In C++, an array of references is not directly supported, but you can create an array of pointers to achieve similar behavior, allowing you to reference multiple objects efficiently.
#include <iostream>
using namespace std;
int main() {
int a = 10, b = 20, c = 30;
int* arr[] = { &a, &b, &c }; // array of pointers to int
for (int i = 0; i < 3; ++i) {
cout << *arr[i] << " "; // dereferencing to access values
}
return 0;
}
Understanding References in C++
What is a Reference?
A reference in C++ can be thought of as an alias for another variable. Once a reference variable is initialized to a variable, it cannot be changed to refer to another variable, making it effectively immutable. This characteristic distinguishes references from pointers, which can point to different variables over their lifetime.
Benefits of using references include:
- Safer Memory Access: References cannot be null, which reduces the risk of dereferencing a null pointer leading to program crashes.
- Cleaner Syntax: Accessing the value via a reference does not require the dereference operator (`*`), making the syntax look cleaner and more natural.
Syntax of References
Declaring a reference is straightforward because it follows the variable type but includes an ampersand (`&`) directly after:
int x = 10;
int& y = x; // y is now a reference to x
In this example, the variable `y` is a reference to `x`. Any changes made to `y` will modify `x` as well, demonstrating the true nature of references.
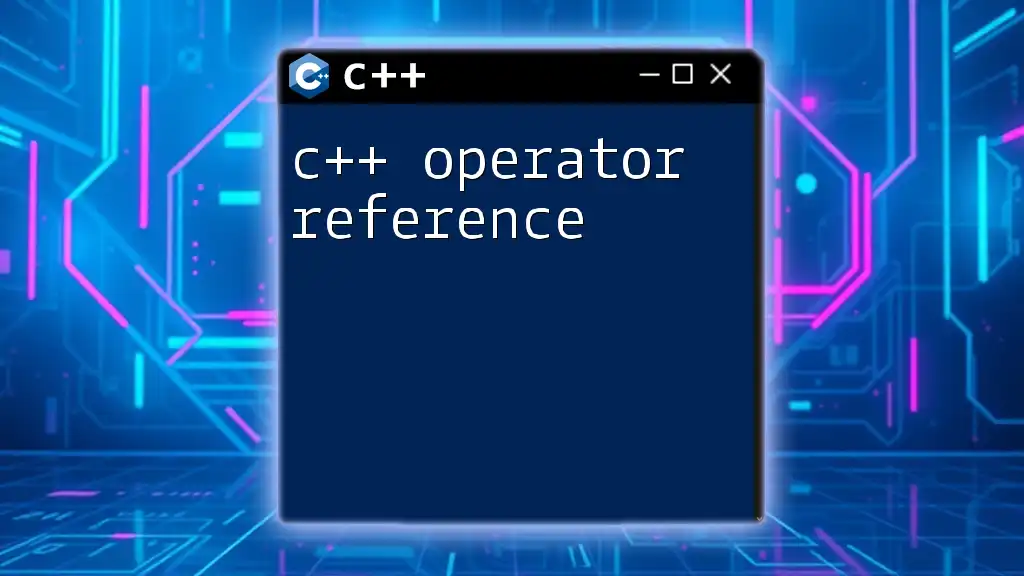
C++ Arrays: A Quick Overview
What is an Array?
An array in C++ is a powerful tool that allows you to store multiple values of the same type in a fixed-size collection. Arrays are stored in contiguous memory locations, which allows for efficient access and manipulation of the data they contain.
Basic Syntax for Arrays can be demonstrated with the following example:
int arr[3] = {1, 2, 3}; // An array of integers with three elements
Here, `arr` is an array containing three integers, initialized to 1, 2, and 3, respectively.
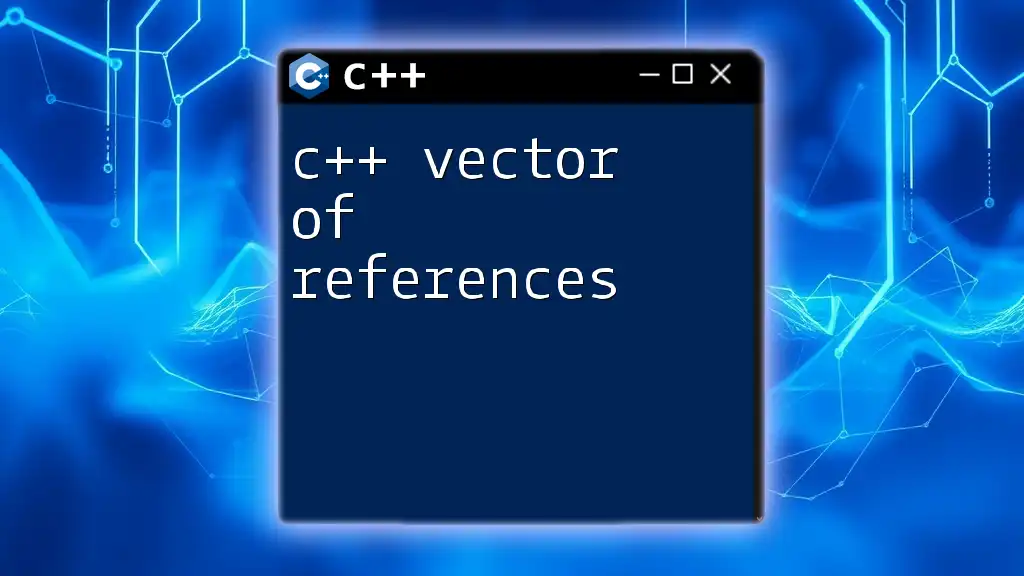
C++ Array of References
What is an Array of References?
The concept of creating an array of references might initially seem appealing. It would allow developers to manage multiple references conveniently. However, C++ does not support direct arrays of references due to the way references operate. They must be initialized upon creation and cannot be reassigned later.
Why use an array of references? The primary motivation would be to simplify data manipulation when you want multiple references to existing variables or objects in a clean manner. However, this functionality is achieved through alternative means.
Declaring an Array of References
While you cannot declare an array of references directly in C++, understanding this limitation is crucial. Attempting to do so will result in a compilation error. For instance, the following code will not compile:
int a = 10, b = 20, c = 30;
int& arr[3] = {a, b, c}; // This is NOT valid
This code fails because C++ does not allow references to be stored in an array as if they were regular variables.
The Right Way: Using Pointers Instead
Fortunately, you can achieve similar functionality by using an array of pointers. Instead of referring to the actual variables with references, you can create pointers that point to the addresses of the variables. This approach provides the desired effect of managing multiple references.
Here’s how you can achieve that:
int a = 10, b = 20, c = 30;
int* arr[3] = {&a, &b, &c}; // Using pointers to refer to the addresses of a, b, and c
In this example, `arr` is an array of three pointers, each pointing to the respective variables `a`, `b`, and `c`. You can manipulate the values through these pointers easily.
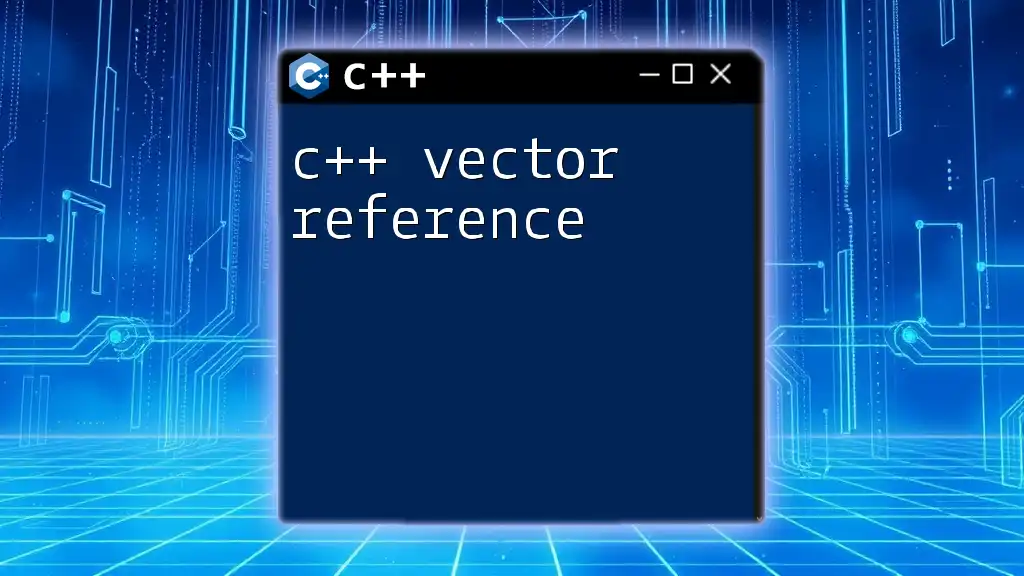
Use Cases for Array of References in C++
Observations in Data Structures
When dealing with complex data structures, you’ll often find that pointers serve well in situations where dynamic allocation is necessary. For instance, if you are implementing a linked list or other complex structures, pointers allow you to create flexible and dynamic connections between data elements.
Performance Considerations
Using an array of pointers allows for efficient operations, particularly when dealing with larger datasets. By working with pointers, you manipulate memory addresses directly rather than copying entire objects or structures, leading to performance benefits. This becomes increasingly relevant in scenarios where duplicating large datasets would incur significant computational overhead.
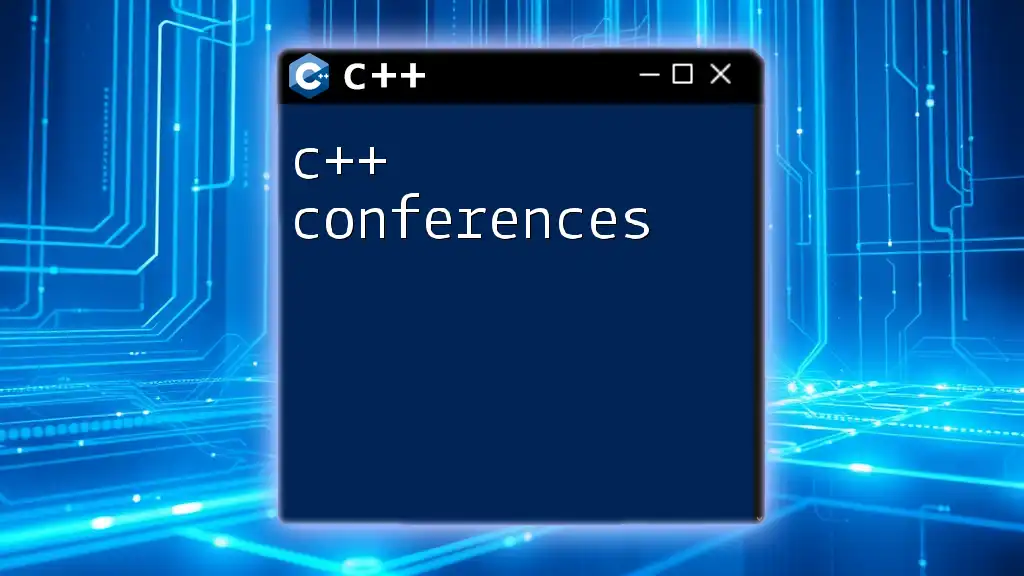
Conclusion
References and arrays are essential concepts within C++, but the direct use of an array of references is not permissible due to language restrictions. Instead, understanding how to effectively use arrays of pointers can achieve similar objectives while providing flexibility and efficiency.
By exploring these nuances, you will enhance your programming skills and master more advanced C++ techniques, ultimately leading to better software design and development practices.
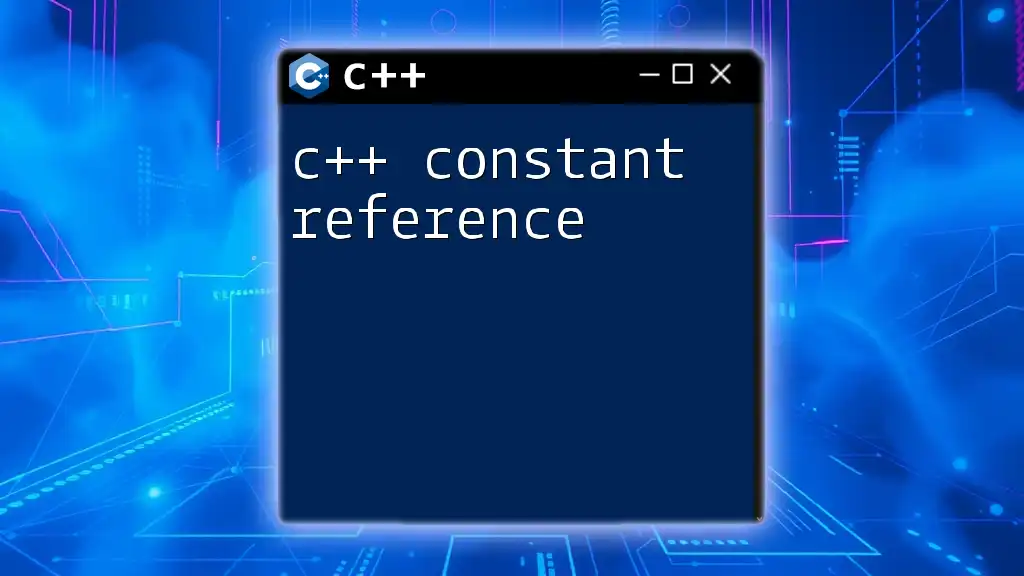
Additional Resources
For anyone interested in diving deeper into C++, numerous texts and online resources focus on advanced programming topics, including pointers, references, and data structures. Engaging in practical exercises will solidify your understanding and provide hands-on experience with these concepts.