In C++, `std::optional` is a feature that allows you to represent an optional value, which can either contain a value or be empty (not set), enhancing safety and expressiveness in your code.
Here's a code snippet demonstrating how to use `std::optional`:
#include <iostream>
#include <optional>
std::optional<int> findValue(bool returnValue) {
if (returnValue) {
return 42; // Returning a value
}
return std::nullopt; // No value
}
int main() {
auto value = findValue(true);
if (value) {
std::cout << "Found value: " << *value << std::endl;
} else {
std::cout << "No value found." << std::endl;
}
return 0;
}
What is `std::optional`?
`std::optional` is a powerful feature introduced in C++17 that allows developers to represent values that may or may not be present. This class template provides an elegant solution to situations where a variable may not have a valid value, eliminating the need for pointers and manual checks.
Key Features
-
Value Semantics: Unlike pointers, which can lead to issues like dangling pointers and null dereferences, `std::optional` embodies value semantics. This means it can be treated just like regular variables without the added complexity of memory management.
-
Nullable Types: `std::optional` serves as a safer alternative to using `nullptr`, providing a clear and explicit way to represent the absence of a value. It raises the readability of your code and minimizes the mental overhead of reasoning about "null" states.
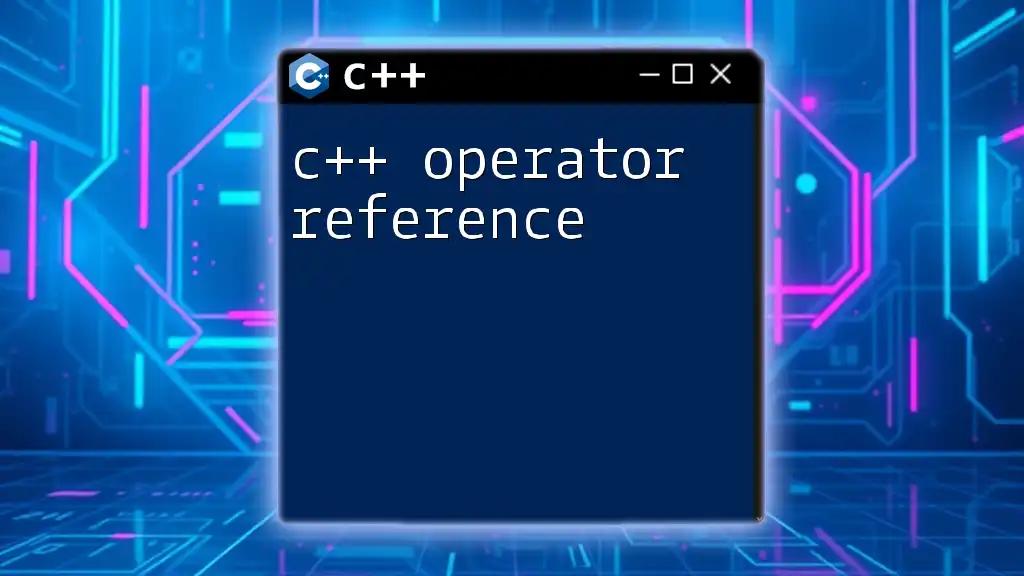
How to Use `std::optional`
Including Necessary Headers
To make use of `std::optional`, you first need to include the correct header file in your C++ program:
#include <optional>
Basic Syntax and Declaration
Declaring an optional variable comes with straightforward syntax. Here's how to define an optional integer:
std::optional<int> optInt; // An empty optional integer
Creating and Assigning Values
You can initialize an optional variable with a value at the time of declaration:
std::optional<int> optInt = 5; // Initializes with value 5
If you aim to create an optional variable and assign it later, you can simply assign it like you would with a regular variable:
std::optional<int> optInt;
optInt = 10; // Assigns value 10
Checking for Value with `has_value()`
Before accessing the value stored in `std::optional`, it's prudent to check if a value exists using the `has_value()` member function. This function returns a boolean indicating whether the optional contains a value or not:
if (optInt.has_value()) {
// Safe to use optInt
}
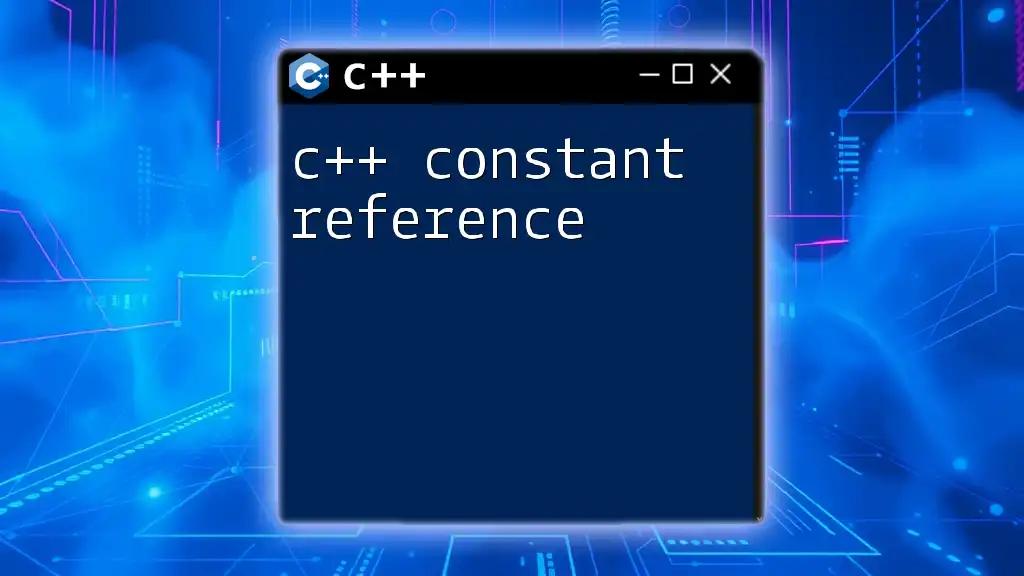
Accessing Values in `std::optional`
Using `value()`
To safely access the underlying value, you can use the `value()` member function. Keep in mind that this function will throw a `std::bad_optional_access` exception if no value is present, making it essential to perform checks beforehand:
try {
int value = optInt.value(); // Throws if optInt is empty
} catch (const std::bad_optional_access&) {
// Handle exception for empty value
}
Using `value_or()`
In situations where you want to provide a default value, `value_or()` can be handy. This method returns the contained value if it exists; otherwise, it returns a specified default:
int value = optInt.value_or(10); // Returns 10 if optInt is empty

Modifying Values in `std::optional`
Assigning New Values
Updating an existing optional is as simple as reassigning it:
optInt = 20; // Updates the value to 20
Resetting an Optional
If you no longer need the value stored in your optional, you can clear it using the `reset()` method. This sets the optional to a state of "not containing a value":
optInt.reset(); // Resets the optional to empty
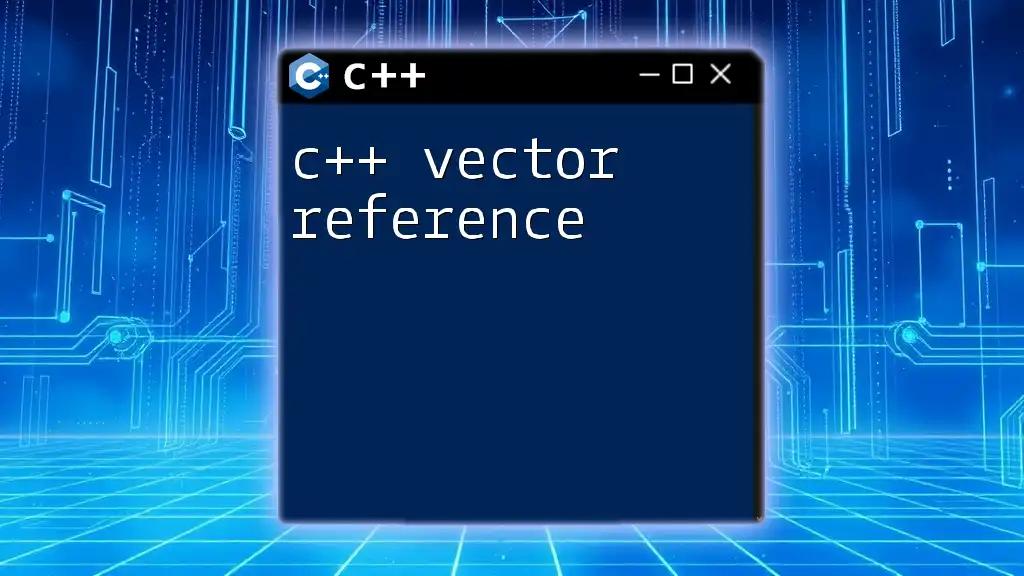
Practical Use Cases of `std::optional`
Function Return Values
One of the most practical applications of `std::optional` is using it as a return type for functions that might not be able to provide a valid response. Consider a function that searches for a value in a vector:
std::optional<int> find_value(std::vector<int>& v, int target) {
for (int value : v) {
if (value == target) return value; // Found the value
}
return std::nullopt; // Indicates value not found
}
In this example, the function can return an `int` wrapped in an `std::optional`, allowing the caller to easily check if the value was found.
Configurable Settings
Another common use case for `std::optional` is within configuration settings where certain parameters may not always be defined by users. By using `std::optional`, you can clearly indicate which parameters are optional, leading to cleaner and more maintainable code.
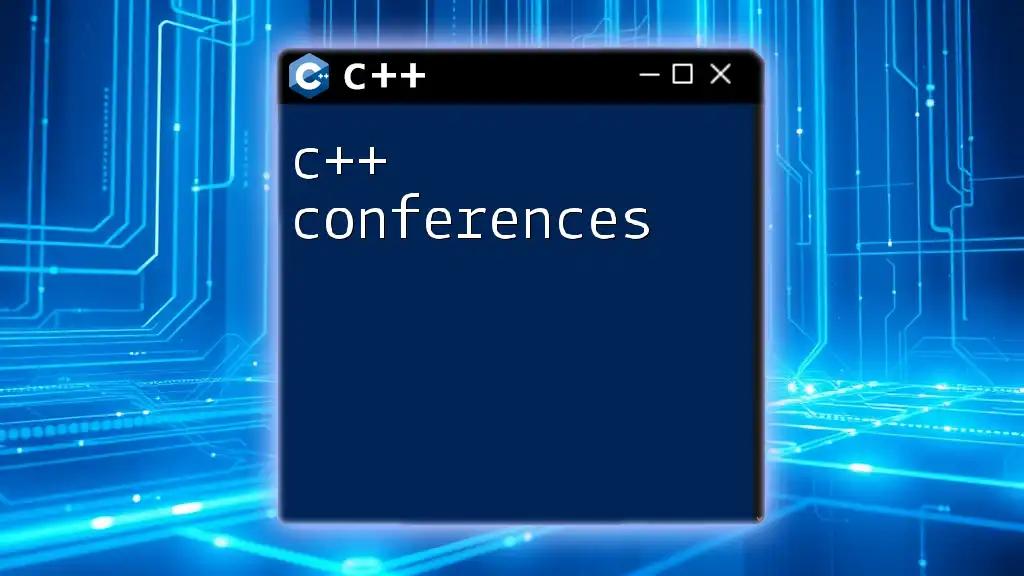
Common Pitfalls and Best Practices
Overusing Optional Types
While `std::optional` is a powerful tool, it's essential to use it judiciously. Overusing optional types in situations where pointers or references would suffice can lead to unnecessary complexity and performance costs. Understanding when each type is most appropriate is crucial for writing efficient code.
Performance Considerations
It's worth noting that `std::optional` introduces some overhead compared to using regular values, especially in scenarios where the optional type is non-trivial. Always consider the performance implications when deciding to use optional types, particularly in performance-critical applications.

Conclusion
In summary, `std::optional` enhances your ability to handle values that may or may not exist in C++, fostering better code readability and reducing error-prone null checks. Its clear semantics and robust functionality make it an invaluable addition to the C++ developer's toolkit.
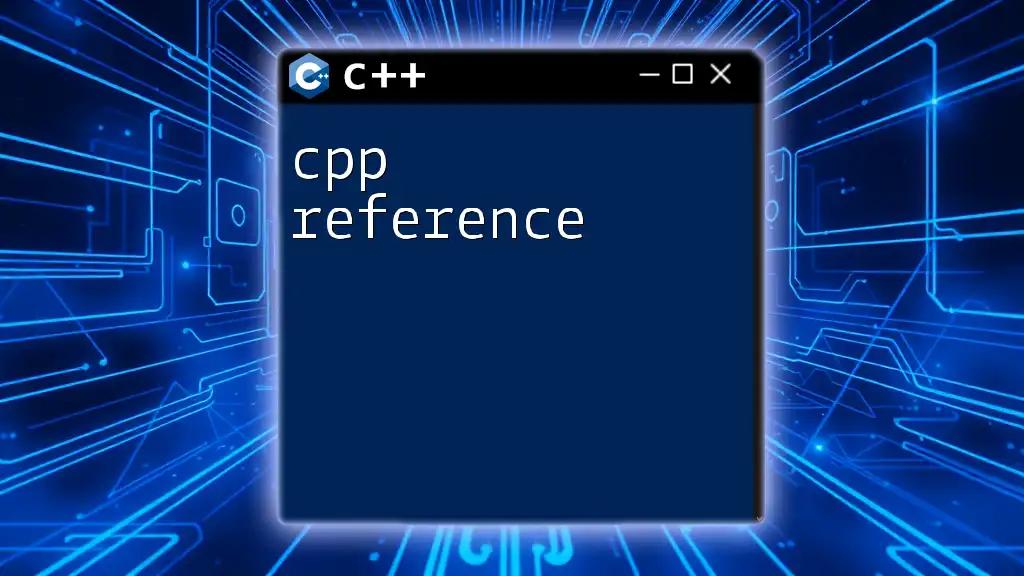
Frequently Asked Questions
What is the difference between `std::optional` and pointers?
Unlike pointers, which can be null or dereferenced to access garbage values if mismanaged, `std::optional` provides a safer interface with built-in checks. The compiler ensures that you deal correctly with the presence or absence of values.
When should I use `std::optional` over default values?
Use `std::optional` when the absence of a value is semantically meaningful. Default values can mask issues where it may be necessary to distinguish between a 'real' value and an absence of value.
Can `std::optional` be used with custom types?
Absolutely! One of the strengths of `std::optional` is its versatility with various data types, including user-defined types. Just be mindful that the types used must be copyable or movable to function effectively with `std::optional`.

Code Repository
For additional examples and exercises related to `std::optional` in C++, feel free to check out [your GitHub repository link here]. This resource will help you solidify your understanding and practical use of C++ optional reference in real-world applications.