In C++, `std::make_shared` is a function that creates a `shared_ptr` to an object, allocating both the object and the control block in a single memory allocation for efficiency.
Here’s an example:
#include <iostream>
#include <memory>
class MyClass {
public:
MyClass() { std::cout << "MyClass constructor\n"; }
~MyClass() { std::cout << "MyClass destructor\n"; }
};
int main() {
std::shared_ptr<MyClass> ptr = std::make_shared<MyClass>();
return 0;
}
What is `make_shared`?
`make_shared` is a utility function in C++ that greatly simplifies the management of dynamic memory by combining the allocation of an object and the creation of a `std::shared_ptr` into a single operation. This ensures that the object is allocated in the same memory block as the control structure used by the `shared_ptr`, thereby improving efficiency and memory usage.
In the context of smart pointers, `make_shared` is part of the C++11 standard and offers a more efficient way to create shared ownership of dynamically allocated objects compared to directly instantiating a `std::shared_ptr`. With `make_shared`, you can create shared pointers without having to deal with raw pointers directly, reducing the risk of memory leaks.
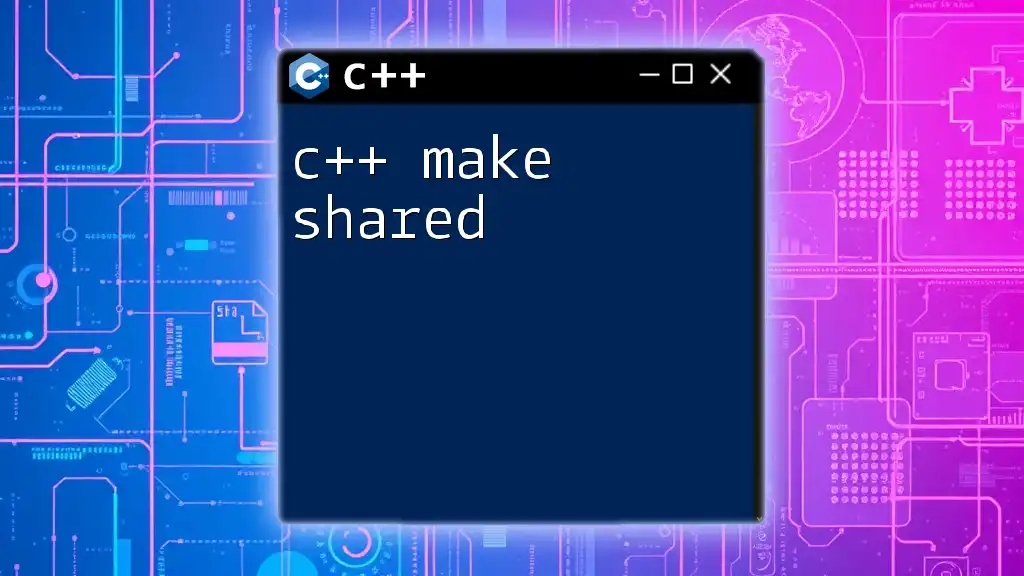
Why Use `make_shared`?
Memory Efficiency
One of the primary advantages of `make_shared` lies in its memory efficiency. When you create an object through `make_shared`, the memory for both the object and the control block (which keeps track of reference counts) is allocated in one contiguous block. This contrasts with the traditional method of creating a `shared_ptr` using `new`, which allocates the object and control block separately. Since most memory management systems perform better when allocating single contiguous blocks, using `make_shared` can lead to reduced memory overhead and improved performance.
Performance Benefits
Performance also sees significant benefits when using `make_shared`. By combining the allocation steps, you reduce the overhead compared to creating a shared pointer from a raw pointer. Allocating in one go not only speeds up creation but also decreases the likelihood of memory fragmentation, which can degrade performance over time, especially in long-running applications.
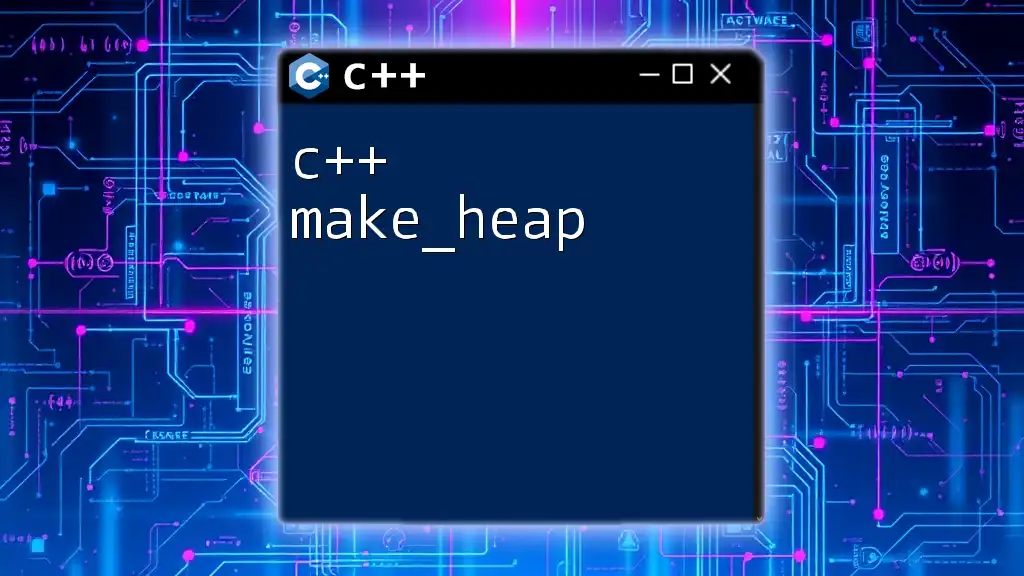
How to Use `make_shared`
The basic syntax for `make_shared` is simple:
std::shared_ptr<ClassName> ptr = std::make_shared<ClassName>(constructor_args);
Example: Creating a Shared Pointer
Here’s a practical example that demonstrates how to use `make_shared`:
#include <iostream>
#include <memory>
class MyClass {
public:
MyClass(int value) : m_value(value) {}
void display() { std::cout << "Value: " << m_value << std::endl; }
private:
int m_value;
};
int main() {
auto myObject = std::make_shared<MyClass>(10);
myObject->display();
return 0;
}
In the example above, we define a simple class `MyClass`, which takes an integer in its constructor. Using `make_shared`, we create a `shared_ptr` pointing to a new `MyClass` instance with the value `10`. The `display` method prints that value to the console.
Handling Dynamic Memory
When you use `make_shared`, you don’t need to worry about explicitly managing the memory. Once all `shared_ptr`s pointing to the object are destroyed, the memory is automatically deallocated. This greatly simplifies dynamic memory management and helps avoid memory leaks, a common pitfall for programmers dealing with raw pointers.
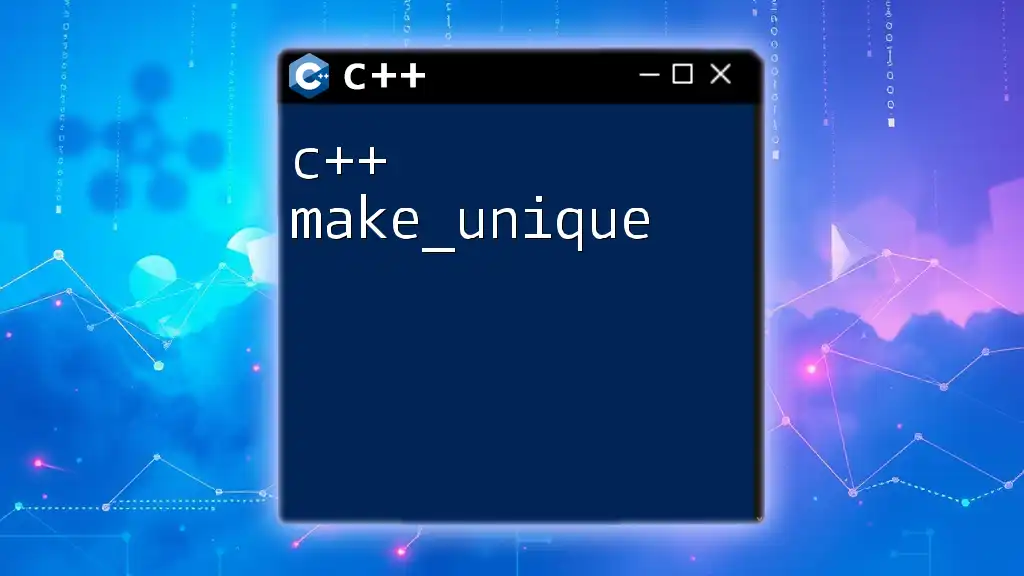
Important Considerations When Using `make_shared`
Exception Safety
Exception safety is another noteworthy aspect of `make_shared`. If an exception is thrown during the object creation (e.g., in the constructor), then `make_shared` does the right thing by not leaking memory. The control block will only be created after the object is fully constructed, which means if the construction fails, no memory is lost.
Limitations of `make_shared`
Despite its advantages, `make_shared` does have some limitations. It cannot be used when you need a custom deleter, as `make_shared` always uses `delete` to deallocate memory. Also, while it simplifies object construction, `make_shared` is not suitable for cases where the type is incompatible with the arguments being passed.
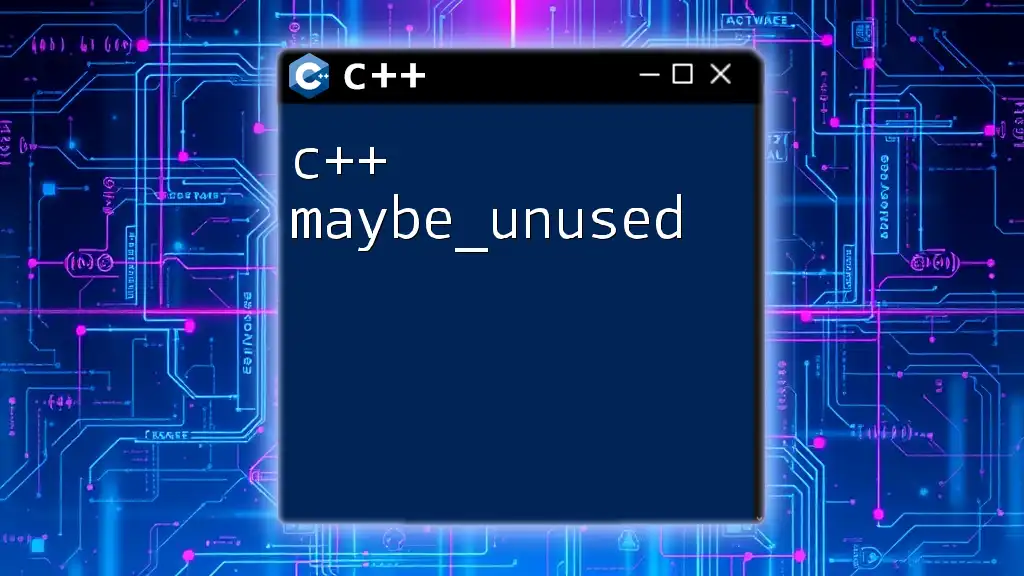
`make_shared` vs `shared_ptr` Creation
Directly Using `shared_ptr`
Here's how creating a shared pointer directly compares to using `make_shared`:
std::shared_ptr<MyClass> ptr1(new MyClass(10)); // Directly using shared_ptr
std::shared_ptr<MyClass> ptr2 = std::make_shared<MyClass>(10); // Using make_shared
When you instantiate a `shared_ptr` directly using `new`, there are two separate allocations: one for the object and another for its control block. This separation can increase the overhead for managing memory compared to the combined approach offered by `make_shared`.
Breakdown of Performance and Safety
Using `make_shared` is generally recommended over the raw `new` keyword due to its performance benefits and inherent safety features. By minimizing the number of allocations and ensuring that memory is cleaned up properly, `make_shared` is often the preferred choice for managing shared memory in C++ applications.
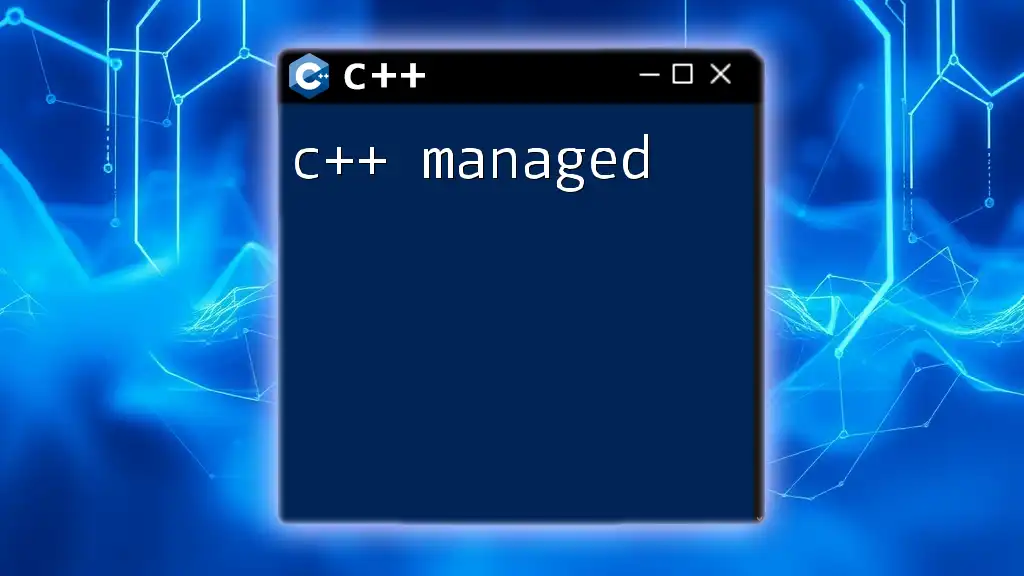
Best Practices for Using `make_shared`
To maximize the benefits of `make_shared`, follow these best practices:
- Use `make_shared` whenever you need shared ownership of an object, especially in performance-critical applications.
- Avoid using `make_shared` when you need to specify a custom deleter for your shared pointer.
- Be cautious about circular references, as they can lead to memory leaks even with smart pointers.
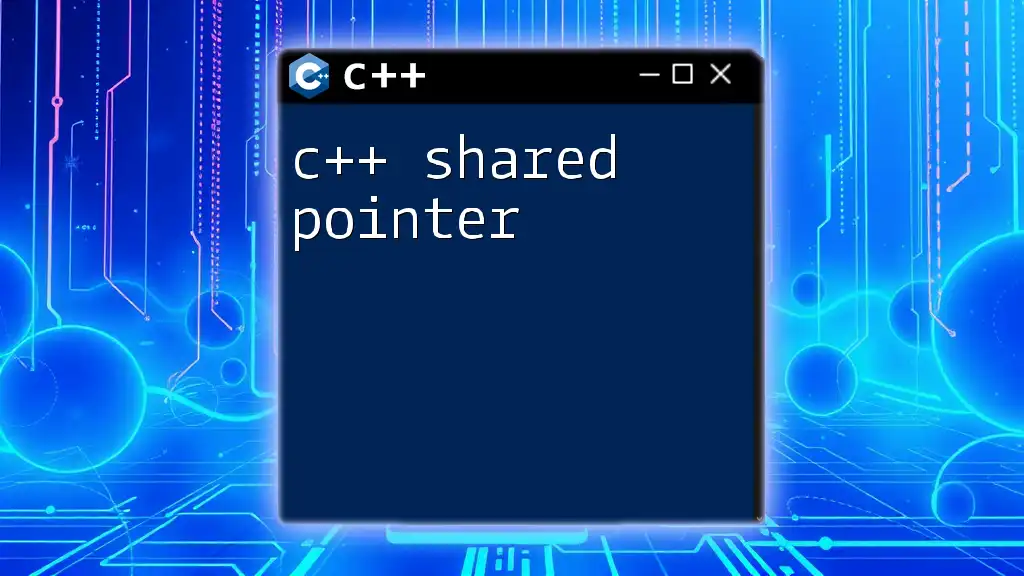
Conclusion
In summary, C++ `make_shared` is an efficient and safer approach to managing dynamically allocated objects in shared memory scenarios. By understanding and implementing `make_shared`, you can greatly enhance your memory management practices and avoid common pitfalls associated with raw pointers. Consider using `make_shared` in your projects to promote cleaner, more efficient code.
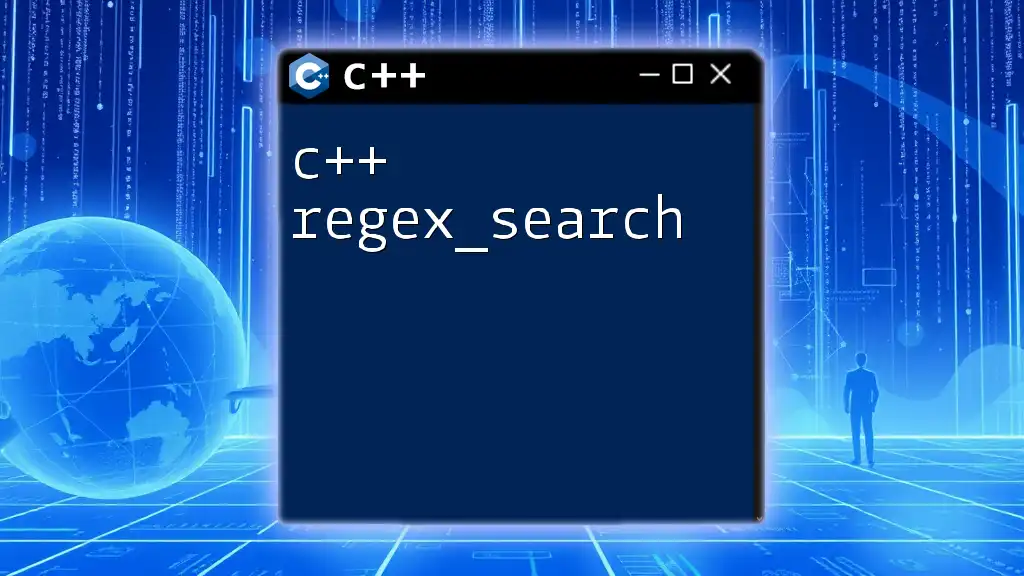
Additional Resources
For those looking to delve deeper into smart pointers and memory management in C++, official documentation, tutorials, and community forums can provide valuable insights and assistance. Enhancing your understanding of smart pointers is key to effective C++ development.
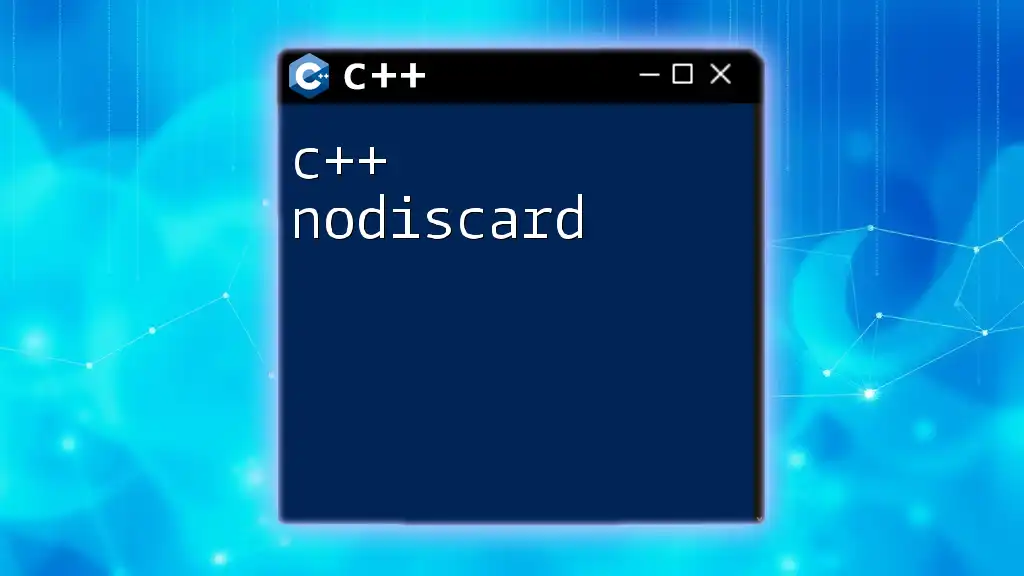
FAQ Section
What should you do if you have more questions on `make_shared`? Don't hesitate to consult available resources or reach out to the programming community. With its simple syntax and powerful capabilities, `make_shared` can greatly simplify your programming experience with C++.