C++ has evolved through several versions, each introducing new features and improvements, such as C++11's range-based for loops and type inference with `auto`.
Here’s a simple example demonstrating the use of `auto` and a range-based for loop in C++11:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (auto num : numbers) {
std::cout << num << " ";
}
return 0;
}
History of C++
C++ was created by Bjarne Stroustrup in the early 1980s as an enhancement to the C programming language. Its first version followed principles of object-oriented programming, primarily influenced by Simula. The language has undergone several transformations since its inception, driven by the necessity for robustness, efficiency, and the adaptation to modern programming paradigms. Understanding the history of C++ helps us appreciate the distinct versions that have shaped its functionality.
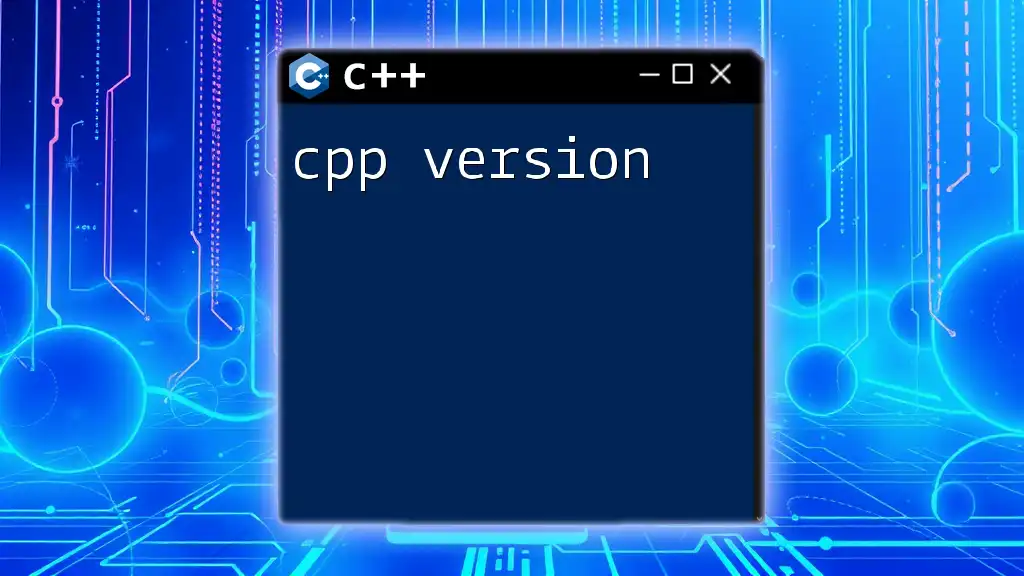
C++98: The First Standard
Overview
C++98 marked the first official standardization of C++ through the International Organization for Standardization (ISO). This version was essential as it established a common foundation to drive widespread adoption and support across different compilers and development environments.
Key Features
C++98 introduced several foundational features that shaped the language, including:
-
Support for Object-Oriented Programming: Classes, inheritance, and polymorphism were core to C++’s design.
-
Templates and the Standard Template Library (STL): These features allowed for generic programming and powerful data structures. The STL provided essential components like vectors, lists, and maps that changed the landscape of C++ programming.
Example Code Snippet:
#include <iostream> #include <vector> int main() { std::vector<int> numbers = {1, 2, 3, 4, 5}; for (int n : numbers) { std::cout << n << " "; } return 0; }
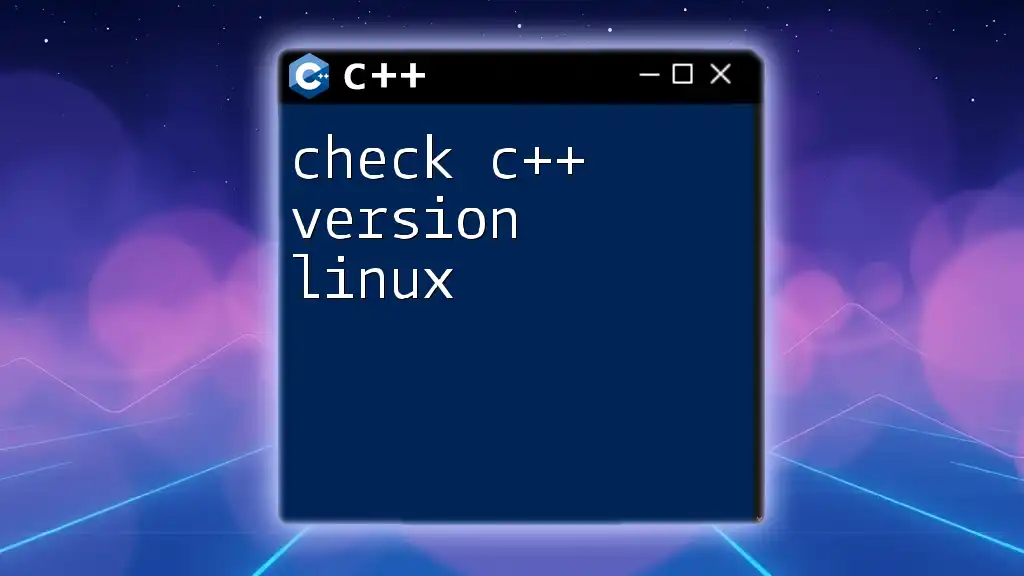
C++03: Minor Updates
Overview
C++03 was a minor update that primarily focused on fixing bugs and clarifying ambiguities found in C++98. While not a major revision, it served as an important step in refining the language.
Key Features
-
Language Corrections: C++03 addressed multiple unclear specifications, ensuring that the language was more consistent and easier to implement across various compilers.
Example of a Corrected Feature:
// C++03 corrected template-related ambiguities
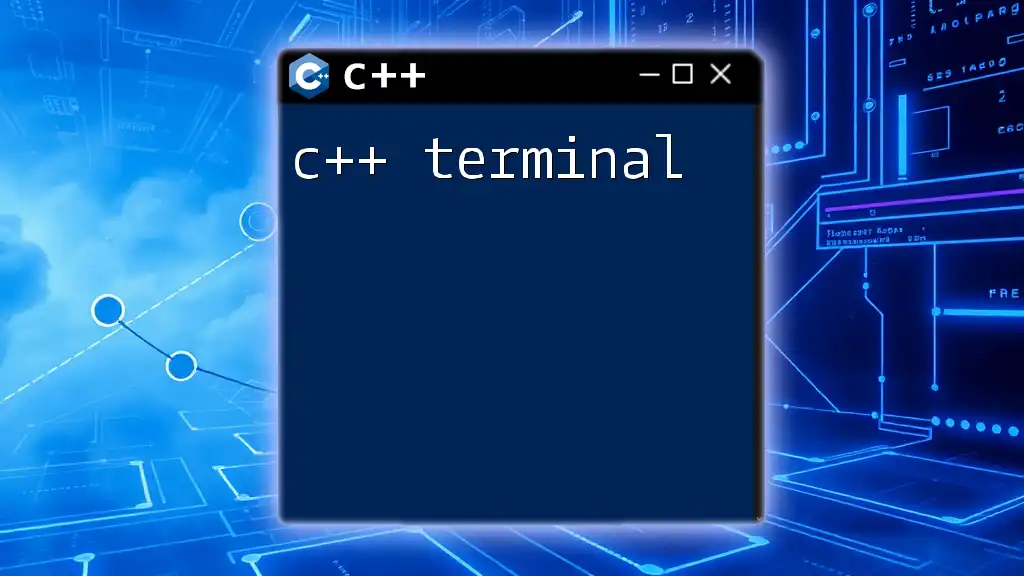
C++11: The Game Changer
Overview
C++11 is widely regarded as a major turning point in C++ programming. This version introduced several new features aimed at improving code efficiency and readability, significantly modernizing the language.
Key Features
-
Lambda Expressions: C++11 introduced lambda expressions, facilitating functional programming styles that improved code succinctness.
Example:
auto add = [](int a, int b) { return a + b; }; std::cout << add(4, 5); // Output: 9
-
Smart Pointers: These new constructs (such as std::unique_ptr and std::shared_ptr) helped automate memory management and reduce memory leaks.
-
Range-Based For Loops: This feature enabled more intuitive iteration over collections, simplifying loop constructions.
-
Multithreading Support: C++11 introduced support for multithreading via the std::thread class, making concurrent programming more robust and accessible.
-
Move Semantics and Rvalue References: This innovation allowed developers to optimize resource management, enhancing performance significantly.
Example:
std::string str1 = "Hello"; std::string str2 = std::move(str1); // str1 is now empty
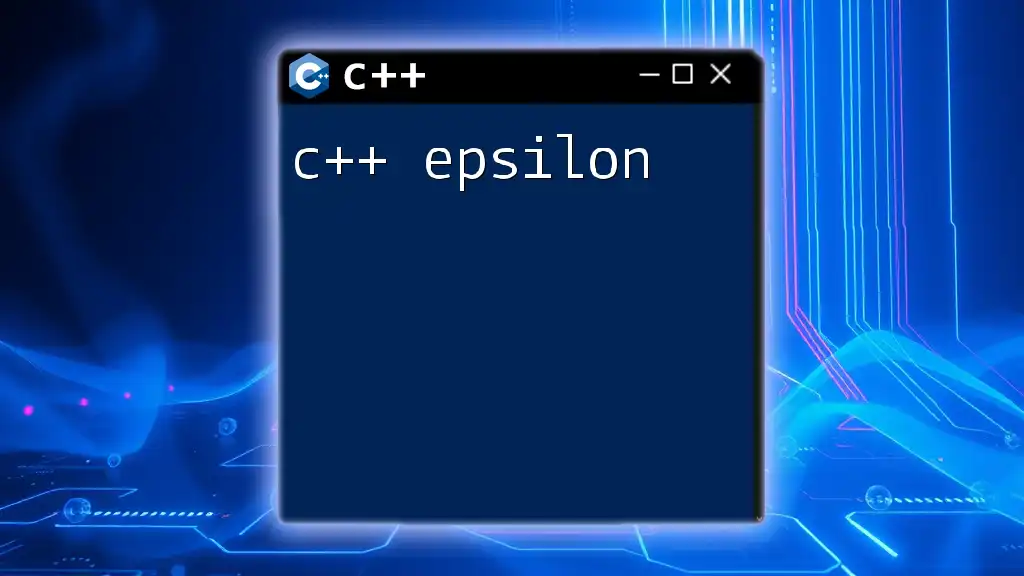
C++14: Incremental Improvements
Overview
C++14 was more of an incremental update rather than a complete redesign. It introduced minor improvements and enhanced usability of C++11 features for developers.
Key Features
-
Generic Lambdas: C++14 allowed lambda expressions to accept parameters of any type without having to specify the type ahead of time.
-
Improved Binary Literals: This version made it easier to work with binary numbers directly.
Example:
auto binary = 0b1010; // Binary representation equivalent to 10 in decimal
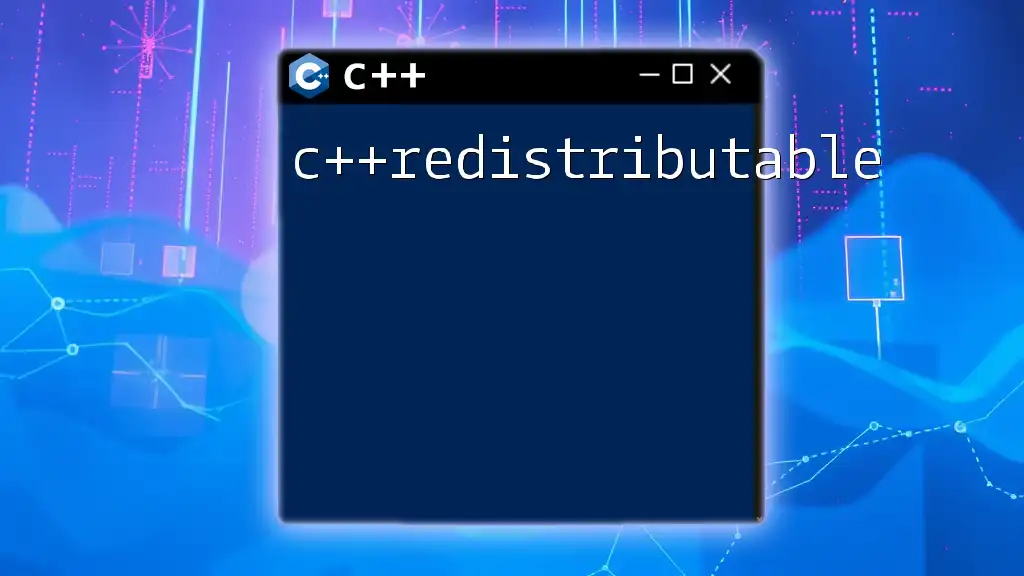
C++17: More Modern Features
Overview
C++17 continued the trend of refinement seen in earlier versions, delivering further enhancements aimed at usability and code simplicity.
Key Features
-
Optional and Variant Types: These feature types allowed developers to represent values that may not be present or could be of multiple types.
-
Structured Bindings: This addition allowed developers to unpack tuple-like objects in a more concise manner.
-
Inline Variables: C++17 allowed the definition of variables as inline, facilitating their usage in header files without violating the One Definition Rule.
Example:
inline int globalVariable = 42; // A variable that can be shared across multiple translation units
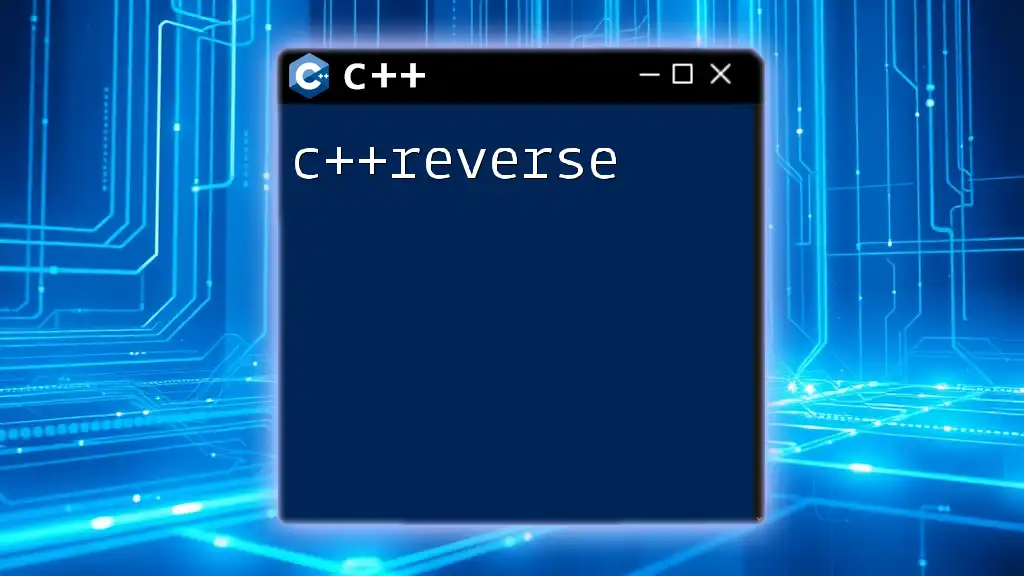
C++20: A New Era
Overview
C++20 ushered in a new era for the language by introducing groundbreaking features that empower developers to write better, more efficient code.
Key Features
-
Concepts for Template Programming: Concepts provided a way to specify template requirements, improving code clarity and error messages.
-
Coroutines for Asynchronous Programming: This feature allowed functions to be paused and resumed, dramatically simplifying asynchronous programming patterns.
-
The "Spaceship" Operator (<=>): This operator offered a concise way to implement comparisons, automatically generating the relevant comparison methods.
Example:
struct Compare { auto operator<=>(const Compare&) const = default; // Automatically defines all comparison operations };
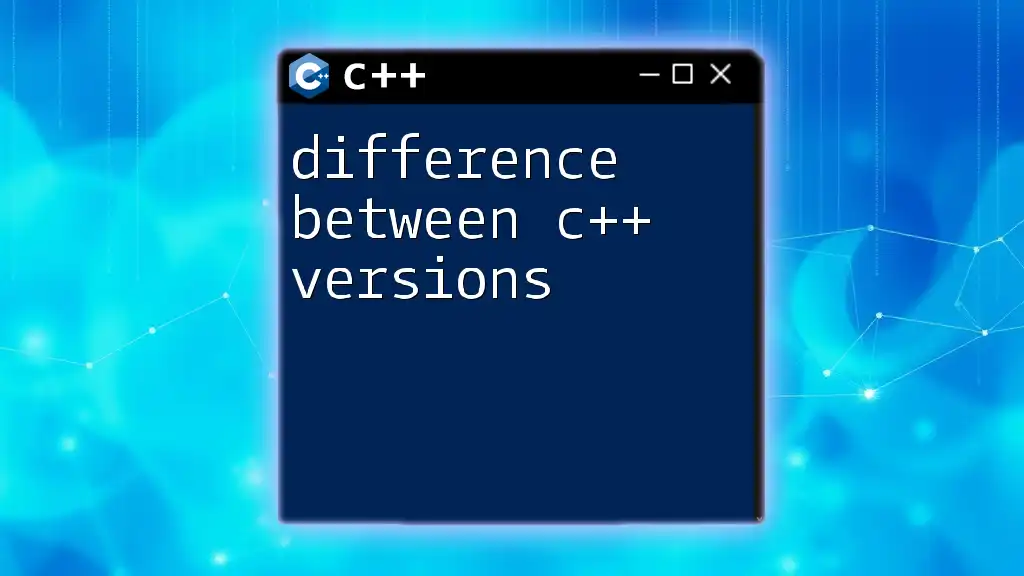
Upcoming C++ Standards
Overview
As we look to the future, discussions have emerged around C++23 and subsequent standards. These upcoming versions are anticipated to introduce further enhancements and capabilities that continue to expand the language's versatility.
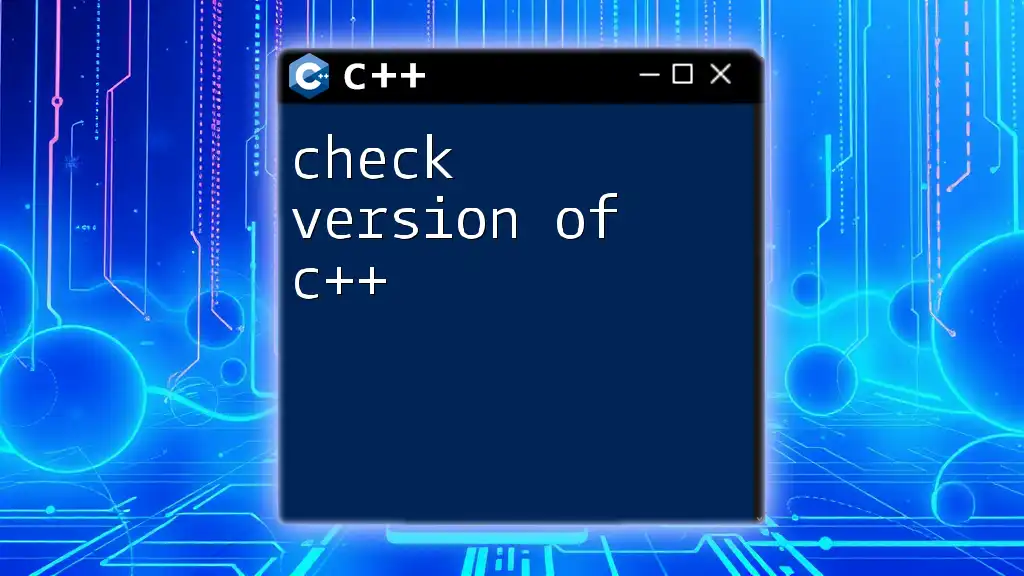
Conclusion
Understanding the various C++ versions is crucial for any programmer seeking to leverage the full power of the language. Each version builds upon its predecessors, introducing features that enhance usability, efficiency, and code architecture. Engaging with these advancements will enable developers to create more robust applications and adapt to the ever-evolving landscape of software development.
Resources for Further Learning
To stay updated on C++ versions, consider exploring official documentation, reputable programming blogs, and comprehensive tutorials that can deepen your understanding of the language.