In C++, the `make_shared` function creates a `shared_ptr` instance that manages a dynamically allocated object, optimizing memory usage and sharing ownership across multiple pointers.
Here's an example of how to use `make_shared`:
#include <iostream>
#include <memory>
class MyClass {
public:
MyClass() { std::cout << "MyClass created\n"; }
~MyClass() { std::cout << "MyClass destroyed\n"; }
};
int main() {
std::shared_ptr<MyClass> ptr = std::make_shared<MyClass>();
// Use ptr as needed
return 0;
}
Understanding Smart Pointers
What Are Smart Pointers?
Smart pointers are objects that manage the lifetime of dynamically allocated memory in C++. Unlike traditional pointers, which require manual management and can lead to memory leaks or undefined behavior, smart pointers automatically take care of memory management through automatic resource management (ARM). This ensures that once a smart pointer goes out of scope or is no longer needed, the associated memory is automatically released.
Types of Smart Pointers
In modern C++, there are several types of smart pointers, but the most commonly used are:
-
`std::unique_ptr`: Represents exclusive ownership of a dynamically allocated object. Only one `unique_ptr` can own a specific resource at a time. It cannot be copied but can be moved.
-
`std::shared_ptr`: Allows multiple pointers to share ownership of the same resource. It uses reference counting to keep track of how many pointers point to that resource.
-
`std::weak_ptr`: Acts as a companion to `std::shared_ptr` but does not contribute to the reference count. This is useful for avoiding circular references.
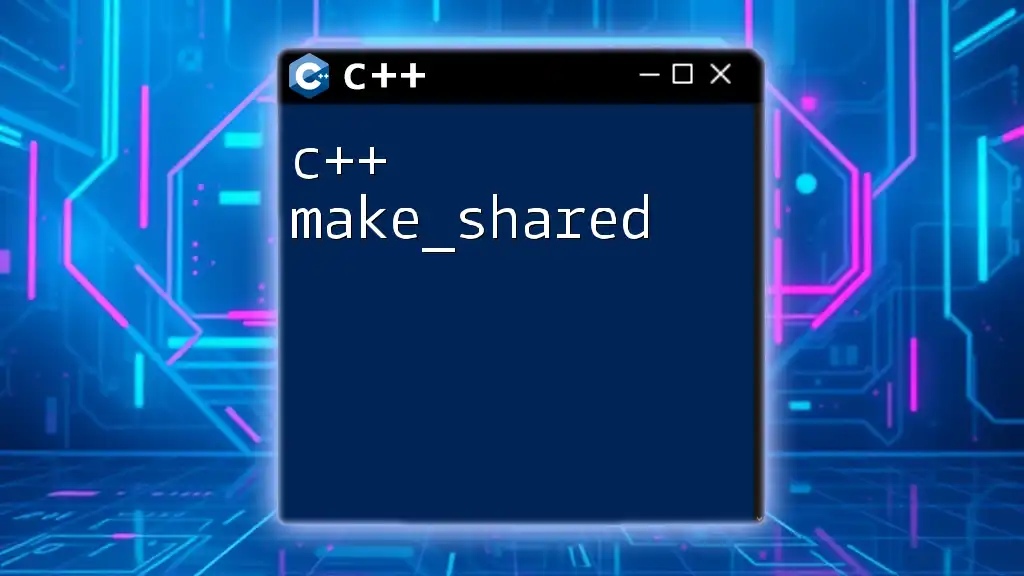
Introduction to `std::shared_ptr`
What is `std::shared_ptr`?
`std::shared_ptr` is part of the C++ Standard Library and is designed for shared ownership of dynamically allocated objects. This means that multiple `shared_ptr` instances can own the same resource. The object will only be destroyed when the last `shared_ptr` pointing to it is destroyed or reset.
How `std::shared_ptr` Works
`std::shared_ptr` implements a reference counting mechanism. Each time a new `shared_ptr` is created from an existing one, the reference count is incremented. When a `shared_ptr` is destroyed, the reference count is decremented. Once the reference count reaches zero, the shared resource is automatically deallocated, thus preventing memory leaks.
Example of creating a shared pointer:
#include <memory>
#include <iostream>
int main() {
std::shared_ptr<int> ptr1 = std::make_shared<int>(10);
std::cout << "Value: " << *ptr1 << std::endl; // Outputs: Value: 10
return 0;
}
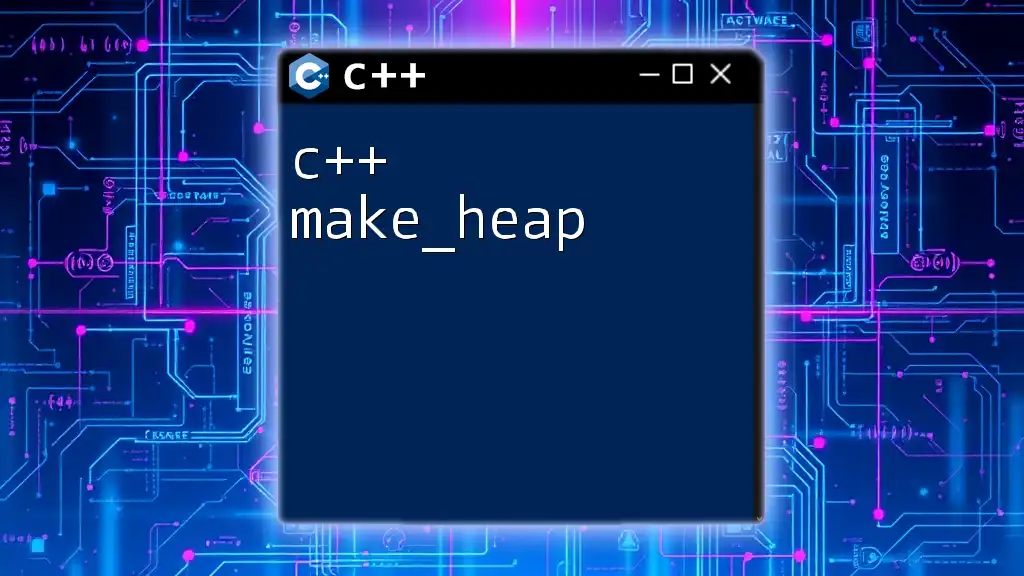
Creating a `std::shared_ptr`
Using `std::make_shared`
To create a `std::shared_ptr`, the recommended approach is using `std::make_shared`. This function allocates memory and constructs the object in a single memory allocation, which leads to improved performance and safety compared to manually using `new`.
Benefits of Using `std::make_shared`
-
Efficiency: By combining memory allocation into a single step, it reduces the overhead associated with separate allocations for the control block and the managed object.
-
Safety: It eliminates potential mismatches in pointer types and simplifies memory management, reducing the risk of memory leaks.
Example: Creating and Using `std::shared_ptr`
Here's a simple example demonstrating the creation and usage of `std::shared_ptr`:
#include <iostream>
#include <memory>
class MyClass {
public:
MyClass() { std::cout << "Constructor Called" << std::endl; }
~MyClass() { std::cout << "Destructor Called" << std::endl; }
};
int main() {
std::shared_ptr<MyClass> myObject = std::make_shared<MyClass>();
return 0; // Destructor will be called automatically when myObject goes out of scope
}
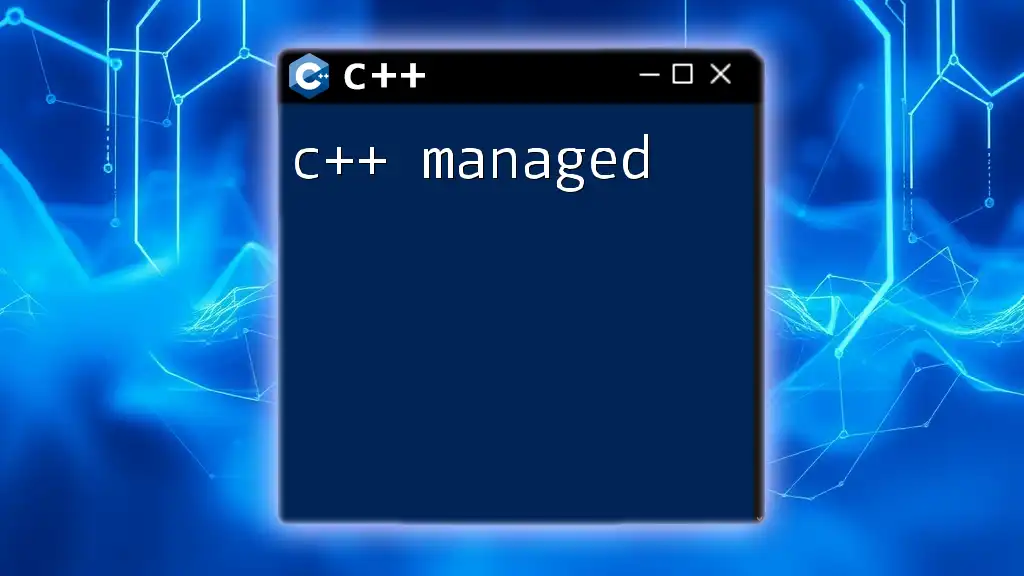
Managing Shared Resources
Sharing Ownership
With `std::shared_ptr`, you can easily share ownership of a resource among multiple pointers. For example:
std::shared_ptr<int> ptr1 = std::make_shared<int>(20);
std::shared_ptr<int> ptr2 = ptr1; // ptr1 and ptr2 share ownership
In this code, both `ptr1` and `ptr2` point to the same integer value, and they will manage its lifetime together. When both `shared_ptr`s go out of scope, the integer value will be deleted.
Weak Pointers
What is `std::weak_ptr`?
`std::weak_ptr` is a type of smart pointer that provides a non-owning reference to an object managed by `std::shared_ptr`. It does not affect the reference count, which helps to prevent memory leaks, especially in the case of cyclic references.
Preventing Cyclic References
When two `shared_ptr`s hold references to each other, it creates a cycle that prevents memory from being freed. By using `std::weak_ptr`, you can break this cycle. Here’s how it works:
class Node {
public:
int value;
std::shared_ptr<Node> next;
std::weak_ptr<Node> prev; // weak pointer to avoid cycle
};
In this example, `Node` has a `shared_ptr` to `next`, allowing forward navigation in a linked list, while `weak_ptr` to `prev` prevents a cycle that would lead to memory not being reclaimed.
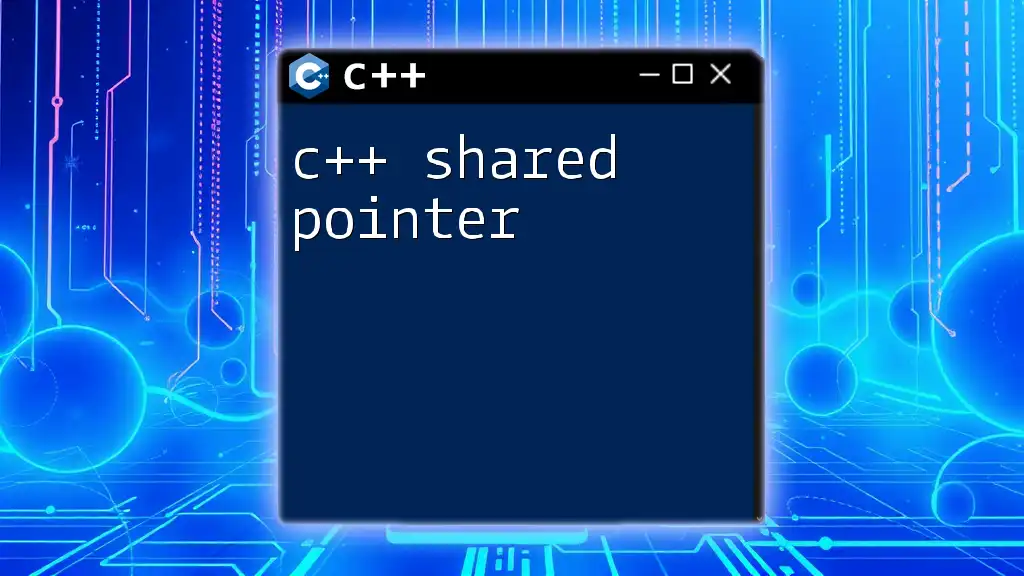
Best Practices with `std::shared_ptr`
When to Use `std::shared_ptr`
Use `std::shared_ptr` when:
- You require shared ownership semantics, where multiple parts of your program need to access the same resource.
- You want to avoid manual memory management and prevent memory leaks.
Avoid using `std::shared_ptr` when:
- You are the sole owner of a resource; in such cases, prefer `std::unique_ptr` for better performance and simplicity.
Performance Considerations
While `std::shared_ptr` provides invaluable memory management benefits, it's essential to consider the performance trade-offs. The reference counting system involves atomic operations, which can introduce overhead, particularly in multithreaded scenarios. Use it judiciously and prefer lightweight alternatives like `std::unique_ptr` when shared ownership is not necessary.
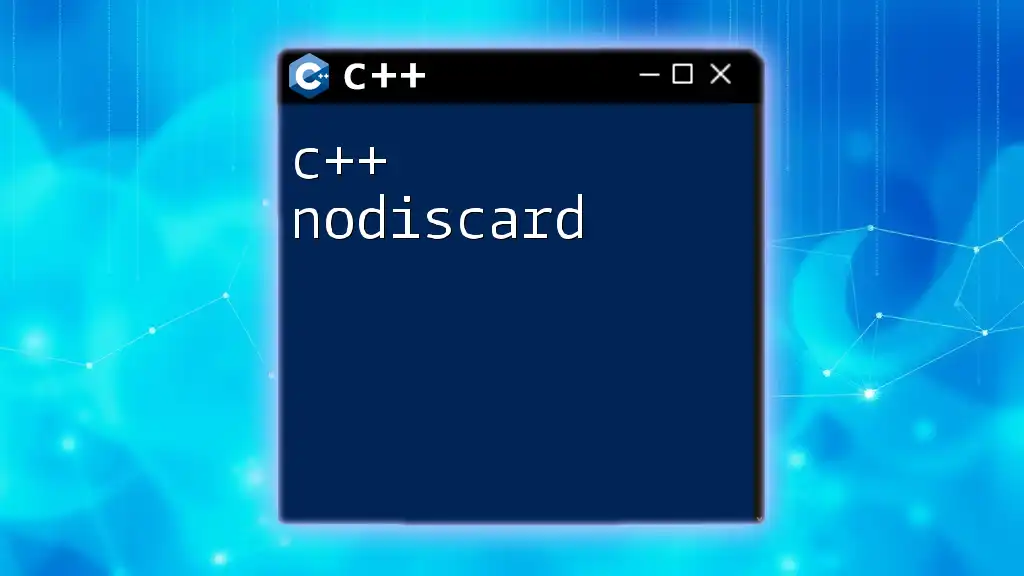
Conclusion
In summary, using `std::shared_ptr` and `std::make_shared` in C++ brings safer and more efficient memory management to your applications. By understanding the principles of smart pointers, you can effectively manage dynamic resources, prevent memory leaks, and improve code readability. As you continue to explore C++, remember the power of smart pointers and how they can simplify your memory management tasks. Embrace these tools for a more robust and maintainable codebase.
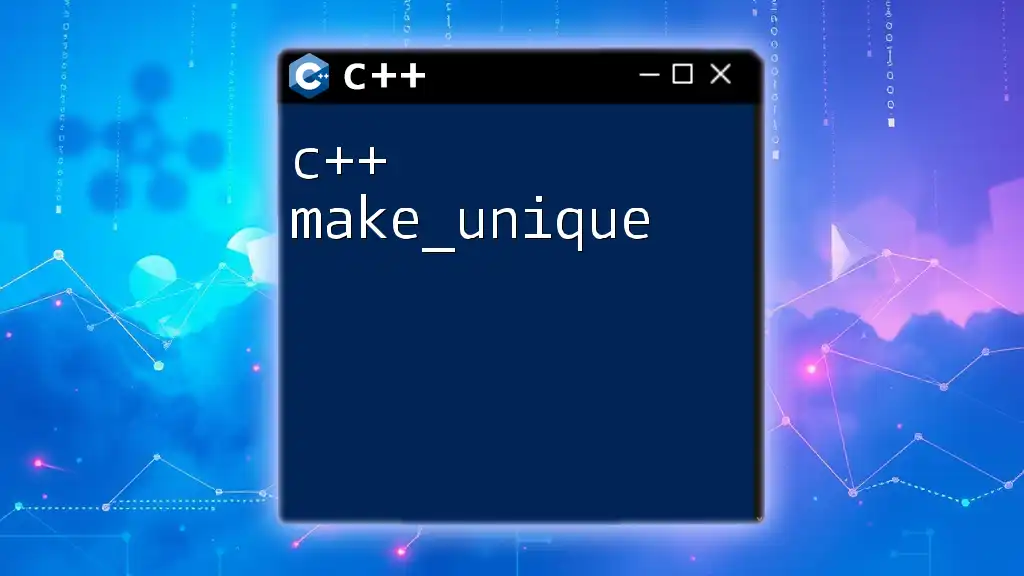
Additional Resources
To deepen your understanding of `std::shared_ptr` and smart pointers, consider exploring the following:
- C++ Standard Library Documentation
- Tutorials on memory management in C++
- Recommended tools and IDEs for practicing C++ commands and smart pointers
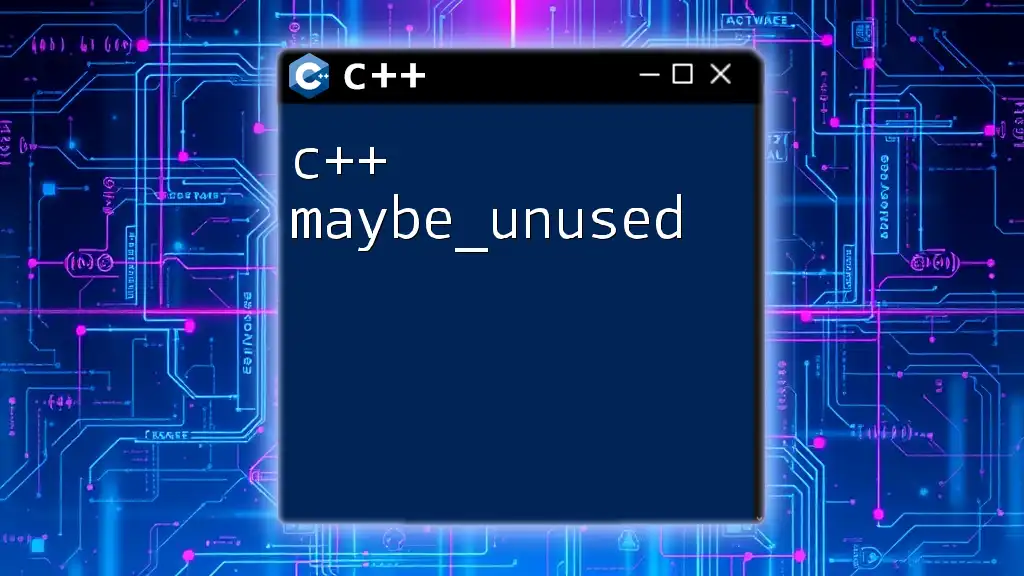
Call to Action
Join our course to master C++ commands and enhance your programming skills. Subscribe for more insights into effective C++ programming and resource management techniques!