The `main` function in C++ is the entry point of every C++ program, where execution starts, and must return an integer value.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding the `main()` Function
What is the `main()` Function?
The `main()` function is the heart of every C++ program. It serves as the starting point for execution and is where the program begins its operation. Whenever you run a C++ application, the system looks for the `main()` function to know where to start executing the code. Without it, the program simply cannot run.
Syntax of the `main()` Function
The basic structure of the `main()` function looks like this:
int main() {
// code
return 0;
}
In this syntax, `int` denotes that the function returns an integer value, `main` is the function name, and the curly braces `{}` enclose the body of the function where the code logic resides.
Understanding each component is vital for writing clean and effective C++ code.
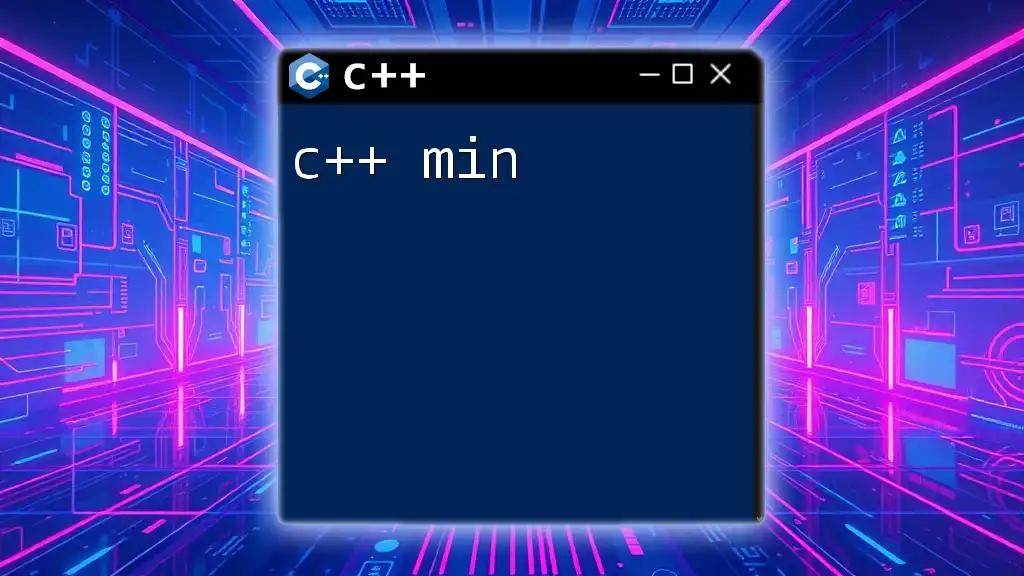
Return Type of `main()`
Why Use `int` as the Return Type?
In C++, functions can return various data types. The `main()` function specifically uses int for its return type to communicate the status of program execution back to the operating system. A return value of 0 typically indicates that the program finished successfully, while a non-zero value signifies that an error occurred.
For instance, consider this simple example:
int main() {
return 0; // Indicates successful execution
}
In this case, `0` signals to the operating system that everything went smoothly.
Possible Return Values
While the convention dictates that returning 0 means success, it is also a good practice to return other integers to signify specific error states. For example:
int main() {
if (someCondition) {
return 1; // Indicates error
}
return 0; // Indicates success
}
This practice allows programmers to troubleshoot their applications effectively by checking the status returned by the program.
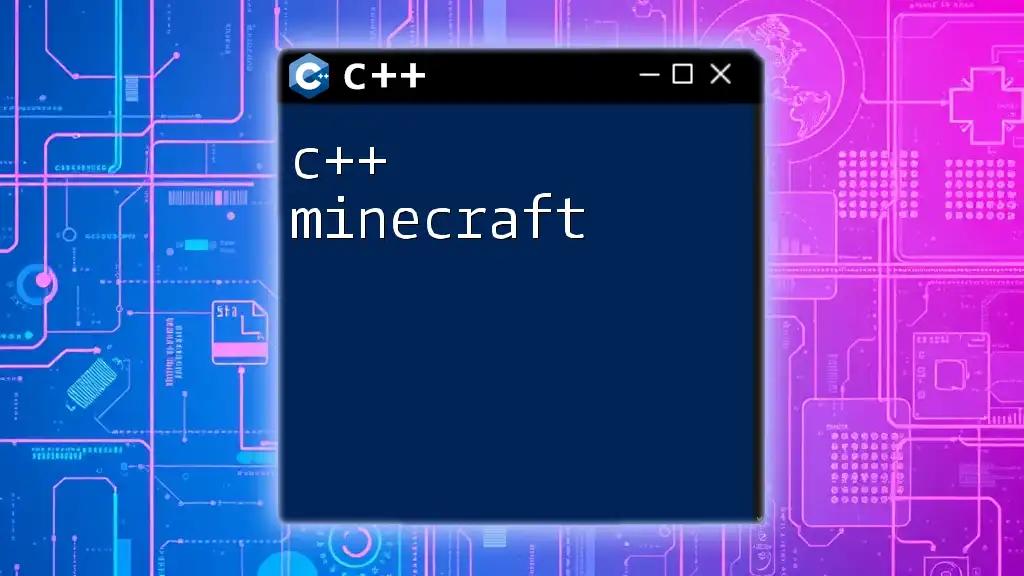
The Role of Command-Line Arguments
Understanding Command-Line Arguments
Command-line arguments enable users to pass parameters directly when invoking a program. This flexibility allows the program to behave differently based on user input.
The two primary parameters that a `main()` function can accept are argc (argument count) and argv (argument vector).
Syntax for Using Command-Line Arguments
The syntax for using command-line arguments in the `main()` function is as follows:
int main(int argc, char *argv[]) {
// code utilizing argc and argv
}
- argc: The number of command-line arguments, including the program name.
- argv: An array of C-style strings (char) containing the arguments passed.
Practical Example of Command-Line Arguments
Let's demonstrate how to work with command-line arguments in a simple C++ program:
#include <iostream>
int main(int argc, char *argv[]) {
for (int i = 0; i < argc; i++) {
std::cout << "Argument " << i << ": " << argv[i] << std::endl;
}
return 0;
}
When running this program with additional parameters, it will print out each argument received. This capability is invaluable for creating dynamic applications that adapt to user input.
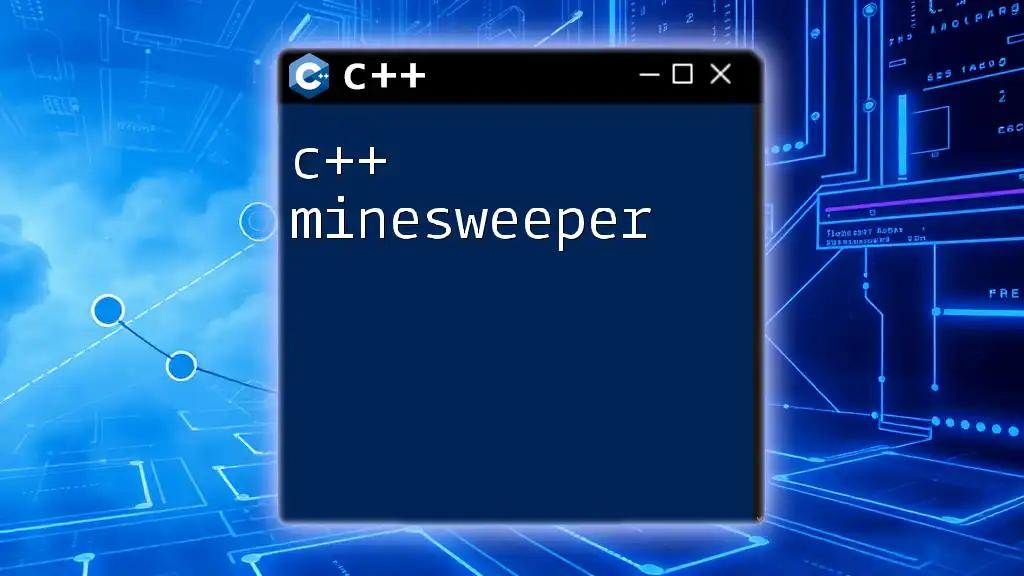
Common Patterns in the `main()` Function
Hello World Example
No programming language tutorial is complete without a "Hello, World!" example. This simple program emphasizes the basic structure of a C++ application.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Here, we include the iostream library to use `std::cout`, which outputs text to the console. The example clearly illustrates the basic syntax and gives new programmers a starting point.
Returning Values Based on Conditions
Advanced functionality can also be incorporated into the `main()` function, where return values can depend on certain conditions. This introduces the capability to execute different logic based on whether specific criteria are met.
#include <iostream>
int main() {
int x = 10;
if (x > 5) {
return 0; // Success
} else {
return 1; // Error
}
}
In this scenario, the program evaluates the variable `x`. If it exceeds 5, it returns 0, indicating success. Otherwise, it returns 1, signifying an error condition.
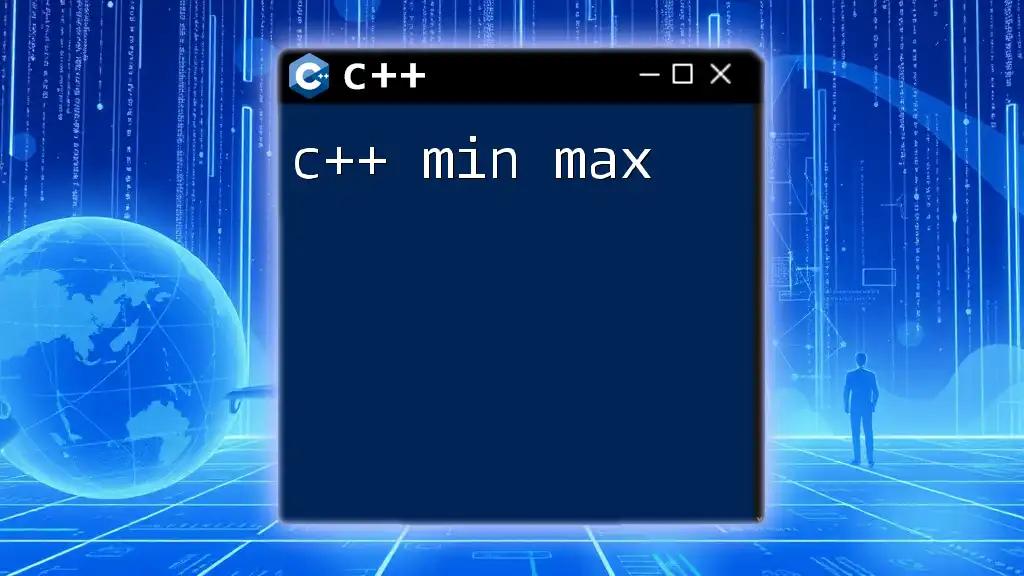
Best Practices for Writing `main()`
Keeping `main()` Concise
One of the keys to effective coding practices is maintaining clarity in the `main()` function. A concise `main()` simplifies understanding and debugging. Avoid complex logic and deep nesting within this primary function; instead, separate functionalities into different functions.
Handling Errors Gracefully
Error handling is crucial for creating robust applications. By implementing appropriate checks and return values, you can ensure that your program gracefully handles unexpected scenarios.
int main() {
// Example error handling
if (/* error condition */) {
return 1; // Report error
}
return 0; // Successful completion
}
Utilizing return values to signal errors not only enhances your program's reliability but also helps future debugging efforts.
Documentation and Comments
Documenting your code is an essential practice for better readability. When adding comments, make sure they clearly explain the purpose of logical blocks within your code.
int main() {
// Main entry point of the program
return 0; // Indicate successful execution
}
Proper documentation acts as a guide for others and for yourself when revisiting the code in the future.
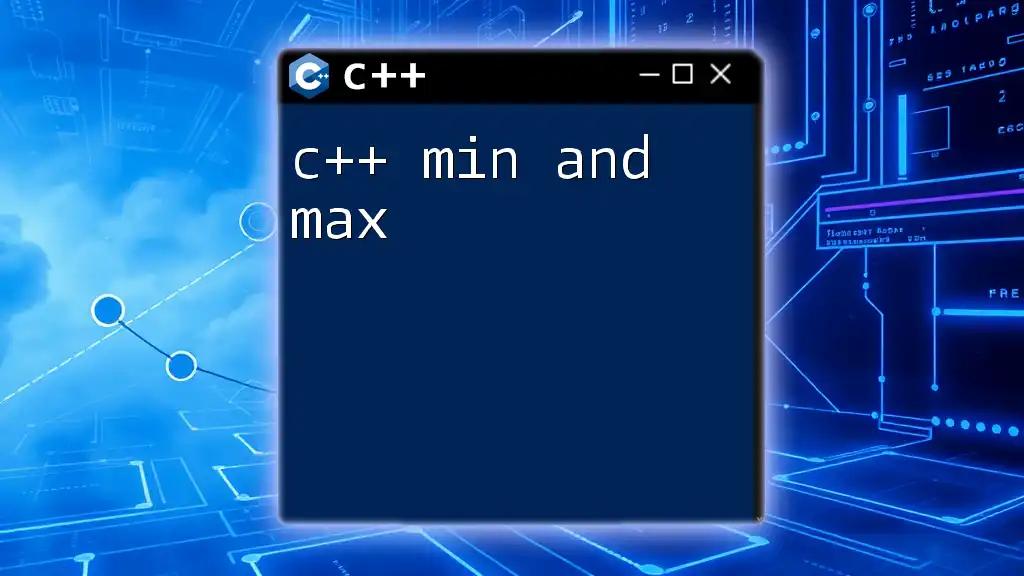
Conclusion
In summary, the `main()` function serves as a critical element of every C++ program. It establishes the starting point for execution and plays a vital role in defining the behavior of the application. By experimenting with various examples and understanding the return types and command-line arguments, you can enhance your programming skills and build versatile applications.
Engaging with your programming community and sharing experiences can help deepen your understanding of the nuances involved in C++ programming. Happy coding!
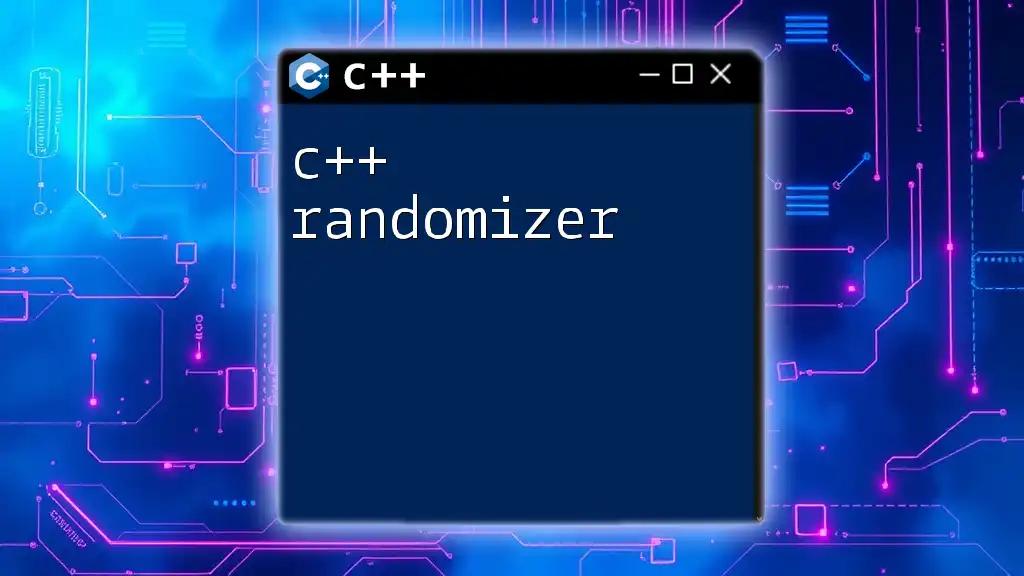
Additional Resources
For further knowledge, consider exploring comprehensive tutorials, books, and community forums that delve into the intricacies of C++. Engaging with seasoned programmers can provide insights and tips to refine your craft.
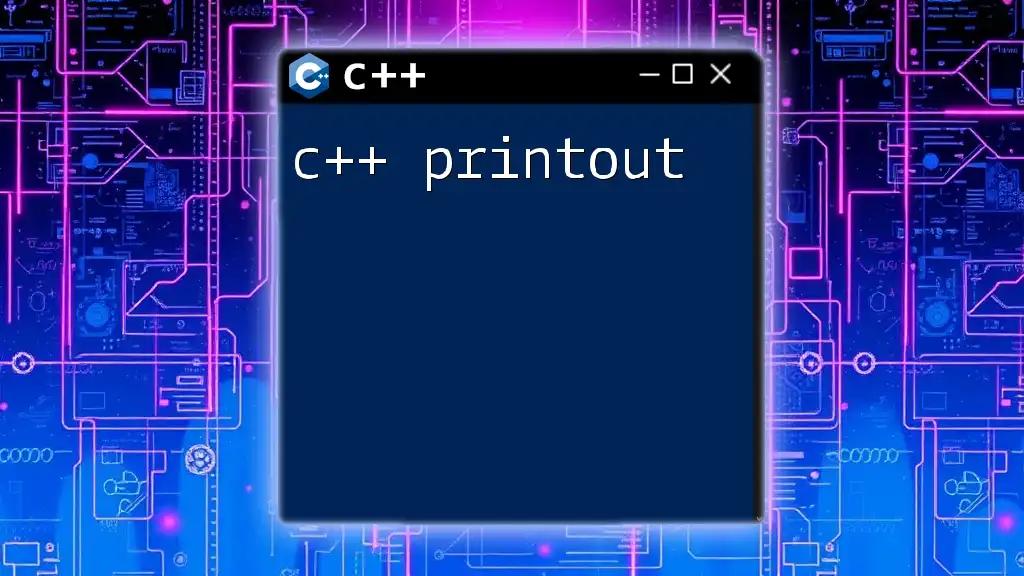
Call to Action
Subscribe for more tips and tricks on mastering C++ commands! Share your experiences or questions regarding the `main()` function in the comments below, and let's build a community of learning together.