A C++ mutex (mutual exclusion) is a synchronization primitive that prevents multiple threads from accessing a shared resource simultaneously, ensuring data consistency and thread safety.
Here's a simple example of using a mutex in C++:
#include <iostream>
#include <thread>
#include <mutex>
std::mutex mtx; // Create a mutex
void printThreadId(int id) {
mtx.lock(); // Lock the mutex
std::cout << "Thread ID: " << id << std::endl;
mtx.unlock(); // Unlock the mutex
}
int main() {
std::thread threads[5];
for (int i = 0; i < 5; ++i) {
threads[i] = std::thread(printThreadId, i);
}
for (auto& th : threads) {
th.join(); // Wait for threads to finish
}
return 0;
}
Understanding C++ Mutex
What is a Mutex?
A mutex (short for mutual exclusion) is a synchronization primitive used in programming to manage access to shared resources by multiple threads. It acts as a lock that allows only one thread to access a resource at a time, preventing unexpected behavior due to concurrent modifications.
Using a mutex is crucial in a multithreading environment where threads may concurrently read from and write to the same resource. Without proper coordination, this can lead to data corruption or inconsistent program states.
Imagine a busy restaurant kitchen where only one chef can use the stove at a time. A mutex serves as this single key that lets only one chef cook (or thread execute) at a time, ensuring that the food doesn’t get burnt or mixed up.
Why Use a Mutex?
The primary reason to use a mutex is to prevent data races, which occur when two or more threads access shared data simultaneously and at least one thread modifies the data. Preventing data races ensures data consistency and maintains the integrity of the application's state.
In a concurrent environment, properly managing access to data is essential. A mutex not only guards against simultaneous access but also helps in improving program reliability and performance by ensuring that shared resources are used safely.
Thread Synchronization Concepts
Synchronization is the coordination of operation in a program to ensure predictability in concurrent execution. In C++, multithreading allows several operations to occur simultaneously, leading to potential issues if threads interfere with each other.
A critical section is a portion of code where shared resources are accessed. When multiple threads execute these critical sections, they can cause unpredictable results without proper synchronization, underscoring the need for mutexes.

C++ Mutex in Depth
Introduction to `std::mutex`
In C++, `std::mutex` is a standard library class designed for mutual exclusion. It provides a straightforward way to control access to shared resources and enforce thread safety.
Moreover, `std::mutex` is distinct from other synchronization mechanisms, like semaphores or events, because it provides a simple locking mechanism that blocks other threads from accessing the locked resource until it is unlocked.
Basic Usage of `std::mutex`
To begin using `std::mutex`, you first need to create an instance of it:
std::mutex myMutex;
Locking a Mutex
Locking a mutex is performed using the `lock()` function. When a thread calls `lock()`, it gains exclusive access to the mutex. If another thread attempts to call `lock()` while the mutex is held, it will block until the mutex is released.
myMutex.lock();
Unlocking a Mutex
To release the mutex and allow other threads access, you use the `unlock()` method:
myMutex.unlock();
It’s crucial to remember that failing to unlock a mutex will lead to resource leaks and potentially deadlock scenarios where threads are stuck waiting for each other indefinitely.
RAII: Resource Acquisition Is Initialization
RAII is a programming idiom that ties resource management to object lifetime. In the context of a mutex, this is beautifully illustrated through `std::lock_guard`, which automatically locks the mutex upon creation and unlocks it when the object goes out of scope.
Using `std::lock_guard` simplifies coding and dramatically reduces errors associated with manual locking and unlocking:
{
std::lock_guard<std::mutex> lock(myMutex);
// Critical section code here
}
// Mutex is automatically unlocked here
Common Mistakes Using Mutexes
Common pitfalls with mutexes include:
- Forgetting to unlock: This can cause deadlocks since other threads will be blocked from obtaining the mutex.
- Deadlocks: These occur when two or more threads wait indefinitely for mutexes held by each other. Proper design and careful locking order can mitigate this risk.
- Over-locking: Locking a mutex unnecessarily can reduce the program's performance. It’s essential to keep critical sections as short as possible.

Advanced Mutex Features
`std::recursive_mutex`
A `std::recursive_mutex` allows a thread to lock the mutex multiple times without causing a deadlock, making it useful for scenarios where functions might call themselves.
Here is an example use case:
std::recursive_mutex recMutex;
recMutex.lock();
// locked once
recMutex.lock();
// locked recursively
recMutex.unlock();
recMutex.unlock(); // must be unlocked twice
`std::timed_mutex`
The `std::timed_mutex` allows locking attempts that timeout after a specified duration, preventing indefinite waiting.
With a `std::timed_mutex`, you can try to lock with a timeout:
std::timed_mutex timedMtx;
if (timedMtx.try_lock_for(std::chrono::milliseconds(100))) {
// Mutex acquired
// Critical section code here
timedMtx.unlock();
} else {
// Could not acquire mutex
}
`std::shared_mutex`
A `std::shared_mutex` allows multiple threads to read from shared data simultaneously, while still enabling exclusive write access. It is beneficial in scenarios where reads are more frequent than writes.
Example usage:
std::shared_mutex sharedMtx;
void readFunction() {
std::shared_lock<std::shared_mutex> lock(sharedMtx);
// Read operation here
}
void writeFunction() {
std::unique_lock<std::shared_mutex> lock(sharedMtx);
// Write operation here
}

Practical Examples
Example 1: Simple Mutex in Action
Consider a simple counter scenario where multiple threads increment a counter. Without a mutex, race conditions can corrupt the final count:
int counter = 0;
std::mutex counterMutex;
void increment() {
for (int i = 0; i < 1000; ++i) {
counterMutex.lock();
++counter;
counterMutex.unlock();
}
}
// Launch multiple threads to increment the counter
In this example, the mutex ensures that only one thread modifies the counter at a time, yielding a correct and consistent result.
Example 2: Recursive Mutex Usage
Using a `std::recursive_mutex`, here’s how recursive locking works:
std::recursive_mutex recMutex;
void recursiveFunction(int count) {
if (count == 0) return;
recMutex.lock();
// Critical operations here
recursiveFunction(count - 1);
recMutex.unlock();
}
recursiveFunction(5);
Example 3: Using Shared Mutex for Reader/Writer Problem
The reader/writer problem exemplifies the advantage of `std::shared_mutex`:
std::shared_mutex rwMutex;
int sharedData;
void readData() {
std::shared_lock<std::shared_mutex> lock(rwMutex);
// Read from sharedData
}
void writeData(int data) {
std::unique_lock<std::shared_mutex> lock(rwMutex);
sharedData = data; // Write operation
}
By using a shared mutex, multiple threads can read shared data concurrently, but writes are exclusive.
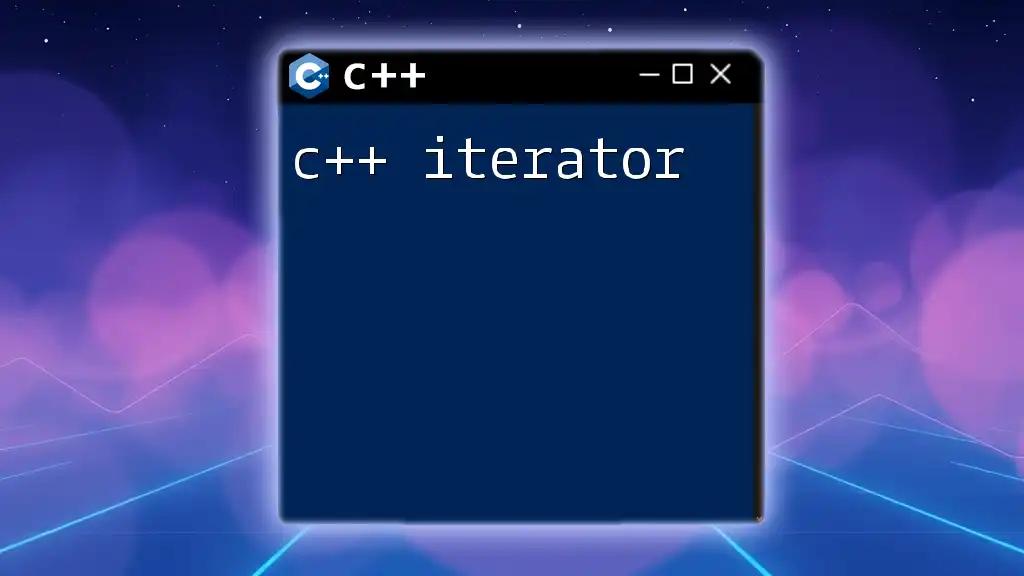
Performance Considerations
Analyzing the Overhead of Mutexes
While mutexes provide necessary synchronization, they can also introduce overhead that may affect performance, particularly if contention is high or critical sections are lengthy. It’s crucial to minimize the time spent within locked regions.
Alternatives to Mutexes
While mutexes serve a vital function, they are not always the optimal solution. Alternatives include:
- Spinlocks: Suitable for situations where threads are expected to wait briefly.
- Atomic variables: Ideal for simple data manipulations without the overhead of locking.
- Condition variables: Useful for thread signaling, allowing threads to wait for certain conditions to be met before proceeding.
It’s essential to carefully evaluate the synchronization tool based on the specific requirements of your application.
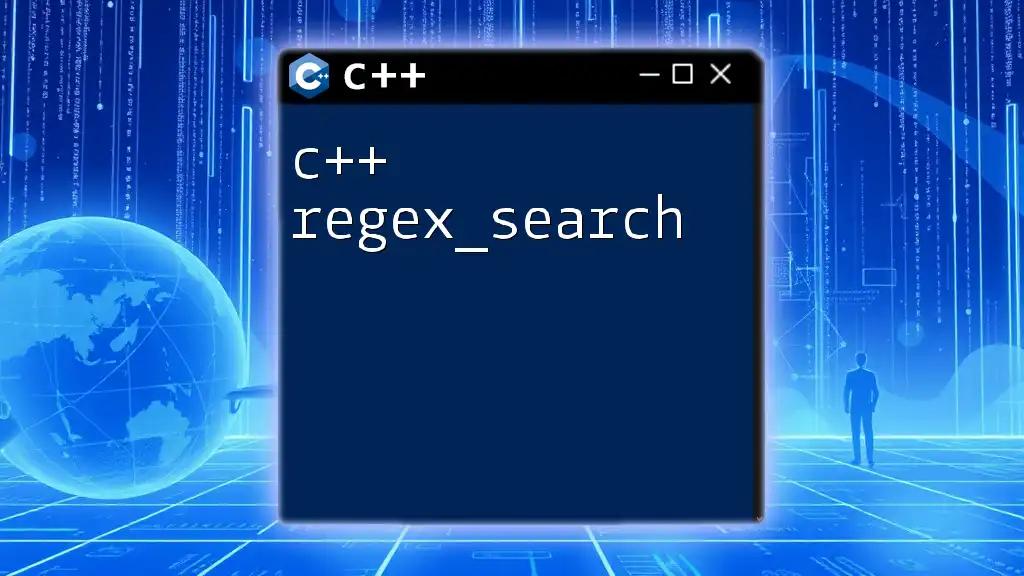
Conclusion
C++ mutexes are an essential part of multithreaded programming that promote safe access to shared resources in concurrent environments. Their ability to prevent data races, ensure consistency, and provide thread safety makes them indispensable tools for developers. Understanding the nuances of different types of mutexes, their proper implementation, and the accompanying best practices will prepare you to tackle the challenges associated with multithreading effectively.
For further reading, consider delving into advanced threading and synchronization techniques through books or online resources focused on C++. Developing fluency in these areas will enhance your programming skills and project outcomes.