C++ move semantics enable the transfer of resources from one object to another, optimizing performance by eliminating unnecessary deep copies.
Here's a simple example:
#include <iostream>
#include <vector>
class Resource {
public:
Resource(size_t size) : data(size) {
std::cout << "Resource acquired\n";
}
Resource(Resource&& other) noexcept : data(std::move(other.data)) {
std::cout << "Resource moved\n";
}
~Resource() {
std::cout << "Resource destroyed\n";
}
private:
std::vector<int> data;
};
int main() {
Resource r1(10); // Resource acquired
Resource r2(std::move(r1)); // Resource moved
return 0;
}
Understanding C++ Move Semantics
What is Move Semantics?
In C++, traditional copy semantics involve creating a duplicate of an object, which can lead to inefficiencies, especially with large resources such as dynamic memory or file handles. Move semantics introduce a new way of transferring ownership of resources from one object to another without copying, allowing for more efficient resource management.
Benefits of Move Semantics
One of the primary benefits of C++ move semantics is performance optimization. By transferring ownership of resources rather than replicating them, move semantics can significantly reduce the overhead associated with object copying. This is particularly beneficial in scenarios involving complex objects or large amounts of data.
Additionally, memory management becomes more efficient. Move semantics minimize unnecessary memory allocations and deallocations, which can lead to lower fragmentation and reduced strain on the memory allocator.
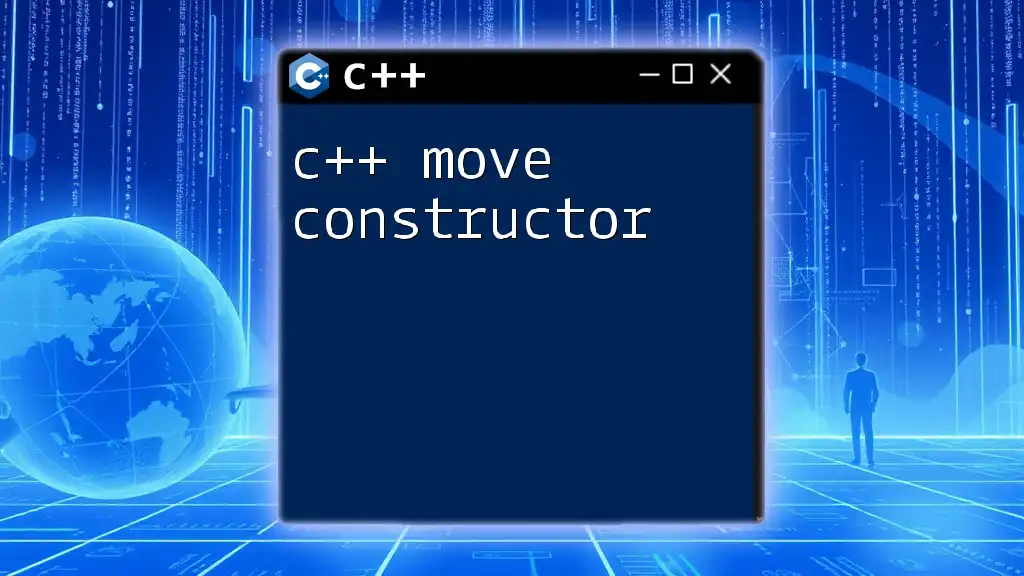
Core Concepts of Move Semantics
Lvalue and Rvalue
To fully grasp move semantics, it’s essential to understand the concepts of lvalues and rvalues:
- Lvalue: An expression that refers to a memory location. For example, variables and arrays are lvalues as they can be assigned new values.
- Rvalue: An expression that does not have a persistent memory location, such as a temporary object or a literal.
The introduction of rvalue references via the syntax `&&` allows developers to capture these temporary objects efficiently, paving the way for move semantics.
The Move Constructor
What is a Move Constructor?
A move constructor is a special constructor that transfers ownership of resources from a temporary rvalue to a new object. This is distinctly different from a copy constructor, which creates a duplicate of an object.
Implementing a Move Constructor
To implement a move constructor, we typically initialize the new object’s resource directly from the source object (the rvalue) and nullify the source's original resource to prevent double-deletion. Here’s an example:
class MyClass {
public:
MyClass(MyClass&& other) noexcept : data(other.data) {
other.data = nullptr; // Nullify the source to avoid dangling pointers
}
// Additional members and methods...
private:
int* data; // Assume this points to a dynamically allocated array or similar
};
In this implementation, the `data` pointer from the `other` object is moved to the new object, and `other.data` is set to `nullptr`. This ensures that when `other` is destroyed, it does not inadvertently try to delete the same resource again.
The Move Assignment Operator
What is a Move Assignment Operator?
Separate from the move constructor, a move assignment operator transfers resources from one existing object to another. This is useful when an object is already initialized and needs to adopt resources from another object.
Implementing a Move Assignment Operator
The implementation mirrors that of the move constructor but adds checks to manage existing resources:
MyClass& operator=(MyClass&& other) noexcept {
if (this != &other) { // Self-assignment check
delete[] data; // Clean up existing resources to prevent memory leaks
data = other.data; // Move ownership
other.data = nullptr; // Nullify the source to avoid dangling references
}
return *this; // Return the current object
}
This function first checks for self-assignment, which is crucial to avoid accidentally deleting the current object’s resources. It releases any owned resources and then transfers the resource from `other`.
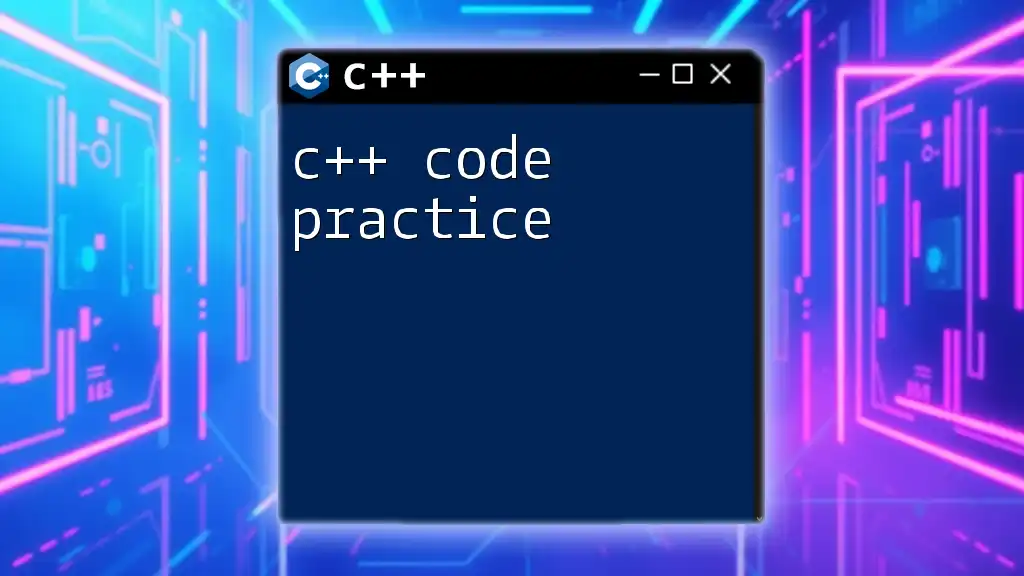
Best Practices in Move Semantics
When to Use Move Semantics
Move semantics is a powerful tool in scenarios where you are dealing with resource-heavy types, like STL containers (e.g., `std::vector`, `std::string`). They allow for efficient reallocation and can minimize object copying, especially when objects are returned from functions.
Exception Safety
When implementing move constructors and move assignment operators, it’s vital to ensure exception safety. Using `noexcept` guarantees that your move operations won’t throw. This allows the compiler to optimize further.
Avoiding Common Pitfalls
A common mistake when using move semantics is accessing moved-from objects. Always ensure that after moving from an object, you do not use it unless it is explicitly reset or reinitialized. This helps avoid undefined behavior and memory issues.
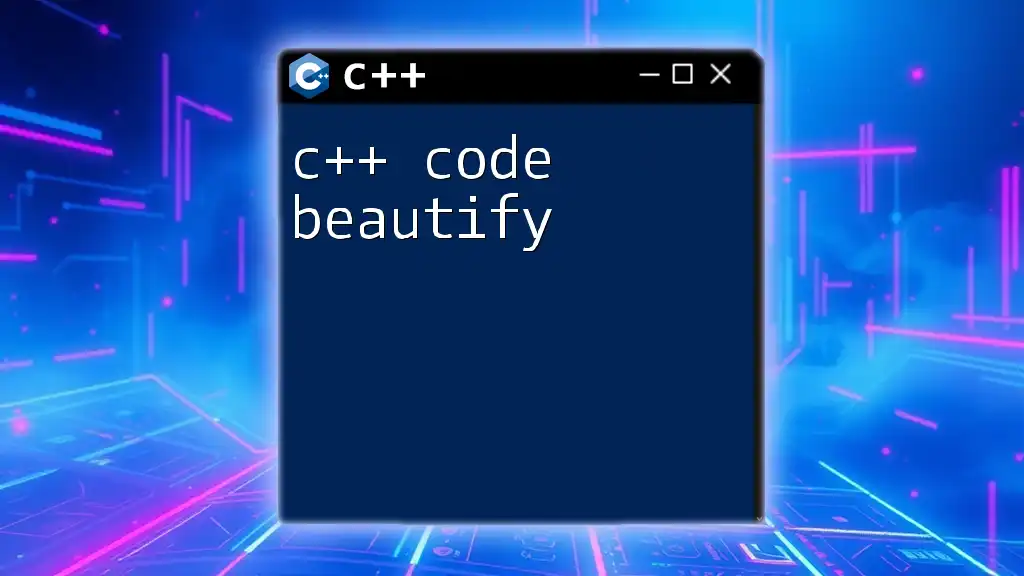
Real-World Applications of Move Semantics
Example: Custom Smart Pointer Implementation
A practical application of move semantics is in custom smart pointers, which manage dynamic memory automatically:
template<typename T>
class UniquePtr {
public:
UniquePtr(T* ptr) : data(ptr) {}
UniquePtr(UniquePtr&& other) noexcept : data(other.data) {
other.data = nullptr; // Prevent dangling pointers
}
~UniquePtr() { delete data; }
private:
T* data; // Pointer to the managed resource
};
In this example, the move constructor enables efficient resource management by enabling ownership transfer without copying the resource itself.
Example: Performance Gains with Standard Containers
Using move semantics can yield considerable performance improvements. When returning a local `std::vector` from a function, for instance, the default behavior without move semantics would require copying the entire vector. Implementing move semantics allows the vector to transfer resources directly, significantly reducing overhead.
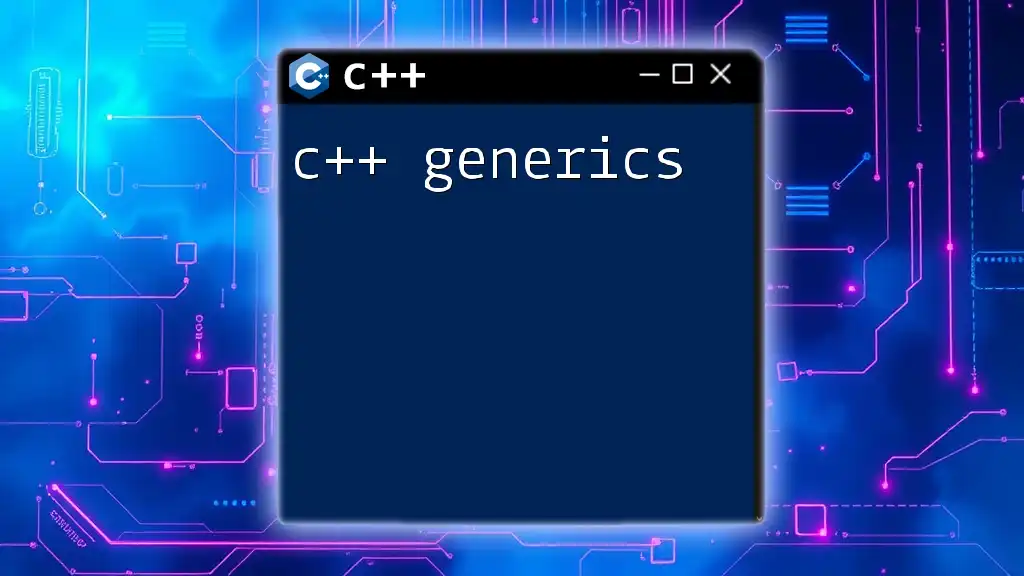
Conclusion
Recap of Move Semantics Importance
C++ move semantics provide a significant advantage in performance and memory efficiency by allowing resources to be transferred instead of copied. Understanding how to implement move constructors and move assignment operators is crucial for optimizing resource management in modern C++ code.
Additional Resources
For those eager to extend their knowledge, numerous resources are available, including C++ standards documentation, online tutorials, and community forums dedicated to C++ programming practices. Engaging with these resources can enhance your grasp of move semantics and improve your coding skills.
Call to Action
We encourage you to implement move semantics in your own C++ projects and share your experiences or questions in the comments. Embrace the power of move semantics and transform how you manage resources in C++.