In C++, epsilon (often represented as `std::numeric_limits<T>::epsilon()`) is a small value that helps determine the precision of floating-point calculations, indicating the difference between 1 and the least value greater than 1 that is representable for a specific type `T`.
#include <iostream>
#include <limits>
int main() {
std::cout << "Epsilon for double: " << std::numeric_limits<double>::epsilon() << std::endl;
return 0;
}
What is Epsilon in C++?
Definition of Epsilon
In the context of C++, epsilon refers to a very small positive number used to define the threshold for comparing floating-point values. Since floating-point arithmetic can result in precision errors, particularly when performing calculations on real numbers, it becomes crucial to establish a margin of error. This margin is known as epsilon.
Why Epsilon Matters in C++
Floating-point numbers cannot always be represented accurately in binary form due to their inherent limitations. These limitations can lead to unexpected behavior when comparing two floating-point numbers directly. For instance, calculations that should theoretically yield the same result may differ slightly due to rounding errors. Thus, epsilon is vital for safe comparisons to prevent flawed logic in your programs.
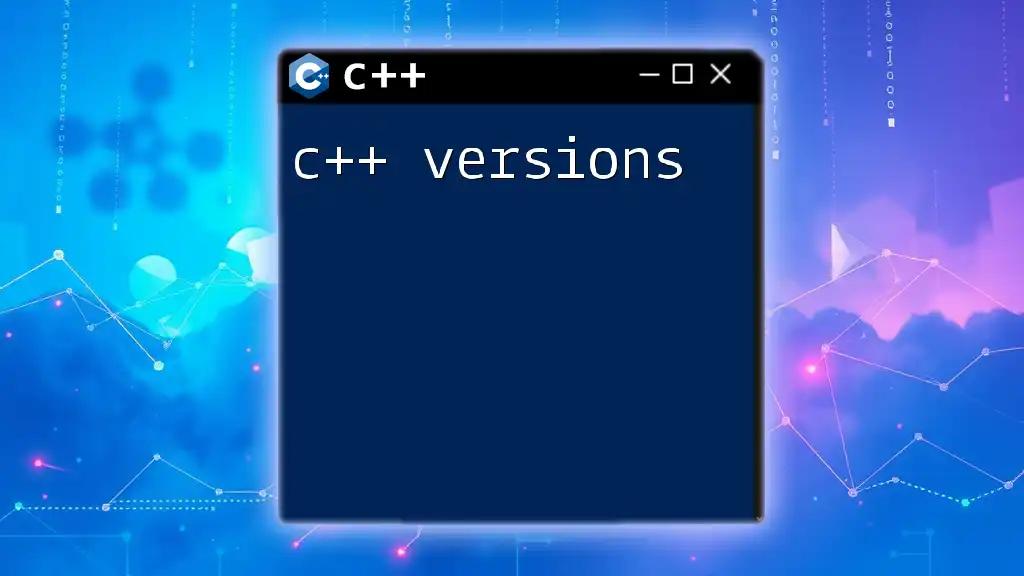
When to Use Epsilon in C++
Common Scenarios for Using Epsilon
Epsilon should be employed in various scenarios to mitigate issues arising from floating-point comparisons:
- Comparing floating-point numbers: Direct equality checks between floating-point numbers can yield incorrect results (e.g., `0.1 + 0.2` might not equal `0.3` exactly).
- Handling geometric calculations: In graphical applications or physics simulations, checking for intersections or overlaps often requires using epsilon to accommodate minor variations.
- Financial applications: Accurate calculations of currency or monetary values, where small discrepancies can lead to significant issues, necessitate the use of epsilon.
Examples of Epsilon Usage
Consider a simple example of comparing two floating-point numbers for equality. Here’s how to implement this using epsilon:
bool areAlmostEqual(double a, double b, double epsilon) {
return fabs(a - b) < epsilon;
}
This function allows for flexibility in floating-point comparisons, ensuring they are treated as equal if their difference falls below a specified threshold (epsilon). This prevents false positives and enhances robustness in numerical computations.

Choosing the Right Epsilon Value
Factors to Consider for Determining Epsilon
When determining the appropriate epsilon value, several factors must be taken into account:
- Data type precision: The choice between `float` and `double` impacts the magnitude of epsilon. Since `double` offers more precision, a smaller epsilon might be necessary.
- Application-specific precision requirements: Different applications may require different levels of precision. A scientific computation may demand tighter tolerances than a gaming application, for example.
Guidelines for Setting Epsilon
As a rule of thumb, the epsilon value should be:
- A small constant that reflects the acceptable tolerance for the given application.
- Adjusted based on the scale of the numbers being compared. For large numbers, you might consider a relative epsilon.
Examples of Epsilon Values
Common choices for epsilon might look like this:
const double EPSILON_FLOAT = 1e-6; // Suitable for float comparisons
const double EPSILON_DOUBLE = 1e-9; // Suitable for double comparisons
These values provide a starting point and can be refined based on testing and specific requirements.

Defining Epsilon in Your Codebase
Standardizing Epsilon Values
To maintain consistency and improve code manageability, it's advisable to declare global epsilon constants. This approach allows the same epsilon value to be used throughout your project seamlessly.
const double EPSILON = std::numeric_limits<double>::epsilon();
This line of code retrieves the machine epsilon for the `double` data type, representing the smallest difference that can occur between two floating-point numbers. Using this standard helps to ensure uniformity and reduce errors.
Utilizing Epsilon in Functions
Functions using a defined epsilon not only improve readability but also follow best coding practices. Here’s an implementation example:
bool isClose(double a, double b) {
return fabs(a - b) < EPSILON;
}
By utilizing standardized functions, code becomes cleaner and more maintainable, making it easier to adapt to any changes in epsilon values.
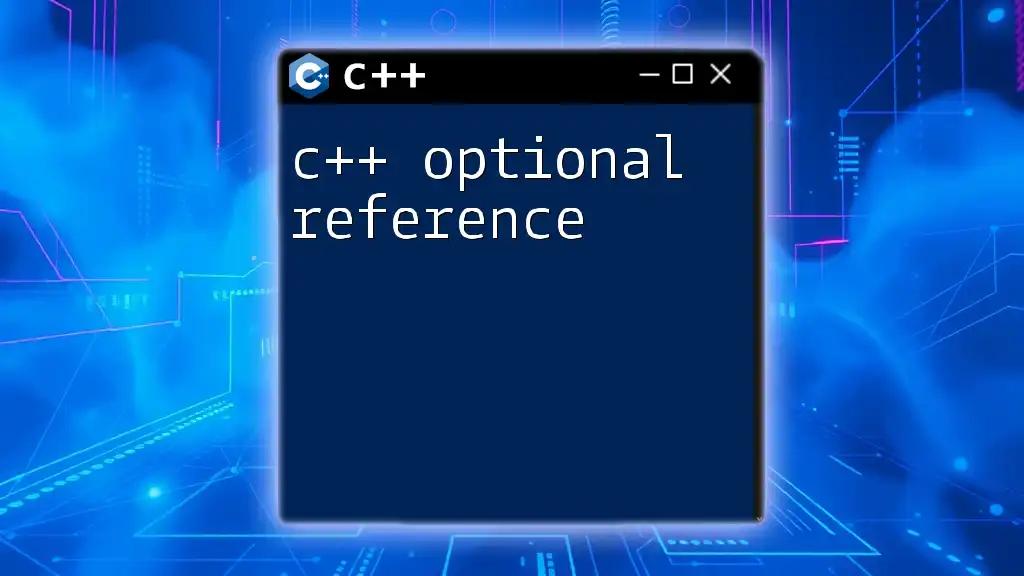
Advanced Topics Surrounding Epsilon
Epsilon in Algorithms and Libraries
Epsilon also plays a crucial role in various algorithms and libraries. For example, sorting algorithms might utilize epsilon to manage precision in comparisons effectively, and computational geometry libraries depend on epsilon to accurately determine point relations and intersections.
Epsilon and Performance
While using epsilon comparisons is essential for accuracy, developers must also consider the performance impact. Excessive floating-point comparisons or incorrectly set epsilon values can lead to unnecessary computations. To minimize performance hits, aim for optimization through smart code refactoring and efficient logic, ensuring that the benefits of using epsilon outweigh any potential downsides.
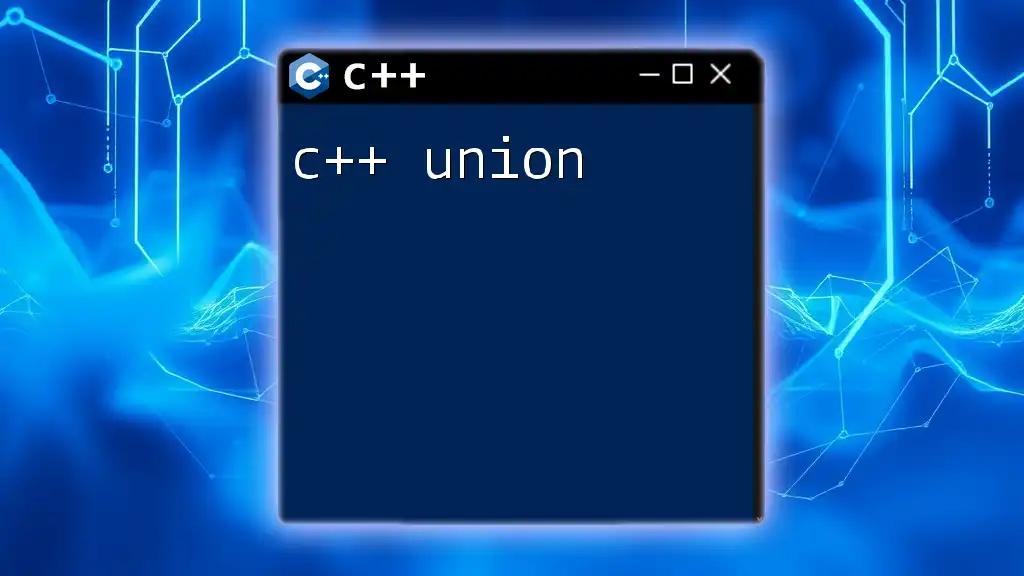
Debugging and Testing Epsilon Comparisons
Common Pitfalls and How to Avoid Them
When implementing epsilon comparisons, many developers encounter common mistakes. These include using too wide a margin, resulting in false outcomes, or neglecting to test for edge cases. Avoidance strategies include thorough testing and employing logging to monitor floating-point behavior throughout execution.
Writing Robust Unit Tests for Epsilon-based Functions
To ensure reliability, writing unit tests for functions that rely on epsilon is crucial. Here’s a simple set of tests for the `areAlmostEqual` function:
#include <cassert>
void testAreAlmostEqual() {
assert(areAlmostEqual(0.1 + 0.2, 0.3, 1e-9) == true);
assert(areAlmostEqual(1.0, 1.0 + 1e-8, 1e-9) == false);
}
These assertions help confirm that the function behaves appropriately under various conditions, ultimately leading to a more robust codebase.
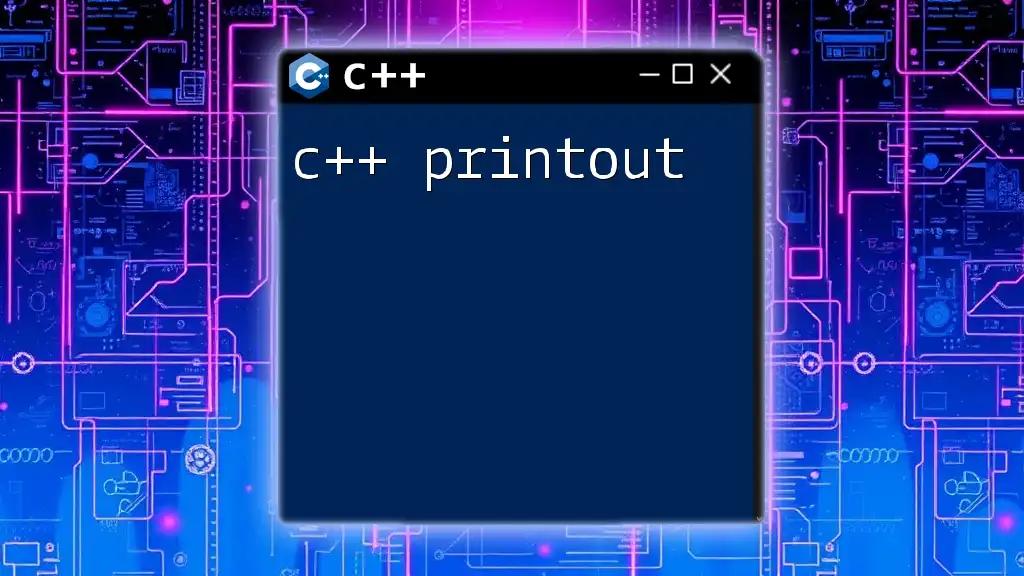
Conclusion
Recap of Key Points on Epsilon
C++ epsilon is an integral concept that helps developers navigate the complexities posed by floating-point arithmetic. Understanding when and how to utilize epsilon ensures accurate comparisons, preventing potential bugs and logical errors in your code.
Further Resources and Reading
For those looking to deepen their understanding of floating-point arithmetic and epsilon usage in C++, consider exploring textbooks, online courses, and participating in community discussions. Engaging with robust materials can provide richer insights and practical applications to apply in your coding endeavors.