In C++, the `std::max` function is used to determine the maximum of two values, returning the greater of the two.
#include <iostream>
#include <algorithm> // for std::max
int main() {
int a = 5, b = 10;
std::cout << "The maximum value is: " << std::max(a, b) << std::endl;
return 0;
}
Understanding the Basics of `max`
What is `max` in C++?
The `max` function in C++ is a powerful utility that allows programmers to obtain the maximum value between two specified inputs. Its fundamental role in comparison functions makes it an essential part of many programming tasks. Whether you're working with integers, floating-point numbers, characters, or even custom objects, `max` can streamline your comparisons and enhance code readability.
`max`: Part of the Standard Library
The `max` function is defined in the `<algorithm>` header, which is part of the C++ Standard Library. By incorporating this function into your code, you can leverage optimized algorithms that improve performance. This utility is commonly employed in everyday programming tasks where quick comparisons are required, such as data analysis, gaming, and real-time applications.
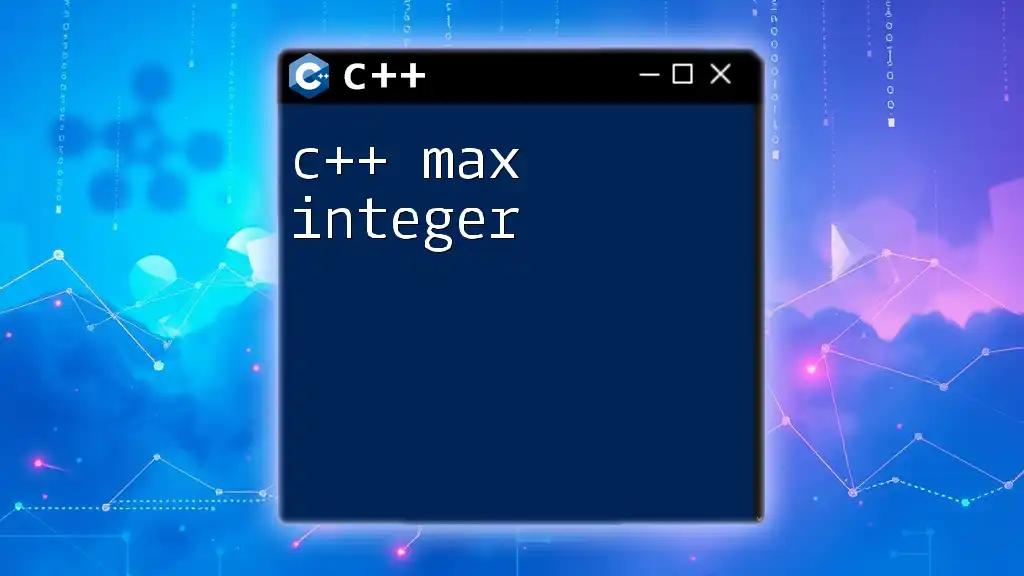
Using `max` with Different Data Types
Finding the Maximum of Integers
To demonstrate the basic usage of `max`, let’s consider a simple comparison of integers.
#include <iostream>
#include <algorithm>
int main() {
int a = 5, b = 10;
int maximum = std::max(a, b);
std::cout << "Maximum: " << maximum << std::endl;
return 0;
}
In this example, `std::max(a, b)` directly compares the two integer values: 5 and 10. The function returns 10, which is printed as the maximum value.
Key Points: The return value of `max` is the larger of the two inputs. Understanding how the function operates can help you make efficient decisions in your code.
Finding the Maximum of Floating-Point Numbers
Comparing floating-point numbers is crucial in many applications, particularly in those requiring more precision.
#include <iostream>
#include <algorithm>
int main() {
double x = 3.14, y = 2.71;
double maximum = std::max(x, y);
std::cout << "Maximum: " << maximum << std::endl;
return 0;
}
Here, `std::max(x, y)` evaluates 3.14 and 2.71. The function returns 3.14 as the larger floating-point number.
Important Note: Floating-point comparisons can lead to precision issues if not used correctly. Always check that your comparisons are accurate when involving floating-point arithmetic.
Using `max` with Char and Strings
The `max` function can also be utilized with characters and strings. Lexicographical comparisons are often useful in sorting.
#include <iostream>
#include <algorithm>
int main() {
char c1 = 'a', c2 = 'b';
char maximum = std::max(c1, c2);
std::cout << "Maximum: " << maximum << std::endl;
return 0;
}
In this snippet, `std::max(c1, c2)` returns 'b' because it is the greater character based on its ASCII value.
When working with strings, `max` will use lexicographical order.
Finding Maximum Strings
#include <iostream>
#include <algorithm>
#include <string>
int main() {
std::string str1 = "Apple", str2 = "Banana";
std::string maximum = std::max(str1, str2);
std::cout << "Maximum: " << maximum << std::endl;
return 0;
}
This piece of code would output "Banana" as it has a higher lexicographical order than "Apple".

Working with Containers and Iterators
Using `max_element` to Find Maximum in Collections
When dealing with collections, such as arrays or `std::vector`, the `max_element` function from the `<algorithm>` header is invaluable.
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {1, 5, 3, 9, 6};
auto max_it = std::max_element(numbers.begin(), numbers.end());
std::cout << "Maximum Element: " << *max_it << std::endl;
return 0;
}
In this example, `std::max_element(numbers.begin(), numbers.end())` searches through the vector for the maximum value. The output will be `9`.
Key Takeaway: Using iterators with `max_element` enhances efficiency when working with larger data sets, as it eliminates the need for manual comparisons.
Custom Comparators with `max`
Sometimes, you may need to compare custom data types. The `max` function can accept custom comparison functions.
#include <iostream>
#include <vector>
#include <algorithm>
struct Person {
std::string name;
int age;
};
bool compareAge(const Person &p1, const Person &p2) {
return p1.age < p2.age;
}
int main() {
std::vector<Person> people = {{"Alice", 30}, {"Bob", 25}};
auto oldest = std::max_element(people.begin(), people.end(), compareAge);
std::cout << "Oldest: " << oldest->name << std::endl;
return 0;
}
The function `compareAge` defines the criteria for comparison, allowing `max_element` to find the person with the greatest age in the vector. The output will be "Alice" as she is the oldest.

Performance Considerations
Efficiency of Using `max`
When optimizing your code, it's worth noting that using the `max` function allows for constant time complexity (O(1)), as it simply compares two values. In contrast, finding a maximum in a collection—if done through loops—will have linear time complexity (O(n)), making `max` a quicker alternative for direct comparisons.
Error Handling
Be cautious of potential pitfalls when using `max`, especially with integer types that can cause overflow. For instance, if two large integers are compared, their sum could exceed the maximum limit of the data type, leading to undefined behavior.
Tip: Always verify data types and limit ranges before performing comparisons, especially when dealing with user input or calculations that could lead to overflow.

Conclusion
Recap of Key Takeaways
The `max` function in C++ is versatile and efficient for finding the maximum value among given inputs. Whether you are working with simple variables, containers, or custom types, understanding and utilizing `max` can greatly enhance your coding efficiency and clarity.
Further Learning Resources
For those looking to deepen their knowledge, consider exploring additional C++ resources such as documentation on the Standard Library, online forums, and tutorial courses tailored toward effective programming practices.
Call to Action
Put your newfound understanding of `c++ max` into practice! Experiment with different data types and scenarios, and do share your experiences or questions in the comments. Your coding journey is always enriched by community engagement!