The `std::make_unique` function in C++ is a convenience function that creates a unique pointer, ensuring proper memory management and preventing memory leaks.
Here's a code snippet demonstrating its usage:
#include <iostream>
#include <memory>
int main() {
auto ptr = std::make_unique<int>(10); // creates a unique pointer to an int initialized to 10
std::cout << *ptr << std::endl; // outputs: 10
return 0;
}
Understanding Smart Pointers in C++
What are Smart Pointers?
Smart pointers are a critical concept in C++ aimed at managing the lifetime of dynamically allocated objects. They encapsulate raw pointers while providing automatic memory management, significantly reducing the risks of memory leaks and dangling pointers. Unlike raw pointers, which require explicit deletion, smart pointers automatically deallocate memory when they are no longer needed.
Using smart pointers comes with several benefits:
- Automatic Resource Management: They automatically clean up resources, making your code safer and easier to maintain.
- Exception Safety: Smart pointers can automatically release their resources even when exceptions are thrown, reducing the likelihood of memory leaks.
The Role of `std::unique_ptr`
`std::unique_ptr` is one of the widely used smart pointer types in C++. It represents exclusive ownership of a dynamically allocated object, meaning that only one `std::unique_ptr` can own a particular object at any time. When the `std::unique_ptr` goes out of scope, it automatically deletes the object it owns, preventing memory leaks and managing resources more effectively.
The scenarios where `std::unique_ptr` shines include:
- Resource Management: When dealing with object lifetimes tied to function scope.
- Dynamic Object Creation: For allocating resources in a more controlled way.
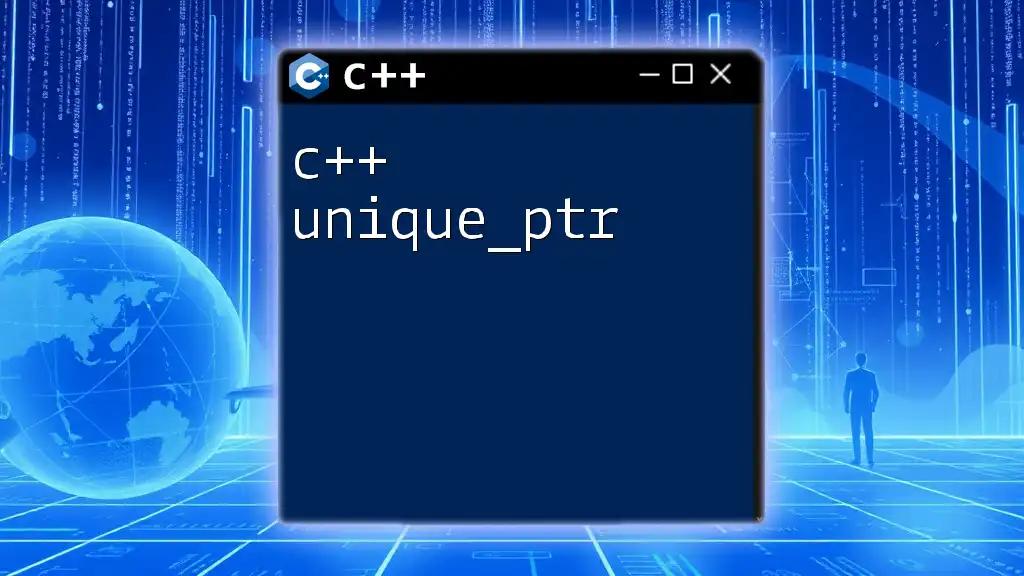
Getting Started with `make_unique`
What is `make_unique`?
`make_unique` is a utility function introduced in C++14 that simplifies the creation of `std::unique_ptr`. It allocates an object and wraps it in a `std::unique_ptr`, ensuring that the newly allocated memory is properly managed without requiring explicit deletion. This function improves code safety and readability by preventing common mistakes associated with raw pointers.
Syntax and Usage
The syntax for using `make_unique` is straightforward. Here’s how you can create a unique pointer to an object:
auto ptr = std::make_unique<MyClass>();
Here, `MyClass` is the type of the object you're creating. `make_unique` allocates memory for the object and returns an instance of `std::unique_ptr` that manages it.
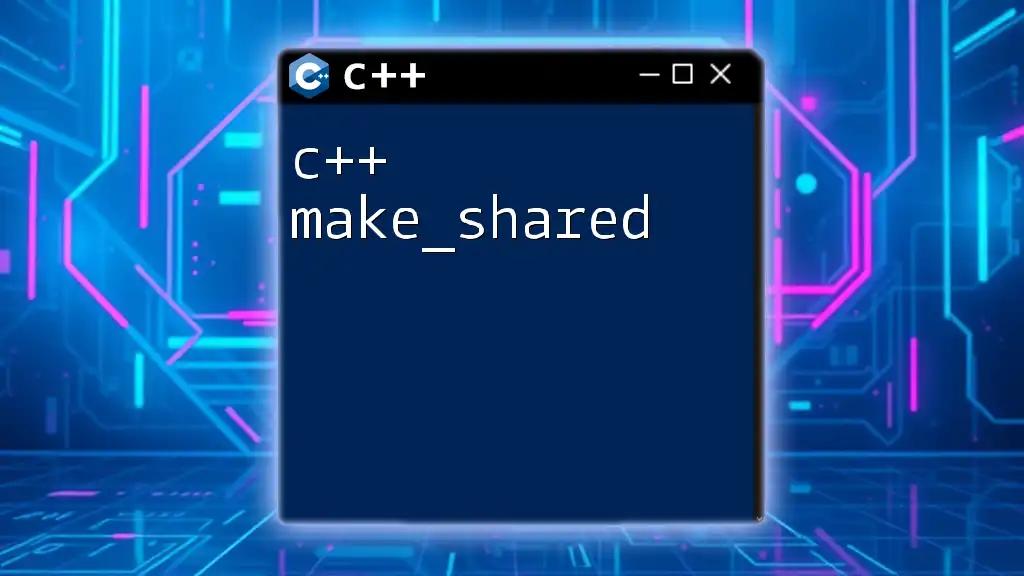
Advantages of Using `make_unique`
Performance Benefits
Using `make_unique` can enhance performance by:
- Reducing the Risk of Memory Leaks: Since `std::unique_ptr` automatically deletes the object when out of scope, it significantly reduces the chances of memory leaks that can occur with raw pointers.
- Improved Performance: Memory allocation using `make_unique` is more efficient. It combines allocation and ownership in one step, leading to fewer overall allocations compared to using raw pointers.
Safety and Exception Handling
`make_unique` also improves safety in scenarios involving exceptions. If an exception occurs during object construction, `make_unique` ensures that no memory is leaked because it encapsulates the memory allocation and the pointer ownership into a single construct. Thus, when an exception is thrown, the memory is released automatically, leading to more robust code.

Key Features of `make_unique`
Type Inference
One of the strengths of `make_unique` lies in type inference. You don’t need to specify the type explicitly when declaring the unique pointer. For example:
auto myPtr = std::make_unique<MyClass>();
The type of `myPtr` is automatically deduced to be `std::unique_ptr<MyClass>`, making the code cleaner and more maintainable.
Forwarding Arguments
`make_unique` also supports forwarding arguments, allowing you to pass constructor arguments directly. For example:
auto myPtr = std::make_unique<MyClass>(arg1, arg2);
In this case, `arg1` and `arg2` are forwarded to the constructor of `MyClass`, demonstrating the flexibility of `make_unique` in handling complex constructor scenarios.

Comparing `make_unique` with Other Memory Management Techniques
`make_shared` vs. `make_unique`
While `make_unique` provides exclusive ownership, `std::make_shared` creates a shared pointer that can have shared ownership of a single object. The choice between the two comes down to specific use cases:
- Use `make_unique` when you need unique ownership and want to limit the lifetime of the object to a single owner.
- Use `make_shared` when you need shared ownership, allowing multiple pointers to point to the same resource.
Raw Pointers vs. `make_unique`
When comparing raw pointers with `make_unique`, a clear distinction arises:
- Raw Pointers require manual memory management, increasing the risk of memory leaks and dangling pointers.
- `make_unique` simplifies memory management, automatically handling object destruction and making code safer and more reliable.
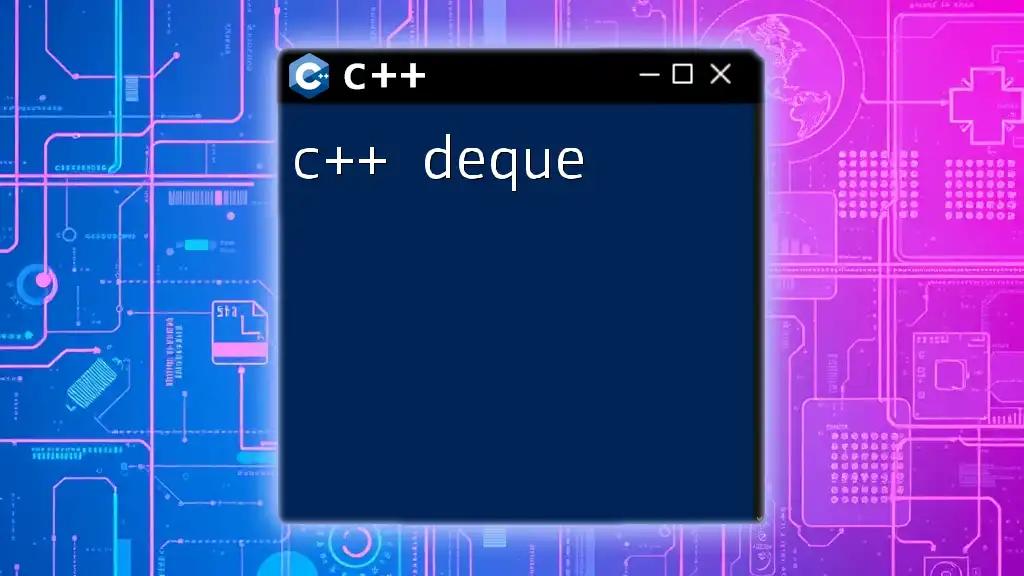
Best Practices when Using `make_unique`
When to Use `make_unique`
`make_unique` should be your go-to tool under the following circumstances:
- When creating new objects: It is a best practice to use `make_unique` whenever you need to allocate memory for a new object.
- When working with dynamic resource management: It should be the standard approach for managing resources dynamically in modern C++.
Common Mistakes to Avoid
Some key pitfalls to watch out for include:
- Using `make_unique` with non-trivial types: Ensure that the type you are creating is suitable for dynamic allocation.
- Forgetting to use `make_unique` when appropriate, leading to unnecessary raw pointer usage and increased risk of memory-related issues.
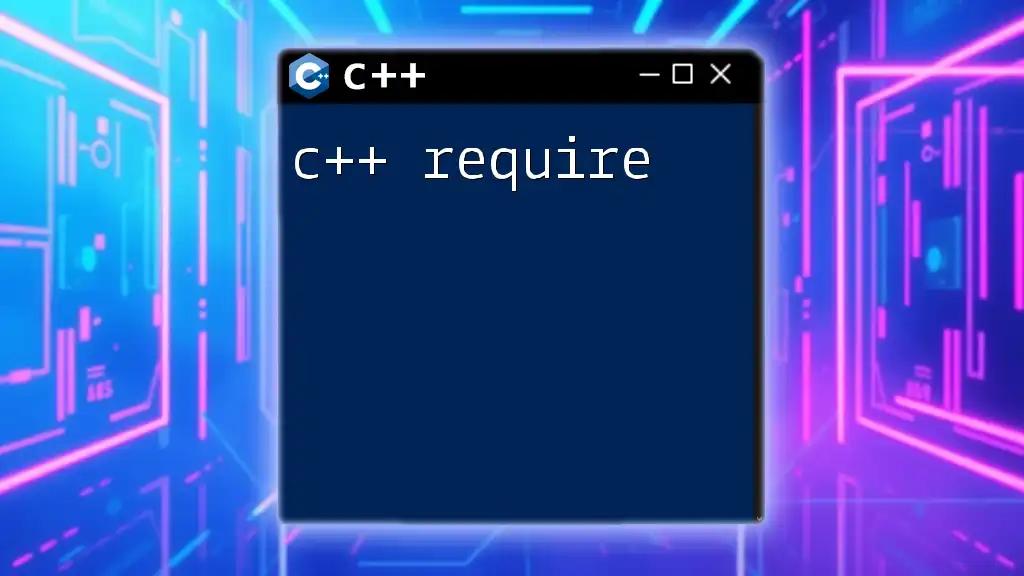
Real-world Examples
Example 1: Using `make_unique` in a Simple Application
Here’s a simple application demonstrating usage of `make_unique`.
#include <iostream>
#include <memory>
class MyClass {
public:
MyClass() { std::cout << "MyClass constructed\n"; }
~MyClass() { std::cout << "MyClass destroyed\n"; }
};
int main() {
auto ptr = std::make_unique<MyClass>();
return 0;
}
This program creates a unique pointer to `MyClass`. When `ptr` goes out of scope, the destructor of `MyClass` is called automatically, and the memory is freed.
Example 2: Resource Management
Consider the following example, where `make_unique` is used to manage resources efficiently in a function.
#include <iostream>
#include <memory>
class Resource {
public:
Resource() { std::cout << "Resource acquired\n"; }
~Resource() { std::cout << "Resource released\n"; }
};
std::unique_ptr<Resource> createResource() {
return std::make_unique<Resource>(); // Automatically manage the resource
}
int main() {
auto resourcePtr = createResource();
// Resource is automatically cleaned up when resourcePtr goes out of scope
return 0;
}
In this example, `createResource` demonstrates how `make_unique` can be used to return a dynamically allocated resource while maintaining ownership semantics.
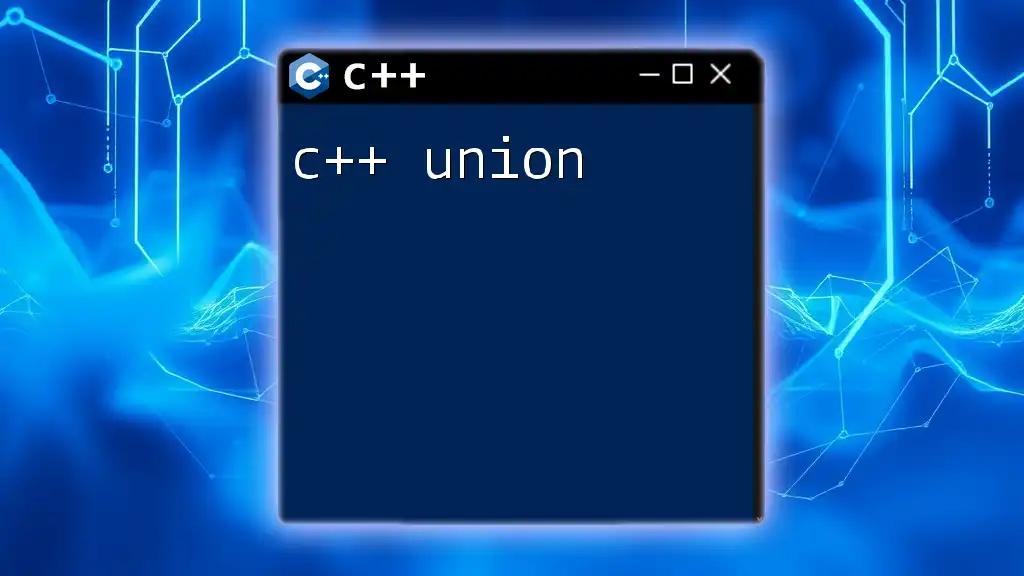
Conclusion
In conclusion, `c++ make_unique` is a powerful tool for modern C++ programming, significantly enhancing memory management through smart pointers. By using `make_unique`, you can minimize the risks commonly associated with dynamic memory allocation while promoting safer, cleaner, and more maintainable code. Adopt `make_unique` in your codebase today to embrace the benefits of modern C++ practices!
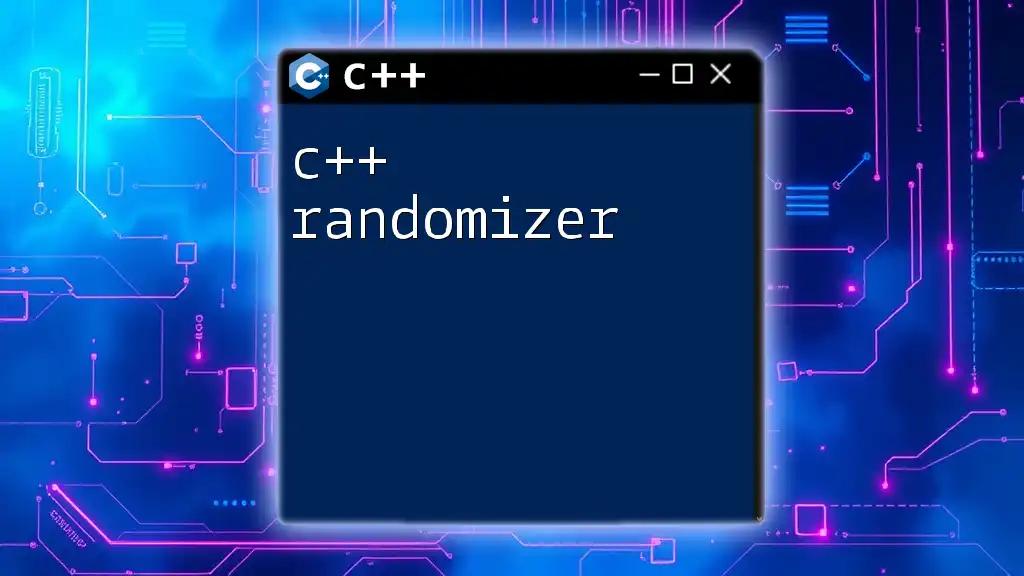
Further Reading and Resources
For deeper exploration of smart pointers and C++ memory management practices, consult reputable resources like the C++ documentation, modern C++ books, and tutorials focused on effective C++ programming techniques.