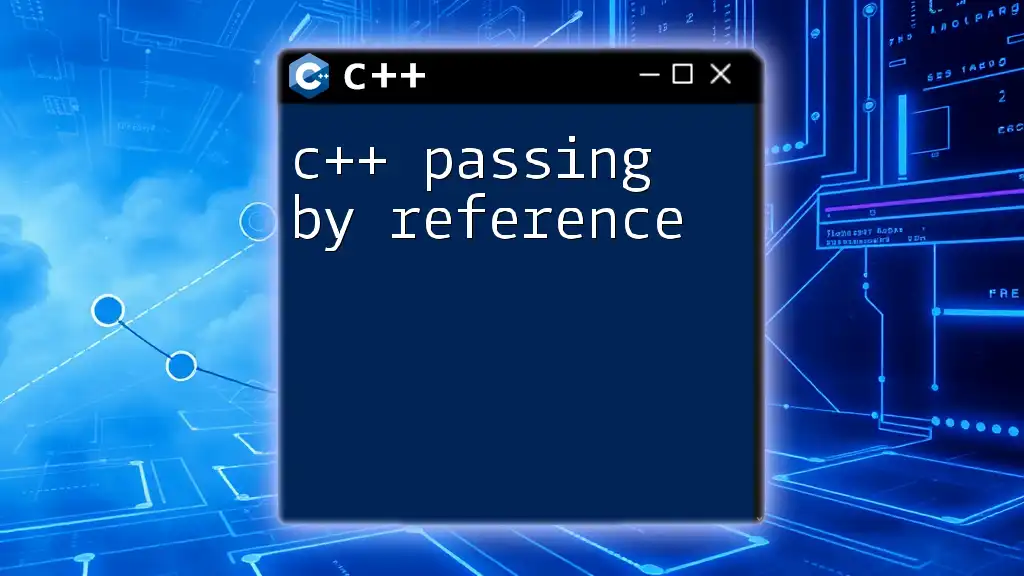
Advanced Gaussian Techniques
Multivariate Gaussian Distribution
A multivariate Gaussian distribution extends the concept of a single variable to multiple variables, allowing the modeling of complex datasets. It is characterized by a mean vector and a covariance matrix.
Implementation in C++
Consider the following example using the Eigen library for matrix operations:
#include <Eigen/Dense>
using namespace Eigen;
double multivariate_gaussian(VectorXd x, VectorXd mean, MatrixXd covariance) {
int dimension = mean.size();
double coeff = 1 / pow((2 * M_PI), dimension / 2.0) * pow(covariance.determinant(), -0.5);
VectorXd diff = x - mean;
return coeff * exp(-0.5 * diff.transpose() * covariance.inverse() * diff);
}
This code illustrates the calculation of a multivariate Gaussian value. Understanding how to manipulate vectors and matrices is crucial in advanced applications.
Gaussian Processes
Gaussian processes are powerful models used in machine learning to perform regression and classification tasks. They provide a flexible framework for understanding and modeling uncertainties in data.
Certain libraries in C++, such as GPy and other machine learning frameworks, allow for Gaussian processes to be implemented effectively.
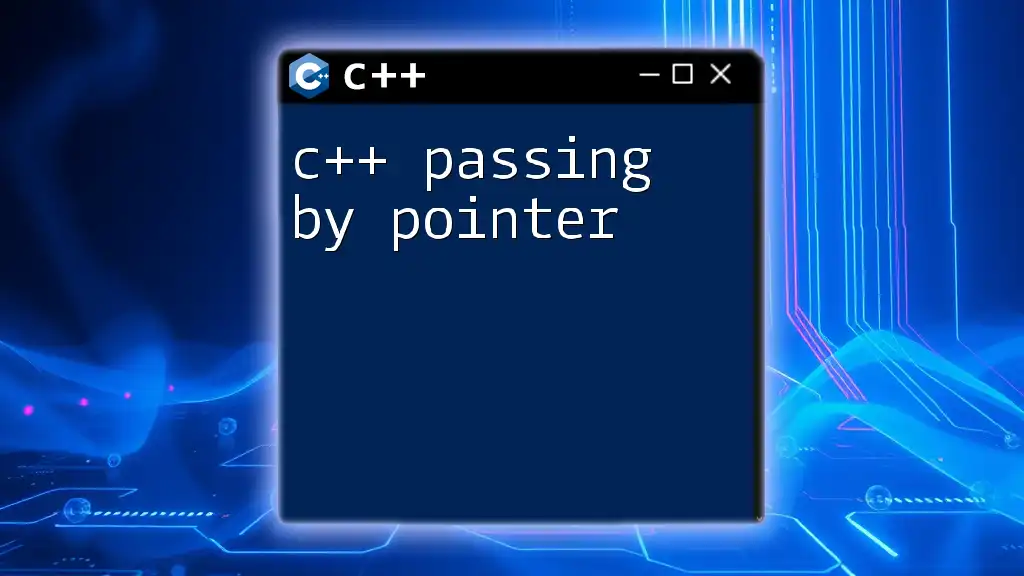
Conclusion
In conclusion, C++ Gaussian functions offer a robust framework for understanding and applying statistical principles in programming. From basic implementations to complex applications in fields such as image processing and machine learning, mastering Gaussian functions will undoubtedly enhance your programming toolkit.
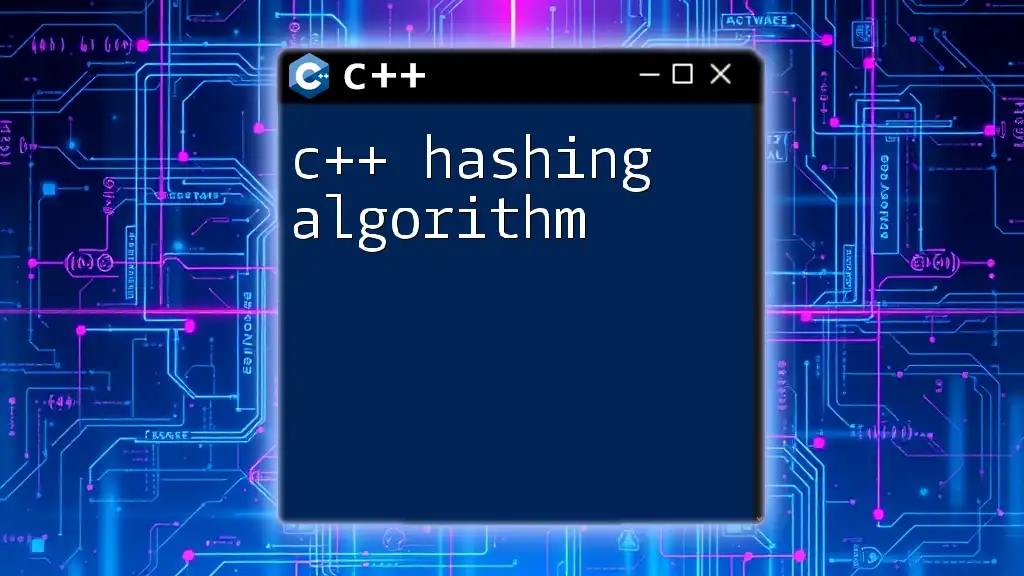
Additional Resources
To further enrich your knowledge on the subject, consider exploring additional literature on statistics and advanced C++ programming. Tools such as OpenCV and machine learning libraries will provide hands-on experience with Gaussian applications, facilitating a deeper understanding of this essential concept.
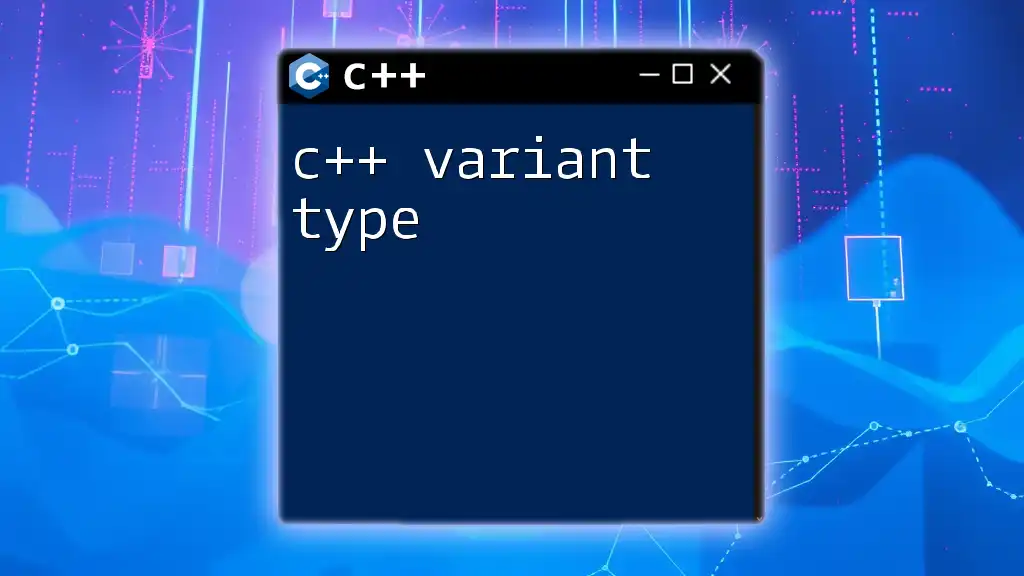
FAQs
Common Questions about Gaussian in C++
What are the best practices for implementing Gaussian in C++?
Utilize libraries that provide optimized implementations, and always validate your input parameters to ensure data integrity.
How to effectively visualize Gaussian distributions?
Employ visualization tools or libraries like Matplotlib in Python to graph Gaussian curves, helping to reinforce your understanding of the concepts.
Can I use Gaussian techniques in real-time applications?
Yes, with optimized code, Gaussian techniques can be integrated into real-time systems, especially in applications requiring noise filtering or statistical modeling.